Overview
Smart.PivotTable represents a pivot table - a table of statistics that summarizes tabular data that was originally displayed in a Grid or Table.
Getting Started with PivotTable Web Component
Smart UI for Web Components is distributed as smart-webcomponents NPM package. You can also get the full download from our website with all demos from the Download page.Setup the PivotTable
Smart UI for Web Components is distributed as smart-webcomponents NPM package
- Download and install the package.
npm install smart-webcomponents
- Once installed, import the PivotTable module in your application.
<script type="module" src="node_modules/smart-webcomponents/source/modules/smart.pivottable.js"></script>
-
Adding CSS reference
The smart.default.css CSS file should be referenced using following code.
<link rel="stylesheet" type="text/css" href="node_modules/smart-webcomponents/source/styles/smart.default.css" />
- Add the PivotTable tag to your Web Page
<smart-pivot-table id="pivotTable"></smart-pivot-table>
- Create the PivotTable Component
<script type="text/javascript"> Smart('#pivotTable', class { get properties() { return { dataSource: new Smart.DataAdapter({ dataSource: generateData(50), dataFields: [ 'firstName: string', 'lastName: string', 'productName: string', 'quantity: number', 'price: number' ] }), keyboardNavigation: true, columns: [ { label: 'First Name', dataField: 'firstName', dataType: 'string' }, { label: 'Last Name', dataField: 'lastName', dataType: 'string', allowRowGroup: true, rowGroup: true }, { label: 'Product Name', dataField: 'productName', dataType: 'string', allowPivot: true, pivot: true }, { label: 'Quantity', dataField: 'quantity', dataType: 'number', summary: 'sum' }, { label: 'Price', dataField: 'price', dataType: 'number', summary: 'sum', summarySettings: { prefix: '$', decimalPlaces: 2 } } ] }; } }); </script>
Another option is to create the PivotTable is by using the traditional Javascript way:
const pivotTable = document.createElement('smart-pivot-table'); pivotTable.keyboardNavigation = true; pivotTable.columns = [ { label: 'First Name', dataField: 'firstName', dataType: 'string' }, { label: 'Last Name', dataField: 'lastName', dataType: 'string', allowRowGroup: true, rowGroup: true }, { label: 'Product Name', dataField: 'productName', dataType: 'string', allowPivot: true, pivot: true }, { label: 'Quantity', dataField: 'quantity', dataType: 'number', summary: 'sum' }, { label: 'Price', dataField: 'price', dataType: 'number', summary: 'sum', summarySettings: { prefix: '$', decimalPlaces: 2 } } ]; document.body.appendChild(pivotTable);
Smart framework provides a way to dynamically create a web component on demand from a DIV tag which is used as a host. The following imports the web component's module and creates it on demand, when the document is ready. The #pivotTable is the ID of a DIV tag.
import "../../source/modules/smart.pivottable.js"; document.readyState === 'complete' ? init() : window.onload = init; function init() { const pivotTable = new Smart.PivotTable('#pivotTable', { dataSource: new Smart.DataAdapter({ dataSource: generateData(50), dataFields: [ 'firstName: string', 'lastName: string', 'productName: string', 'quantity: number', 'price: number' ] }), keyboardNavigation: true, columns: [ { label: 'First Name', dataField: 'firstName', dataType: 'string' }, { label: 'Last Name', dataField: 'lastName', dataType: 'string', allowRowGroup: true, rowGroup: true }, { label: 'Product Name', dataField: 'productName', dataType: 'string', allowPivot: true, pivot: true }, { label: 'Quantity', dataField: 'quantity', dataType: 'number', summary: 'sum' }, { label: 'Price', dataField: 'price', dataType: 'number', summary: 'sum', summarySettings: { prefix: '$', decimalPlaces: 2 } } ] }); }
- Open the page in your web server.
Pivot Table Concepts, Columns, and Column Roles
Smart.PivotTable is a table of statistics that summarizes the data of a more extensive table (such as from a database, spreadsheet, or business intelligence program). This summary might include sums, averages, or other statistics, which the pivot table groups together in a meaningful way. The PivotTable arranges and rearranges (or "pivots") statistics in order to draw attention to useful information.
PivotTable has two types of columns - the original columns, that correspond to the ones from the grid/table/database the data is retrieved from, and the dynamic columns which are the columns visible in the PivotTable after the data has been processed.
Original Columns
The original columns are defined in the PivotTable's settings by setting the columns property. Here is a sample configuration:
columns: [ { label: 'Last Name', dataField: 'lastName', dataType: 'string', allowRowGroup: true, rowGroup: true }, { label: 'First Name', dataField: 'firstName', dataType: 'string', allowRowGroup: true, rowGroup: true }, { label: 'Product Name', dataField: 'productName', dataType: 'string', allowPivot: true, pivot: true }, { label: 'Quantity', dataField: 'quantity', dataType: 'number', summary: 'sum' }, { label: 'Price', dataField: 'price', dataType: 'number', summary: 'sum', summarySettings: { prefix: '$', decimalPlaces: 2 } } ]
To be visualized in the PivotTable, a column has to have at least one role, configured by setting one of the properties:
- pivot - data from pivot columns is represented as column hierarchy in the PivotTable (see image below - (1)). Whether or not a column can be a pivot column is determined by allowPivot. Pivot columns are optional.
- rowGroup - data from row group columns is represented as rows in the PivotTable (see image below - (2)). Whether a column can be a row group column is determined by allowRowGroup. Row group columns are optional.
- summary - determines the summary function to aggregate the column's data by and produce
dynamic summary columns for each unique pivot data point. There must always be at least one
summary column for the PivotTable to make sense (see image below - (3)). Possible summary
functions are:
- avg - Average
- count - Count
- max - Maximum
- median - Median
- min - Minimum
- product - Product
- stdev - Standard deviation
- stdevp - Standard deviation based on the entire population
- sum - Sum
- var - Variance
- varp - Variance based on the entire population
Dynamic Columns
Once the PivotTable's data source and original columns array are processed, dynamic columns with summarized data are produced and populated. Here is an image that shows a Smart.PivotTable with dynamic columns based on the columns array from above:
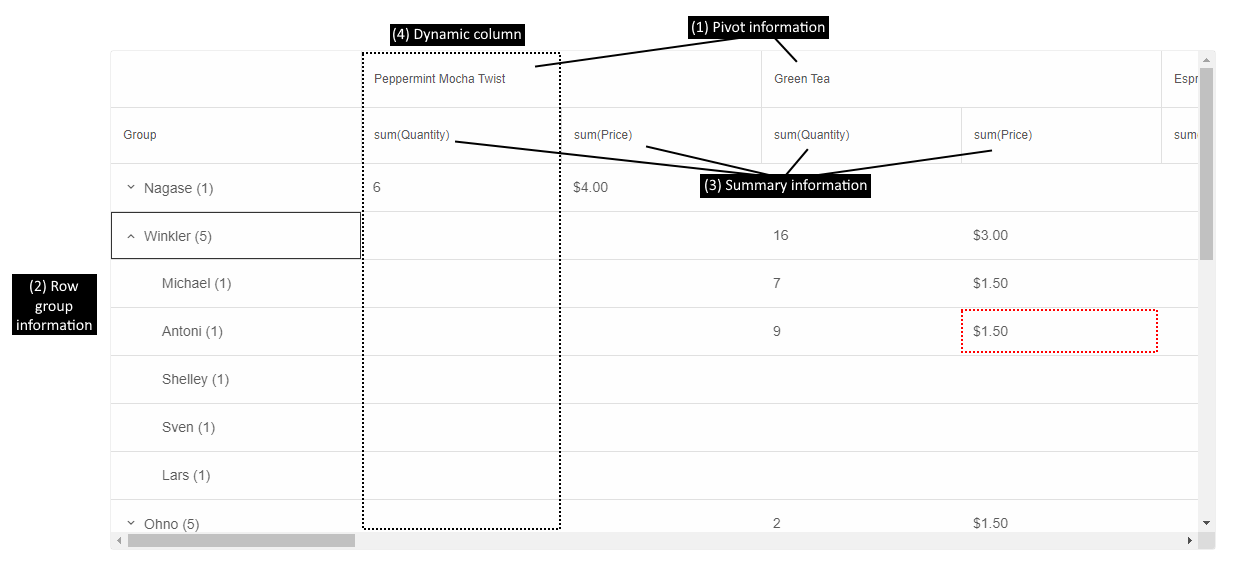
The cell highlighted in red in the image shows the sum of the prices (summary (3)) of all products Green Tea (pivot (1)), purchased by everyone named Antony Winkler (rowGroup (2)).
There can be multiple pivot, row group, and summary columns. The order in which they appear in the PivotTable depends on the order in which they are configured in the columns array. That is why, in this case, rows are first grouped by Last Name, then by First Name and sum(Quantity) is before sum(Price).
Information about all currently displayed dynamic columns can be retrieved by calling the method getDynamicColumns.
Drill Down
Each cell in PivotTable may summarize one or more original data points. If the property drillDown is enabled, the user can double-click a cell to show a Smart.Table in a dialog with all records aggregated in the clicked PivotTable cell.
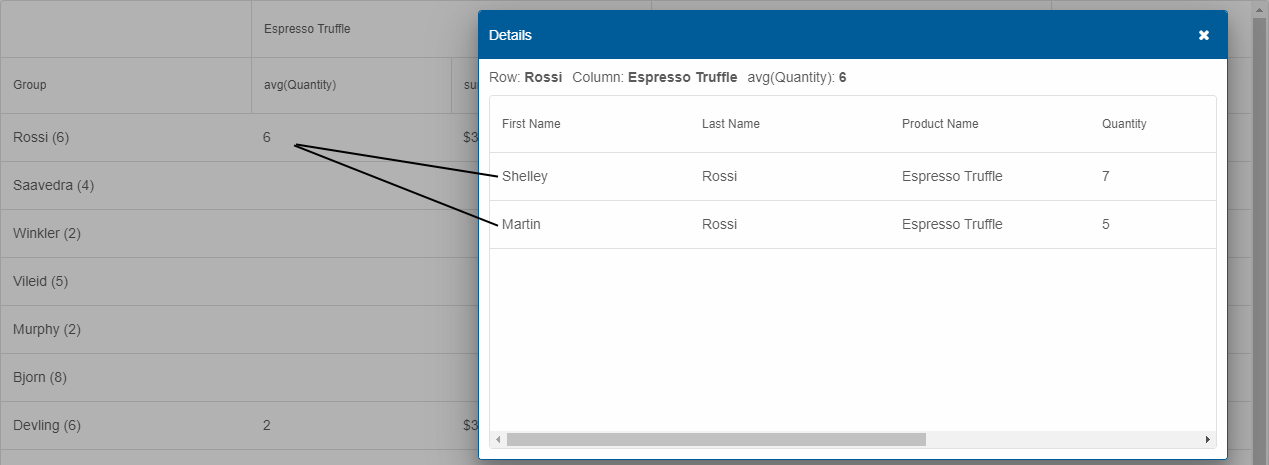
Pivot Table Operations
A variety of operations can be performed on Smart.PivotTable's aggregated data or dynamic columns. Here is an overview:
Column Reorder
Dynamic columns can be reordered by clicking a summary header and dragging with the mouse. Reorder is enabled by setting the columnReorder property to true.
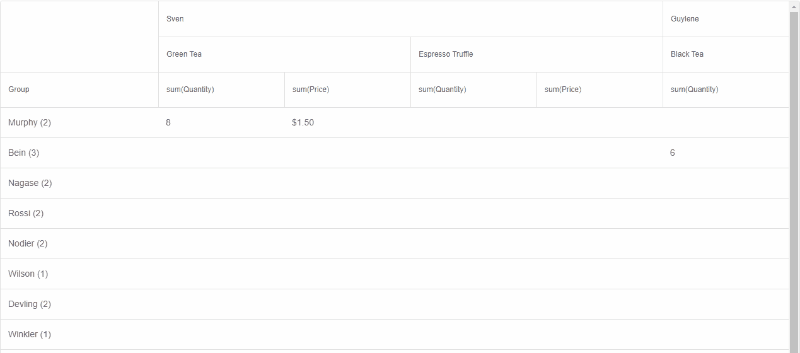
Data Export
The PivotTable's data can be exported to a wide variety of file formats:
- CSV
- HTML
- JSON (depends on the external free plug-in JSZip)
- PDF (depends on the external free plug-in PDFMake)
- TSV
- XLSX
- XML
by calling the element's method exportData, e.g.:
const pivotTable = document.getElementById('pivotTable'); pivotTable.exportData('html', 'pivotTable');
This sample code exports the PivotTable to the file pivotTable.html.
Filtering
The original data bound to Smart.PivotTable can be filtered in order to summarize a more focused set of data points. Filtering can be applied in two ways:
- Programmatically by calling the method addFilter. Related methods are removeFilter and clearFilters.
- Via the "Filters" panel of the PivotTable's designer. It can be displayed as a stand-alone part of the element by setting designer to true or can be opened in a dialog by clicking the "Fields" button of the PivotTable's toolbar. The designer is described in detail in the help topic Pivot Table Toolbar, Designer, and Settings.
Selection
Checkbox row selection can be enabled in Smart.PivotTable by setting the property selection to true. There are two selection modes available:
- selectionMode: 'many' (default) - multiple rows can be selected by clicking the rows or their respective checkboxes.
- selectionMode: 'extended' - single row can be selected by clicking it. Multiple rows can be selected by Ctrl- or Shift-clicking the rows or just clicking their respective checkboxes.
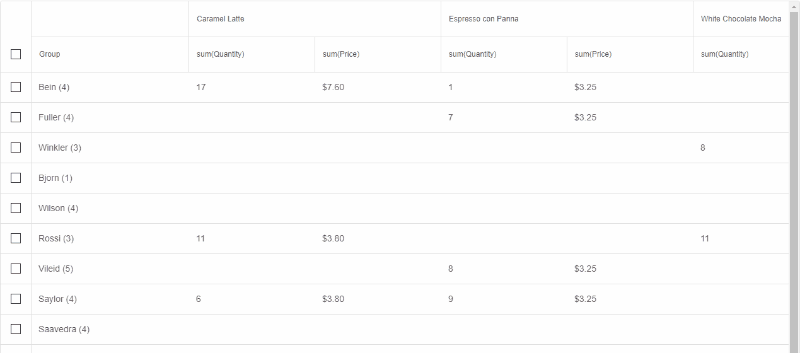
Sorting
The PivotTable can be sorted by its dynamic columns (including the Group column). The sorting behavior is controlled by the property sortMode with the following values:
- 'none' (default) - sorting is disabled.
- 'one' - sorting by one column only is enabled.
- 'many' - sorting by multiple columns is enabled.
Users can apply sorting by clicking on a column's summary header. Clicking multiple times toggles between ascending direction, descending direction, and no sorting.
Alternatively, sorting can be modified with the methods sortBy and clearSort.
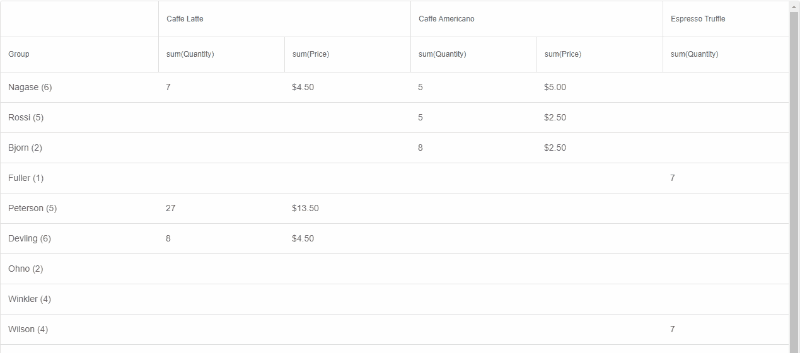
Classic Layout
When there are row group columns in the PivotTable, an alternative layout, inspired by classic, OLAP, pivot tables can be enabled. In this layout, there is a separate column for each level of nesting (row group applied) as opposed to the default, tree grid-like, style with a separate row for each record in the hierarchy. Moreover, in classic layout, the data shown in a table row depends on whether the row is collapsed ("parent" data) or expanded (first "child" data).
The classic, OLAP, layout can be enabled by setting the property groupLayout to 'classic':
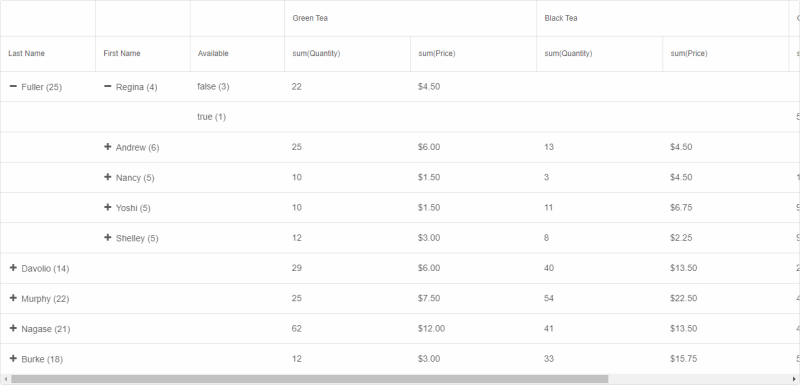
Advanced Features
Additional, advanced, features of Smart.PivotTable are described in the following help topics:
Methods
Smart.PivotTable has the following methods:
- addFilter(dataField, filter) - adds a filter to a specific column.
- clearFilters() - clears applied filters.
- clearSelection() - clears selection.
- clearSort() - clears the PivotTable sorting.
- collapseAllRows() - collapses all rows (when multiple row groups are applied).
- collapseRow(rowId) - collapses a row (when multiple row groups are applied).
- expandAllRows() - expands all rows (when multiple row groups are applied).
- expandRow(rowId) - expands a row (when multiple row groups are applied).
- exportData(dataFormat, fileName, callback) - exports the PivotTable's data.
- getDynamicColumns() - returns the current dynamic pivot columns.
- getSelection() - returns an array of selected row ids.
- refresh() - refreshes the PivotTable.
- removeFilter(dataField) - removes filters applied to a specific column.
- select(rowId) - selects a row.
- sortBy(columnDefinition,sortOrder) - sorts by a summary or group column.
- unselect(rowId) - unselects a row.
Create, Append, Remove, Get/Set Property, Invoke Method, Bind to Event
Create a new element:
const pivotTable = document.createElement('smart-pivot-table');
Append it to the DOM:
document.body.appendChild(pivotTable);
Remove it from the DOM:
pivotTable.parentNode.removeChild(pivotTable);
Set a property:
pivotTable.propertyName = propertyValue;
Get a property value:
const propertyValue = pivotTable.propertyName;
Invoke a method:
pivotTable.methodName(argument1, argument2);
Add Event Listener:
const eventHandler = (event) => { // your code here. }; pivotTable.addEventListener(eventName, eventHandler);
Remove Event Listener:
pivotTable.removeEventListener(eventName, eventHandler, true);
Using with Typescript
Smart Web Components package includes TypeScript definitions which enable strongly-typed access to the Smart UI Components and their configuration.
Inside the download package, the typescript directory contains .d.ts file for each web component and a smart.elements.d.ts typescript definitions file for all web components. Copy the typescript definitions file to your project and in your TypeScript file add a reference to smart.elements.d.ts
Read more about using Smart UI with Typescript.Getting Started with Angular PivotTable Component
Setup Angular Environment
Angular provides the easiest way to set angular CLI projects using Angular CLI tool.
Install the CLI application globally to your machine.
npm install -g @angular/cli
Create a new Application
ng new smart-angular-pivottable
Navigate to the created project folder
cd smart-angular-pivottable
Setup the PivotTable
Smart UI for Angular is distributed as smart-webcomponents-angular NPM package
- Download and install the package.
npm install smart-webcomponents-angular
- Adding CSS reference
The following CSS file is available in ../node_modules/smart-webcomponents-angular/ package folder. This can be referenced in [src/styles.css] using following code.@import 'smart-webcomponents-angular/source/styles/smart.default.css';
Another way to achieve the same is to edit the angular.json file and in the styles add the style."styles": [ "node_modules/smart-webcomponents-angular/source/styles/smart.default.css" ]
If you want to use Bootstrap, Fluent or other themes available in the package, you need to add them after 'smart.default.css'. -
Example with Angular Standalone Components
app.component.html
<div class="demo-description">This demo showcases the basic functionality of smart-pivot-table - a table of statistics that summarizes tabular data.</div> <smart-pivot-table #pivottable id="pivotTable" [dataSource]="dataSource" [freezeHeader]="freezeHeader" [grandTotal]="grandTotal" [keyboardNavigation]="keyboardNavigation" [columns]="columns"></smart-pivot-table>
app.component.ts
import { Component, ViewChild, OnInit, AfterViewInit } from '@angular/core'; import { PivotTableColumn, PivotTableComponent } from 'smart-webcomponents-angular/pivottable'; import { GetData } from './../../common/data'; import { CommonModule } from '@angular/common'; import { RouterOutlet } from '@angular/router'; import { PivotTableModule } from 'smart-webcomponents-angular/pivottable'; @Component({ selector: 'app-root', standalone: true, imports: [CommonModule, PivotTableModule, RouterOutlet], templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements AfterViewInit, OnInit { @ViewChild('pivottable', { read: PivotTableComponent, static: false }) pivottable!: PivotTableComponent; dataSource = new window.Smart.DataAdapter({ dataSource: GetData(50), dataFields: [ 'firstName: string', 'lastName: string', 'productName: string', 'quantity: number', 'price: number', 'date: date' ] }); freezeHeader = true; grandTotal = true; keyboardNavigation = true; columns = [ { label: 'First Name', dataField: 'firstName', dataType: 'string' }, { label: 'Last Name', dataField: 'lastName', dataType: 'string', allowRowGroup: true, rowGroup: true }, { label: 'Product Name', dataField: 'productName', dataType: 'string', allowPivot: true, pivot: true }, { label: 'Quantity', dataField: 'quantity', dataType: 'number', summary: 'sum' }, { label: 'Price', dataField: 'price', dataType: 'number', summary: 'sum', summarySettings: { prefix: '$', decimalPlaces: 2 } }, { label: 'Date Purchased', dataField: 'date', dataType: 'date' } // column is not rendered, because it is neither "pivot", "rowGroup", nor it has "summary" ] as PivotTableColumn[]; ngOnInit(): void { // onInit code. } ngAfterViewInit(): void { // afterViewInit code. this.init(); } init(): void { // init code. } }
-
Example with Angular NGModule
app.component.html
<div class="demo-description">This demo showcases the basic functionality of smart-pivot-table - a table of statistics that summarizes tabular data.</div> <smart-pivot-table #pivottable id="pivotTable" [dataSource]="dataSource" [freezeHeader]="freezeHeader" [grandTotal]="grandTotal" [keyboardNavigation]="keyboardNavigation" [columns]="columns"></smart-pivot-table>
app.component.ts
import { Component, ViewChild, OnInit, AfterViewInit } from '@angular/core'; import { PivotTableColumn, PivotTableComponent } from 'smart-webcomponents-angular/pivottable'; import { GetData } from './../../common/data'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements AfterViewInit, OnInit { @ViewChild('pivottable', { read: PivotTableComponent, static: false }) pivottable!: PivotTableComponent; dataSource = new window.Smart.DataAdapter({ dataSource: GetData(50), dataFields: [ 'firstName: string', 'lastName: string', 'productName: string', 'quantity: number', 'price: number', 'date: date' ] }); freezeHeader = true; grandTotal = true; keyboardNavigation = true; columns = [ { label: 'First Name', dataField: 'firstName', dataType: 'string' }, { label: 'Last Name', dataField: 'lastName', dataType: 'string', allowRowGroup: true, rowGroup: true }, { label: 'Product Name', dataField: 'productName', dataType: 'string', allowPivot: true, pivot: true }, { label: 'Quantity', dataField: 'quantity', dataType: 'number', summary: 'sum' }, { label: 'Price', dataField: 'price', dataType: 'number', summary: 'sum', summarySettings: { prefix: '$', decimalPlaces: 2 } }, { label: 'Date Purchased', dataField: 'date', dataType: 'date' } // column is not rendered, because it is neither "pivot", "rowGroup", nor it has "summary" ] as PivotTableColumn[]; ngOnInit(): void { // onInit code. } ngAfterViewInit(): void { // afterViewInit code. this.init(); } init(): void { // init code. } }
app.module.ts
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { PivotTableModule } from 'smart-webcomponents-angular/pivottable'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, PivotTableModule ], bootstrap: [ AppComponent ] }) export class AppModule { }
Running the Angular application
After completing the steps required to render a PivotTable, run the following command to display the output in your web browser
ng serveand open localhost:4200 in your favorite web browser.
Read more about using Smart UI for Angular: https://www.htmlelements.com/docs/angular-cli/.
Getting Started with React PivotTable Component
Setup React Environment
The easiest way to start with React is to use NextJS Next.js is a full-stack React framework. It’s versatile and lets you create React apps of any size—from a mostly static blog to a complex dynamic application.
npx create-next-app my-app cd my-app npm run devor
yarn create next-app my-app cd my-app yarn run dev
Preparation
Setup the PivotTable
Smart UI for React is distributed as smart-webcomponents-react package
- Download and install the package.
In your React Next.js project, run one of the following commands to install Smart UI PivotTable for ReactWith NPM:
npm install smart-webcomponents-react
With Yarn:yarn add smart-webcomponents-react
- Once installed, import the React PivotTable Component and CSS files in your application and render it.
app.js
import 'smart-webcomponents-react/source/styles/smart.default.css'; import React from "react"; import ReactDOM from 'react-dom/client'; import { PivotTable } from 'smart-webcomponents-react/pivottable'; import { GetData } from './common/data'; const App = () => { const dataSourceSettings = { dataFields: [ 'firstName: string', 'lastName: string', 'productName: string', 'quantity: number', 'price: number', 'date: date' ] }; const dataSource = GetData(50); const freezeHeader = true; const grandTotal = true; const keyboardNavigation = true; const columns = [{ label: 'First Name', dataField: 'firstName', dataType: 'string' }, { label: 'Last Name', dataField: 'lastName', dataType: 'string', allowRowGroup: true, rowGroup: true }, { label: 'Product Name', dataField: 'productName', dataType: 'string', allowPivot: true, pivot: true }, { label: 'Quantity', dataField: 'quantity', dataType: 'number', summary: 'sum' }, { label: 'Price', dataField: 'price', dataType: 'number', summary: 'sum', summarySettings: { prefix: '$', decimalPlaces: 2 } }, { label: 'Date Purchased', dataField: 'date', dataType: 'date' } // column is not rendered, because it is neither "pivot", "rowGroup", nor it has "summary" ]; return ( <div> <div className="demo-description">This demo showcases the basic functionality of PivotTable - a table of statistics that summarizes tabular data.</div> <PivotTable id="pivotTable" dataSourceSettings={dataSourceSettings} dataSource={dataSource} freezeHeader={freezeHeader} grandTotal={grandTotal} keyboardNavigation={keyboardNavigation} columns={columns}> </PivotTable> </div> ); } export default App;
Running the React application
Start the app withnpm run devor
yarn run devand open localhost:3000 in your favorite web browser to see the output.
Setup with Vite
Vite (French word for "quick", pronounced /vit/, like "veet") is a build tool that aims to provide a faster and leaner development experience for modern web projectsWith NPM:
npm create vite@latestWith Yarn:
yarn create viteThen follow the prompts and choose React as a project.
Navigate to your project's directory. By default it is 'vite-project' and install Smart UI for React
In your Vite project, run one of the following commands to install Smart UI PivotTable for ReactWith NPM:
npm install smart-webcomponents-reactWith Yarn:
yarn add smart-webcomponents-reactOpen src/App.tsx App.tsx
import 'smart-webcomponents-react/source/styles/smart.default.css'; import React from "react"; import ReactDOM from 'react-dom/client'; import { PivotTable } from 'smart-webcomponents-react/pivottable'; import { GetData } from './common/data'; const App = () => { const dataSourceSettings = { dataFields: [ 'firstName: string', 'lastName: string', 'productName: string', 'quantity: number', 'price: number', 'date: date' ] }; const dataSource = GetData(50); const freezeHeader = true; const grandTotal = true; const keyboardNavigation = true; const columns = [{ label: 'First Name', dataField: 'firstName', dataType: 'string' }, { label: 'Last Name', dataField: 'lastName', dataType: 'string', allowRowGroup: true, rowGroup: true }, { label: 'Product Name', dataField: 'productName', dataType: 'string', allowPivot: true, pivot: true }, { label: 'Quantity', dataField: 'quantity', dataType: 'number', summary: 'sum' }, { label: 'Price', dataField: 'price', dataType: 'number', summary: 'sum', summarySettings: { prefix: '$', decimalPlaces: 2 } }, { label: 'Date Purchased', dataField: 'date', dataType: 'date' } // column is not rendered, because it is neither "pivot", "rowGroup", nor it has "summary" ]; return ( <div> <div className="demo-description">This demo showcases the basic functionality of PivotTable - a table of statistics that summarizes tabular data.</div> <PivotTable id="pivotTable" dataSourceSettings={dataSourceSettings} dataSource={dataSource} freezeHeader={freezeHeader} grandTotal={grandTotal} keyboardNavigation={keyboardNavigation} columns={columns}> </PivotTable> </div> ); } export default App;
Read more about using Smart UI for React: https://www.htmlelements.com/docs/react/.
Getting Started with Vue PivotTable Component
Setup Vue with Vite
In this section we will introduce how to scaffold a Vue Single Page Application on your local machine. The created project will be using a build setup based on Vite and allow us to use Vue Single-File Components (SFCs). Run the following command in your command linenpm create vue@latestThis command will install and execute create-vue, the official Vue project scaffolding tool. You will be presented with prompts for several optional features such as TypeScript and testing support:
✔ Project name: …If you are unsure about an option, simply choose No by hitting enter for now. Once the project is created, follow the instructions to install dependencies and start the dev server:✔ Add TypeScript? … No / Yes ✔ Add JSX Support? … No / Yes ✔ Add Vue Router for Single Page Application development? … No / Yes ✔ Add Pinia for state management? … No / Yes ✔ Add Vitest for Unit testing? … No / Yes ✔ Add an End-to-End Testing Solution? … No / Cypress / Playwright ✔ Add ESLint for code quality? … No / Yes ✔ Add Prettier for code formatting? … No / Yes Scaffolding project in ./ ... Done.
cdnpm install npm install smart-webcomponents npm run dev
-
Make Vue ignore custom elements defined outside of Vue (e.g., using the Web Components APIs). Otherwise, it will throw a warning about an Unknown custom element, assuming that you forgot to register a global component or misspelled a component name.
Open vite.config.js in your favorite text editor and change its contents to the following:
vite.config.js
import { fileURLToPath, URL } from 'node:url' import { defineConfig } from 'vite' import vue from '@vitejs/plugin-vue' // https://vitejs.dev/config/ export default defineConfig({ plugins: [ vue({ template: { compilerOptions: { isCustomElement: tag => tag.startsWith('smart-') } } }) ], resolve: { alias: { '@': fileURLToPath(new URL('./src', import.meta.url)) } } })
-
Open src/App.vue in your favorite text editor and change its contents to the following:
App.vue
<template> <div class="vue-root"> <div class="demo-description"> This demo showcases the basic functionality of smart-pivot-table - a table of statistics that summarizes tabular data. </div> <smart-pivot-table id="pivotTable"></smart-pivot-table> </div> </template> <script> import { onMounted } from "vue"; import "smart-webcomponents/source/styles/smart.default.css"; import "smart-webcomponents/source/modules/smart.pivottable.js"; export default { name: "app", setup() { onMounted(() => { window.Smart( "#pivotTable", class { get properties() { return { dataSource: new window.Smart.DataAdapter({ dataSource: window.generateData(50), dataFields: [ "firstName: string", "lastName: string", "productName: string", "quantity: number", "price: number", "date: date" ] }), freezeHeader: true, grandTotal: true, keyboardNavigation: true, columns: [ { label: "First Name", dataField: "firstName", dataType: "string" }, { label: "Last Name", dataField: "lastName", dataType: "string", allowRowGroup: true, rowGroup: true }, { label: "Product Name", dataField: "productName", dataType: "string", allowPivot: true, pivot: true }, { label: "Quantity", dataField: "quantity", dataType: "number", summary: "sum" }, { label: "Price", dataField: "price", dataType: "number", summary: "sum", summarySettings: { prefix: "$", decimalPlaces: 2 } }, { label: "Date Purchased", dataField: "date", dataType: "date" } // column is not rendered, because it is neither "pivot", "rowGroup", nor it has "summary" ] }; } } ); }); } }; </script> <style> .smart-pivot-table { height: 800px; } </style>
We can now use the smart-pivot-table with Vue 3. Data binding and event handlers will just work right out of the box.
Running the Vue application
Start the app withnpm run devand open http://localhost:5173/ in your favorite web browser to see the output below:
When you are ready to ship your app to production, run the following:
npm run buildThis will create a production-ready build of your app in the project's ./dist directory.
Read more about using Smart UI for Vue: https://www.htmlelements.com/docs/vue/.