Using with Typescript
Smart Web Components package includes TypeScript definitions which enables strongly-typed access to the Smart UI Components and their configuration.
Installation
npm install smart-webcomponents
The source code for all typescript definitions is available in the typescript folder.
Using the Smart's Redistributable Package
Inside the download package, the typescript directory contains .d.ts file for each web component and a smart.elements.d.ts typescript definitions file for all web components. Copy the typescript definitions file to your project and in your TypeScript file add a reference as demonstrated below:Getting the instance of existing web component
/// <reference path="./typescript/smart.elements.d.ts" /> import { Accordion, AccordionExpandMode } from "./typescript/smart.elements"; window.onload = function() { const accordion: Accordion =document.getElementById('smart-accordion'); accordion.expandMode = AccordionExpandMode.Multiple; }
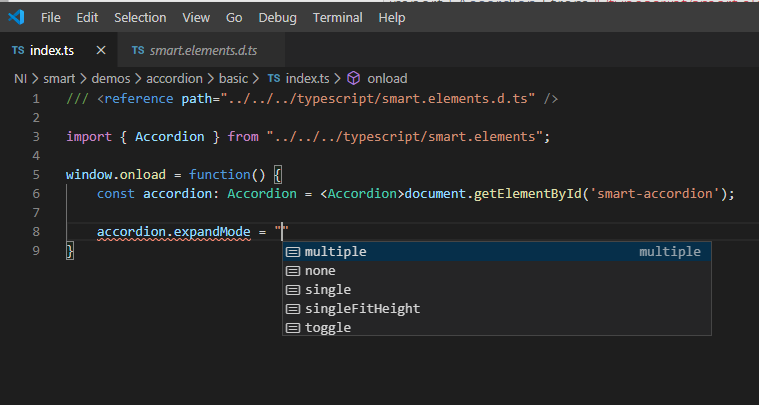
Create and Setup Web Components with Typescript
/// <reference path="../../../typescript/smart.elements.d.ts" /> import { Accordion, AccordionItem } from "../../../typescript/smart.elements"; window.onload = function() { // create a new Smart.Accordion web component. const accordion: Accordion = document.createElement("smart-accordion"); // Add Smart.AccordionItem instances to the Smart.Accordion web component. for(let i = 0; i < 5; i++) { const accordionItem: AccordionItem = document.createElement("smart-accordion-item"); accordionItem.label + "Header " + i; accordionItem.content = "Content " + i; accordion.appendChild(accordionItem); } // Add the Smart.Accordion web component to the DOM. document.body.appendChild(accordion); }
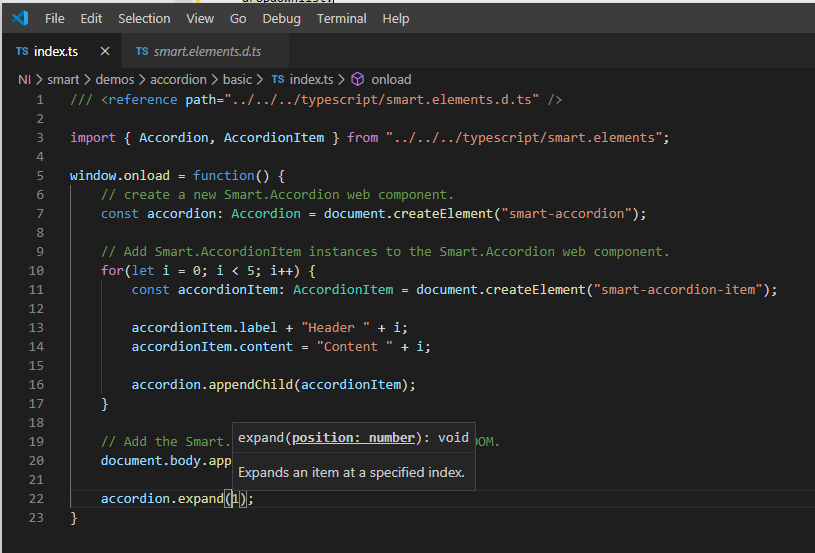