Smart UI with Electron
Electron is a framework for building desktop applications using JavaScript, HTML, and CSS. Smart UI components cab be used both in a standalone Angular application and in an Angular-Electron project.
Setup The Project
The demo will utilize the Angular-Electron template created by Maxime Gris
- In the command prompt, clone the angular-electron repository and install the dependancies:
git clone https://github.com/maximegris/angular-electron.git npm install
- To quickly scaffold the Angular application, install Angular-cli
npm install -g @angular/cli
- Navigate inside your project and install the dependancies:
cd angular-electron/ npm install
- After running npm start, the app will open the desktop electron application
Use Smart UI
When setting up Smart UI, you can either install the whole set of components or only the ones you need
- Run the following command to install the whole Smart framework:
npm install smart-webcomponents-angular
- Import the Grid module in home.module.ts:
import { NgModule } from '@angular/core'; import { CommonModule } from '@angular/common'; import { GridModule } from 'smart-webcomponents-angular/grid'; import { HomeRoutingModule } from './home-routing.module'; import { HomeComponent } from './home.component'; import { SharedModule } from '../shared/shared.module'; @NgModule({ declarations: [HomeComponent], imports: [CommonModule, SharedModule, HomeRoutingModule,GridModule] }) export class HomeModule {}
- Set the HTML template inside home.component.html:
<div> <h3>Smart.Grid in Electron</h3> <smart-grid [columns]="columns" [dataSource]="dataSource" id="grid"></smart-grid> </div>
- Set the column and dataSource inside home.component.ts
import { Component, OnInit, AfterViewInit, ViewEncapsulation } from '@angular/core'; import { Router } from '@angular/router'; import { GridComponent, GridColumn, DataAdapter, Smart } from 'smart-webcomponents-angular/grid'; @Component({ selector: 'app-home', templateUrl: './home.component.html', styleUrls: ['./home.component.scss'], encapsulation: ViewEncapsulation.None }) export class HomeComponent implements AfterViewInit { constructor(private router: Router) { } dataSource = new Smart.DataAdapter( { dataSource: [ { "id": 0, "firstName": "Beate", "lastName": "Wilson", "productName": "Caramel Latte", "price": 3.8, "quantity": 6, "total": 22.799999999999997 }, { "id": 1, "firstName": "Ian", "lastName": "Nodier", "productName": "Caramel Latte", "price": 3.8, "quantity": 8, "total": 30.4 }, { "id": 2, "firstName": "Petra", "lastName": "Vileid", "productName": "Green Tea", "price": 1.5, "quantity": 2, "total": 3 }, { "id": 3, "firstName": "Mayumi", "lastName": "Ohno", "productName": "Caramel Latte", "price": 3.8, "quantity": 2, "total": 7.6 }, { "id": 4, "firstName": "Mayumi", "lastName": "Saylor", "productName": "Espresso con Panna", "price": 3.25, "quantity": 4, "total": 13 }, { "id": 5, "firstName": "Regina", "lastName": "Fuller", "productName": "Caffe Americano", "price": 2.5, "quantity": 4, "total": 10 }, { "id": 6, "firstName": "Regina", "lastName": "Burke", "productName": "Caramel Latte", "price": 3.8, "quantity": 8, "total": 30.4 }, { "id": 7, "firstName": "Andrew", "lastName": "Petersen", "productName": "Caffe Americano", "price": 2.5, "quantity": 6, "total": 15 }, { "id": 8, "firstName": "Martin", "lastName": "Ohno", "productName": "Espresso con Panna", "price": 3.25, "quantity": 3, "total": 9.75 }, { "id": 9, "firstName": "Beate", "lastName": "Devling", "productName": "Green Tea", "price": 1.5, "quantity": 9, "total": 13.5 }, { "id": 10, "firstName": "Sven", "lastName": "Devling", "productName": "Espresso Truffle", "price": 1.75, "quantity": 6, "total": 10.5 } ], dataFields: [ 'id: number', 'firstName: string', 'lastName: string', 'productName: string', 'quantity: number', 'price: number', 'total: number' ] }) columns: GridColumn[] = [ { label: 'First Name', dataField: 'firstName', columnGroup: 'name' }, { label: 'Last Name', dataField: 'lastName', columnGroup: 'name' }, { label: 'Product', dataField: 'productName', columnGroup: 'order' }, { label: 'Quantity', dataField: 'quantity', columnGroup: 'order' }, { label: 'Unit Price', dataField: 'price', cellsFormat: 'c2', columnGroup: 'order' }, { label: 'Total', dataField: 'total', cellsFormat: 'c2', columnGroup: 'order' } ] ngOnInit(): void { } ngAfterViewInit(): void { } }
- Finally, set the CSS style inside angular.json:
"projects": { "architect": { "build": { .... "styles": [ "src/styles.scss", "node_modules/smart-webcomponents-angular/source/styles/smart.default.css" ] } .... } .... }
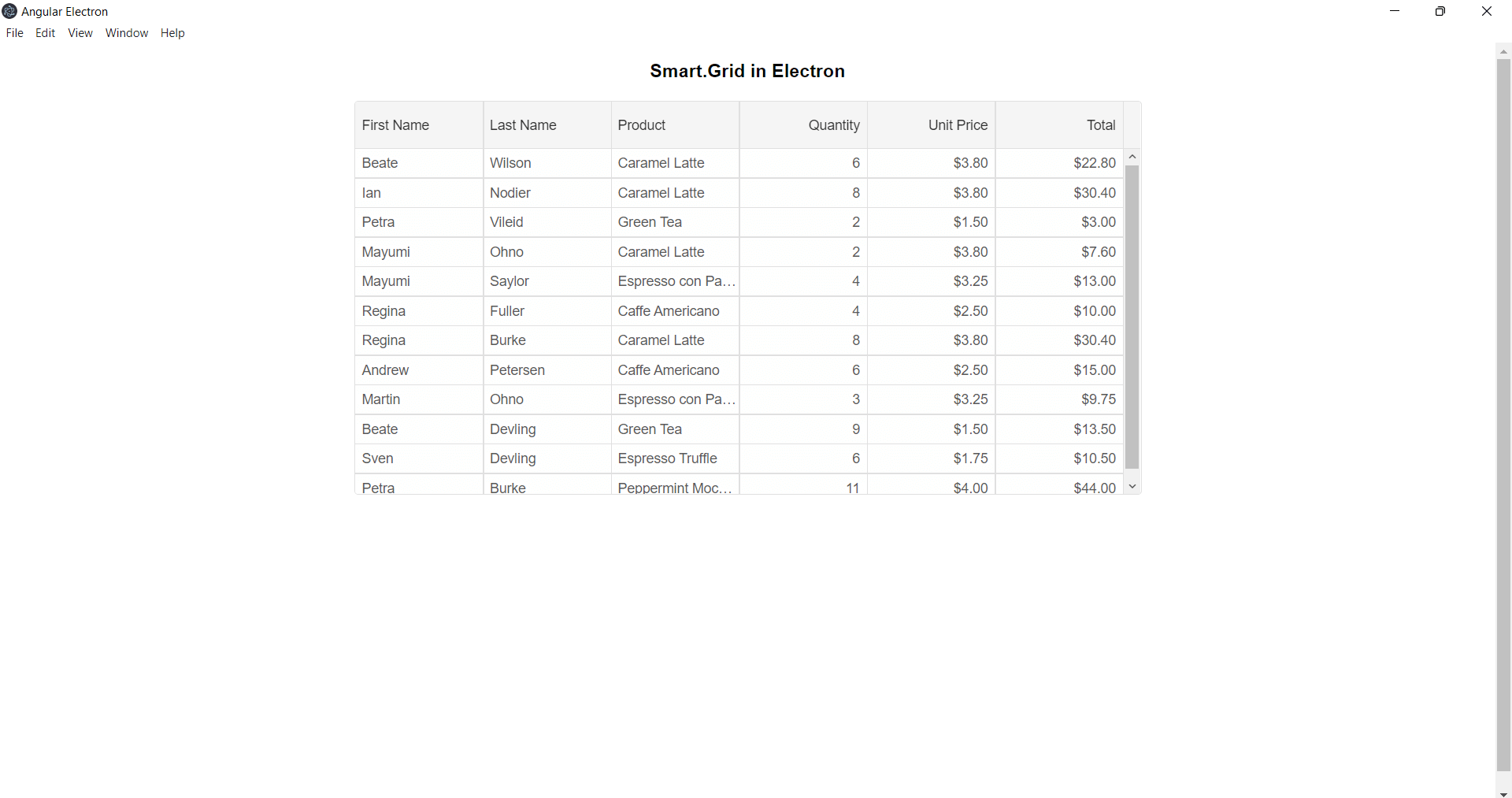