Build Dynamic Smart Table in Angular 10 with ngFor
The *ngFor directive (predefined by Angular) lets you loop through data.
In this tutorial, we will demonstrate how to build a dynamic Smart Table with Angular using the *ngFor directive.
First, you can follow our Getting Started with Angular Cli Tutorial for setting up your project and installing the needed dependencies.
After the project is created you can open the app.component.ts.
Here you can inject and use a data service for getting the data for the table using the HttpClient API.
For the purpose of this tutorial we will be using the generateData method.
Our data-service.ts file will look like this:
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root', }) export class DataService { constructor() { } generateData(rowscount = 100): any[] { var data: any[] = []; var firstNames = [ "Andrew", "Nancy", "Shelley", "Regina", "Yoshi", "Antoni", "Mayumi", "Ian", "Peter", "Lars", "Petra", "Martin", "Sven", "Elio", "Beate", "Cheryl", "Michael", "Guylene"]; var lastNames = [ "Fuller", "Davolio", "Burke", "Murphy", "Nagase", "Saavedra", "Ohno", "Devling", "Wilson", "Peterson", "Winkler", "Bein", "Petersen", "Rossi", "Vileid", "Saylor", "Bjorn", "Nodier"]; var productNames = [ "Black Tea", "Green Tea", "Caffe Espresso", "Doubleshot Espresso", "Caffe Latte", "White Chocolate Mocha", "Caramel Latte", "Caffe Americano", "Cappuccino", "Espresso Truffle", "Espresso con Panna", "Peppermint Mocha Twist"]; var priceValues = [ "2.25", "1.5", "3.0", "3.3", "4.5", "3.6", "3.8", "2.5", "5.0", "1.75", "3.25", "4.0"]; for (var i = 0; i < rowscount; i++) { var row = {}; var productindex = Math.floor(Math.random() * productNames.length); var price = parseFloat(priceValues[productindex]); var quantity = 1 + Math.round(Math.random() * 10); row["firstName"] = firstNames[Math.floor(Math.random() * firstNames.length)]; row["lastName"] = lastNames[Math.floor(Math.random() * lastNames.length)]; row["name"] = row["firstName"] + " " + row["lastName"]; row["productName"] = productNames[productindex]; row["price"] = price; row["quantity"] = quantity; row["total"] = price * quantity; data[i] = row; } return data; } }
Now you can open the app.component.ts file and add the following code:
import { Component, OnInit } from '@angular/core'; import { DataService } from './data-service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { tableData = []; constructor(private dataService: DataService) { } ngOnInit() { this.tableData = this.dataService.generateData(); } }
We injected the data service and loaded the table's data.
Building HTML Table using ngFor
HTML table is pretty easy element to create using table tag.
Every single table row is defined with the tr tag. A table header is created with the th tag. By default, table headings are set to bold and centered and a table cell is created using td tag.
Open the app.component.html file and put the following code:
<smart-table #table id="table" style="width: 850px;"> <table> <thead> <tr> <th scope="col">Name</th> <th scope="col">Product Name</th> <th scope="col">Quantity</th> <th scope="col">Price</th> <th scope="col">Total</th> </tr> </thead> <tbody> <tr *ngFor="let row of tableData"> <td>{{ row.name }}</td> <td>{{ row.productName }}</td> <td>${{ row.price }}</td> <td>{{ row.quantity }}</td> <td>{{ row.total }}</td> </tr> </tbody> </table> </smart-table>
In the above HTML table we are using the ngFor directive to iterate the tableData variable and display a tr row element for each entry.
Conclusion
Now when you run serve you will get this result:
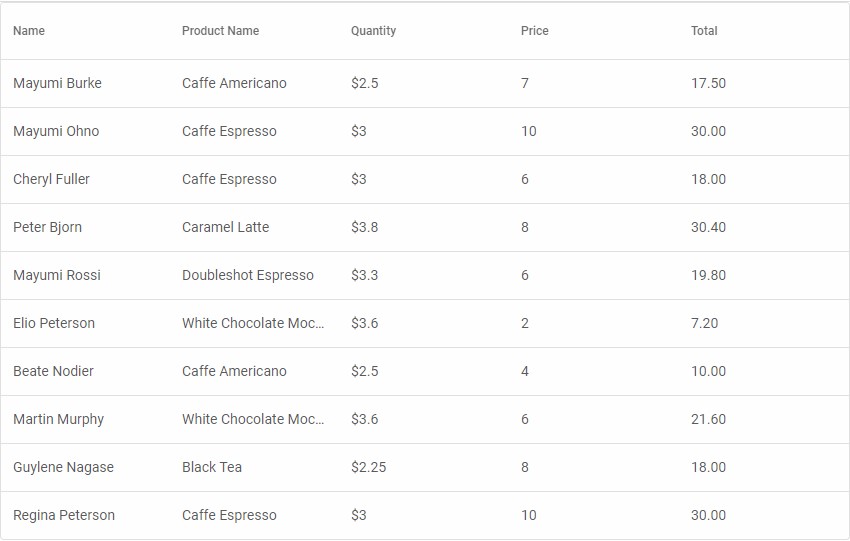
In this tutorial, we've learned how to create a dynamic Smart Table with Angular using the *ngFor directive.