Export Data
Smart.Grid can export the visualized grid into a new file, while applying the current filtering, sorting and grouping rules. The following file formats are supported:
- XLSX
- JSON
- XML
- CSV
- TSV
- HTML
- JPEG
- PNG
Required Files
To enable data export, it is necessary to include additional JavaScript files in your project.
Open the _Layout.cshtml(.NET 6) or _Host.cshtml file (server-side Blazor) or wwwroot/index.html (client-side WebAssembly Blazor)
and add the following JS files:
<script src="_content/Smart.Blazor/js/assets/jszip.min.js"></script> <script src="_content/Smart.Blazor/js/assets/pdfmake.min.js"></script> <script src="_content/Smart.Blazor/js/assets/vfs_fonts.js"></script> <script src="_content/Smart.Blazor/js/assets/html2canvas.min.js"></script>
Export Example
Create a new Button which calls the custom exportGrid
function when clicked.
The file format is set as a parameter of the ExportData
function
@inject WeatherForecastService ForecastService <Grid @ref="grid" DataSource="@forecast"> <Columns> <Column DataField="Date" Label="Date" DataType="date" CellsFormat="M" AllowReorder="true"></Column> <Column DataField="TemperatureC" Label="Temp. (C)" AllowReorder="true"></Column> <Column DataField="TemperatureF" Label="Temp. (F)" AllowReorder="true"></Column> <Column DataField="Summary" Label="Summary" AllowReorder="true"></Column> </Columns> </Grid> <Button OnClick="@exportGrid">Export Data</Button> @code { Grid grid; private void exportGrid(){ grid.ExportData("xlsx"); } private WeatherForecast[] forecast; protected override async Task OnInitializedAsync() { forecast = await ForecastService.GetForecastAsync(DateTime.Now); } }
The demo above generates the following .xlsx Excel file:
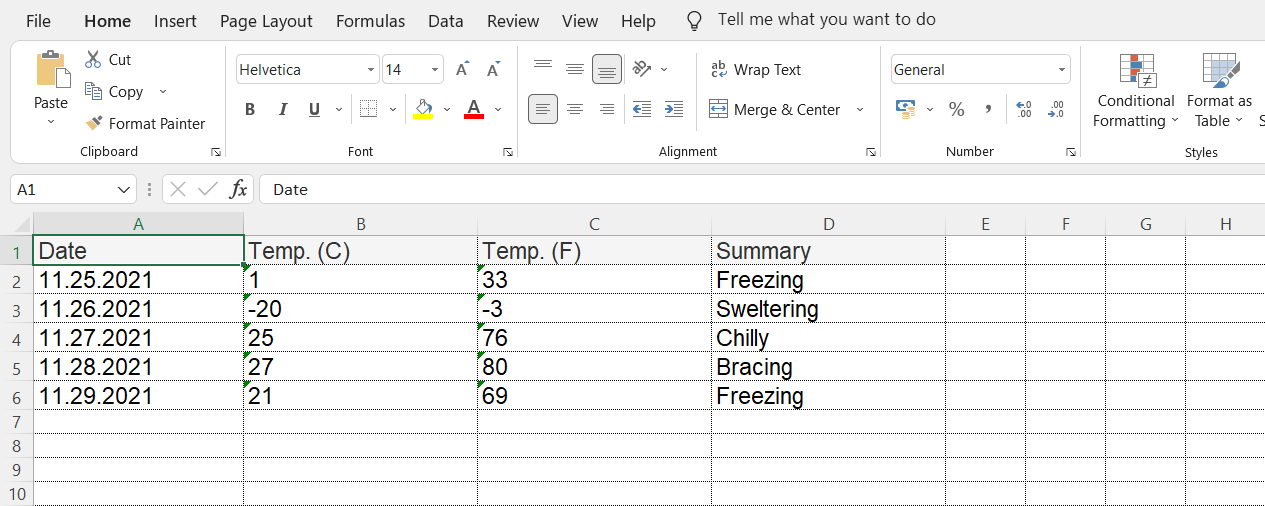
Grid with row hierarchy(TreeGrid / Grouped) can also maintain its hierarchy even when exported. In the Excel file, the rows can be collpased / expanded:
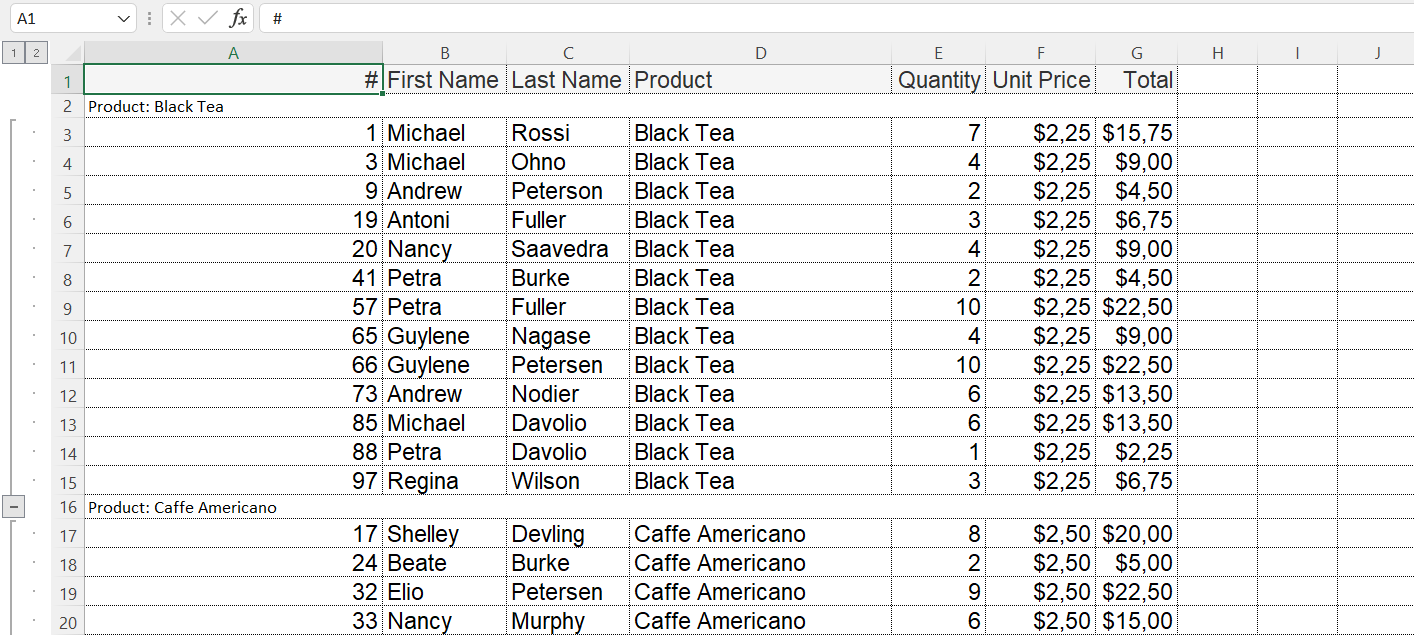
Handling Timezones
Due to the differnt ways browsers and Excel handle dates, there are cases when the values in Excel will not match those in the Grid.
For example, 2022/01/01 00:00:00
will export to 2021-12-31
for anyone to the east of the UTC Timezone.
Similarly, 2021/12/31 23:59:00
will export to 2022-01-01
for anyone to the west of the UTC TImezone.
To avoid this behavior, we strongly encourage developers to specify the timezone of the date values when setting them as DataSource of the Grid.
For example, in the date 2022-01-01T00:00:00.000Z
, Z specifies that the timezone is set to UTC.
The local browser will automatically convert the date to the local timezone.
After the export, the Excel sheet will contain the original 2022-01-01T00:00:00
date.
Example of date behavior:
- For the purpose of the demo, we want to display the date
2022/01/01 15:00
in our Grid. Create two data records.
Set the first date value to the desired date, converted to the UTC Timezone. For example, for users in Central Europe, the value will be2022-01-01T14:00:00.000Z
.
Because the first date value has a specified timezone, the browser will automatically convert it to the user's timezone.
Set the second date value to the date we want to display -20201-01-01T15:00:00
. Since there is not timezone, the browser will assume it is local time.private object[] forecast = new object[]{ new { Date = new DateTime(2022, 1, 1, 15, 0, 0).ToUniversalTime().ToString("o", System.Globalization.CultureInfo.InvariantCulture) //equal to "2022-01-01T14:00:00.000Z" for Central Europe, TemperatureC = 50, TemperatureF = 50, Summary = "Hot" }, new { Date = "2022-01-01T15:00:00", TemperatureC = 50, TemperatureF = 50, Summary = "Hot" }, };
We can see that after the conversion, the two dates are exactly the same - equal to
2022/01/01 15:00
: - In the exported Excel file, we can see that the values remain the same, but were converted from local to UTC Timezone -
2022/01/01 14:00
(for users in Central Europe)
We can see that the first date value (the one with timezone) is the same as the original value we set to the Grid -2022/01/01 14:00
.
But the second date value has changed, because it was converted from local timezone(15:00
) to Universal Timezone (14:00
).
That is why, we strongly recommend specifying the timezone of each date, so that the behavior of the date after the export is more predictable.
GridDataExport
You can apply additional exporting options by creating a GridDataExport object and setting it as a DataExport property of the Grid
The GridDataExport objects accepts the following properties:
Header
- sets whether the columns header will be exported.
Acceptstrue(default) | false
FilterBy
- sets whether the export uses the applied filters.
Acceptsobject - default: null
GroupBy
- sets the groups of the exported data.
Acceptsobject - default: null
Style
- sets a custom style object of the dataExport.
Acceptsobject - default: null
FileName
- sets the exported file's name.
Acceptsstring - default: "jqxGrid"
PageOrientation
- sets the page orientation, when exporting to PDF.
AcceptsGridDataExportPageOrientation.Landscape | Portrait(default)
ExpandChar
- sets the expand char displayed when the Grid with row hierarchy(TreeGrid / Grouped) is exported.
Acceptsstring - default: "+"
CollapseChar
- sets the collapse char displayed when the Grid with row hierarchy(TreeGrid / Grouped) is exported.
Acceptsstring - default: "-"
View
- sets whether to export only the visible data of the Grid.
Acceptstrue | false(default)
ViewStart
- determines the start row index that will be exported or printed.View
should be set to true.
Acceptsint - default: null
ViewEnd
- determines the end row index that will be exported or printed.View
should be set to true.
Acceptsint - default: null
RowIds
- an array of row ids that denotes the rows to export.
AcceptsIEnumerable<object> - default: null