Edit Scheduler Appointments
Setup The Blazor Application
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Bind data to Blazor Smart.Scheduler
Follow the Scheduler Data Bind guide to bind the appointments to the scheduler.
Editing
By default the editing of the scheduler events is enabled. You can create appointments, delete appointments or edit appointments by an edit window dialog or by dragging, dropping and resizing the existing appointments.
You can use the following properties for disabling the edit functionality of the Scheduler:
DisableWindowEditor
- Disables the window editor for the events.DisableResize
- Disables resizing of events.DisableDrag
- Disables event dragging. Events cannot be dragged when this property is set to true.DisableDrop
- Disables event dropping. Events cannot be dropped inside a Smart.Scheduler when this property is set to true.
The DisableDrag
and DisableResize
properties are also applicable to specific events. For example, when we need a specific event not to be draggable and resizable we set the DisableDrag and DisableResize properties to true in the event object like this:
new SchedulerDataSource() { Label = "Brochure Design Review", DateStart = new DateTime(today.Year, today.Month, today.Day, 13, 15, 0).AddDays(2), DateEnd = new DateTime(today.Year, today.Month, today.Day, 16, 15, 0).AddDays(2), BackgroundColor = "#7986CB", DisableDrag = true, DisableResize = true }
As a result all other events will be draggable and resizable except the one with DisableDrag and DisableResize properties set to 'true'.
The following events are related to the editing of the Scheduler appointments:
OnItemChange
- This event is triggered when an appointment has been updated/inserted/removed/dragged/resized.OnItemClick
- This event is triggered when an appointment, appointment item or a context menu item is clicked.OnItemInsert
- This event is triggered when an appointment is inserted.OnItemRemove
- This event is triggered when an appointment is removed.OnItemUpdate
- This event is triggered when an appointment is updated.
OnDragStart
- Fired when an appointment is about to be dragged. The event contains details regarding the appointment object that is going to be dragged.OnDragEnd
- Fired when an appointment is dropped. The event contains details regarding the Scheduler appointment object that is being dropped.OnResizeStart
- Fired when an appointment is about to be resized. The event contains details regarding the Scheduler appointment object that is about to be resized.OnResizeEnd
- Fired when resizing of an appointment is finished. The event contains details regarding the appointment object that has been resized.
Editing options
The Scheduler provides the following options for interacting with the appointments:
- Double click (or double tap) on an empty cell opens a dialog window for inserting a new appointment in the Scheduler. If you double-click in the all-day row, the new appointment will start as an all-day appointment. You can still change the start and end date and time in the dialog.
-
Double click (or double tap) on an appointment opens a dialog for editing it. If you double click on a recurring appointment a prompt window will be opened asking if you want to edit only the current event or the whole series.
- Dragging and dropping an appointment changes it start and end dates.
- Resizing an appointment changes its duration.
-
Right-click on an scheduler's empty cell opens a context menu with the option for creating a new appointment. Right-click on an appointment opens a context menu with the options for creating a new appointment, editing or deleting the appointment. This functionality can be disabled by setting the DisableContextMenu property to true.
Example
Here is an example of how you can enable and disable the editing funtionality:
<div> <Scheduler DataSource="dataRecords" View="SchedulerViewType.Day" HourStart="9" HourEnd="18" FirstDayOfWeek="1" DisableContextMenu="@disableContextMenu" DisableWindowEditor="@disableWindowEditor" DisableDrag="@disableDrag" DisableDrop="@disableDrop" DisableResize="@disableResize" /> <div class="options"> <div class="option"> <h4>Settings: <CheckBox OnChange="OnDisableContextMenu">Disable Context Menu</CheckBox> <CheckBox OnChange="OnDisableWindowEditor">Disable Window Editor</CheckBox> <CheckBox OnChange="OnDisableDrag">Disable Event Drag</CheckBox> <CheckBox OnChange="OnDisableDrop">Disable Event Drop</CheckBox> <CheckBox OnChange="OnDisableResize">Disable Event Resize</CheckBox> </div> </div> </div> @code { private List<SchedulerDataSource> dataRecords; bool disableContextMenu = false; bool disableWindowEditor = false; bool disableDrag = false; bool disableDrop = false; bool disableResize = false; protected override void OnInitialized() { base.OnInitialized(); dataRecords = GetData(); } private List<SchedulerDataSource> GetData() { DateTime today = DateTime.Today; List<SchedulerDataSource> data = new List<SchedulerDataSource>() { new SchedulerDataSource() { Label = "Website Re-Design Plan", DateStart = new DateTime(today.Year, today.Month, today.Day, 9, 30, 0).AddDays(-2), DateEnd = new DateTime(today.Year, today.Month, today.Day, 11, 30, 0).AddDays(-2), BackgroundColor = "#689F38" }, new SchedulerDataSource() { Label = "Book Flights to San Fran for Sales Trip", DateStart = new DateTime(today.Year, today.Month, today.Day, 10, 0, 0).AddDays(-1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 12, 0, 0).AddDays(-1), BackgroundColor = "#7986CB" }, new SchedulerDataSource() { Label = "Install New Router in Conference Room", DateStart = new DateTime(today.Year, today.Month, today.Day, 12, 0, 0).AddDays(-2), DateEnd = new DateTime(today.Year, today.Month, today.Day, 14, 30, 0).AddDays(-2), BackgroundColor = "#8D6E63" }, new SchedulerDataSource() { Label = "Add a new desk to the Dev Room", DateStart = new DateTime(today.Year, today.Month, today.Day, 12, 30, 0).AddDays(-1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 14, 45, 0).AddDays(-1), BackgroundColor = "#00C853" }, new SchedulerDataSource() { Label = "Install New Router in Dev Room", DateStart = new DateTime(today.Year, today.Month, today.Day, 13, 0, 0), DateEnd = new DateTime(today.Year, today.Month, today.Day, 15, 30, 0), BackgroundColor = "#2196F3" }, new SchedulerDataSource() { Label = "Approve Personal Computer Upgrade Plan", DateStart = new DateTime(today.Year, today.Month, today.Day, 10, 0, 0).AddDays(1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 11, 0, 0).AddDays(1), BackgroundColor = "#689F38" }, new SchedulerDataSource() { Label = "Final Budget Review", DateStart = new DateTime(today.Year, today.Month, today.Day, 12, 0, 0).AddDays(1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 13, 35, 0).AddDays(1), BackgroundColor = "#FDD835" }, new SchedulerDataSource() { Label = "Old Brochures", DateStart = new DateTime(today.Year, today.Month, today.Day, 13, 0, 0), DateEnd = new DateTime(today.Year, today.Month, today.Day, 15, 15, 0).AddDays(1), BackgroundColor = "#FF8A65" }, new SchedulerDataSource() { Label = "New Brochures", DateStart = new DateTime(today.Year, today.Month, today.Day, 13, 0, 0).AddDays(1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 15, 15, 0).AddDays(2), BackgroundColor = "#0D47A1" }, new SchedulerDataSource() { Label = "Install New Database", DateStart = new DateTime(today.Year, today.Month, today.Day, 9, 0, 0).AddDays(1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 12, 15, 0).AddDays(1), BackgroundColor = "#004D40" }, new SchedulerDataSource() { Label = "Approve New Online Marketing Strategy", DateStart = new DateTime(today.Year, today.Month, today.Day, 12, 0, 0).AddDays(2), DateEnd = new DateTime(today.Year, today.Month, today.Day, 14, 0, 0).AddDays(2), BackgroundColor = "#FF3D00" }, new SchedulerDataSource() { Label = "Upgrade Personal Computers", DateStart = new DateTime(today.Year, today.Month, today.Day, 9, 0, 0), DateEnd = new DateTime(today.Year, today.Month, today.Day, 11, 30, 0), BackgroundColor = "#689F38" }, new SchedulerDataSource() { Label = "Prepare current Year Marketing Plan", DateStart = new DateTime(today.Year, today.Month, today.Day, 11, 0, 0).AddDays(3), DateEnd = new DateTime(today.Year, today.Month, today.Day, 13, 30, 0).AddDays(3), BackgroundColor = "#2196F3" }, new SchedulerDataSource() { Label = "Prepare current Year Marketing Plan", DateStart = new DateTime(today.Year, today.Month, today.Day, 11, 0, 0).AddDays(4), DateEnd = new DateTime(today.Year, today.Month, today.Day, 13, 30, 0).AddDays(4), BackgroundColor = "#CE93D8" } }; return data; } private void OnDisableContextMenu(Event eventObj) { CheckBoxChangeEventDetail args = eventObj["Detail"]; disableContextMenu = args.Value; } private void OnDisableWindowEditor(Event eventObj) { CheckBoxChangeEventDetail args = eventObj["Detail"]; disableWindowEditor = args.Value; } private void OnDisableDrag(Event eventObj) { CheckBoxChangeEventDetail args = eventObj["Detail"]; disableDrag = args.Value; } private void OnDisableDrop(Event eventObj) { CheckBoxChangeEventDetail args = eventObj["Detail"]; disableDrop = args.Value; } private void OnDisableResize(Event eventObj) { CheckBoxChangeEventDetail args = eventObj["Detail"]; disableResize = args.Value; } }Result:
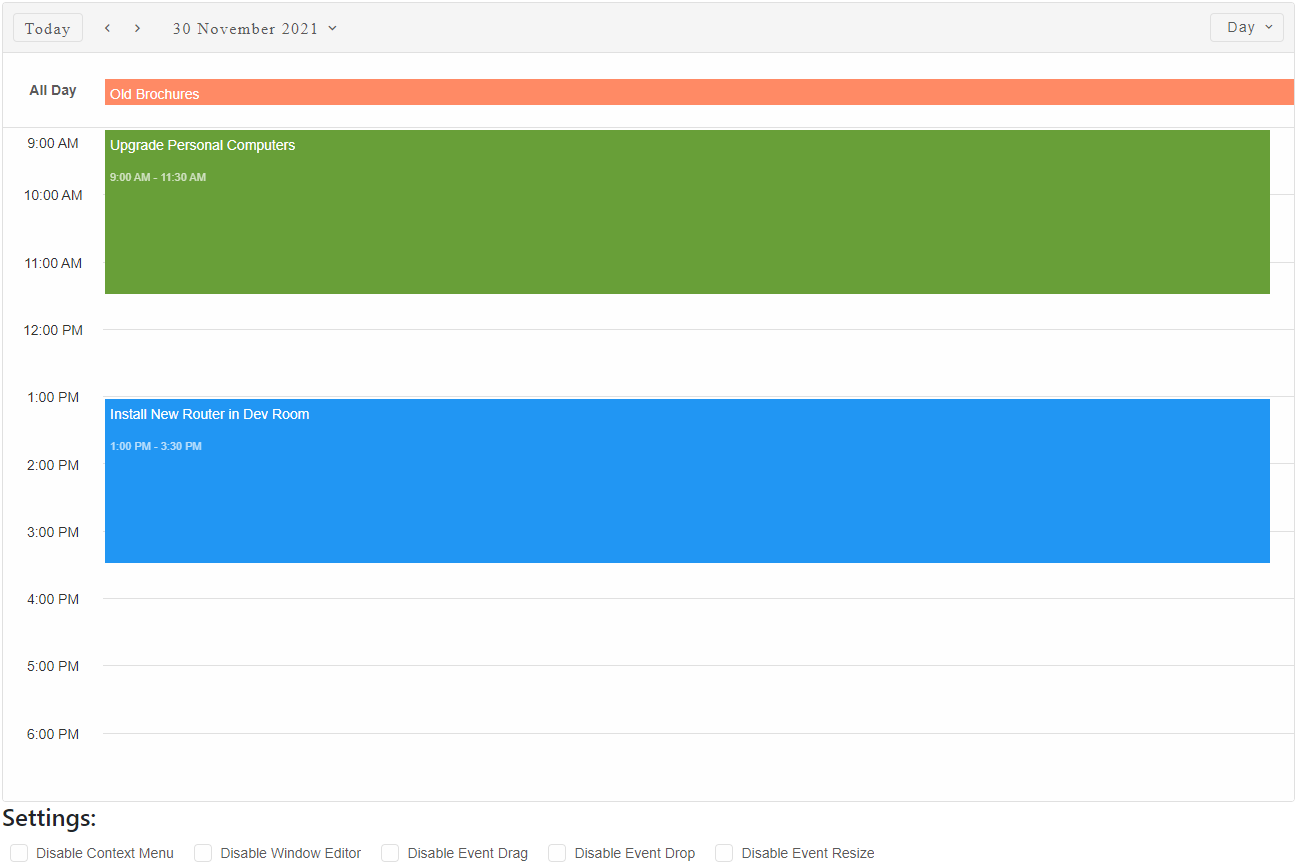