Blazor Kanban Server-side CRUD
Setup The Blazor Application
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Bind to SQL
Follow our Binding to SQL
guide to set up the connection between your database and Smart UI.
At the end of the tutorial, the Smart.Kanban will be bounded to a SQL DataBase:
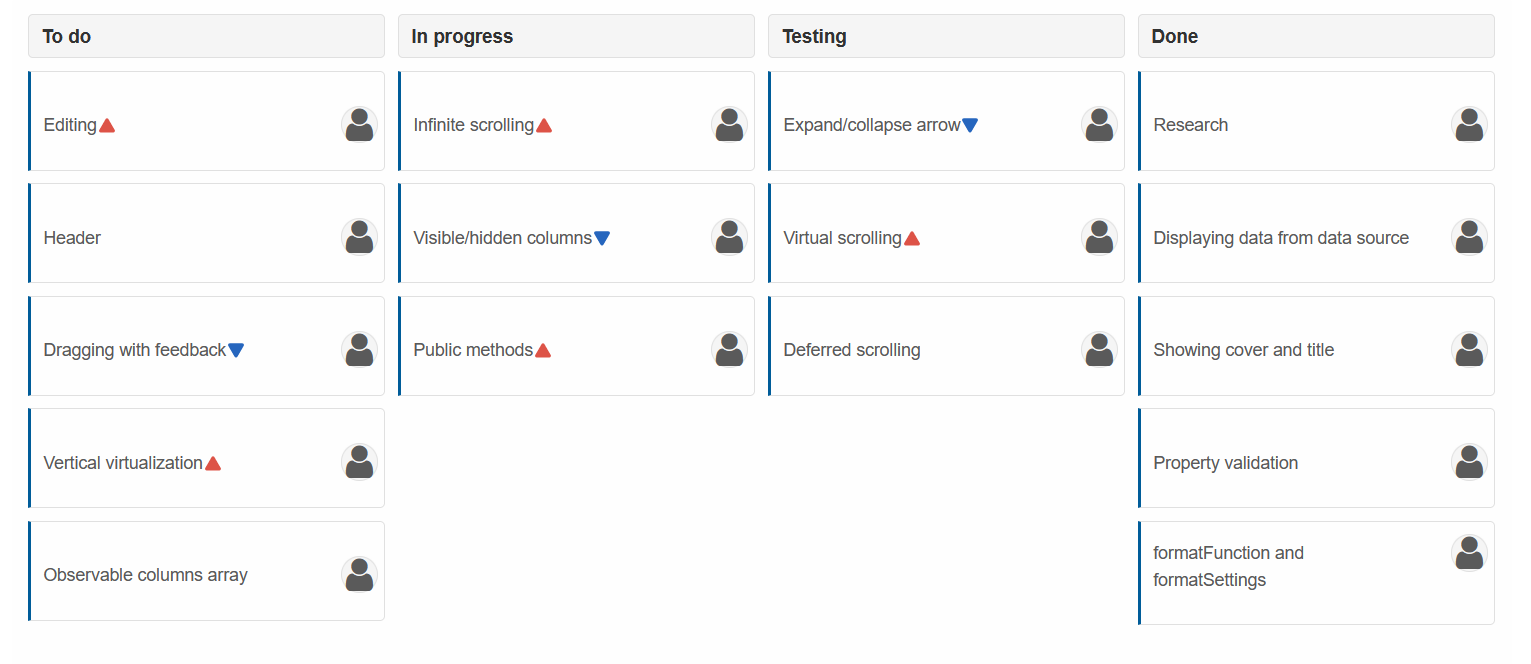
Create Methods
To enable adding new tasks to the Kanban, we must first create the functions for the CRUD operations in the
TaskData class.
Navigate to TaskData.cs and implement the additional methods:
..... public Task<List<TaskModel>> GetTasks() { string sql = "select * from dbo.KanbanTable"; return _db.LoadData<TaskModel, dynamic>(sql, new { }); } public Task<List<TaskModel>> InsertTask(string Text, string Status, string Priority) { string sql = "INSERT INTO [dbo].[KanbanTable] ([Text], [Status], [Priority]) OUTPUT INSERTED.Id, INSERTED.Text, INSERTED.Status, INSERTED.Priority VALUES (@Text, @Status, @Priority)"; return _db.LoadData<TaskModel, dynamic>(sql, new { Text, Status, Priority }); } public Task<List<TaskModel>> DeleteTask(int Id) { string sql = "DELETE FROM [dbo].[KanbanTable] WHERE [Id]=@Id"; return _db.LoadData<TaskModel, dynamic>(sql, new { Id }); } public Task<List<TaskModel>> UpdateTask(int Id, string Text, string Status, string Priority) { string sql = "UPDATE [dbo].[KanbanTable] SET [Text] = @Text, [Status] = @Status, [Priority] = @Priority WHERE [Id] = @Id"; return _db.LoadData<TaskModel, dynamic>(sql, new { Text, Status, Priority, Id }); } .....
Then, add the new methods to the ITasksData interface:
..... Task<List<TaskModel>> GetTasks(); Task<List<TaskModel>> DeleteTask(int Id); Task<List<TaskModel>> InsertTask(string Text, string Status, string Priority); Task<List<TaskModel>> UpdateTask(int Id, string Text, string Status, string Priority); .....
Add Create functionality
Navigate to the Index.razor
page and create a "Add new task" Button.
Then create an AddTask
function that creates a new Task and then fetches the updated SQL Table:
<Button OnClick="AddTask">Add new task</Button> ..... @code{ ..... private async Task AddTask() { TaskModel newTask = (await _db.InsertTask("Update UI", "ToDo", "low"))[0]; tasks = await _db.GetTasks(); } }
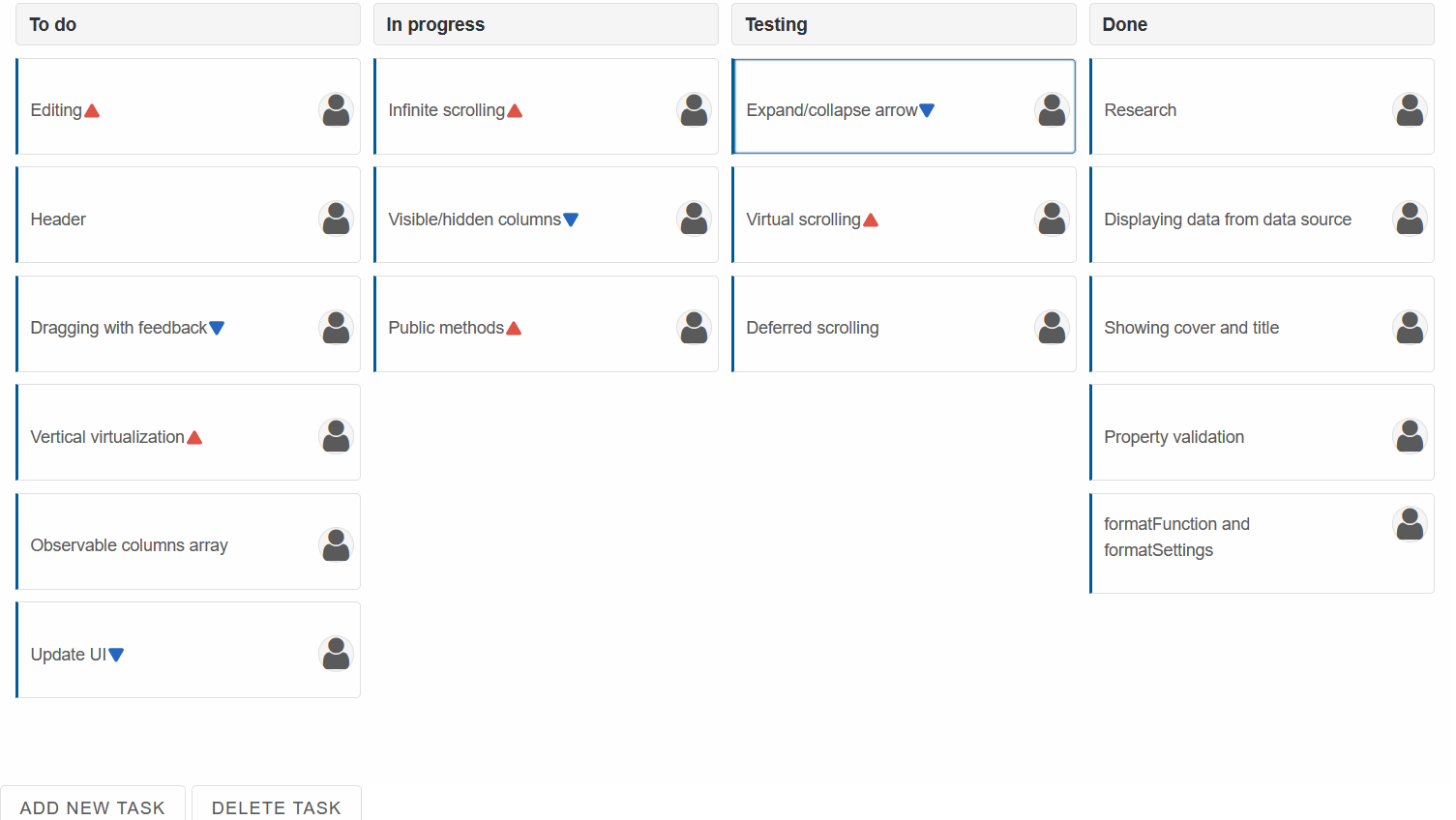
The new Task is created in the SQL Table:
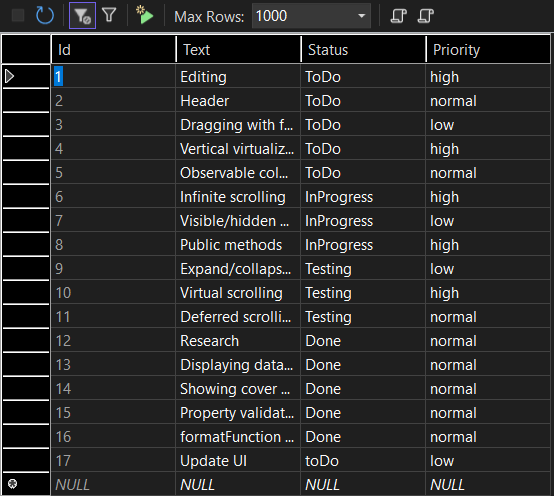
Add Delete functionality
Add a "Delete last task" Button. Then create a DeleteLastTask
function that removes the last SQL Record and then fetches the updated SQL Table:
<Button OnClick="DeleteLastTask">Delete task</Button> ..... @code{ ..... private async Task DeleteLastTask() { int lastId = tasks[tasks.Count - 1].Id; await _db.DeleteTask(lastId); tasks = await _db.GetTasks(); } }
The last Task is removed from the SQL Table after clicking the button:
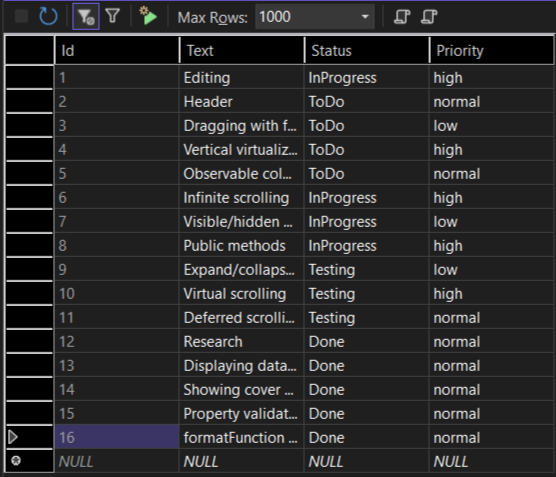
Add Update functionality
To add Update functionality, first import the Newtonsoft JSON library.
We will use the OnChange
Event to update the SQL Table each time a task has been modified or moved to another column:
@using Newtonsoft.Json.Linq <Kanban @ref="kanban" Columns="columns" DataSource="@tasks" DataSourceMap="dataSourceMap" OnChange="OnChange"></Kanban> @code{ .... private void OnChange(Event ev) { KanbanChangeEventDetail detail = ev["Detail"]; TaskModel taskObject = JObject.Parse(detail.Value.ToString()).ToObject<TaskModel>(); _db.UpdateTask(taskObject.Id, taskObject.Text, taskObject.Status, taskObject.Priority); } }
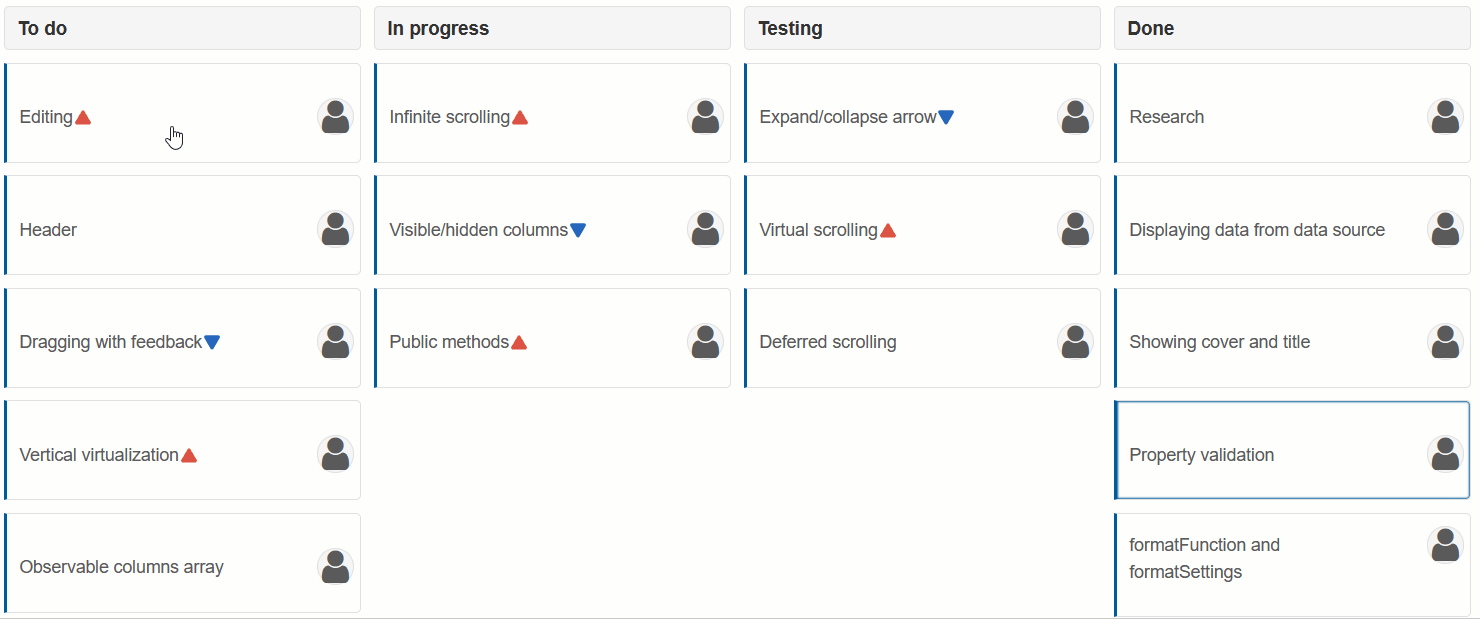
After editing, the changes are applied to the SQL Table:
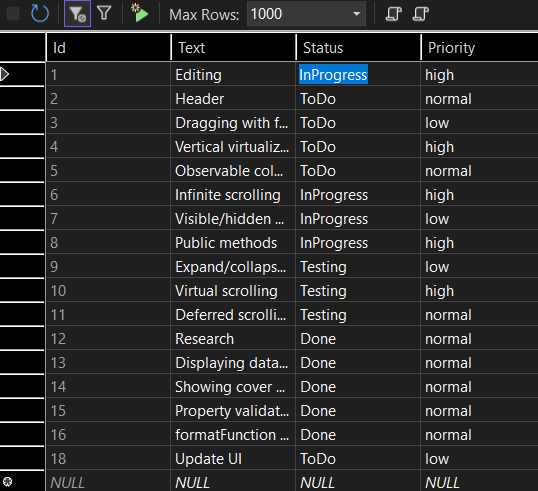