Blazor Smart.PivotTable Toolbar
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup the Blazor Smart.PivotTable
Follow the Get Started with Smart.PivotTable guide to set up the component.
Toolbar
In Smart.PivotTable, a toolbar can be displayed at the top of the element. It provides several tools that allow for Pivot Table column customization, conditional formatting, and filtering.
The toolbar is enabled by setting the property Toolbar to true
.
The toolbar contains the following:
-
(1) Row group columns breadcrumb
Allows for removing and reordering the currently applied Row group columns by dragging the breadcrumb items. Items can also be dragged into (2) Pivot columns breadcrumb if their corresponding columns have AllowPivot enabled.
You can learn more about the column roles in the Blazor Smart.PivotTable Columns guide. -
(2) Pivot columns breadcrumb
Allows for removing and reordering the currently applied Pivot columns by dragging the breadcrumb items. Items can also be dragged into (1) Row group columns breadcrumb if their corresponding columns have AllowRowGroup enabled.
-
(3) Conditional Formatting button
When clicked, opens a dialog with a formatting panel inside.
The formatting panel allows the configuration of background and text color of certain cells under certain conditions.
Available settings in the formatting panel are:- Column to apply formatting to (applicable to all dyncamic summary columns);
- Condition to apply formatting on - Less Than, Greater Than, Equal To, Not Equal To, Between;
- Value based on which to evaluate condition; if condition is Between, a second, "to", value is configurable;
- Font family to apply when condition is met;
- Font size to apply when condition is met;
- Background and text color to apply when condition is met - both configurable via additional pop-up opened from the formatting panel.
All of these settings can be configured on initialization in the Pivot Table by setting the property ConditionalFormatting (IEnumerable<IPivotTableConditionalFormatting>
) the value of which is always in sync with the Conditional Formatting panel in the dialog. -
(4) Fields button
When clicked, opens a dialog with the Pivot Table designer. Full description of the designer is available in Blazor Smart.PivotTable Designer guide.
When the property Designer is enabled, thus showing the designer to the side of the table, the Fields button is not available.
Example
Here is a full example of Pivot Table with Toolbar and Conditional Formatting:
@using Smart.Blazor.Demos.Data; @inject RandomDataService RandomDataService <PivotTable DataSource="Data" ConditionalFormatting="conditionalFormatting" FreezeHeader KeyboardNavigation Toolbar Columns="@columns"/> @code { List<PivotTableConditionalFormatting> conditionalFormatting = new List<PivotTableConditionalFormatting>() { new PivotTableConditionalFormatting() { Column = "Quantity", Condition = PivotTableConditionalFormattingCondition.GreaterThan, FirstValue = 7, Text = "#FFFFFF", Highlight = "#6AA84F" }, new PivotTableConditionalFormatting() { Column = "Quantity", Condition = PivotTableConditionalFormattingCondition.Between, FirstValue = 1, SecondValue = 3, FontSize = PivotTableConditionalFormattingFontSize.Tenpx, Text = "#FFFFFF", Highlight = "#CC0000" } }; List<PivotTableColumn> columns = new List<PivotTableColumn>() { new PivotTableColumn() { Label = "First Name", DataField = "FirstName", DataType = PivotTableColumnDataType.String, AllowRowGroup = true, RowGroup = true }, new PivotTableColumn() { Label = "Last Name", DataField = "LastName", DataType = PivotTableColumnDataType.String, AllowPivot = true, AllowRowGroup = true, RowGroup = true }, new PivotTableColumn() { Label = "Product Name", DataField = "ProductName", DataType = PivotTableColumnDataType.String, AllowPivot = true, Pivot = true }, new PivotTableColumn() { Label = "Quantity", DataField = "Quantity", DataType = PivotTableColumnDataType.Number, Summary = PivotTableColumnSummary.Sum }, new PivotTableColumn() { Label = "Price", DataField = "Price", DataType = PivotTableColumnDataType.Number, Summary = PivotTableColumnSummary.Sum, SummarySettings = new { prefix = "$", decimalPlaces = 2 } }, new PivotTableColumn() { Label = "Date Purchased", DataField = "TimeOfPurchase", DataType = PivotTableColumnDataType.Date } }; public DataRecord[] Data; protected override async Task OnInitializedAsync() { Data = await RandomDataService.GetDataAsync(25); } }Result:
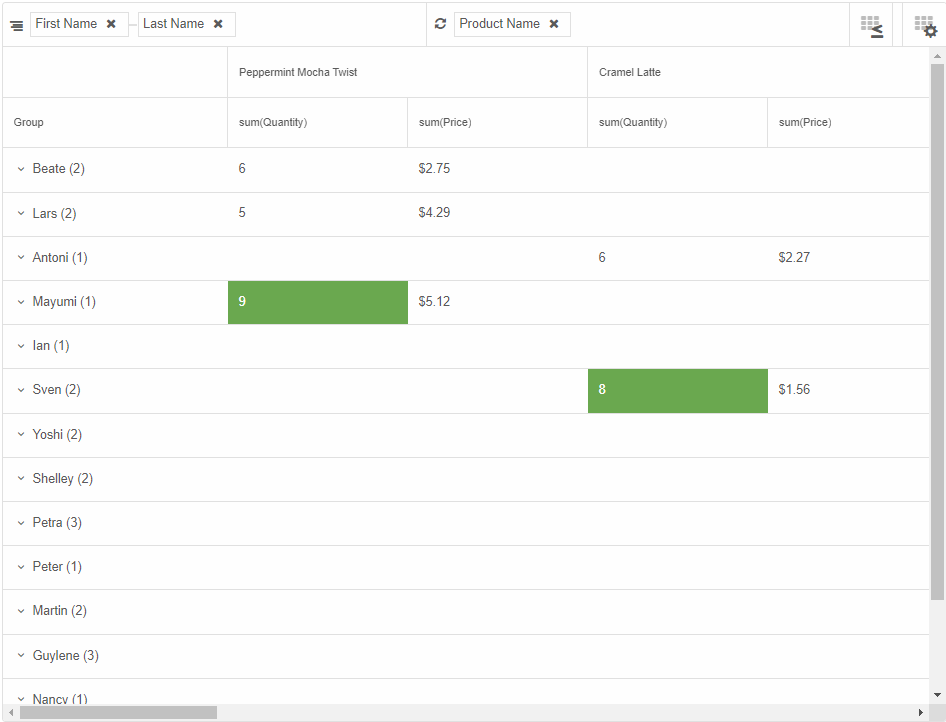