Bind Web API data to Blazor Smart.Grid
Setup The Blazor Application
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Connect to Web API
The following steps detail how to connect to a Web API and retrieve its data inside your Blazor Application. If you already have the API's data, continue to Bind Web API to Grid.
- For the purpose of the Demo, we will use the following API, which returns a JSON Array of users:
Random Data API
-
Using the Visual Studio NuGet Package Manager, add the following dependency, which will be required to create
a HTTP call and parse the JSON Array:
System.Net.Http.Json
-
Add the HTTP Client Service to the
ConfigureServices
method inside the Startup.cs file.... public void ConfigureServices(IServiceCollection services) { services.AddRazorPages(); services.AddServerSideBlazor(); services.AddSingleton<WeatherForecastService>(); services.AddSmart(); services.AddHttpClient(); } ....
-
Inside your project, create a new folder "Models", then create a new item of type Class called
UserModel.cs
This is where we will define the properties of each individual User from the Web API:namespace BlazorGrid.Models { public class UserModel { public int Id { get; set; } public string Password { get; set; } public string First_name { get; set; } public string Last_name { get; set; } public string Username { get; set; } public string Email { get; set; } public string Phone_number { get; set; } public string Date_of_birth { get; set; } } }
Bind Web API to Grid
-
Inside the
Pages/Index.razor
page, add the Grid component to the Pages/Index.razor file of your Blazor Application and set the Columns you want to display:<Grid DataSource="@usersData" DataSourceSettings="@dataSourceSettings"> <Columns> <Column DataField="First_name" Label="First Name"></Column> <Column DataField="Last_name" Label="Email"></Column> <Column DataField="Phone_number" Label="Phone"></Column> </Columns> </Grid>
-
Inject the User Model, as well as
IHTTPClientFactory
, which will be used to make the call to the API:@page "/" @using System.Net.Http.Json @using Smart.Blazor @using BlazorGrid.Models @inject IHttpClientFactory _clientFactory
-
When the page has initialized, create a request to the API and set the returned value to the userData
Array, which is the DataSource of the Grid.
Note that setting the DataType of the Columns is not mandatory, but it is recommended if you plan to use the Smart.Grid's Filtering & Sorting functionalities@page "/" @using System.Net.Http.Json @using Smart.Blazor @using BlazorGrid.Models @inject IHttpClientFactory _clientFactory <h2>Users Table </h2> @if (usersData == null) { <p> <em>Loading... </em> </p> } else { <Grid DataSource="@usersData" DataSourceSettings="@dataSourceSettings"> <Columns> <Column DataField="First_name" Label="First Name"> </Column> <Column DataField="Last_name" Label="Email"> </Column> <Column DataField="Phone_number" Label="Phone"> </Column> </Columns> </Grid> } @code { GridDataSourceSettings dataSourceSettings = new GridDataSourceSettings() { DataFields = new List<IGridDataSourceSettingsDataField>() { new GridDataSourceSettingsDataField() { Name = "First_name", DataType = GridDataSourceSettingsDataFieldDataType.String }, new GridDataSourceSettingsDataField() { Name = "Last_name", DataType = GridDataSourceSettingsDataFieldDataType.String }, new GridDataSourceSettingsDataField() { Name = "Phone_number", DataType = GridDataSourceSettingsDataFieldDataType.String } }, DataSourceType = GridDataSourceSettingsDataSourceType.Json }; UserModel[] usersData; protected override async Task OnInitializedAsync() { var request = new HttpRequestMessage(HttpMethod.Get, "https://random-data-api.com/api/users/random_user?size=10"); var client = _clientFactory.CreateClient(); HttpResponseMessage response = await client.SendAsync(request); usersData = await response.Content.ReadFromJsonAsync<UserModel[]>(); } }
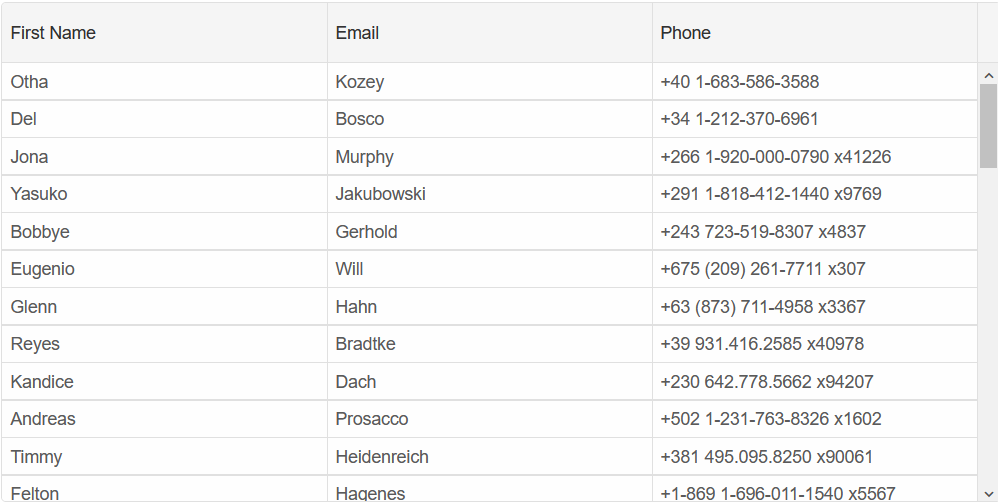
Continue from here
Follow the Get Started with Grid guide to learn more about many of the features offered by Blazor Smart.Grid component.
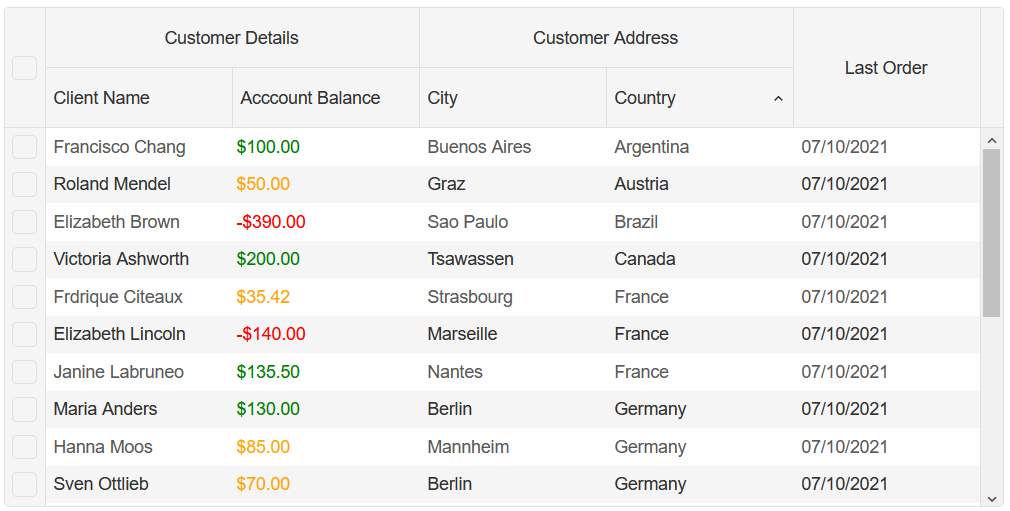