Getting Started
Webpack is used to compile JavaScript modules. Once installed, you can interface with webpack either from its CLI or API. If you're still new to webpack, please look at: https://webpack.js.org/guides/getting-started/.
.Using Smart Elements with Webpack
For the purpose of the tutorial, we will create a web page with a Smart.Button web component.project
webpack-demo |- package.json + |- webpack.config.js |- /dist |- index.html |- /src |- index.js |- /source |- /smart.elements.js |- /styles |- /smart.default.css |- /font |- /images
As you can see from the project structure, we place the smart scripts and CSS files which we want to use within the /src/source folder.
webpack.config.js
const path = require('path'); module.exports = { entry: './src/index.js', output: { filename: 'main.js', path: path.resolve(__dirname, 'dist') } };
package.json
{ "name": "webpack-demo", "version": "1.0.0", "description": "", "scripts": { "build": "webpack" }, "keywords": [], "author": "", "license": "ISC", "devDependencies": { "webpack": "^4.20.2", "webpack-cli": "^3.1.2" }, "dependencies": { "lodash": "^4.17.5" } }
index.html
<!doctype html> <html> <head> <title>Getting Started</title> <link rel="stylesheet" href="../src/source/styles/smart.default.css"> </head> <body> <script src="main.js"></script> </body> </html>
main.js
import './source/smart.elements.js'; const button = document.createElement('smart-button'); button.innerHTML = 'Click Me'; document.body.appendChild(button);
Install and Run Webpack
1. Run the npm install command to install the required node modules. (When installing a package that will be bundled into your production bundle, you should use npm install --save. If you're installing a package for development purposes (e.g. a linter, testing libraries, etc.) then you should use npm install --save-dev.)2. Now the npm run build command can be used. Note that within scripts we can reference locally installed npm packages by name.
output
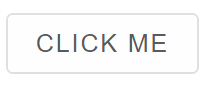
download
webpack.zip