Blazor - Get Started with Smart.ProgressBar
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic ProgressBar
Smart.ProgressBar is a custom that displays the progress of a given task. There are two main bar types - Linear and Circular.
- Add the ProgressBar and CircularProgressBar components to the Pages/Index.razor file. Linear Bars can be Vertical or Horizontal.
<ProgressBar></ProgressBar> <ProgressBar Orientation="Orientation.Vertical"></ProgressBar> <CircularProgressBar></ProgressBar>
- Set the
Value
property to a number in the range 0 - 100<ProgressBar Value="50"></ProgressBar> <ProgressBar Orientation="Orientation.Vertical" Value="50"></ProgressBar> <CircularProgressBar Value="50"></ProgressBar>
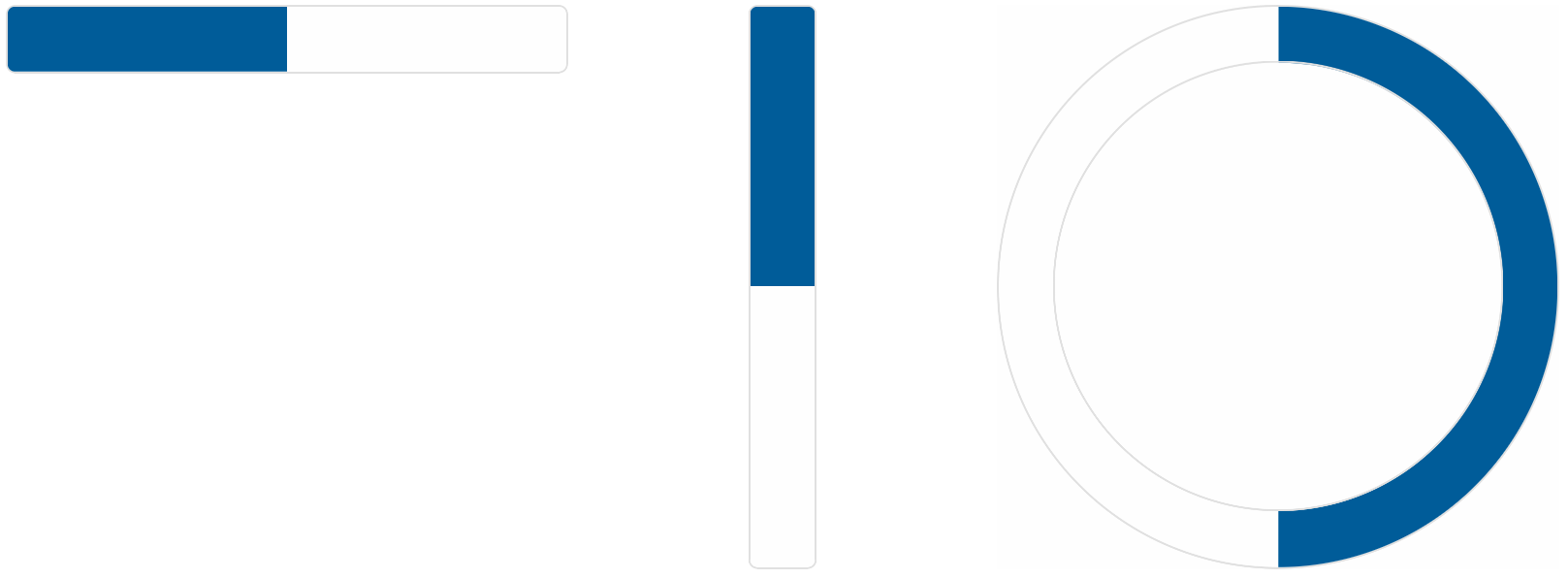
Indeterminate
When the ProgressBar is set to Indeterminate, it is used to visualize an unspecified wait time
<ProgressBar Indeterminate></ProgressBar> <ProgressBar Orientation="Orientation.Vertical" Indeterminate></ProgressBar> <CircularProgressBar Indeterminate></ProgressBar>
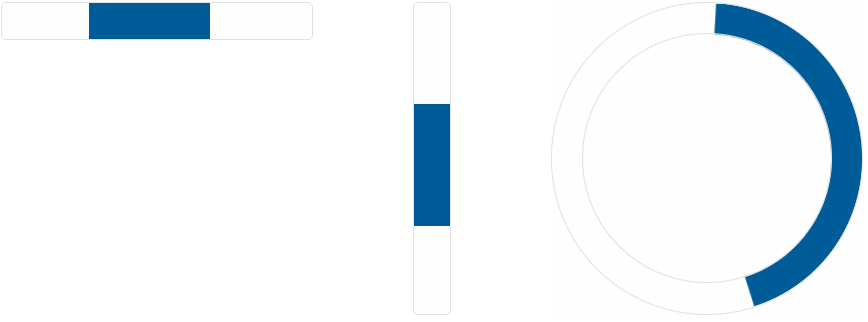
Customization
The ProgressBar component can be customized in "Barber Shop" style by setting it in the Class
property.
<ProgressBar Indeterminate Class="barber-shop-effect"></ProgressBar> <ProgressBar Orientation="Orientation.Vertical" Indeterminate Class="barber-shop-effect"></ProgressBar>
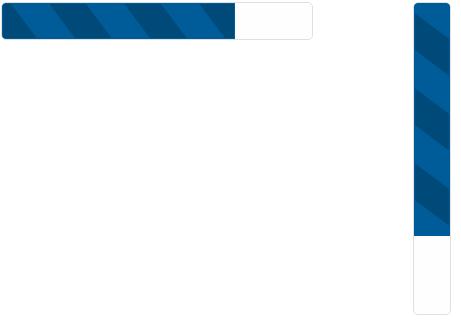
ProgressBar Events
Smart.ProgressBar and Smart.CircularProgressBar provide an OnChange event that can help you expand the components' functionality.
OnChange
- triggered when the value is changed.
Event Details
: N/A
The demo below uses the OnChange
Event to return to display the difference between the current and the previous value:
<NumberInput @ref="input" Value="10"></NumberInput> <Button OnClick="Increase">Increase value</Button> <h3>@message</h3> <ProgressBar @ref="progress" Class="barber-shop-effect" ShowProgressValue OnChange="OnChange"></ProgressBar> @code{ ProgressBar progress; NumberInput input; string message = ""; private void Increase(){ progress.Value += Int32.Parse(input.Value.ToString()); } private void OnChange(){ message = "Value changed! New value is " + progress.Value; } }
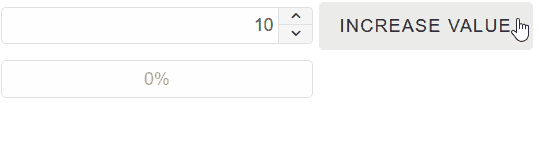