Form - Value Handling
Smart.Form allows you to set the intial value of the form fields, as well as to get the new values which the user entred.
Set Form Value
Create the inital Form. For this example, we will create a Profile Form:
<Form id="profileForm"> <FormGroup Label="Personal" DataField="personal"> <FormControl Info="Enter First Name" Required Placeholder="First Name" ControlType="FormControlControlType.Input" DataField="firstName" Label="First Name" Class="outlined"></FormControl> <FormControl Info="Enter Last Name" Required Placeholder="Last Name" ControlType="FormControlControlType.Input" DataField="lastName" Label="Last Name" Class="outlined"></FormControl> <FormControl Info="Enter Age" Required Placeholder="Age" ControlType="FormControlControlType.Number" DataField="age" Label="Age" Class="outlined"></FormControl> </FormGroup> <FormGroup Label="Details" DataField="details"> <FormControl Placeholder="Company Name" Required ControlType="FormControlControlType.Input" DataField="company" Label="Company" Class="outlined"></FormControl> <FormControl Placeholder="Address" Required ControlType="FormControlControlType.Input" DataField="address" Label="Address" Class="outlined"></FormControl> <FormControl Placeholder="City" Required ControlType="FormControlControlType.Input" DataField="city" Label="City" Class="outlined"></FormControl> <FormControl Placeholder="State" Required ControlType="FormControlControlType.Input" DataField="state" Label="State" Class="outlined"></FormControl> <FormControl Placeholder="Zip / Postal Code" Required ControlType="FormControlControlType.Input" DataField="zip" Label="Zip / Postal Code" Class="outlined"></FormControl> <FormControl Required ControlType="FormControlControlType.Boolean" DataField="available" Label="Available"></FormControl> </FormGroup> </Form>
The prefilled values of a Form are set using the Value
property. Create a generic object, which matches the structure of the Form:
<Form id="profileForm" Value="defaultProfileForm">...</Form> @code{ object defaultProfileFormValues = new { personal = new { firstName = "John", lastName = "Doe", age = 30 }, details = new { company = "Acme Corp", address = "123 Main St", city = "Metropolis", state = "Stateville", zip = "12345", available = true } }; }
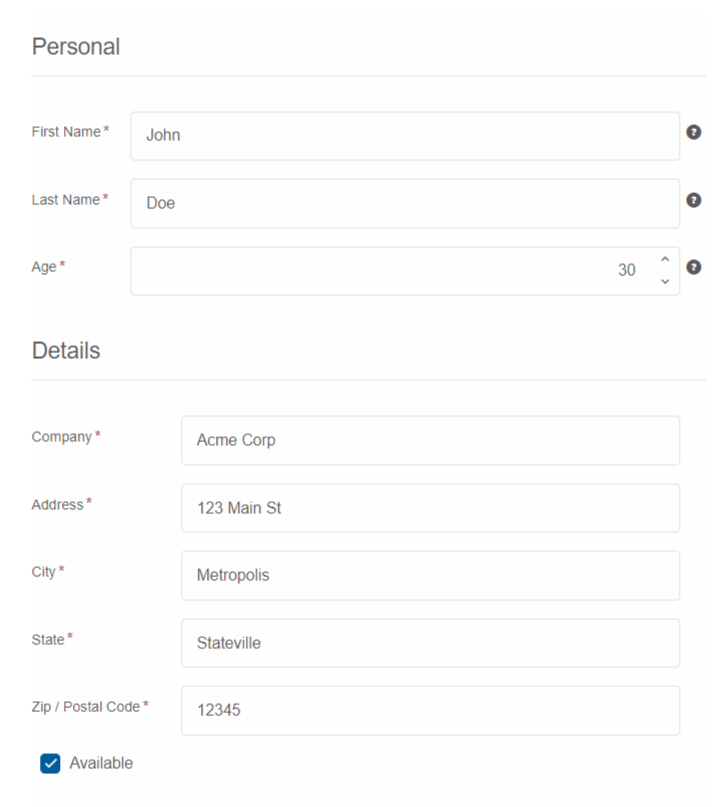
Get Form Value
Each time the user changes the value of a Form Control, the OnValueChanges
callback functino will be called.
The callback will contain the new state of the Form. We can create a C# class that matches the structure of the Form and create a new instance of this class.
<Form id="profileForm" Value="defaultProfileForm" OnValueChanges="OnValueChanges">...</Form> @code{ public class ProfileForm { public Personal personal { get; set; } public Details details { get; set; } public class Personal { public string firstName { get; set; } public string lastName { get; set; } public int age { get; set; } } public class Details { public string company { get; set; } public string address { get; set; } public string city { get; set; } public string state { get; set; } public string zip { get; set; } public bool available { get; set; } } } private void OnValueChanges(object newValue) { ProfileForm profileForm = JsonConvert.DeserializeObject<ProfileForm>(newValue.ToString()); } }
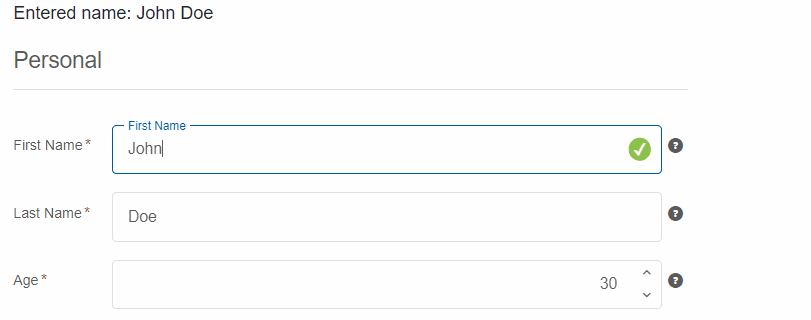