Export Data
Smart.Gantt can export the visualized grid into a new file, while taking into account the current state of the Tasks. The following file formats are supported:
- XLSX
- XML
- CSV
- TSV
- HTML
Create a new Button which calls the custom exportGantt
function when clicked.
The file format is set as a parameter of the ExportData
function
@using System.Text.Json.Serialization; <Button OnClick="ExportToXLSX">Export to XLSX</Button> <GanttChart @ref="gantt" DataSource="Records" TaskColumns="taskColumns" DurationUnit="Duration.Hour" TreeSize="treeSize" NonworkingHours="nonworkingHours" NonworkingDays="nonworkingDays" /> @code { public partial class GanttDataRecord { [JsonPropertyName("id")] public string Id { get; set; } [JsonPropertyName("label")] public string Label { get; set; } [JsonPropertyName("dateStart")] public string DateStart { get; set; } [JsonPropertyName("dateEnd")] public string DateEnd { get; set; } [JsonPropertyName("class")] public string Class { get; set; } [JsonPropertyName("type")] public string Type { get; set; } [JsonPropertyName("progress")] public int Progress { get; set; } [JsonPropertyName("duration")] public int Duration { get; set; } [JsonPropertyName("synchronized")] public bool Synchronized { get; set; } [JsonPropertyName("expanded")] public bool Expanded { get; set; } [JsonPropertyName("tasks")] public List < GanttDataRecord > Tasks { get; set; } [JsonPropertyName("disableResources")] public bool DisableResources { get; set; } [JsonPropertyName("resources")] public object Resources { get; set; } = new object[] {}; [JsonPropertyName("connections")] public List < Dictionary < string, int >> Connections { get; set; } = new List < Dictionary < string, int >> () {}; [JsonPropertyName("disableDrag")] public bool DisableDrag { get; set; } [JsonPropertyName("disableResize")] public bool DisableResize { get; set; } [JsonPropertyName("minDateStart")] public string MinDateStart { get; set; } [JsonPropertyName("maxDateStart")] public string MaxDateStart { get; set; } [JsonPropertyName("minDateEnd")] public string MinDateEnd { get; set; } [JsonPropertyName("maxDateEnd")] public string MaxDateEnd { get; set; } [JsonPropertyName("minDuration")] public int MinDuration { get; set; } [JsonPropertyName("maxDuration")] public int MaxDuration { get; set; } [JsonPropertyName("value")] public int Value { get; set; } [JsonPropertyName("dragProject")] public bool DragProject { get; set; } } GanttChart gantt; string treeSize = "30%"; IEnumerable<object> nonworkingHours = new List<object> { 0, 1, 2, 3, 4, 5, 6, 7, 18, 19, 20, 21, 22, 23 }; int[] nonworkingDays = new int[] { 0, 6 }; List<GanttChartTaskColumn> taskColumns = new List<GanttChartTaskColumn> { new GanttChartTaskColumn() { Label = "Tasks", Value = "label", Size = "50%" }, new GanttChartTaskColumn() { Label = "Date Start", Value = "dateStart", Size = "25%" }, new GanttChartTaskColumn() { Label = "Date End", Value = "dateEnd" } }; public List<GanttDataRecord> Records; protected override void OnInitialized() { Records = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Market Research", DateStart = "2021-01-10", DateEnd = "2021-03-10", Class = "product-team", Type = "project", Expanded = true, Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Questions and Answers", DateStart = "2021-01-10", DateEnd= "2021-02-10", Type = "task" }, new GanttDataRecord() { Label = "Data Analysis", DateStart = "2021-02-11", DateEnd= "2021-03-09", Type = "task" } } }, new GanttDataRecord() { Label = "Architecture & Planning", DateStart = "2021-03-10", DateEnd = "2021-03-31", Class = "marketing-team", Type = "task" }, new GanttDataRecord() { Label = "Development", DateStart = "2021-04-01", DateEnd = "2021-10-31", Class = "product-team", Type = "project", Expanded = true, Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "UI Designing", DateStart = "2021-04-01", DateEnd = "2021-08-31", Type = "task" }, new GanttDataRecord() { Label = "Business Logic Coding", DateStart = "2021-04-01", DateEnd = "2021-10-01", Type = "project", Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Data Transfer and Security", Duration = 200, Type = "task" }, new GanttDataRecord() { Label = "Data Manipulation", Duration = 400, Type = "task" } } }, new GanttDataRecord() { Label = "Database Connectivity", DateStart = "2021-04-01", DateEnd = "2021-05-30", Type = "task" } } }, new GanttDataRecord() { Label = "Quality Assurance", DateStart = "2021-11-01", DateEnd = "2021-12-31", Class = "dev-team", Type = "task" }, new GanttDataRecord() { Label = "Release v1", DateStart = "2021-01-01", DateEnd = "2021-01-31", Class = "design-team", Type = "task" } }; } private void ExportToXLSX() { gantt.ExportData("xlsx"); gantt.ClearSelection(); } }
The demo above generates the following .xlsx Excel file:
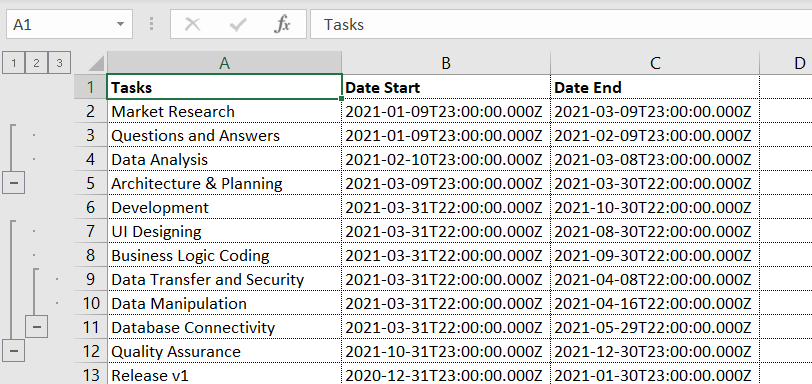
Note that Smart.Gantt with Project hierarchy can also maintain its hierarchy even when exported. In the Excel file, the rows can be collpased and expanded according to the task hierarchy
GanttChartDataExport
You can apply additional exporting options by creating a GanttChartDataExport object and setting it as a DataExport property of the Gantt
The GanttChartDataExport objects accepts the following properties:
ExportFiltered
- determines whether to export filtered items or not.
Acceptstrue | false(default)
FileName
- sets the exported file's name.
Acceptsstring
-default: "jqxGanttChart"
ItemType
- determines the type of items that is going to be exported.
AcceptsGanttChartDataExportItemType.Task(default) | GanttChartDataExportItemType.Resource