Editor - Image Upload
Smart.Editor allows you to upload the user attached images in the server before they are displayed in the editor content area.
Set up the Editor
The Image toolbar item represents a Window Modal that allows users to attach images which will be placed in the editor content area.
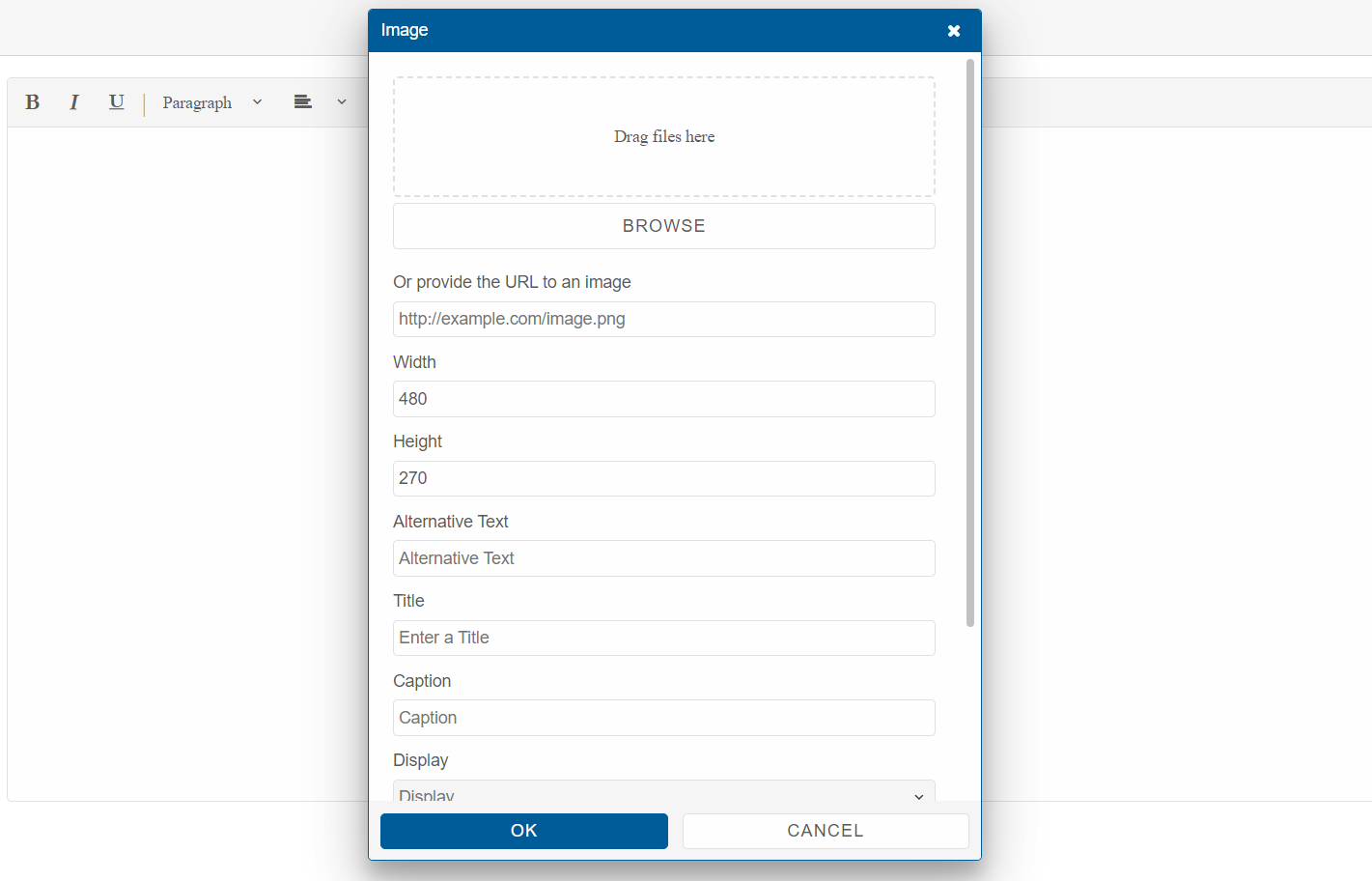
Create an Editor and set the UploadUrl
property to the url of the file upload handler and enable AutoUpload
.
@inject NavigationManager navigationManager; <Editor UploadUrl="@($"{navigationManager.BaseUri}api/fileupload")" AutoUpload="true"></Editor>
Set File Upload Handler
To handle the uploaded files, first create a "Controllers" folder. Inside create a new file called FileUploadController.cs
The handler must return a JSON object with a "file_url" or "fileURL" property that points to the url of the uploaded image
The following code uploads the receivied image and returns a JSON response:
using Microsoft.AspNetCore.Mvc; using Microsoft.AspNetCore.Hosting; using System.IO; using System.Threading.Tasks; namespace MyBlazorApp.Controllers { [Route("api/[controller]")] [ApiController] public class FileUploadController : ControllerBase { private readonly IWebHostEnvironment _hostingEnvironment; public FileUploadController(IWebHostEnvironment hostingEnvironment) { _hostingEnvironment = hostingEnvironment; } [HttpPost] public async Task<IActionResult> UploadFile([FromForm] IFormCollection form) { var file = form.Files[0]; if (file == null || file.Length == 0) { return BadRequest("No file uploaded."); } var uploadsFolder = Path.Combine(_hostingEnvironment.WebRootPath, "uploads"); // Ensure the uploads directory exists if (!Directory.Exists(uploadsFolder)) { Directory.CreateDirectory(uploadsFolder); } var filePath = Path.Combine(uploadsFolder, file.FileName); // Save the file using (var stream = new FileStream(filePath, FileMode.Create)) { await file.CopyToAsync(stream); } var fileUrl = $"{Request.Scheme}://{Request.Host}/uploads/{file.FileName}"; return Ok(new { file_url = fileUrl }); } } }
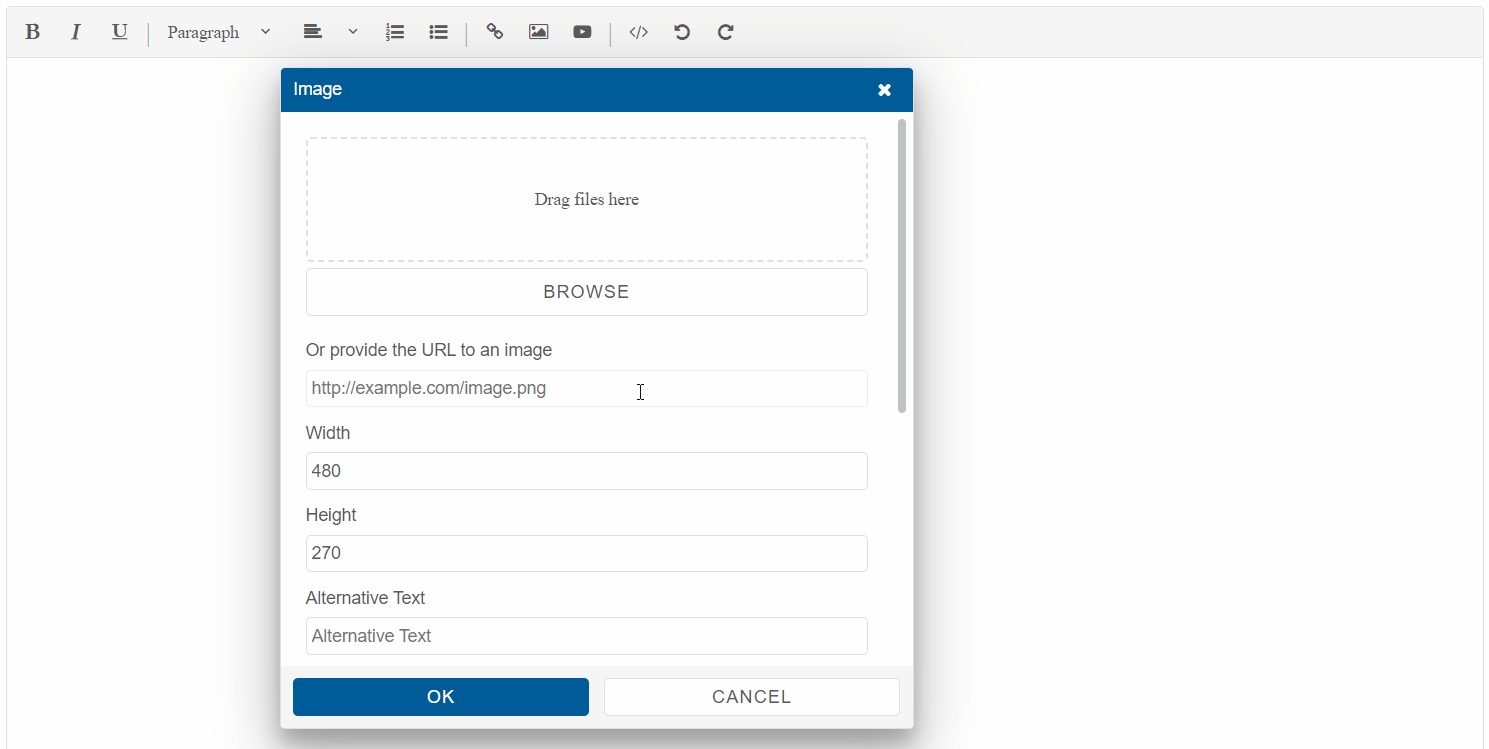
The image is dsiplayed in the editor content area and successfully uploaded on the server:
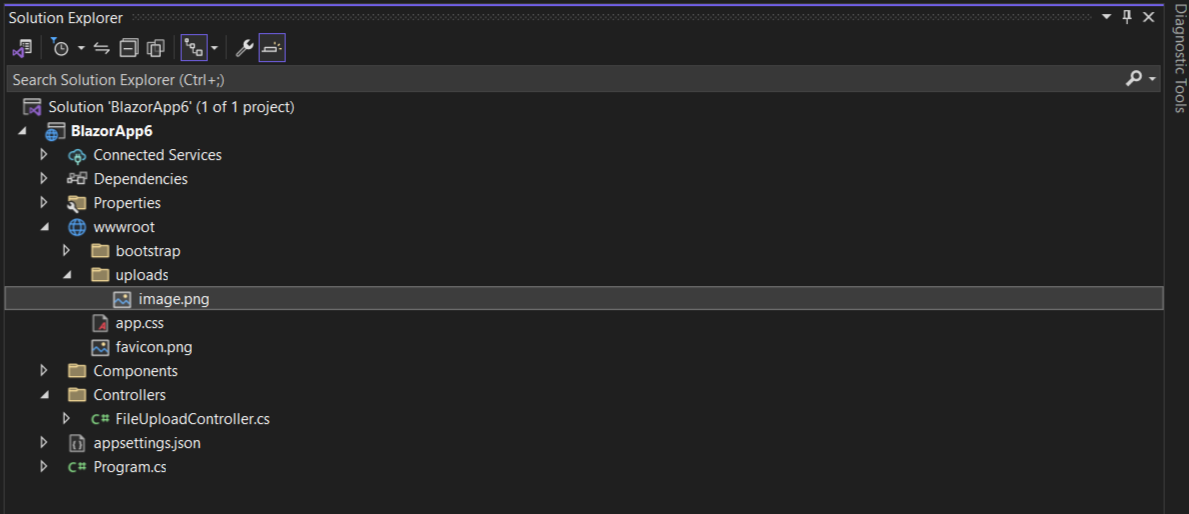