Refresh Data
Smart.Grid allows refreshing data, as well as dynamically adding or removing columns and rows
Smart.Grid can automatically keep track of changes to the DataSource array bounded to the Grid.
In the demo below, the Grid data will change everytime we refresh the dataRecords array
<Grid @ref="grid" DataSource="@dataRecords"> <Columns> <Column DataField="FirstName" Label="First Name"></Column> <Column DataField="LastName" Label="Last Name"></Column> <Column DataField="ProductName" Label="Product"></Column> <Column DataField="Quantity" Label="Quantity" DataType="number"></Column> <Column DataField="Price" Label="Unit Price" DataType="number" CellsFormat="c2"></Column> <Column DataField="Total" Label="Total" DataType="number" CellsFormat="c2"></Column> </Columns> </Grid> <Button OnClick="OnUpdateSource">Update New DataSource</Button> @code{ private Grid grid; private List<DataRecord> dataRecords; protected override void OnInitialized() { base.OnInitialized(); dataRecords = dataService.GenerateData(100); } private void OnUpdateSource() { dataRecords = dataService.GenerateData(100); } }
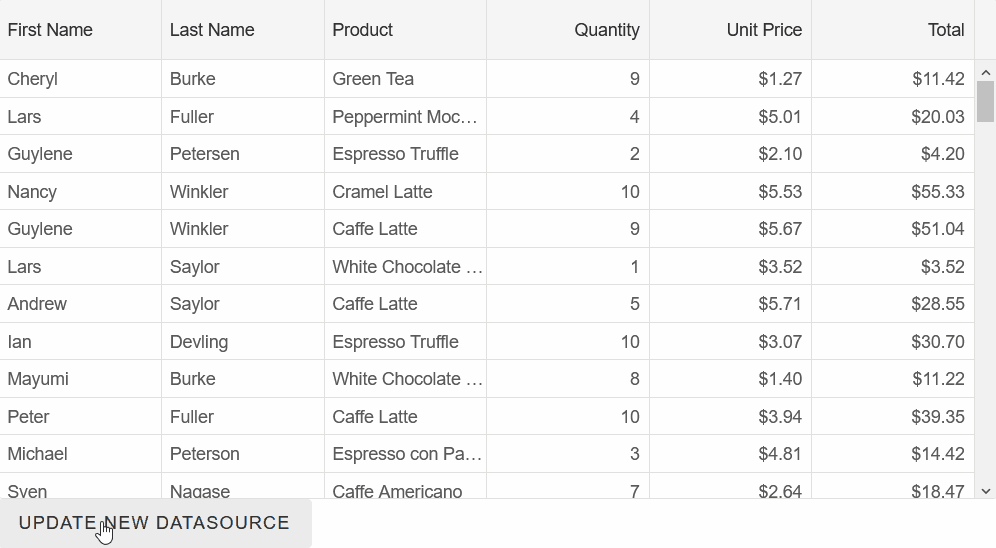
Dynamically Modify Data
In addition to refreshing data, it is also possible to add, update or remove Rows and modify Cell Values
To add or remove rows, you need to modify the DataSource array. Smart.Grid will then automatically make the changes to the Grid
//Add Row protected void OnAddNewRow() { dataRecords = new List<DataRecord>(dataRecords); dataRecords.Add(dataService.GenerateData(1)[0]); } //Remove Row at index private void RemoveFirstRow() { dataRecords = new List<DataRecord>(dataRecords); dataRecords.RemoveAt(0); }
To update row or cell value, you need to invoke the UpdateRow
and SetCellValue
Grid methods
//Update Row at index private void OnUpdateFirstRow() { grid.UpdateRow(0, dataService.GenerateData(1)[0]); } //Change Cell value at specific column of a given row private void OnUpdateFirstCell() { grid.SetCellValue(0, "FirstName", "Andrea"); }
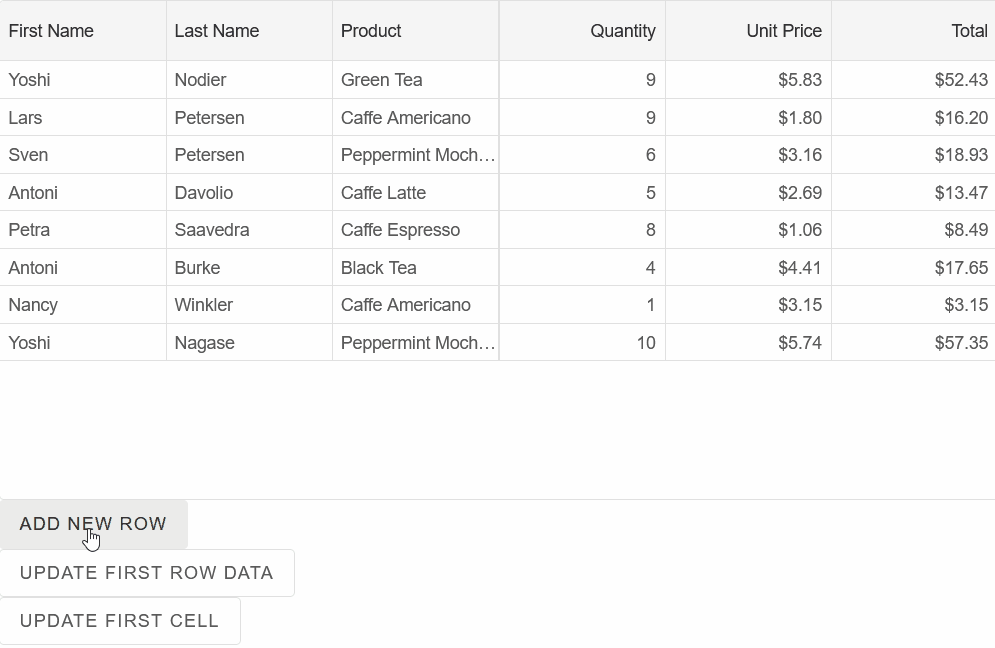