Creating a TypeScript React app with Smart UI for React
Smart UI for React components are available in two forms - Javascript & Typescript. When you use Smart UI for React with Typescript, the TSX version of the component is used i.e we have typed properties, method arguments and VS Code intellisense built-in.In this page, you will learn how to use Smart UI for React with Typescript.
Getting Started
1. Installation
The easiest way to start with React is to use create-react-app. To scaffold your project structure, follow the installation instructions.
Create React App is an officially supported way to create single-page React applications. It offers a modern build setup with no configuration
2. Typescript template
You can start a new TypeScript app using templates. To use ReactJS TypeScript template, append --template typescript to the creation commandcreate-react-app my-app --template typescript
3. Open my-app
cd my-app
Install Smart UI for React
npm install smart-webcomponents-react
4. Setup App.tsx
cd src/app.tsx. Open app.tsx in a text editorReplace its contents with:
import React from "react"; import ReactDOM from "react-dom"; import 'smart-webcomponents-react/source/styles/smart.default.css'; import { Smart, Grid, GridBehavior, GridAppearance, GridPaging, GridPager, GridSorting, GridEditing, GridColumn } from 'smart-webcomponents-react/grid'; class App extends React.Component { behavior: GridBehavior = { columnResizeMode: 'growAndShrink' } appearance: GridAppearance = { alternationCount: 2, showRowHeader: true, showRowHeaderSelectIcon: true, showRowHeaderFocusIcon: true } paging: GridPaging = { enabled: true } pager: GridPager = { visible: true } sorting: GridSorting = { enabled: true } editing: GridEditing = { enabled: true } dataSource = new Smart.DataAdapter({ dataSource: [ { "firstName": "Beate", "lastName": "Wilson", "productName": "Caramel Latte"}, { "firstName": "Ian", "lastName": "Nodier", "productName": "Caramel Latte"}, { "firstName": "Petra", "lastName": "Vileid", "productName": "Green Tea"}, { "firstName": "Mayumi", "lastName": "Ohno", "productName": "Caramel Latte"}, { "firstName": "Mayumi", "lastName": "Saylor", "productName": "Espresso con Panna"}, { "firstName": "Regina", "lastName": "Fuller", "productName": "Caffe Americano" }, { "firstName": "Regina", "lastName": "Burke", "productName": "Caramel Latte"}, { "firstName": "Andrew", "lastName": "Petersen", "productName": "Caffe Americano"}, { "firstName": "Martin", "lastName": "Ohno", "productName": "Espresso con Panna"}, { "firstName": "Beate", "lastName": "Devling", "productName": "Green Tea"}, { "firstName": "Sven", "lastName": "Devling", "productName": "Espresso Truffle"}, { "firstName": "Petra", "lastName": "Burke", "productName": "Peppermint Mocha Twist"}, { "firstName": "Marco", "lastName": "Johnes", "productName": "Caffe Mocha"} ], dataFields: [ 'firstName: string', 'lastName: string', 'productName: string' ] }) columns: GridColumn[] = [{ label: 'First Name', dataField: 'firstName' }, { label: 'Last Name', dataField: 'lastName' }, { label: 'Product', dataField: 'productName' } ] componentDidMount() { } render() { return ( <div> <Grid dataSource={this.dataSource} columns={this.columns} appearance={this.appearance} behavior={this.behavior} paging={this.paging} pager={this.pager} sorting={this.sorting} editing={this.editing} > </Grid> </div> ); } } ReactDOM.render(<App />, document.querySelector("#root")); export default App;The above adds our React Grid to your application and enables paging, sorting, editing and columns resize features.
5. Run the React Typescript app
Inside the newly created project, you can run some built-in commands
npm start or yarn start
Runs the app in development mode. Open http://localhost:3000 to view it in the browser.
The page will automatically reload if you make changes to the code. You will see the build errors and lint warnings in the console.
6. Output
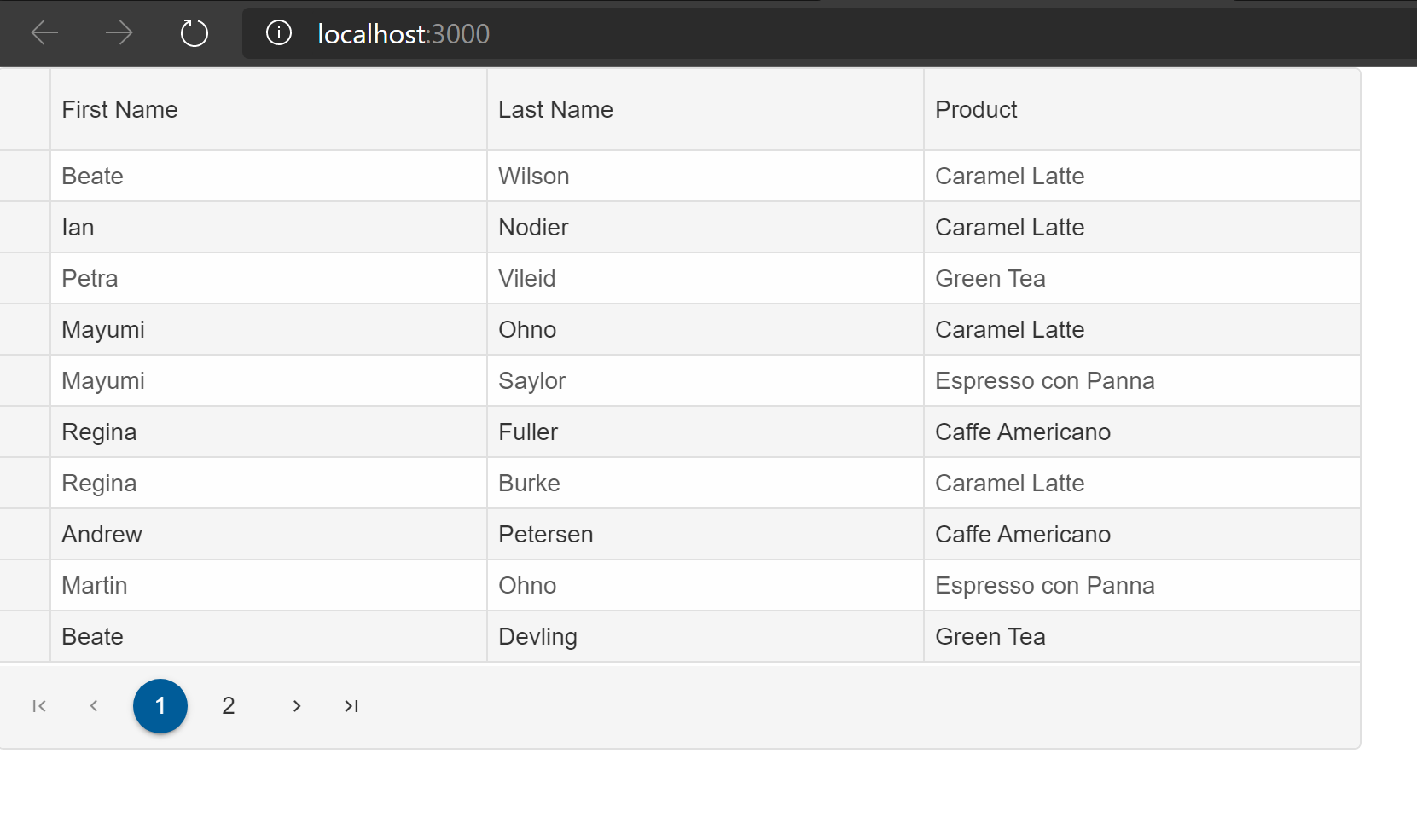