Blazor - Get Started with Smart.CheckInput
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic Check Input
Smart.CheckInput is an input that allows selecting from a predefined checkbox style options drop down list.
The list of avaible options is set using the DataSource property.
- Add the CheckInput component to the Pages/Index.razor file
<CheckInput></CheckInput>
- Inside the
@code
block, create an array of elements and set it as DataSource of the component<CheckInput DataSource="drinks"></CheckInput> @code{ private string[] drinks = new string[]{"Water", "Juice", "Soda", "Tea", "Beer", "Wine", }; }
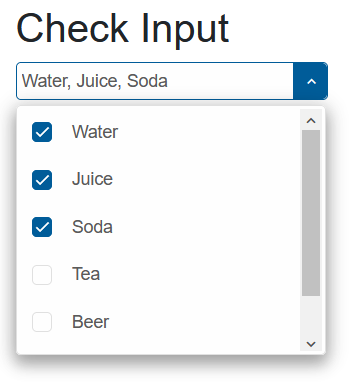
Readonly
When the Readonly property is set to true, the input field will be disabled and changes will be allowed only by using the dropdown list.
<CheckInput DataSource="drinks" Readonly="true"></CheckInput>

CheckInput Methods
Smart.CheckInput can be easily controlled programmatically using some of the built-in Methods.
Create buttons that can control the Open/Close state of the dropdown list:
<Button OnClick="Open">Open</Button> <Button OnClick="Close">Close</Button> <CheckInput @ref="checkInput" DataSource="drinks"></CheckInput> @code{ CheckInput checkInput; private string[] drinks = new string[]{"Water", "Juice", "Soda", "Tea", "Beer", "Wine", }; private void Open(){ checkInput.Open(); } private void Close(){ checkInput.Close(); } }
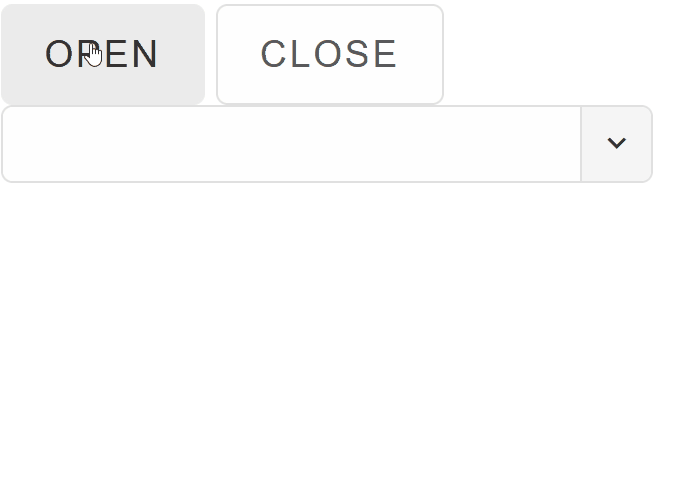
CheckInput Customization
Smart.CheckInput can be styled in different ways by setting the Class property.
The CheckInput can be default
, outlined
or underlined
<CheckInput DataSource="drinks" Class="underlined"></CheckInput>
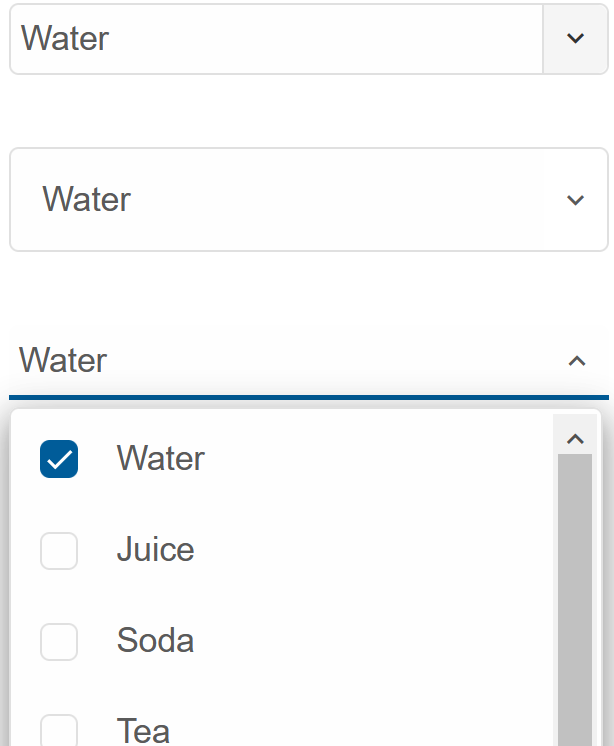
CheckInput Events
Smart.CheckInput provides an OnChange Event that can help you expand the component's functionality.
The event object has unique event.detail parameters.
OnChange
- triggered when the selection is changed.
Event Details
: string label, dynamic oldLabel, dynamic oldValue, dynamic value
The demo below uses the OnChange Event to display the selected options:
<h2>Selected value: @values</h2> <CheckInput DataSource="drinks" OnChange="OnChange"></CheckInput> @code{ private string[] drinks = new string[]{"Water", "Juice", "Soda", "Tea", "Beer", "Wine", }; string values; private void OnChange(Event ev){ if(ev.ContainsKey("Detail")){ CheckInputChangeEventDetail detail = ev["Detail"]; values = detail.Value; } } }
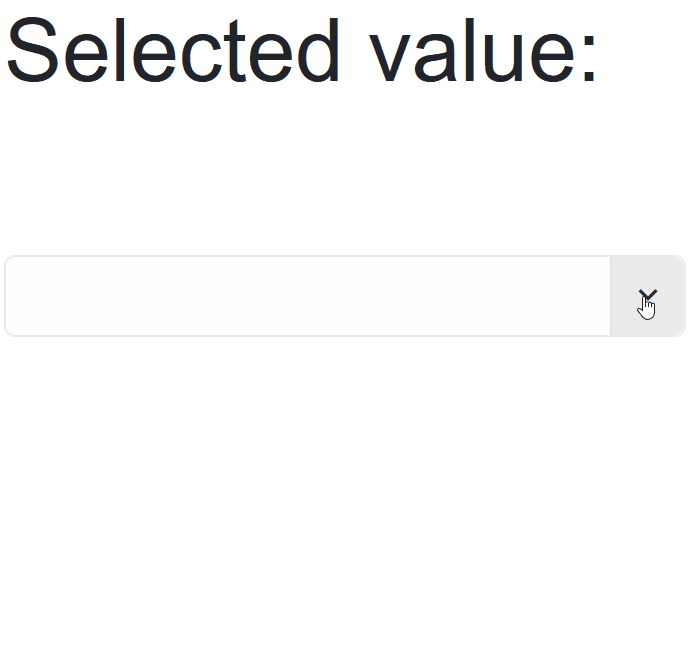
Two-way Value Binding
The CheckInput component also supports two-way value binding:
<h3>@textValue</h3> <CheckInput DataSource="drinks" @bind-Value = "@textValue"></CheckInput> @code{ private string[] drinks = new string[]{"Water", "Juice", "Soda", "Tea", "Beer", "Wine" }; string textValue = ""; }
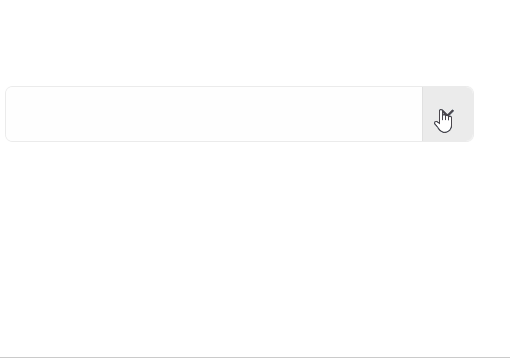