Blazor CardView Editing
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup the Blazor Smart.CardView
Follow the Get Started with Smart.CardView guide to set up the component.
CardView Editing
When the property Editable is set to true
, card data can be modified in an edit dialog. Editor elements are dynamically created based on DataSource and Columns settings.
The edit dialog also allows directly navigating to other cards by pressing the "Previous record" and "Next record" buttons in its header.
The edit dialog is opened when you click on a card.
You can also add new cards by clicking the "+" button. This functionality is enabled when you set the AddNewButton property to true
.
They can be set like this:
<CardView Editable AddNewButton />
Example
Here is a full example of a CardView with editing and adding new card functionalities enabled:
@using Smart.Blazor.Demos.Data @inject RandomDataService dataService <CardView DataSource="dataRecords" TitleField="FirstName" CoverField="Attachments" Columns="@columns" AllowDrag Editable AddNewButton /> @code { List<CardViewColumn> columns = new List<CardViewColumn>() { new CardViewColumn() { DataField = "FirstName", Label = "First Name", Icon = "firstName" }, new CardViewColumn() { DataField = "LastName", Label = "Last Name", Icon = "lastName" }, new CardViewColumn() { DataField = "Birthday", Label = "Birthday", Icon = "birthday", FormatSettings = new Dictionary<string, string>() { { "formatString", "d" } } }, new CardViewColumn() { DataField = "PetName", Label = "Pet Name", Icon = "petName" }, new CardViewColumn() { DataField = "Country", Label = "Country", Icon = "country" }, new CardViewColumn() { DataField = "ProductName", Label = "Product Name", Icon = "productName" }, new CardViewColumn() { DataField = "Price", Label = "Price", Icon = "price", FormatSettings = new Dictionary<string, string>() { { "formatString", "c2" } } }, new CardViewColumn() { DataField = "Quantity", Label = "Quantity", Icon = "quantity" }, new CardViewColumn() { DataField = "TimeOfPurchase", Label = "Time of Purchase", Icon = "timeOfPurchase", FormatSettings = new Dictionary<string, string>() { { "formatString", "hh:mm tt" } } }, new CardViewColumn() { DataField = "Expired", Label = "Expired", Icon = "expired" }, new CardViewColumn() { DataField = "Attachments", Label = "Attachments", Image = true } }; private List<DataRecord> dataRecords; protected override void OnInitialized() { base.OnInitialized(); dataRecords = dataService.GenerateData(50); } }Result:
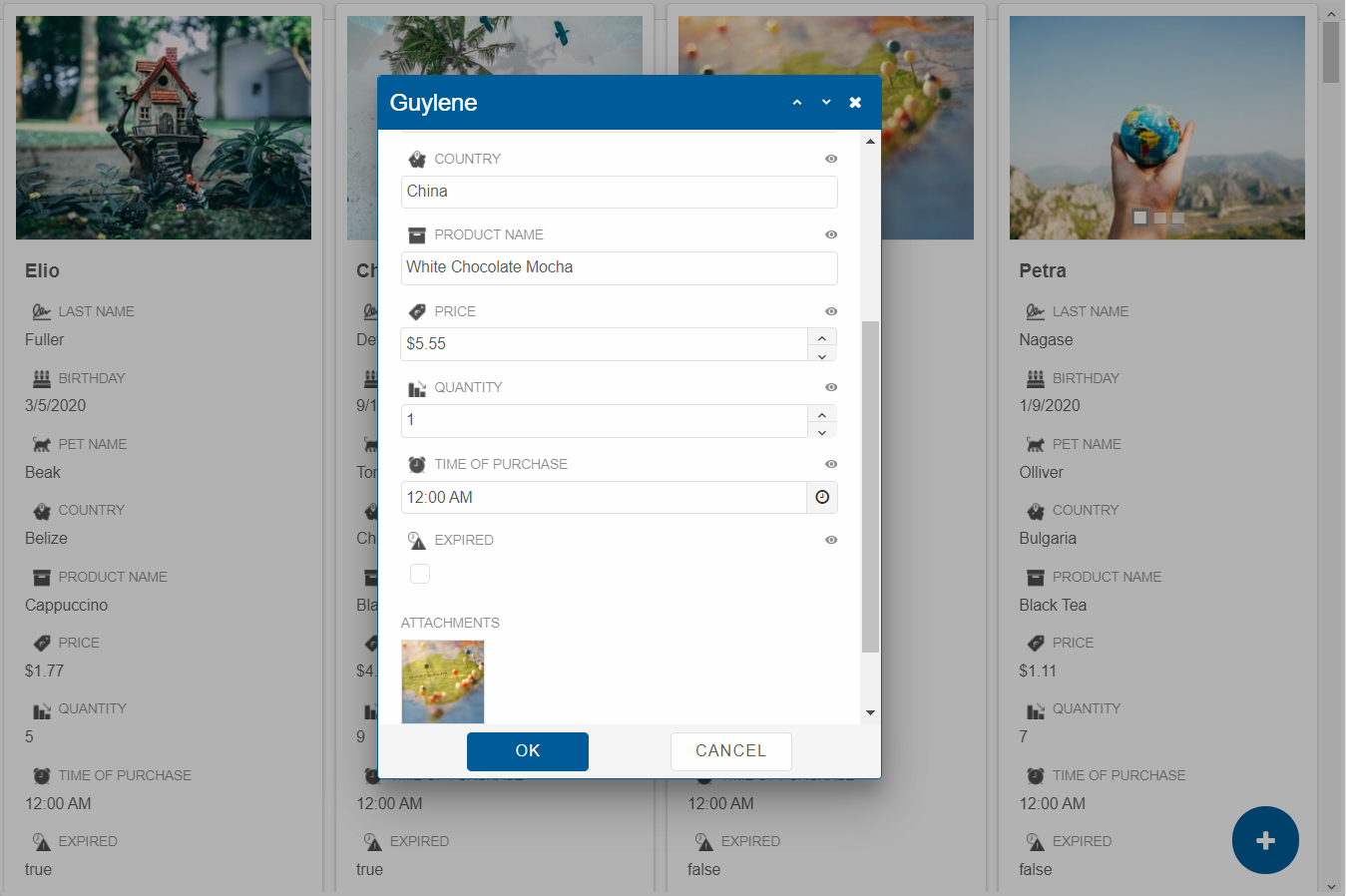