Blazor - Get Started with Smart.Scheduler
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic Scheduler
- Add the Scheduler component to the Pages/Index.razor file.And set its
DataSource
andHourStart
properties.<Scheduler DataSource="@dataSource" HourStart="@hourStart"></Scheduler>
- Create an example Events data and bind it to the Scheduler using the
Datasource
property.
Each Event in the Scheduler requires a Label and DateStart. If DateEnd is not given, the Event's period is 1 hour.
Smart.Scheduler also offers many other properties to customize and add functionality to Events.
Inside the@code
block set the start hour of the Scheduler.int hourStart = 9; List<SchedulerDataSource> dataSource = new List<SchedulerDataSource>() { new SchedulerDataSource() { Label = "Google AdWords Strategy", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 10, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 11, 30, 0), BackgroundColor = "#E67C73" }, new SchedulerDataSource() { Label = "New Brochures", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 12, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 15, 0, 0), BackgroundColor = "#8E24AA" }, new SchedulerDataSource() { Label = "Brochure Design Review", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 15, 30, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 18, 15, 0), }, new SchedulerDataSource() { Label = "Website Re-Design Plan", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 11, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 12, 15, 0), }, new SchedulerDataSource() { Label = "Rollout of New Website and Marketing Brochures", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 12, 15, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 14, 30, 0), }, new SchedulerDataSource() { Label = "Update Sales Strategy Documents", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 15, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 16, 0, 0), }, new SchedulerDataSource() { Label = "Non-Compete Agreements", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+2, 10, 30, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+2, 12, 0, 0), }, new SchedulerDataSource() { Label = "Approve Hiring of John Jeffers", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+2, 14, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+2, 16, 0, 0), }, new SchedulerDataSource() { Label = "Conference", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+3, 10, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+3, 12, 0, 0), }, new SchedulerDataSource() { Label = "Update NDA Agreement", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+3, 12, 30, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+3, 17, 0, 0), }, new SchedulerDataSource() { Label = "Update Employee Files with New NDA", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 10, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 13, 30, 0), }, new SchedulerDataSource() { Label = "Submit Questions Regarding New NDA", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 14, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 15, 0, 0), }, new SchedulerDataSource() { Label = "Submit Signed ND", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 16, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 17, 30, 0), }, new SchedulerDataSource() { Label = "Review Revenue Projections", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+5, 11, 45, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+5, 15, 0, 0), } };
- By clicking on an emplty time slot, the user can create their own Events through an easy-to-use modal form.
- By clicking on an already existing Event, the user can modify or delete the data.
Note that these changes do not modify the original
DataSource
Customize Views
Smart.Scheduler allows you to view the events of a particular period of time either by using any of the default
views or by creating custom ones.
In the @code
block declare the Array of views as well as the defualt view
@code{ private IEnumerable<SchedulerViewType> views = new List<SchedulerViewType>() { SchedulerViewType.Day, SchedulerViewType.Week, SchedulerViewType.Agenda, SchedulerViewType.Month }; SchedulerViewType view = SchedulerViewType.Week; ..... }
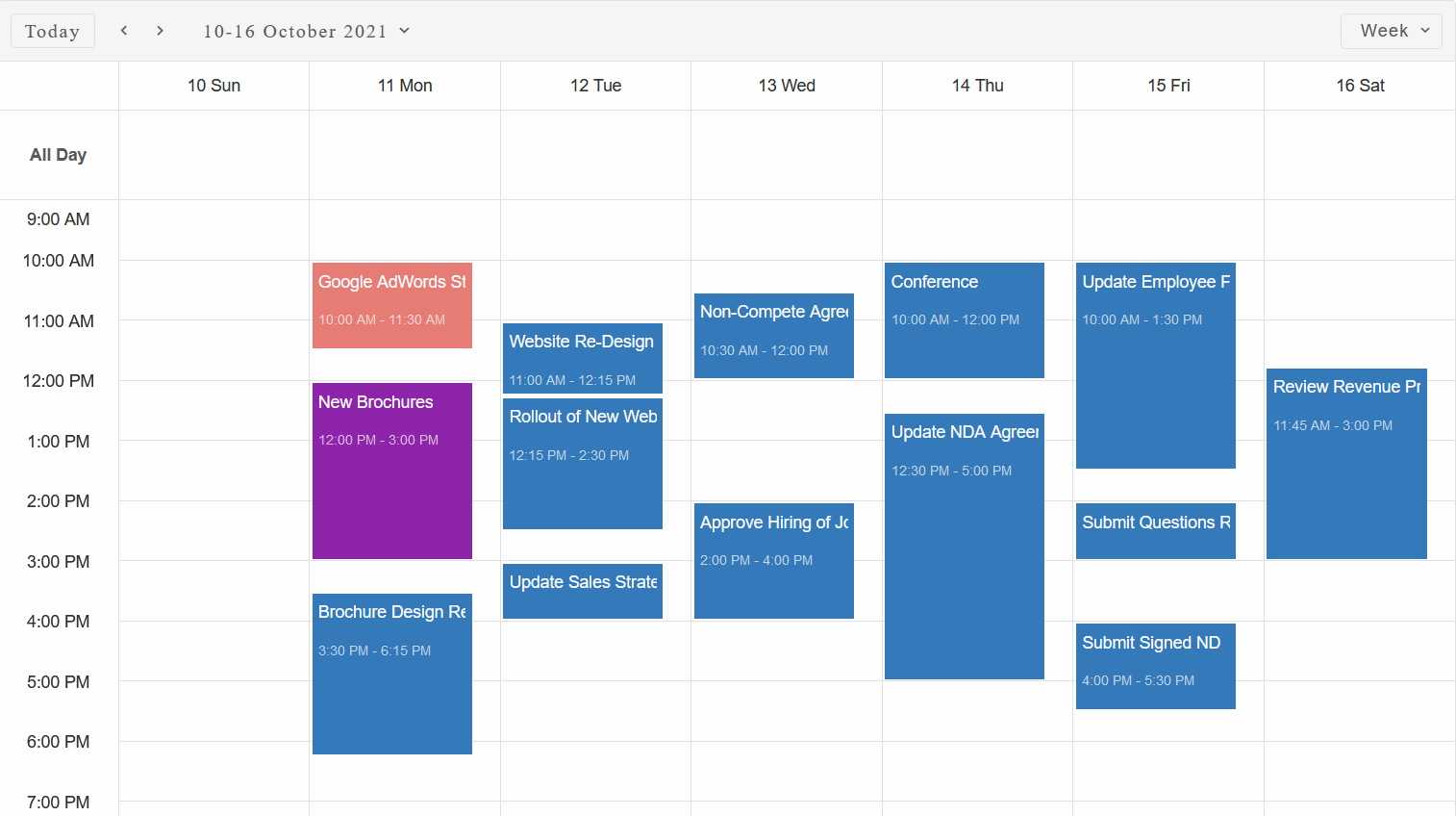
Repeating Events
The SchedulerDataSourceRepeat
object allows to set a Repeating Event.
Add the following Event to the dataSource:
List<SchedulerDataSource> dataSource = new List<SchedulerDataSource>() { new SchedulerDataSource() { Label = "Breakfast", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day-1, 9, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day-1, 10, 0, 0), BackgroundColor = "#00A300", Description = "Have Breakfast before work", Repeat = new SchedulerDataSourceRepeat(){ RepeatFreq = SchedulerRepeatFreq.Daily, RepeatInterval = 1 } }, .... }
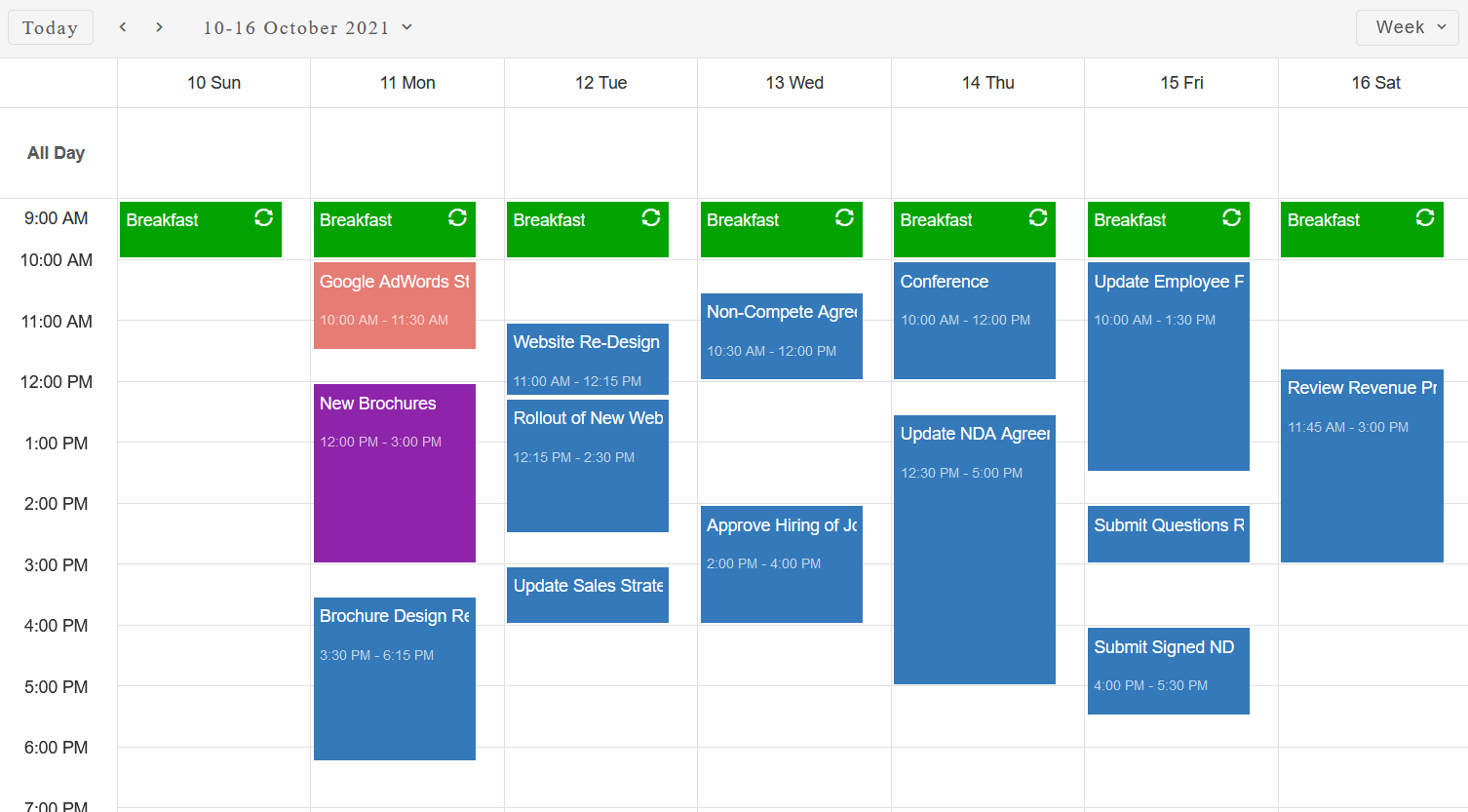
Event Notifications
Smart.Scheduler allows you to create notification that can appear before the start of the Event.
Create an Event and a Notification that starts one day before.
ListdataSource = new List () { ..... new SchedulerDataSource() { Label = "Bryan's Birthday", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 9, 0, 0).AddDays(1), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 10, 30, 0).AddDays(1), AllDay = true, BackgroundColor = "#880E4F", Notifications = new List<SchedulerDataSourceNotification>() { new SchedulerDataSourceNotification() { Interval = 1, Type = SchedulerNotificationType.Days, Time = new int[] { DateTime.Now.Hour, DateTime.Now.Minute }, Message = "Bryan'ss birthday is tomorrow! By him a present !", IconType = "warning" } } } }
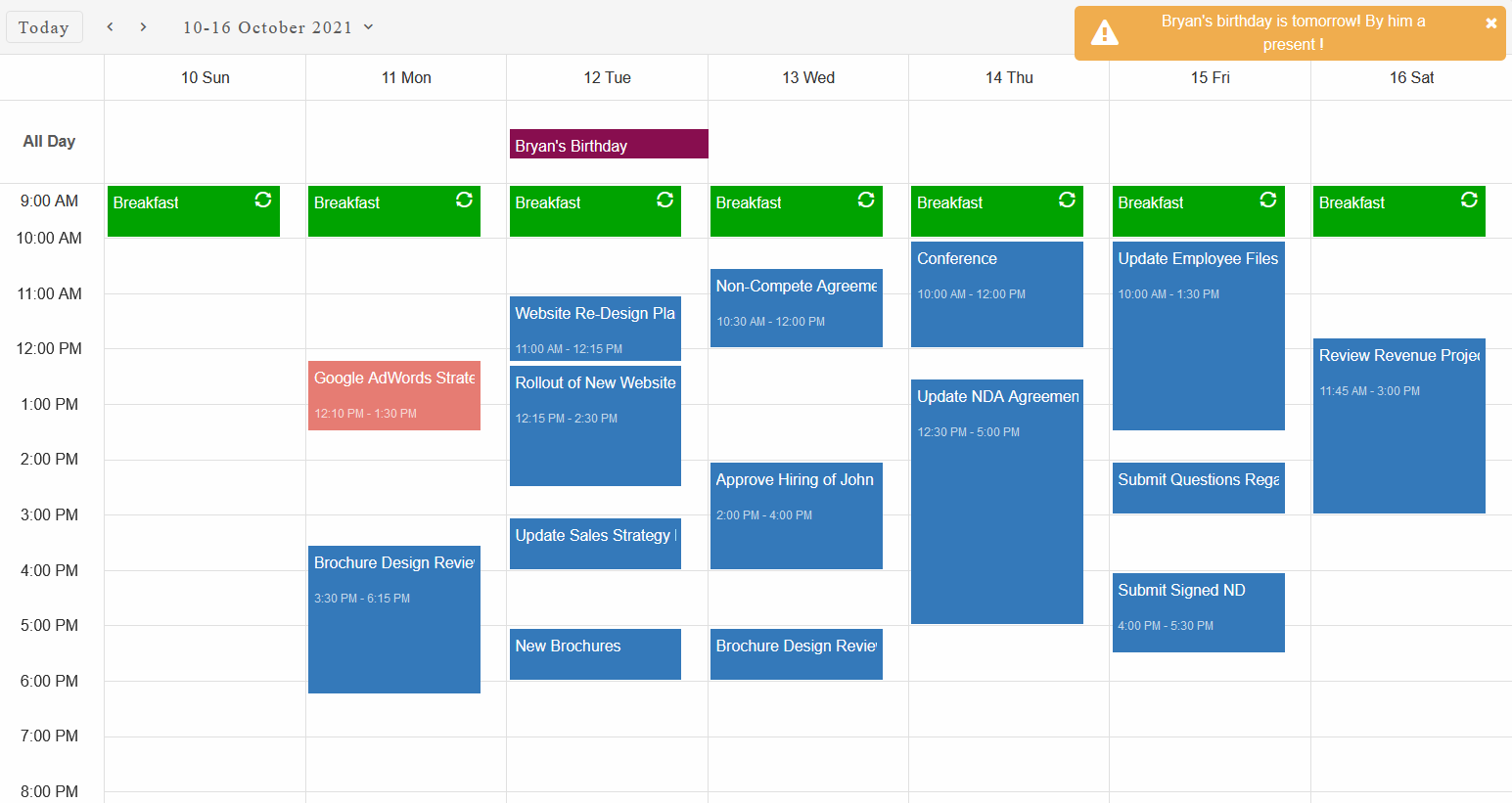
Scheduler Resources
Smart.Scheduler Resources allow you to group and style the assigned Events.
They are assigned via the Resources
property of an Event.
Inside the @code
block, declare the resources array.
private IEnumerable<ISchedulerResource> resources = new List<ISchedulerResource>(){ new SchedulerResource() { Label = "Priority", Value = "priorityId", DataSource = new object[] { new Dictionary<string, object>() { { "label", "Low Priority" }, { "id", 1 }, { "backgroundColor", "#1e90ff" } }, new Dictionary<string, object>() { { "label", "Medium Priority" }, { "id", 2 }, { "backgroundColor", "#ff9747" } } } }, new SchedulerResource() { Label = "Employee", Value = "employeeId", DataSource = new object[] { new Dictionary<string, object>() { { "label", "Andrew Heart" }, { "id", 1 }, { "age", 28 }, { "discipline", "ABS, Fitball, StepFit" }, { "backgroundColor", "#28a745" } }, new Dictionary<string, object>() { { "label", "Nancy Johnson" }, { "id", 2 }, { "age", 31 }, { "discipline", "Tennis, Yoga, Swimming" }, { "backgroundColor", "#8f73af" } } } } };
Then exapnd the SchedulerDataSource class by creating a new SchedulerDataSourceWithResource that can read the additional id properties of the Resources.
private class SchedulerDataSourceWithResource : SchedulerDataSource { private int _priorityId; [JsonPropertyName("priorityId")] [JsonIgnore] public int PriorityId { get { return this._priorityId; } set { var oldValue = this._priorityId; this._priorityId = value; NotifyPropertyChanged("PriorityId", "priorityId", oldValue, value, false); } } private int _employeeId; [JsonPropertyName("employeeId")] [JsonIgnore] public int EmployeeId { get { return this._employeeId; } set { var oldValue = this._employeeId; this._employeeId = value; NotifyPropertyChanged("EmployeeId", "employeeId", oldValue, value, false); } } private void NotifyPropertyChanged(string propertyName, string instancePropertyName, object oldValue, object newValue, bool isEnum) { if (null == Properties) { Properties = new Dictionary<string, object>(); } Properties[instancePropertyName] = newValue; } };
Finally, modify the dataSource array by making it of type SchedulerDataSourceWithResource
and setting the resources ids to the Events you want to group.
List<SchedulerDataSourceWithResource> dataSource = new List<SchedulerDataSourceWithResource>() { new SchedulerDataSourceWithResource() { Label = "Breakfast", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day-1, 9, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day-1, 10, 0, 0), BackgroundColor = "#00A300", Description = "Have Breakfast before work", Repeat = new SchedulerDataSourceRepeat(){ RepeatFreq = SchedulerRepeatFreq.Daily, RepeatInterval = 1 }, }, new SchedulerDataSourceWithResource() { Label = "Google AdWords Strategy", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 12, 10, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 13, 30, 0), BackgroundColor = "#E67C73", EmployeeId=1, PriorityId=2 }, new SchedulerDataSourceWithResource() { Label = "New Brochures", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 17, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 18, 0, 0), EmployeeId=1, PriorityId=1 }, new SchedulerDataSourceWithResource() { Label = "Brochure Design Review", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+2, 17, 00, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+2, 18, 0, 0), EmployeeId=1, PriorityId=2 }, new SchedulerDataSourceWithResource() { Label = "Brochure Design Review", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 15, 30, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 18, 15, 0), EmployeeId=1, PriorityId=1 }, new SchedulerDataSourceWithResource() { Label = "Website Re-Design Plan", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 11, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 12, 15, 0), EmployeeId=1, PriorityId=2 }, new SchedulerDataSourceWithResource() { Label = "Rollout of New Website and Marketing Brochures", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 12, 15, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 14, 30, 0), EmployeeId=2, PriorityId=1 }, new SchedulerDataSourceWithResource() { Label = "Update Sales Strategy Documents", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 15, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+1, 16, 0, 0), EmployeeId=1, PriorityId=2 }, new SchedulerDataSourceWithResource() { Label = "Non-Compete Agreements", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+2, 10, 30, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+2, 12, 0, 0), EmployeeId=2, PriorityId=1 }, new SchedulerDataSourceWithResource() { Label = "Approve Hiring of John Jeffers", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+2, 14, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+2, 16, 0, 0), EmployeeId=2, PriorityId=1 }, new SchedulerDataSourceWithResource() { Label = "Conference", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+3, 10, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+3, 12, 0, 0), EmployeeId=1, PriorityId=2 }, new SchedulerDataSourceWithResource() { Label = "Update NDA Agreement", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+3, 12, 30, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+3, 17, 0, 0), EmployeeId=1, PriorityId=1 }, new SchedulerDataSourceWithResource() { Label = "Update Employee Files with New NDA", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 10, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 13, 30, 0), EmployeeId=2, PriorityId=2 }, new SchedulerDataSourceWithResource() { Label = "Submit Questions Regarding New NDA", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 14, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 15, 0, 0), EmployeeId=1, PriorityId=2 }, new SchedulerDataSourceWithResource() { Label = "Submit Signed ND", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 16, 0, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+4, 17, 30, 0), EmployeeId=2, PriorityId=1 }, new SchedulerDataSourceWithResource() { Label = "Review Revenue Projections", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+5, 11, 45, 0), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day+5, 15, 0, 0), EmployeeId=2, PriorityId=2 }, new SchedulerDataSourceWithResource() { Label = "Bryan's Birthday", DateStart = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 9, 0, 0).AddDays(1), DateEnd = new DateTime(DateTime.Now.Year, DateTime.Now.Month, DateTime.Now.Day, 10, 30, 0).AddDays(1), AllDay = true, BackgroundColor = "#880E4F", Notifications = new List<SchedulerDataSourceNotification>() { new SchedulerDataSourceNotification() { Interval = 1, Type = SchedulerNotificationType.Days, Time = new int[] { DateTime.Now.Hour, DateTime.Now.Minute }, Message = "Bryan's birthday is tomorrow! Buy him a present !", IconType = "warning" } }, EmployeeId=1, PriorityId=1 }, };
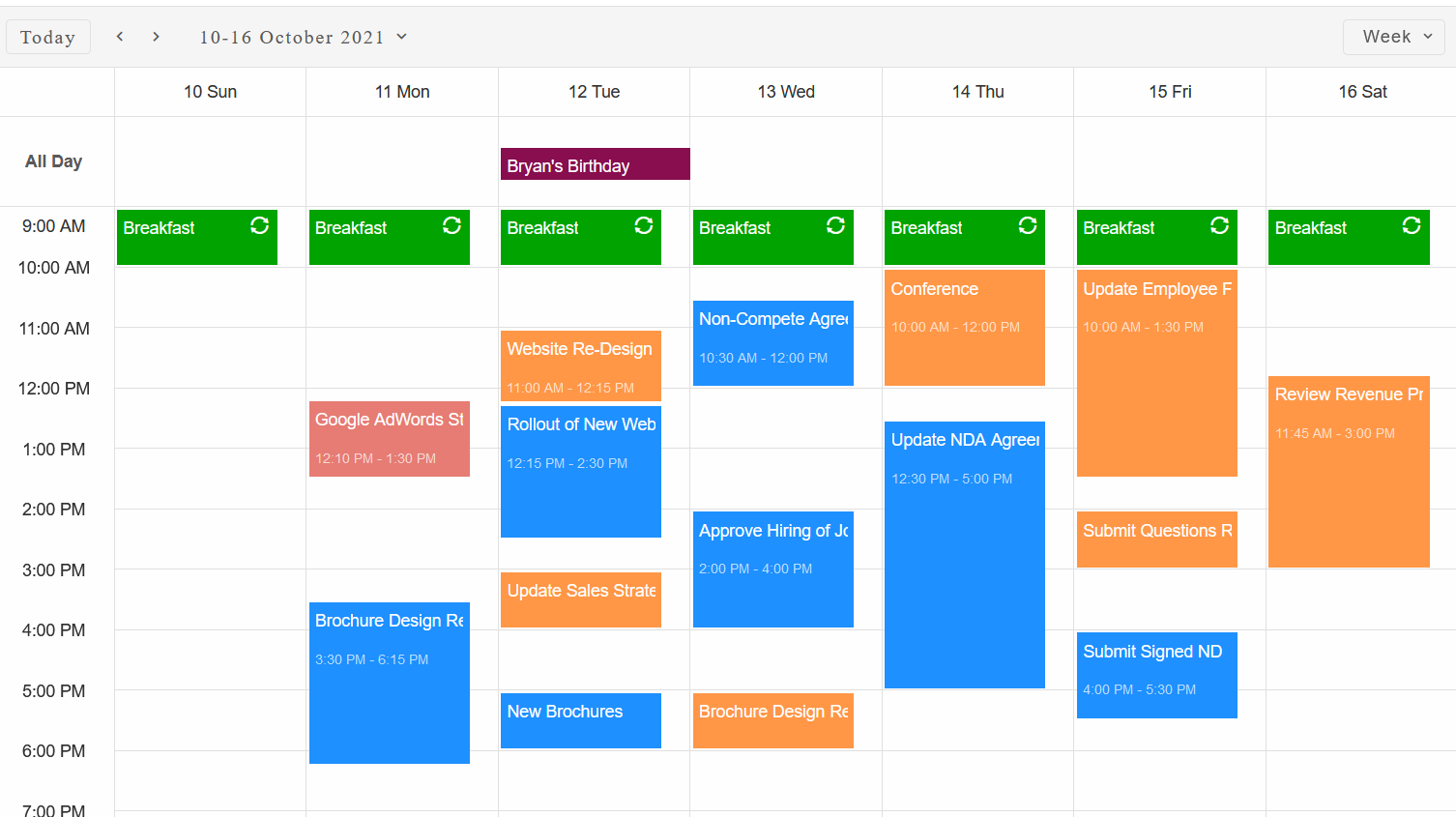