Blazor CardView Scroll
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup the Blazor Smart.CardView
Follow the Get Started with Smart.CardView guide to set up the component.
CardView Scroll
Smart.CardView offers four scrolling modes (configurable through the property Scrolling). Its type is enum
and has the following values:
- Physical - default scrolling.
- Deferred - when scrolling, view is not updated until the scrollbar's thumb is released.
- Virtual - server-bound scrolling. Loads records on demand when the element is scrolled to their position.
- Infinite - server-bound scrolling. Loads only some records initially. Additional records are dynamically loaded when the scrollbar reaches the bottom of the element.
<CardView Scrolling="Scrolling.Deferred" />
Example
Here is a full example of CardView with Deferred scrolling mode:
@using Smart.Blazor.Demos.Data @inject RandomDataService dataService <CardView DataSource="dataRecords" TitleField="FirstName" CoverField="Attachments" Columns="@columns" Scrolling="Scrolling.Deferred" /> @code { List<CardViewColumn> columns = new List<CardViewColumn>() { new CardViewColumn() { DataField = "FirstName", Label = "First Name", Icon = "firstName" }, new CardViewColumn() { DataField = "LastName", Label = "Last Name", Icon = "lastName" }, new CardViewColumn() { DataField = "Birthday", Label = "Birthday", Icon = "birthday", FormatSettings = new Dictionary<string, string>() { { "formatString", "d" } } }, new CardViewColumn() { DataField = "PetName", Label = "Pet Name", Icon = "petName" }, new CardViewColumn() { DataField = "Country", Label = "Country", Icon = "country" }, new CardViewColumn() { DataField = "ProductName", Label = "Product Name", Icon = "productName" }, new CardViewColumn() { DataField = "Price", Label = "Price", Icon = "price", FormatSettings = new Dictionary<string, string>() { { "formatString", "c2" } } }, new CardViewColumn() { DataField = "Quantity", Label = "Quantity", Icon = "quantity" }, new CardViewColumn() { DataField = "TimeOfPurchase", Label = "Time of Purchase", Icon = "timeOfPurchase", FormatSettings = new Dictionary<string, string>() { { "formatString", "hh:mm tt" } } }, new CardViewColumn() { DataField = "Expired", Label = "Expired", Icon = "expired" }, new CardViewColumn() { DataField = "Attachments", Label = "Attachments", Image = true } }; private List<DataRecord> dataRecords; protected override void OnInitialized() { base.OnInitialized(); dataRecords = dataService.GenerateData(10000); } }When the CardView's deferred scrolling feature is enabled, the view stays frozen, while you scroll. The view is updated, after the scrolling has finished.
Result:
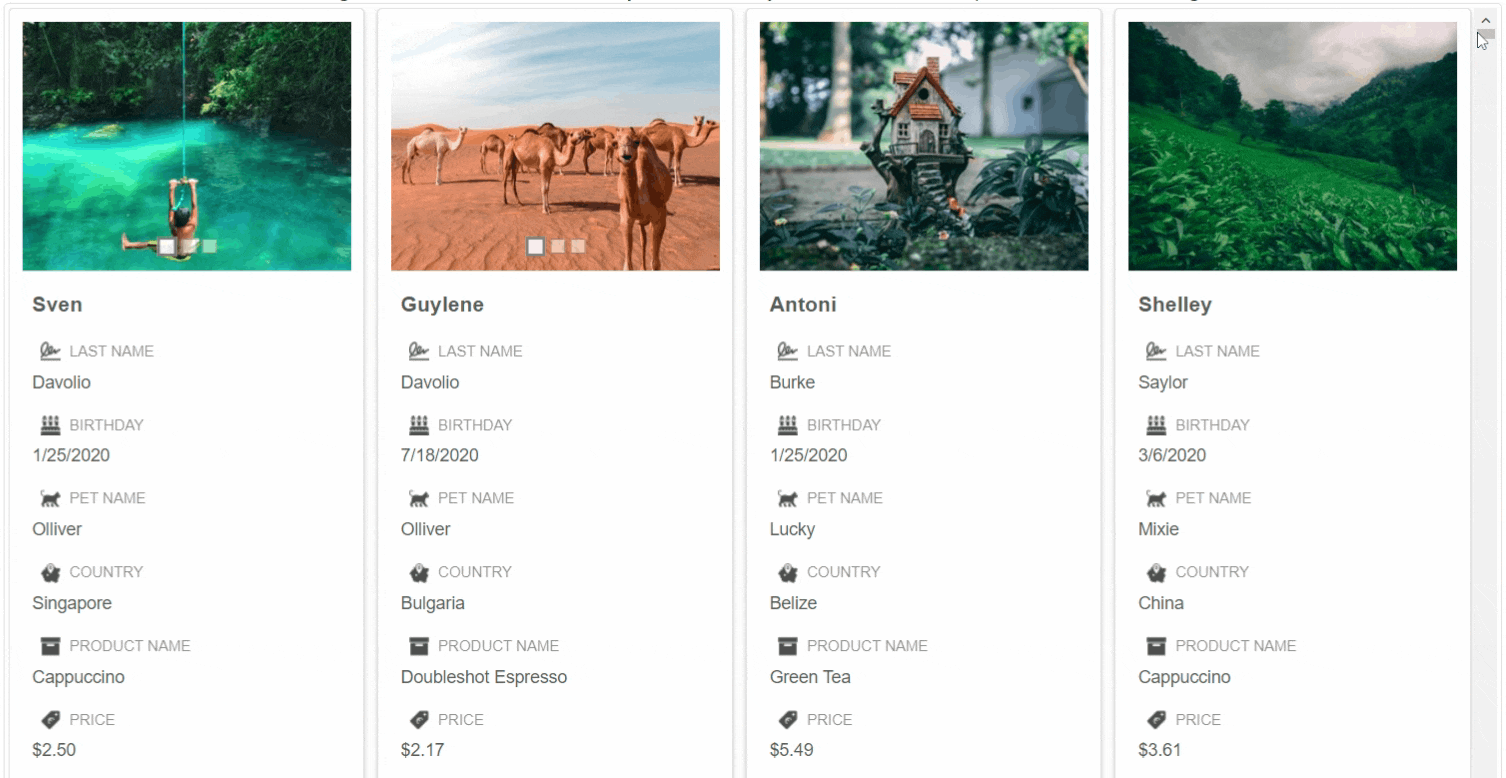