Blazor - Get Started with Smart.Calendar
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic Calendar
Smart.Calendar is a highly-customizable custom component that allows you to highlight and select a range of dates, while customizing date formats, language, layout and much more.
-
Add the Calendar component to the Pages/Index.razor file:
<Calendar></Calendar>
-
Set the First Day of the Week, default is
Sunday - 0
:<Calendar FirstDayOfWeek="1" ></Calendar>
-
Add default selected dates using the
SelectedDates
property:<Calendar FirstDayOfWeek="1" SelectedDates="selectedDates"></Calendar> @code{ string[] selectedDates = new string[]{"2022-01-18", "2022-01-19", "2022-01-20"}; }
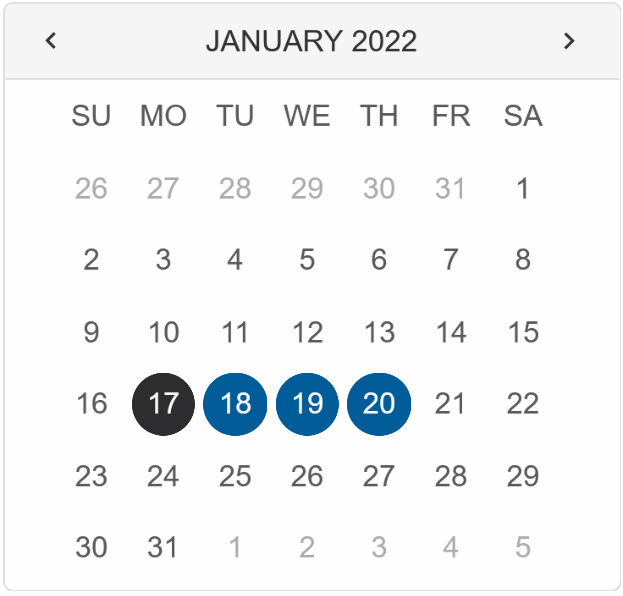
Display Mode
The initial display mode of the Calendar can be set to Month(default)
, Year
or Decade
:
<Calendar SelectedDates="selectedDates" DisplayMode="CalendarDisplayMode.Year"></Calendar>
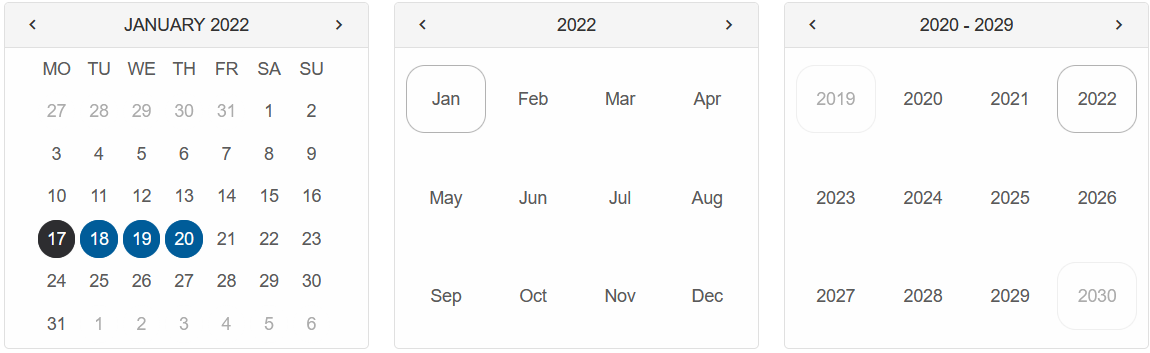
The Year/Decade mode can be diplayed as List
or Table(default)
with the DisplayModeView property:
<Calendar SelectedDates="selectedDates" DisplayMode="CalendarDisplayMode.Year" DisplayModeView="CalendarDisplayModeView.List"></Calendar>
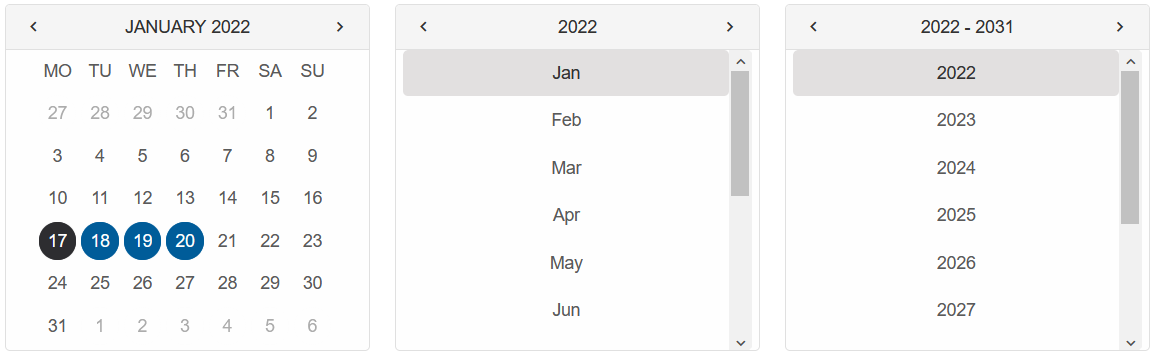
Important Dates
Smart.Calendar allows you to highlight important dates by applying custom templates to selected dates.
First, create an array of the important dates and set them as value of ImportantDates.
Then, create a template and set its id as value of ImportantDatesTemplate:
<template id="someTemplate"><div style="background-color:red">{{value}}</div></template> <Calendar SelectedDates="selectedDates" ImportantDates="importantDates" ImportantDatesTemplate="template"></Calendar> @code{ string[] selectedDates = new string[]{"2022-01-18", "2022-01-19", "2022-01-20"}; string template = "someTemplate"; string[] importantDates = new string[]{"2022-01-24", "2022-01-25"}; }
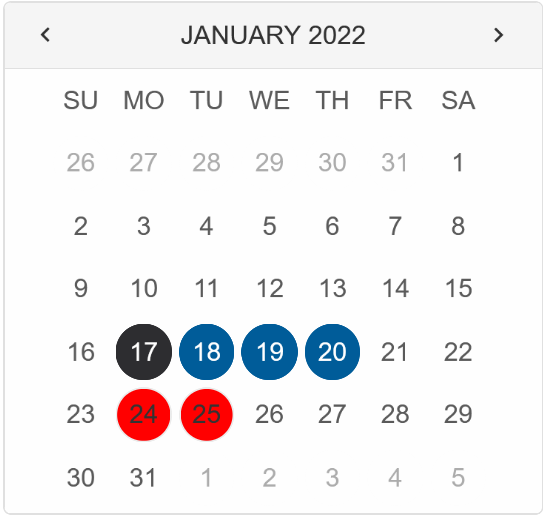
Restricted Dates
Restricted dates are dates that cannot be selected/hovered/focused. They are visualy styled as restricted
They are set as an array of dates to the RestrictedDates
property:
<Calendar SelectedDates="selectedDates" RestrictedDates="restrictedDates"></Calendar> @code{ string[] selectedDates = new string[]{"2022-01-18", "2022-01-19", "2022-01-20"}; string[] restrictedDates = new string[]{"2022-01-26", "2022-01-27", "2022-01-28"}; }
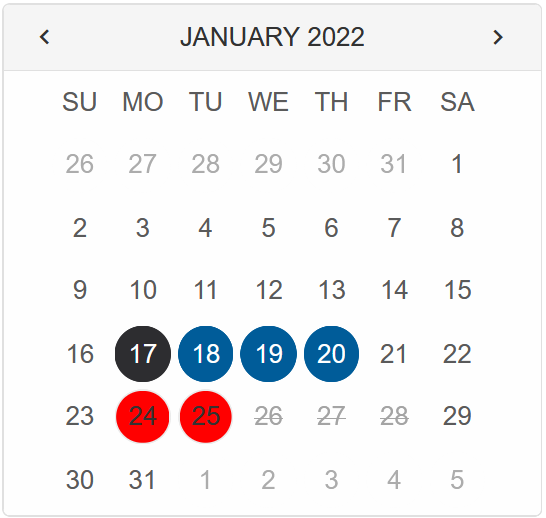
Date Formatting
It is possible to apply date formatting to the Calendar by using the
DayNameFormat
, MonthNameFormat
and YearFormat
properties:"
<Calendar MonthNameFormat="MonthFormat.Short" DayNameFormat="DayFormat.Narrow" ></Calendar>
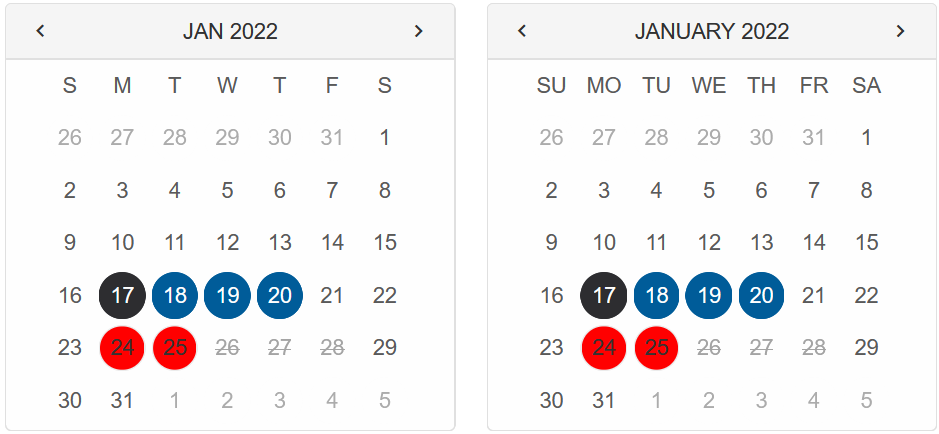
Events
Smart.Calendar provides built-in Events that can help you expand the component's
functionality.
The event object can have unique event.detail parameters.
OnChange
- triggered when a new date has been selected/unselected.
Event Details
: IEnumerable<string> valueOnDisplayModeChanging
- triggered when the displayMode is about to change.
Event Details
: dynamic oldDisplayMode, dynamic newDisplayModeOnDisplayModeChange
- triggered when the display mode has changed.
Event Details
: N/AOnNavigationChanging
- triggered when the view is changing.
Event Details
: DateTime value, string typeOnNavigationChange
- triggered when the view is changed.
Event Details
: DateTime value, string typeOnOpen
- triggered when the tooltip for the important date is opened.
Event Details
: dynamic target, dynamic valueOnClose
- triggered when the tooltip for the important date is closed.
Event Details
: dynamic target, dynamic value
<Calendar @ref="calendar" OnChange="OnChange"></Calendar> @code{ private Calendar calendar; string[] selectedDates = new string[]{"2022-01-18", "2022-01-19", "2022-01-20"}; private void OnChange(Event ev){ calendar.Navigate(1); } }
The demo below navigates to the next month when the user makes a selection:
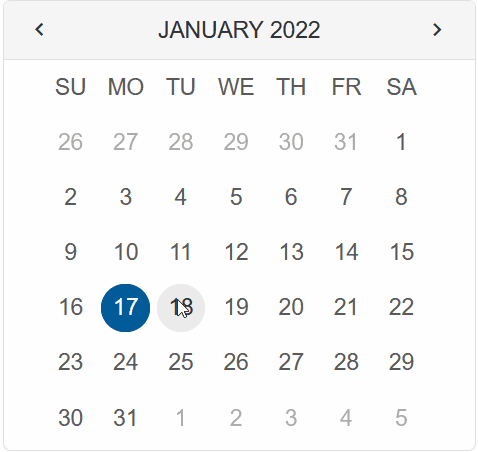