Build your web apps using Smart UI
Smart.Input - configuration and usage
Overview
Smart.Input element represents a simple Auto-Complete Input.
Getting Started with Input Web Component
Smart UI for Web Components is distributed as smart-webcomponents NPM package. You can also get the full download from our website with all demos from the Download page.Setup the Input
Smart UI for Web Components is distributed as smart-webcomponents NPM package
- Download and install the package.
npm install smart-webcomponents
- Once installed, import the Input module in your application.
<script type="module" src="node_modules/smart-webcomponents/source/modules/smart.input.js"></script>
-
Adding CSS reference
The smart.default.css CSS file should be referenced using following code.
<link rel="stylesheet" type="text/css" href="node_modules/smart-webcomponents/source/styles/smart.default.css" />
- Add the Input tag to your Web Page
<smart-input id="input"></smart-input>
- Create the Input Component
<script type="module"> Smart('#input', class { get properties() { return {value: "Peter Brown"} } }); </script>
Another option is to create the Input is by using the traditional Javascript way:
const input = document.createElement('smart-input'); input.disabled = true; document.body.appendChild(input);
Smart framework provides a way to dynamically create a web component on demand from a DIV tag which is used as a host. The following imports the web component's module and creates it on demand, when the document is ready. The #input is the ID of a DIV tag.
import "../../source/modules/smart.input.js"; document.readyState === 'complete' ? init() : window.onload = init; function init() { const input = new Smart.Input('#input', {value: "Peter Brown"}); }
- Open the page in your web server.
The following code adds the custom element to the page.
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> cript type="text/javascript" src="../../source/smart.element.js"></script> <script type="text/javascript" src="../../source/smart.input.js"></script> </head> <body> <smart-input></smart-input> </body> </html>
Note how smart.element.js is declared before everything else. This is mandatory for all custom elements.
Demo

Appearance
If the user wants to change the content of the text box, this can be accomplished by setting the value property of the element, like so:
<!DOCTYPE html> <html lang="en"> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> <script type="text/javascript" src="../../source/smart.element.js"></script> <script type="text/javascript" src="../../source/smart.input.js"></script> <script> window.onload = function () { document.querySelector('smart-input').value = 'input New Value'; } </script> </head> <body> <smart-input></smart-input> </body> </html>
Here's how to initialize a simple Smart.Input element:
<smart-input placeholder="Empty"></smart-input>
Demo

DataSource
To enable the options list popup the user has to define an Array of objects with label and value attributes and pass it to the dataSource property of the element:
const input = document.querySelector('smart-input'); input.dropDownButtonPosition = 'right'; input.dataSource = [ { label: "Andrew Watson" }, { label: "Anne Kean" }, { label: "Avril Janin" } ];
Demo
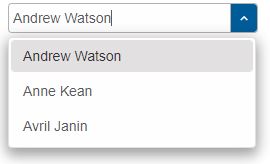
The dropDownButtonPosition Boolean property determines the position of the drop down button.
By default the drop down button is not visible. If no dataSource is provided, clicking on the drop down button will not open the popup.
MultiInput
Smart.MultiInput is a variation of the Input element that allows multiple items to be selected from the drop down. The selected items will be added to the input devided by a separator.
To initialize a Smart.MultiInput element the user has to include the following files to the head of the page:
- smart.default.css - the CSS file containing the styles for the elements.
- smart.element.js - the base element.
- smart.button.js - the Smart Button file definitions needed for the ScrollBars.
- smart.scrollbar.js - this file contains definitions for the Scrollbars inside the input popup.
- smart.input.js - the JS file containing the definitions for the Input element.
- smart.multiinput.js - the JS file containing the definitions for the MultiInput element.
Here's how to initialize a Smart.MultiInput element with dataSource and dropDownButtonPosition:
<smart-multi-input drop-down-button-position="right" placeholder="Please Select" ></smart-multi-input>
window.onload = function() { const multiInput = document.querySelector('smart-multi-input'); multiInput.dataSource = [ { value: "Austria", label: "Austria" }, { value: "Belarus", label: "Belarus" }, { value: "Belgium", label: "Belgium" }, { value: "Bosnia and Herzegovina", label: "Bosnia and Herzegovina" }, { value: "Bulgaria", label: "Bulgaria" }, { value: "Croatia", label: "Croatia" }, ... ]; }
Demo
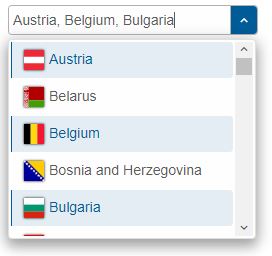
The following demo shows a country list with national flags with three selected items. As a result the selected labels are displayed in the input, separated with a coma. The user can type in a country and the popup will show a match if possible.
Smart.MultiInput is very similar to the Smart.Input but allows multiple item selection.
CheckInput
Smart.CheckInput is a variation of the MultiInput element that allows multiple items to be selected from the drop down via checkboxes. The selected items will be added to the input devided by a separator.
To initialize a Smart.CheckInput element the user has to include the following files to the head of the page:
- smart.default.css - the CSS file containing the styles for the elements.
- smart.element.js - the base element.
- smart.button.js - the Smart Button file definitions needed for the ScrollBars.
- smart.scrollbar.js - this file contains definitions for the Scrollbars inside the input popup.
- smart.input.js - the JS file containing the definitions for the Input element.
- smart.multiinput.js - the JS file containing the definitions for the MultiInput element.
- smart.checkinput.js - the JS file containing the definitions for the CheckInput element.
Here's how to initialize a Smart.CheckInput element with dataSource and dropDownButtonPosition:
<smart-check-input drop-down-button-position="right" placeholder="Please Select" ></smart-check-input>
window.onload = function() { const checkInput = document.querySelector('smart-check-input'); checkInput.dataSource = [ { value: "Austria", label: "Austria" }, { value: "Belarus", label: "Belarus" }, { value: "Belgium", label: "Belgium" }, { value: "Bosnia and Herzegovina", label: "Bosnia and Herzegovina" }, { value: "Bulgaria", label: "Bulgaria" }, { value: "Croatia", label: "Croatia" }, ... ]; }
Demo
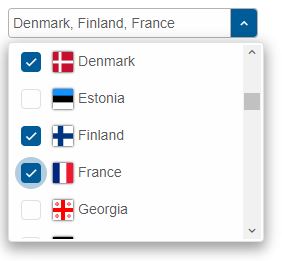
Checkboxes can be selected using the mouse or the Enter key when an item is focused via the keyboard.
Inverted
An additional inverted Boolean property is available which allows to position the checkboxes on the right side instead of the left.
checkInput.inverted = true;
Demo
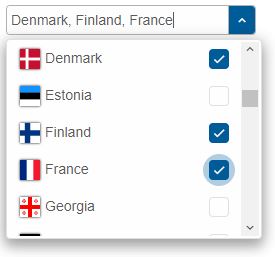
Smart.CheckInput is similar to the Smart.MultiInput but contains checkboxes.
MultiComboInput
Smart.MultiComboInput is a combination between the CheckInput and MultiInput elements which allows to select multiple items from the drop down via checkboxes. The selected items will be added to the input section ( before the input ) as tags.
To initialize a Smart.MultiComboInput element the user has to include the following files to the head of the page:
- smart.default.css - the CSS file containing the styles for the elements.
- smart.element.js - the base element.
- smart.button.js - the Smart Button file definitions needed for the ScrollBars.
- smart.scrollbar.js - this file contains definitions for the Scrollbars inside the input popup.
- smart.input.js - the JS file containing the definitions for the Input element.
- smart.multiinput.js - the JS file containing the definitions for the MultiInput element.
- smart.checkinput.js - the JS file containing the definitions for the CheckInput element.
- smart.multicomboinput.js - the JS file containing the definitions for the MultiComboInput element.
Here's how to initialize a Smart.MultiComboInput element with a list of countries with flags as a dataSource:
<smart-multi-combo-input></smart-multi-combo-input>
window.onload = function() { const multiComboInput = document.querySelector('smart-multi-combo-input'); multiComboInput.dataSource = [ { value: "Austria", label: "Austria" }, { value: "Belarus", label: "Belarus" }, { value: "Belgium", label: "Belgium" }, { value: "Bosnia and Herzegovina", label: "Bosnia and Herzegovina" }, { value: "Bulgaria", label: "Bulgaria" }, { value: "Croatia", label: "Croatia" }, ... ]; }
Demo
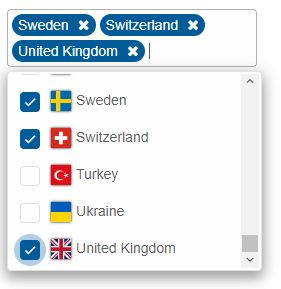
SelectAll
An additional selectAll property is available in this element that shows a Select All button at the top of the popup, allowing to select/unselect all items.
multiComboInput.selectAll = true;
Demo
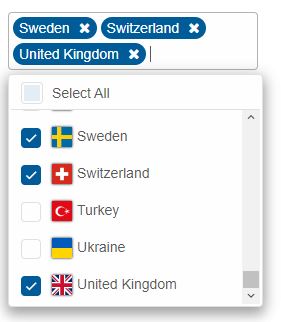
Smart.MutliComboInput is similar to the Smart.CheckInput but creates tags for each selected item in the input section instead of adding them to the input.
DateRangeInput
Smart.DateRangeInput is an Input variation that contains a Smart.Calendar in a drop down that is hidden by default. It accepts valid JS Date format strings and allows the user to pick a date from the popup.
A single click on a date marks the beginning of a date range selection. The second click on a date marks the end of the range. Every other selected date after that is treated as a new range selection.
The selected dates in the Calendar are added to the Input as strings according to the locale of the element and the dateFormat and timeFormat properties.
To initialize a Smart.DateRangeInput element the user has to include the following files to the head of the page:
- smart.default.css - the CSS file containing the styles for the elements.
- smart.element.js - the base element.
- smart.button.js - the Smart Button file definitions needed for the ScrollBars.
- smart.scrollbar.js - this file contains definitions for the Scrollbars inside the input popup.
- smart.listbox.js - the Smart Button file definitions needed for the ListBox used by the Calendar.
- smart.dropdownlist.js - the Smart Button file definitions needed for the DropDownList used by the Calendar.
- smart.tooltip.js - the Smart Button file definitions needed for the Tooltip used by the Calendar.
- smart.calendar.js - the Smart Button file definitions needed for the ScrollBars.
- smart.input.js - the JS file containing the definitions for the Input element.
- smart.daterangeinput.js - the JS file containing the definitions for the DateRangeInput element.
Here's how to initialize a Smart.DateRangeInput element with a dropDownButtonPosition property:
<smart-date-range-input placeholder="Please Select" drop-down-button-position="right"></smart-date-range-input>
Demo
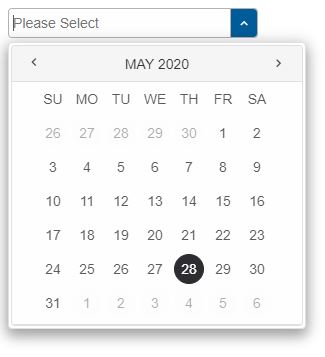
The user can open the popup and select a date range from the Calendar or set the value property to a valid JS Date, for exampe:
window.onload = function() { const dateRangeInput = document.querySelector('smart-date-range-input'); dateRangeInput.value = '5/5/2020 - 5/14/2020'; }
Demo
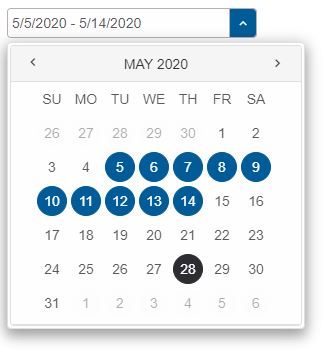
The two dates in the date range are separated by a dash surrounded by a whitespace. This can be configured via the separator property.
Timepicker
Time selection is also available in the Smart.DateRangeInput. It can be enabled via the timepicker Boolean property. Once applied the input accepts time values along with the dates and a Timepicker panel is available inside the popup when a date is selected and the user clicks on the time indicator at the bottom.
dateRangeInput.timepicker = true;
Demo
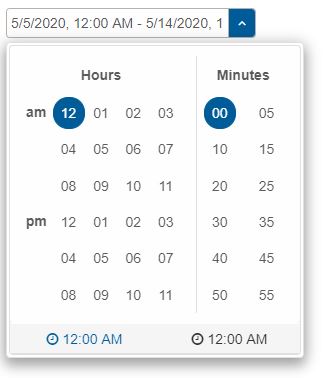
If a date range is selected the time indicators in the popup will be two, one for the start time and another for the end time.
Smart.DateRangeInput is an Input that offers Date selection and acts a lightweight DateTimePicker.
Icons
Another feature of the Smart.DateRangeInput is the icons Boolean property which enables/disables the icon buttons located next to the time indicators inside the drop down. These buttons allow to select the today date or clear the selection.
dateRangeInput.icons = true;
Demo
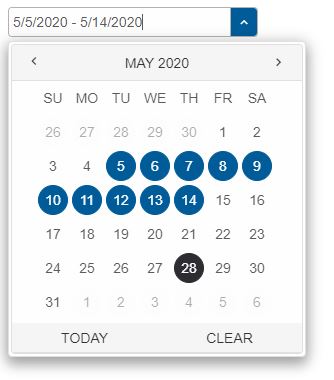
Since the Smart.DateRangeInput is an Input it returns it's value as a string. However it can be configured to return an Object with two attributes - from and to, which hold the start and end date if selected. This can be configured via the valueFormat property.
Readonly
By default the Input components act as ComboBoxes which means that they allow to enter and modify the text value and open the drop down at any time. Entering a value in the input (applies to all Inputs except the DateRangeInput) will trigger the search alghoritm that will open the popup to display matching items, if any. Setting the readonly property to the element will only display text in the input and the element will behave as a DropDownList:
input.readonly = true;
Demo
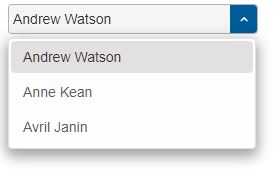
Events
Since they are Inputs they fire a change event with details regarding the old and new value:
input.addEventListener('change', function(event) { console.log('Input Event details: ' + event.detail); });
The event.detail object contains the new and old label and value of the element.
Create, Append, Remove, Get/Set Property, Invoke Method, Bind to Event
Create a new element:
const input = document.createElement('smart-input');
Append it to the DOM:
document.body.appendChild(input);
Remove it from the DOM:
input.parentNode.removeChild(input);
Set a property:
input.propertyName = propertyValue;
Get a property value:
const propertyValue = input.propertyName;
Invoke a method:
input.methodName(argument1, argument2);
Add Event Listener:
const eventHandler = (event) => { // your code here. }; input.addEventListener(eventName, eventHandler);
Remove Event Listener:
input.removeEventListener(eventName, eventHandler, true);
Using with Typescript
Smart Web Components package includes TypeScript definitions which enables strongly-typed access to the Smart UI Components and their configuration.
Inside the download package, the typescript directory contains .d.ts file for each web component and a smart.elements.d.ts typescript definitions file for all web components. Copy the typescript definitions file to your project and in your TypeScript file add a reference to smart.elements.d.ts
Read more about using Smart UI with Typescript.Getting Started with Angular Input Component
Setup Angular Environment
Angular provides the easiest way to set angular CLI projects using Angular CLI tool.
Install the CLI application globally to your machine.
npm install -g @angular/cli
Create a new Application
ng new smart-angular-input
Navigate to the created project folder
cd smart-angular-input
Setup the Input
Smart UI for Angular is distributed as smart-webcomponents-angular NPM package
- Download and install the package.
npm install smart-webcomponents-angular
- Adding CSS reference
The following CSS file is available in ../node_modules/smart-webcomponents-angular/ package folder. This can be referenced in [src/styles.css] using following code.@import 'smart-webcomponents-angular/source/styles/smart.default.css';
Another way to achieve the same is to edit the angular.json file and in the styles add the style."styles": [ "node_modules/smart-webcomponents-angular/source/styles/smart.default.css" ]
If you want to use Bootstrap, Fluent or other themes available in the package, you need to add them after 'smart.default.css'. -
Example with Angular Standalone Components
app.component.html
<div class="demo-description"> <p> <b>Smart.Input</b> is a simple input that can have a predefined options list.</p> <p><b>RenderMode</b> radio buttons allow to change the appearance of the input.</p> </div> <smart-input #input [placeholder]='"Empty"'></smart-input> <div class="options"> <div class="option"> <div class="description">Render Mode</div> <smart-radio-button #radiobutton [checked]="true" class="render-mode">Default</smart-radio-button> <smart-radio-button #radiobutton2 class="render-mode">Outlined</smart-radio-button> <smart-radio-button #radiobutton3 class="render-mode">Underlined</smart-radio-button> </div> </div>
app.component.ts
import { Component, ViewChild, OnInit, AfterViewInit } from '@angular/core'; import { InputComponent } from 'smart-webcomponents-angular/input'; import { RadioButtonComponent } from 'smart-webcomponents-angular/radiobutton'; import { CommonModule } from '@angular/common'; import { RouterOutlet } from '@angular/router'; import { InputModule } from 'smart-webcomponents-angular/input'; @Component({ selector: 'app-root', standalone: true, imports: [CommonModule, InputModule, RouterOutlet], templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements AfterViewInit, OnInit { @ViewChild('input', { read: InputComponent, static: false }) input!: InputComponent; @ViewChild('radiobutton', { read: RadioButtonComponent, static: false }) radiobutton!: RadioButtonComponent; @ViewChild('radiobutton2', { read: RadioButtonComponent, static: false }) radiobutton2!: RadioButtonComponent; @ViewChild('radiobutton3', { read: RadioButtonComponent, static: false }) radiobutton3!: RadioButtonComponent; ngOnInit(): void { // onInit code. } ngAfterViewInit(): void { // afterViewInit code. this.init(); } init(): void { // init code const input = this.input; document.querySelector('.options') ?.addEventListener('change', function (event) { const target = event.target as HTMLElement, inputClassList = input.nativeElement.classList; if (target.classList.contains('render-mode')) { inputClassList.remove('underlined', 'outlined'); const textContent = target.textContent?.toLowerCase() || ''; if (textContent.indexOf('underlined') > -1) { inputClassList.add('underlined'); } else if (textContent.indexOf('outlined') > -1) { inputClassList.add('outlined'); } } }); } }
-
Example with Angular NGModule
app.component.html
<div class="demo-description"> <p> <b>Smart.Input</b> is a simple input that can have a predefined options list.</p> <p><b>RenderMode</b> radio buttons allow to change the appearance of the input.</p> </div> <smart-input #input [placeholder]='"Empty"'></smart-input> <div class="options"> <div class="option"> <div class="description">Render Mode</div> <smart-radio-button #radiobutton [checked]="true" class="render-mode">Default</smart-radio-button> <smart-radio-button #radiobutton2 class="render-mode">Outlined</smart-radio-button> <smart-radio-button #radiobutton3 class="render-mode">Underlined</smart-radio-button> </div> </div>
app.component.ts
import { Component, ViewChild, OnInit, AfterViewInit } from '@angular/core'; import { InputComponent } from 'smart-webcomponents-angular/input'; import { RadioButtonComponent } from 'smart-webcomponents-angular/radiobutton'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements AfterViewInit, OnInit { @ViewChild('input', { read: InputComponent, static: false }) input!: InputComponent; @ViewChild('radiobutton', { read: RadioButtonComponent, static: false }) radiobutton!: RadioButtonComponent; @ViewChild('radiobutton2', { read: RadioButtonComponent, static: false }) radiobutton2!: RadioButtonComponent; @ViewChild('radiobutton3', { read: RadioButtonComponent, static: false }) radiobutton3!: RadioButtonComponent; ngOnInit(): void { // onInit code. } ngAfterViewInit(): void { // afterViewInit code. this.init(); } init(): void { // init code const input = this.input; document.querySelector('.options') ?.addEventListener('change', function (event) { const target = event.target as HTMLElement, inputClassList = input.nativeElement.classList; if (target.classList.contains('render-mode')) { inputClassList.remove('underlined', 'outlined'); const textContent = target.textContent?.toLowerCase() || ''; if (textContent.indexOf('underlined') > -1) { inputClassList.add('underlined'); } else if (textContent.indexOf('outlined') > -1) { inputClassList.add('outlined'); } } }); } }
app.module.ts
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { InputModule } from 'smart-webcomponents-angular/input'; import { RadioButtonModule } from 'smart-webcomponents-angular/radiobutton'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, InputModule, RadioButtonModule ], bootstrap: [ AppComponent ] }) export class AppModule { }
Running the Angular application
After completing the steps required to render a Input, run the following command to display the output in your web browser
ng serveand open localhost:4200 in your favorite web browser.
Read more about using Smart UI for Angular: https://www.htmlelements.com/docs/angular-cli/.
Getting Started with React Input Component
Setup React Environment
The easiest way to start with React is to use NextJS Next.js is a full-stack React framework. It’s versatile and lets you create React apps of any size—from a mostly static blog to a complex dynamic application.
npx create-next-app my-app cd my-app npm run devor
yarn create next-app my-app cd my-app yarn run dev
Preparation
Setup the Input
Smart UI for React is distributed as smart-webcomponents-react package
- Download and install the package.
In your React Next.js project, run one of the following commands to install Smart UI Input for ReactWith NPM:
npm install smart-webcomponents-react
With Yarn:yarn add smart-webcomponents-react
- Once installed, import the React Input Component and CSS files in your application and render it.
app.js
import 'smart-webcomponents-react/source/styles/smart.default.css'; import React, { useRef } from "react"; import ReactDOM from 'react-dom/client'; import { Input } from 'smart-webcomponents-react/input'; import { RadioButton } from 'smart-webcomponents-react/radiobutton'; const App = () => { const inputRef = useRef(null); const handleDefault = (event) => { if (!event.detail.value) { return; } inputRef.current.nativeElement.classList.remove('underlined'); inputRef.current.nativeElement.classList.remove('outlined'); } const handleOutlined = (event) => { if (!event.detail.value) { return; } inputRef.current.nativeElement.classList.remove('underlined'); inputRef.current.nativeElement.classList.add('outlined'); } const handleUnderlined = (event) => { if (!event.detail.value) { return; } inputRef.current.nativeElement.classList.add('underlined'); inputRef.current.nativeElement.classList.remove('outlined'); } return ( <div> <div className="demo-description"> <p> <b>Smart.Input</b> is a simple input that can have a predefined options list.</p> <p><b>RenderMode</b> radio buttons allow to change the appearance of the input.</p> </div> <Input ref={inputRef} placeholder="Empty"></Input> <div className="options"> <div className="option"> <div className="description">Render Mode</div> <RadioButton onChange={handleDefault} checked>Default</RadioButton> <RadioButton onChange={handleOutlined}>Outlined</RadioButton> <RadioButton onChange={handleUnderlined}>Underlined</RadioButton> </div> </div> </div> ); } export default App;
Running the React application
Start the app withnpm run devor
yarn run devand open localhost:3000 in your favorite web browser to see the output.
Setup with Vite
Vite (French word for "quick", pronounced /vit/, like "veet") is a build tool that aims to provide a faster and leaner development experience for modern web projectsWith NPM:
npm create vite@latestWith Yarn:
yarn create viteThen follow the prompts and choose React as a project.
Navigate to your project's directory. By default it is 'vite-project' and install Smart UI for React
In your Vite project, run one of the following commands to install Smart UI Input for ReactWith NPM:
npm install smart-webcomponents-reactWith Yarn:
yarn add smart-webcomponents-reactOpen src/App.tsx App.tsx
import 'smart-webcomponents-react/source/styles/smart.default.css'; import React, { useRef } from "react"; import ReactDOM from 'react-dom/client'; import { Input } from 'smart-webcomponents-react/input'; import { RadioButton } from 'smart-webcomponents-react/radiobutton'; const App = () => { const inputRef = useRef(null); const handleDefault = (event) => { if (!event.detail.value) { return; } inputRef.current.nativeElement.classList.remove('underlined'); inputRef.current.nativeElement.classList.remove('outlined'); } const handleOutlined = (event) => { if (!event.detail.value) { return; } inputRef.current.nativeElement.classList.remove('underlined'); inputRef.current.nativeElement.classList.add('outlined'); } const handleUnderlined = (event) => { if (!event.detail.value) { return; } inputRef.current.nativeElement.classList.add('underlined'); inputRef.current.nativeElement.classList.remove('outlined'); } return ( <div> <div className="demo-description"> <p> <b>Smart.Input</b> is a simple input that can have a predefined options list.</p> <p><b>RenderMode</b> radio buttons allow to change the appearance of the input.</p> </div> <Input ref={inputRef} placeholder="Empty"></Input> <div className="options"> <div className="option"> <div className="description">Render Mode</div> <RadioButton onChange={handleDefault} checked>Default</RadioButton> <RadioButton onChange={handleOutlined}>Outlined</RadioButton> <RadioButton onChange={handleUnderlined}>Underlined</RadioButton> </div> </div> </div> ); } export default App;
Read more about using Smart UI for React: https://www.htmlelements.com/docs/react/.
Getting Started with Vue Input Component
Setup Vue with Vite
In this section we will introduce how to scaffold a Vue Single Page Application on your local machine. The created project will be using a build setup based on Vite and allow us to use Vue Single-File Components (SFCs). Run the following command in your command linenpm create vue@latestThis command will install and execute create-vue, the official Vue project scaffolding tool. You will be presented with prompts for several optional features such as TypeScript and testing support:
✔ Project name: …If you are unsure about an option, simply choose No by hitting enter for now. Once the project is created, follow the instructions to install dependencies and start the dev server:✔ Add TypeScript? … No / Yes ✔ Add JSX Support? … No / Yes ✔ Add Vue Router for Single Page Application development? … No / Yes ✔ Add Pinia for state management? … No / Yes ✔ Add Vitest for Unit testing? … No / Yes ✔ Add an End-to-End Testing Solution? … No / Cypress / Playwright ✔ Add ESLint for code quality? … No / Yes ✔ Add Prettier for code formatting? … No / Yes Scaffolding project in ./ ... Done.
cdnpm install npm install smart-webcomponents npm run dev
-
Make Vue ignore custom elements defined outside of Vue (e.g., using the Web Components APIs). Otherwise, it will throw a warning about an Unknown custom element, assuming that you forgot to register a global component or misspelled a component name.
Open vite.config.js in your favorite text editor and change its contents to the following:
vite.config.js
import { fileURLToPath, URL } from 'node:url' import { defineConfig } from 'vite' import vue from '@vitejs/plugin-vue' // https://vitejs.dev/config/ export default defineConfig({ plugins: [ vue({ template: { compilerOptions: { isCustomElement: tag => tag.startsWith('smart-') } } }) ], resolve: { alias: { '@': fileURLToPath(new URL('./src', import.meta.url)) } } })
-
Open src/App.vue in your favorite text editor and change its contents to the following:
App.vue
<template> <div class="vue-root"> <div class="demo-description"> <p> <b>Smart.Input</b> is a simple input that can have a predefined options list. </p> <p> <b>RenderMode</b> radio buttons allow to change the appearance of the input. </p> </div> <smart-input placeholder="Empty"></smart-input> <div class="options"> <div class="option"> <div class="description">Render Mode</div> <smart-radio-button checked class="render-mode">Default</smart-radio-button> <smart-radio-button class="render-mode">Outlined</smart-radio-button> <smart-radio-button class="render-mode">Underlined</smart-radio-button> </div> </div> </div> </template> <script> import { onMounted } from "vue"; import "smart-webcomponents/source/styles/smart.default.css"; import "smart-webcomponents/source/modules/smart.input.js"; import "smart-webcomponents/source/modules/smart.radiobutton.js"; export default { name: "app", setup() { onMounted(() => { let input = document.querySelector("smart-input"); document .querySelector(".options") .addEventListener("change", function(event) { const target = event.target; if (target.classList.contains("render-mode")) { input.classList.remove("underlined", "outlined"); const textContent = target.textContent.toLowerCase(); if (textContent.indexOf("underlined") > -1) { input.classList.add("underlined"); } else if (textContent.indexOf("outlined") > -1) { input.classList.add("outlined"); } } }); }); } }; </script> <style> </style>
We can now use the smart-input with Vue 3. Data binding and event handlers will just work right out of the box.
Running the Vue application
Start the app withnpm run devand open http://localhost:5173/ in your favorite web browser to see the output below:
When you are ready to ship your app to production, run the following:
npm run buildThis will create a production-ready build of your app in the project's ./dist directory.
Read more about using Smart UI for Vue: https://www.htmlelements.com/docs/vue/.