Chart Types
Build your web apps using Smart UI
Smart.Chart - configuration and usage
Chart Types
Smart.Chart supports over 30 common and specialized chart types. You can easily plot series of different types within the same chart. A type must be specified for each series group. Currently, Smart.Chart supports the following series types:
- 'column' - simple column series
- 'stackedcolumn' - stacked column series
- 'stackedcolumn100' - percentage stacked columns
- 'rangecolumn' - floating column between two values
- 'waterfall' - waterfall series
- 'stackedwaterfall' - stacked waterfall series
- 'line' - simple straight lines connecting the value points
- 'stackedline' - stacked lines
- 'stackedline100' - percentage stacked lines
- 'spline' - smooth lines connecting the value points
- 'stackedspline' - smooth stacked lines
- 'stackedspline100' - percentage stacked smooth lines
- 'stepline' - step line
- 'stackedstepline' - stacked step line
- 'stackedstepline100' - percentage stacked step line
- 'area' - area connecting the value points with straight lines
- 'stackedarea' - stacked area with straight lines between the points
- 'stackedarea100' - percentage stacked area with straight lines between the points
- 'rangearea' - floating area between pairs of value points
- 'splinearea' - smooth area connecting the value points
- 'stackedsplinearea' - stacked smooth area connecting the value points
- 'stackedsplinearea100' - percentage stacked smooth area
- 'splinerangearea' - smooth floating area between pairs of value points
- 'steprangearea' - step area between pairs of value points
- 'stackedsplineara' - smooth stacked area
- 'steparea' - step area connecting the value points
- 'stackedsteparea' - step stacked area
- 'stackedsteparea100' - percentage stacked step area
- 'pie' - circular chart divided into sectors, illustrating proportion
- 'donut' - chart divided into circular sectors with different inner and outer radius
- 'scatter' - data is displayed as a collection of points
- 'stackedscatter' - data is displayed as a collection of points and the values are stacked
- 'stackedscatter100' - data is displayed as a collection of points and the values are percentage stacked
- 'bubble' - data is displayed as a collection of bubbles
- 'stackedbubble' - data is displayed as a collection of bubbles and the values are stacked
- 'stackedbubble100' - data is displayed as a collection of bubbles and the values are percentage stacked
- 'candlestick' - display candlestick series using open, high, low, close data points
- 'ohlc' - display OHLC series using open, high, low, close data points
Different chart types can be used to visualize the same data. The following code defines a single series group with three series which will be rendered as lines:
seriesGroups: [ { type: 'line', series: [ { dataField: 'Keith', displayText: 'Keith' }, { dataField: 'Erica', displayText: 'Erica' }, { dataField: 'George', displayText: 'George' } ] } ]
The following full example:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Chart Line Series Example</title> <link rel="stylesheet" type="text/css" href="../../source/styles/smart.default.css" /> <script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/webcomponentsjs/2.2.7/webcomponents-bundle.js"></script> <script type="text/javascript" src="../../source/smart.elements.js"></script> <script type="text/javascript"> const sampleData = [ { Day: 'Monday', Keith: 30, Erica: 15, George: 25 }, { Day: 'Tuesday', Keith: 25, Erica: 25, George: 30 }, { Day: 'Wednesday', Keith: 30, Erica: 20, George: 25 }, { Day: 'Thursday', Keith: 35, Erica: 25, George: 45 }, { Day: 'Friday', Keith: 20, Erica: 20, George: 25 }, { Day: 'Saturday', Keith: 30, Erica: 20, George: 30 }, { Day: 'Sunday', Keith: 60, Erica: 45, George: 90 } ]; Smart('#chart', class { get properties() { return { caption: 'Fitness & exercise weekly scorecard', description: 'Time spent in vigorous exercise', dataSource: sampleData, colorScheme: 'scheme29', clip: false, xAxis: { dataField: 'Day', gridLines: { visible: true } }, valueAxis: { description: 'Time in minutes', axisSize: 'auto', minValue: 0, maxValue: 100, unitInterval: 10 }, seriesGroups: [ { type: 'line', orientation: 'vertical', columnsGapPercent: 50, seriesGapPercent: 0, series: [ { dataField: 'Keith', displayText: 'Keith' }, { dataField: 'Erica', displayText: 'Erica' }, { dataField: 'George', displayText: 'George' } ] } ] }; } }); </script> </head> <body> <smart-chart id="chart"></smart-chart> </body> </html>
results in the Chart shown in the image below:
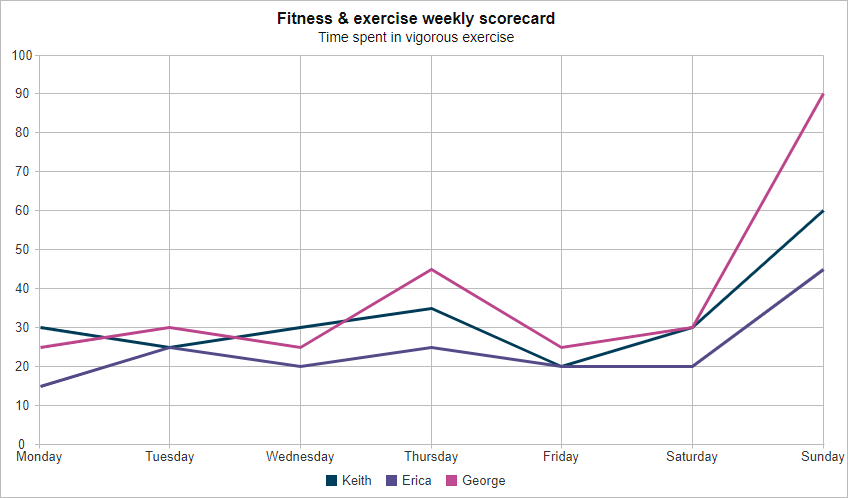
By just changing the type of the series group, we can get the following visualizations:
![]() |
![]() |
![]() |
![]() |
Chart with Multiple Series Types
If you want to display multiple series with different types you can define several series groups and change the type property, as in the following code:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Chart Multiple Series Types Example</title> <link rel="stylesheet" type="text/css" href="../../source/styles/smart.default.css" /> <script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/webcomponentsjs/2.2.7/webcomponents-bundle.js"></script> <script type="text/javascript" src="../../source/smart.elements.js"></script> <script type="text/javascript"> const sampleData = [ { Day: 'Monday', Keith: 30, Erica: 15, George: 25, Monica: 20, Maria: 15, Average: 20 }, { Day: 'Tuesday', Keith: 25, Erica: 25, George: 30, Monica: 25, Maria: 20, Average: 38 }, { Day: 'Wednesday', Keith: 30, Erica: 20, George: 25, Monica: 10, Maria: 20, Average: 33 }, { Day: 'Thursday', Keith: 35, Erica: 25, George: 45, Monica: 30, Maria: 30, Average: 31 }, { Day: 'Friday', Keith: 20, Erica: 20, George: 25, Monica: 45, Maria: 30, Average: 52 }, { Day: 'Saturday', Keith: 30, Erica: 20, George: 30, Monica: 60, Maria: 40, Average: 75 }, { Day: 'Sunday', Keith: 60, Erica: 45, George: 90, Monica: 70, Maria: 50, Average: 73 } ]; Smart('#chart', class { get properties() { return { caption: 'Fitness & exercise weekly scorecard', description: 'Time spent in vigorous exercise', showLegend: true, padding: { left: 5, top: 5, right: 5, bottom: 5 }, titlePadding: { left: 90, top: 0, right: 0, bottom: 10 }, dataSource: sampleData, xAxis: { dataField: 'Day', gridLines: { visible: true } }, valueAxis: { unitInterval: 10, minValue: 0, maxValue: 100, displayValueAxis: true, description: 'Time in minutes', axisSize: 'auto', tickMarksColor: '#888888' }, seriesGroups: [ { type: 'column', columnsGapPercent: 50, seriesGapPercent: 0, colorScheme: 'scheme29', series: [ { dataField: 'Keith', displayText: 'Keith' }, { dataField: 'Erica', displayText: 'Erica' }, { dataField: 'George', displayText: 'George' }, { dataField: 'Monica', displayText: 'Monica' }, { dataField: 'Maria', displayText: 'Maria' } ] }, { type: 'spline', colorScheme: 'scheme05', series: [ { dataField: 'Average', displayText: 'Class average', lineWidth: 5 } ] } ] } } }); </script> </head> <body> <smart-chart id="chart"></smart-chart> </body> </html>
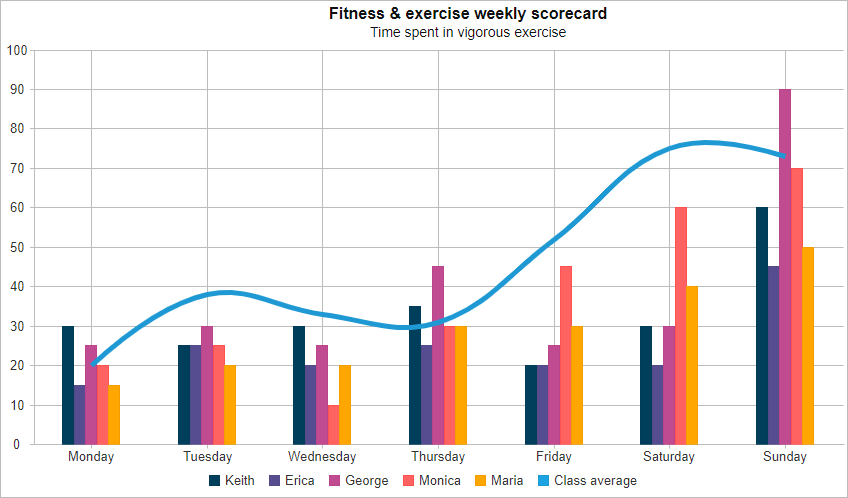
Polar Chart
Most of the aforementioned types of Chart can be rendered as a polar (or spider) chart - a graphical method of displaying multivariate data in the form of a two-dimensional chart of three or more quantitative variables.
In Smart.Chart, this functionality is enabled by setting
polar: trueto a series groups. A full example is given below:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>Polar Chart Example</title> <link rel="stylesheet" type="text/css" href="../../source/styles/smart.default.css" /> <style type="text/css"> #chart { width: 500px; } </style> <script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/webcomponentsjs/2.2.7/webcomponents-bundle.js"></script> <script type="text/javascript" src="../../source/smart.elements.js"></script> <script type="text/javascript"> const sampleData = [{ "Year": "1950", "HPI": "105.8948393", "BuildCost": "59.9171862", "Population": "151.684", "Rate": "0.0232", "uid": 0 }, { "Year": "1951", "HPI": "103.8986687", "BuildCost": "59.2786986", "Population": "154.287", "Rate": "0.0257", "uid": 1 }, { "Year": "1952", "HPI": "103.9743275", "BuildCost": "58.94343772", "Population": "156.954", "Rate": "0.0268", "uid": 2 }, { "Year": "1953", "HPI": "113.8451588", "BuildCost": "60.8392201", "Population": "159.565", "Rate": "0.0283", "uid": 3 }, { "Year": "1954", "HPI": "115.6204181", "BuildCost": "62.25447619", "Population": "162.391", "Rate": "0.0248", "uid": 4 }, { "Year": "1955", "HPI": "115.5419272", "BuildCost": "65.95528267", "Population": "165.275", "Rate": "0.0261", "uid": 5 }, { "Year": "1956", "HPI": "113.7942463", "BuildCost": "68.79148786", "Population": "168.221", "Rate": "0.029", "uid": 6 }, { "Year": "1957", "HPI": "113.6664365", "BuildCost": "69.24632104", "Population": "171.274", "Rate": "0.0346", "uid": 7 }, { "Year": "1958", "HPI": "111.7970971", "BuildCost": "68.9257142", "Population": "174.141", "Rate": "0.0309", "uid": 8 }, { "Year": "1959", "HPI": "110.4583238", "BuildCost": "70.95296772", "Population": "177.13", "Rate": "0.0402", "uid": 9 }, { "Year": "1960", "HPI": "109.6495745", "BuildCost": "71.63614258", "Population": "180.76", "Rate": "0.0472", "uid": 10 }, { "Year": "1961", "HPI": "109.5092421", "BuildCost": "71.568197", "Population": "183.742", "Rate": "0.0384", "uid": 11 }, { "Year": "1962", "HPI": "108.7289429", "BuildCost": "72.59299982", "Population": "186.59", "Rate": "0.0408", "uid": 12 }, { "Year": "1963", "HPI": "109.1195568", "BuildCost": "73.36701774", "Population": "189.3", "Rate": "0.0383", "uid": 13 }, { "Year": "1964", "HPI": "109.8522455", "BuildCost": "74.36711732", "Population": "191.927", "Rate": "0.0417", "uid": 14 }, { "Year": "1965", "HPI": "109.2636597", "BuildCost": "75.45724616", "Population": "194.347", "Rate": "0.0419", "uid": 15 }, { "Year": "1966", "HPI": "106.6787192", "BuildCost": "76.74926786", "Population": "196.599", "Rate": "0.0461", "uid": 16 }, { "Year": "1967", "HPI": "106.3897611", "BuildCost": "77.15051023", "Population": "198.752", "Rate": "0.0458", "uid": 17 }, { "Year": "1968", "HPI": "105.2152935", "BuildCost": "79.39056457", "Population": "200.745", "Rate": "0.0553", "uid": 18 }, { "Year": "1969", "HPI": "106.9530731", "BuildCost": "83.32303834", "Population": "202.736", "Rate": "0.0604", "uid": 19 }, { "Year": "1970", "HPI": "109.5578145", "BuildCost": "83.04289525", "Population": "205.089", "Rate": "0.0779", "uid": 20 }, { "Year": "1971", "HPI": "110.1900229", "BuildCost": "89.43618588", "Population": "207.692", "Rate": "0.0624", "uid": 21 }, { "Year": "1972", "HPI": "110.089853", "BuildCost": "95.74309566", "Population": "209.924", "Rate": "0.0595", "uid": 22 }, { "Year": "1973", "HPI": "105.0761478", "BuildCost": "100.3045578", "Population": "211.939", "Rate": "0.0646", "uid": 23 }, { "Year": "1974", "HPI": "102.5051836", "BuildCost": "97.09327155", "Population": "213.898", "Rate": "0.0699", "uid": 24 }, { "Year": "1975", "HPI": "103.454991", "BuildCost": "94.12250092", "Population": "215.981", "Rate": "0.075", "uid": 25 }, { "Year": "1976", "HPI": "105.5752263", "BuildCost": "96.23389798", "Population": "218.086", "Rate": "0.0774", "uid": 26 }, { "Year": "1977", "HPI": "113.3258904", "BuildCost": "99.16550373", "Population": "220.289", "Rate": "0.0721", "uid": 27 }, { "Year": "1978", "HPI": "118.3947307", "BuildCost": "100.56884", "Population": "222.629", "Rate": "0.0796", "uid": 28 }, { "Year": "1979", "HPI": "118.8156738", "BuildCost": "100", "Population": "225.106", "Rate": "0.091", "uid": 29 }, { "Year": "1980", "HPI": "112.3677501", "BuildCost": "93.67720901", "Population": "227.726", "Rate": "0.108", "uid": 30 }, { "Year": "1981", "HPI": "106.8724657", "BuildCost": "90.5038767", "Population": "230.008", "Rate": "0.1257", "uid": 31 }, { "Year": "1982", "HPI": "103.3129562", "BuildCost": "88.95277083", "Population": "232.218", "Rate": "0.1459", "uid": 32 }, { "Year": "1983", "HPI": "104.5574104", "BuildCost": "91.52830102", "Population": "234.333", "Rate": "0.1046", "uid": 33 }, { "Year": "1984", "HPI": "105.4357808", "BuildCost": "89.06159549", "Population": "236.394", "Rate": "0.1167", "uid": 34 }, { "Year": "1985", "HPI": "108.2776822", "BuildCost": "86.30725178", "Population": "238.506", "Rate": "0.1138", "uid": 35 }, { "Year": "1986", "HPI": "114.6429508", "BuildCost": "85.06563926", "Population": "240.683", "Rate": "0.0919", "uid": 36 }, { "Year": "1987", "HPI": "119.4622422", "BuildCost": "85.80011747", "Population": "242.843", "Rate": "0.0708", "uid": 37 }, { "Year": "1988", "HPI": "123.3192026", "BuildCost": "84.31285438", "Population": "245.061", "Rate": "0.0867", "uid": 38 }, { "Year": "1989", "HPI": "124.8941458", "BuildCost": "81.66945023", "Population": "247.387", "Rate": "0.0909", "uid": 39 }, { "Year": "1990", "HPI": "116.2873698", "BuildCost": "79.63498843", "Population": "250.181", "Rate": "0.0821", "uid": 40 }, { "Year": "1991", "HPI": "113.0775228", "BuildCost": "76.74207454", "Population": "253.53", "Rate": "0.0809", "uid": 41 }, { "Year": "1992", "HPI": "109.7008693", "BuildCost": "77.05381963", "Population": "256.922", "Rate": "0.0703", "uid": 42 }, { "Year": "1993", "HPI": "108.4357895", "BuildCost": "78.88788054", "Population": "260.282", "Rate": "0.066", "uid": 43 }, { "Year": "1994", "HPI": "108.4360571", "BuildCost": "79.89887109", "Population": "263.455", "Rate": "0.0575", "uid": 44 }, { "Year": "1995", "HPI": "107.6666232", "BuildCost": "77.7443098", "Population": "266.588", "Rate": "0.0778", "uid": 45 }, { "Year": "1996", "HPI": "106.7336444", "BuildCost": "77.89285948", "Population": "269.714", "Rate": "0.0565", "uid": 46 }, { "Year": "1997", "HPI": "109.216361", "BuildCost": "79.39146429", "Population": "272.958", "Rate": "0.0658", "uid": 47 }, { "Year": "1998", "HPI": "115.2452499", "BuildCost": "78.79060549", "Population": "276.154", "Rate": "0.0554", "uid": 48 }, { "Year": "1999", "HPI": "121.6232347", "BuildCost": "78.98128131", "Population": "279.328", "Rate": "0.0472", "uid": 49 }, { "Year": "2000", "HPI": "129.0640838", "BuildCost": "78.72200105", "Population": "282.158336", "Rate": "0.0666", "uid": 50 }, { "Year": "2001", "HPI": "136.1331884", "BuildCost": "76.6401607", "Population": "284.915024", "Rate": "0.0516", "uid": 51 }, { "Year": "2002", "HPI": "147.6076356", "BuildCost": "76.8135395", "Population": "287.501476", "Rate": "0.0504", "uid": 52 }, { "Year": "2003", "HPI": "160.0795509", "BuildCost": "76.31543516", "Population": "289.985771", "Rate": "0.0405", "uid": 53 }, { "Year": "2004", "HPI": "177.7766491", "BuildCost": "80.77302579", "Population": "292.805643", "Rate": "0.0415", "uid": 54 }, { "Year": "2005", "HPI": "195.3510726", "BuildCost": "82.79484772", "Population": "295.583436", "Rate": "0.0422", "uid": 55 }, { "Year": "2006", "HPI": "192.2875379", "BuildCost": "82.72701137", "Population": "298.44242", "Rate": "0.0442", "uid": 56 }, { "Year": "2007", "HPI": "170.090729", "BuildCost": "83.19660676", "Population": "301.279593", "Rate": "0.0476", "uid": 57 }, { "Year": "2008", "HPI": "133.9747086", "BuildCost": "83.4461592", "Population": "304.228257", "Rate": "0.0374", "uid": 58 }, { "Year": "2009", "HPI": "130.9370732", "BuildCost": "84.80835495", "Population": "307.212123", "Rate": "0.0252", "uid": 59 }, { "Year": "2010", "HPI": "124.585809", "BuildCost": "84.18072431", "Population": "310.232863", "Rate": "0.0373", "uid": 60 }, { "Year": "2011", "HPI": "120.083801", "BuildCost": "84.18072431", "Population": "312.8936", "Rate": "0.0339", "uid": 61 }, { "Year": "2012", "HPI": "123.9370732", "BuildCost": "84.80835495", "Population": "307.212123", "Rate": "0.0252", "uid": 62 }, { "Year": "2013", "HPI": "131.585809", "BuildCost": "84.18072431", "Population": "310.232863", "Rate": "0.0373", "uid": 63 }, { "Year": "2014", "HPI": "134.083801", "BuildCost": "84.18072431", "Population": "312.8936", "Rate": "0.0339", "uid": 64 }, { "Year": "2015", "HPI": "136.083801", "BuildCost": "84.18072431", "Population": "311.8936", "Rate": "0.0342", "uid": 65 }, { "Year": "2016", "HPI": "138.083801", "BuildCost": "84.18072431", "Population": "310.8936", "Rate": "0.0346", "uid": 66 }, { "Year": "2017", "HPI": "142.083801", "BuildCost": "84.18072431", "Population": "313.8936", "Rate": "0.0374", "uid": 67 }]; Smart('#chart', class { get properties() { return { caption: 'U.S. Real Home Price vs Building Cost Indeces (1950-2010)', description: 'Source: http://www.econ.yale.edu/~shiller/data.htm', showLegend: true, padding: { left: 5, top: 5, right: 5, bottom: 5 }, titlePadding: { left: 0, top: 0, right: 0, bottom: 5 }, dataSource: sampleData, xAxis: { dataField: 'Year', unitInterval: 10, maxValue: 2010, valuesOnTicks: true, labels: { autoRotate: true } }, colorScheme: 'scheme31', seriesGroups: [ { polar: true, radius: 120, type: 'splinearea', valueAxis: { labels: { formatSettings: { decimalPlaces: 0 }, autoRotate: true } }, series: [ { dataField: 'HPI', displayText: 'Real Home Price Index', opacity: 0.7, lineWidth: 1, radius: 2 }, { dataField: 'BuildCost', displayText: 'Building Cost Index', opacity: 0.7, lineWidth: 1, radius: 2 } ] } ] }; } }); </script> </head> <body> <smart-chart id="chart"></smart-chart> </body> </html>
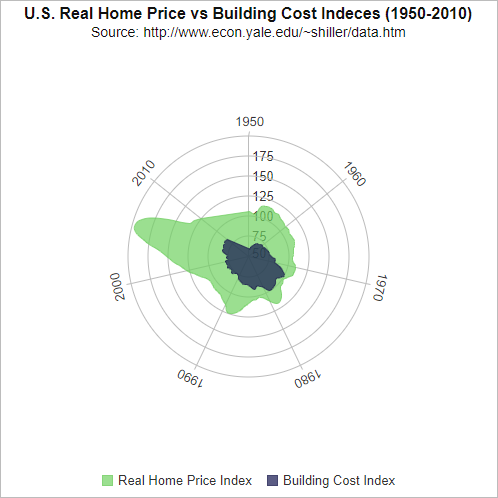
Financial Chart Types (OHLC and Candlestick)
Smart.Chart provides two chart types specifically suitable for displaying financial data - OHLC and candlestick. Both OHLC (open-high-low-close) and candlestick (also known as Japanese candlestick) charts are types of chart typically used to illustrate movements in the price of a financial instrument over time. Each vertical line on the chart shows the price range (the highest and lowest prices) over one unit of time, e.g., one day or one hour. Tick marks project from each side of the line indicating the opening price (e.g., for a daily bar chart this would be the starting price for that day) on the left, and the closing price for that time period on the right. The bars may be shown in different hues depending on whether prices rose or fell in that period.
The following is an example of an OHLC Smart.Chart:
<!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <title>OHLC Example</title> <link rel="stylesheet" type="text/css" href="../../source/styles/smart.default.css" /> <script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/webcomponentsjs/2.2.7/webcomponents-bundle.js"></script> <script type="text/javascript" src="../../source/smart.elements.js"></script> <script type="text/javascript"> const sampleData = [{ "Date": "11/21/2017", "SPOpen": "2057.46", "SPHigh": "2071.46", "SPLow": "2056.75", "SPClose": "2063.5", "SPVolume": "3916420000", "SPAdjClose": "2063.5", "NQOpen": "4751.01", "NQHigh": "4751.6", "NQLow": "4700.73", "NQClose": "4712.97", "NQVolume": "1854340000", "NQAdjClose": "4712.97\r", "uid": 0 }, { "Date": "11/20/2017", "SPOpen": "2045.87", "SPHigh": "2053.84", "SPLow": "2040.49", "SPClose": "2052.75", "SPVolume": "3128290000", "SPAdjClose": "2052.75", "NQOpen": "4655.2", "NQHigh": "4702.97", "NQLow": "4653.33", "NQClose": "4701.87", "NQVolume": "1667330000", "NQAdjClose": "4701.87\r", "uid": 1 }, { "Date": "11/19/2017", "SPOpen": "2051.16", "SPHigh": "2052.14", "SPLow": "2040.37", "SPClose": "2048.72", "SPVolume": "3390850000", "SPAdjClose": "2048.72", "NQOpen": "4694.78", "NQHigh": "4696.2", "NQLow": "4655.72", "NQClose": "4675.71", "NQVolume": "1641560000", "NQAdjClose": "4675.71\r", "uid": 2 }, { "Date": "11/18/2017", "SPOpen": "2041.48", "SPHigh": "2056.08", "SPLow": "2041.48", "SPClose": "2051.8", "SPVolume": "3416190000", "SPAdjClose": "2051.8", "NQOpen": "4674.84", "NQHigh": "4709.83", "NQLow": "4674.3", "NQClose": "4702.44", "NQVolume": "1655760000", "NQAdjClose": "4702.44\r", "uid": 3 }, { "Date": "11/17/2017", "SPOpen": "2038.29", "SPHigh": "2043.07", "SPLow": "2034.46", "SPClose": "2041.32", "SPVolume": "3152890000", "SPAdjClose": "2041.32", "NQOpen": "4678.44", "NQHigh": "4689.53", "NQLow": "4655.2", "NQClose": "4671", "NQVolume": "1695070000", "NQAdjClose": "4671\r", "uid": 4 }, { "Date": "11/14/2017", "SPOpen": "2039.74", "SPHigh": "2042.22", "SPLow": "2035.2", "SPClose": "2039.82", "SPVolume": "3227130000", "SPAdjClose": "2039.82", "NQOpen": "4679.85", "NQHigh": "4688.74", "NQLow": "4664.31", "NQClose": "4688.54", "NQVolume": "1739950000", "NQAdjClose": "4688.54\r", "uid": 5 }, { "Date": "11/13/2017", "SPOpen": "2039.21", "SPHigh": "2046.18", "SPLow": "2030.44", "SPClose": "2039.33", "SPVolume": "3455270000", "SPAdjClose": "2039.33", "NQOpen": "4681.56", "NQHigh": "4703.1", "NQLow": "4664.27", "NQClose": "4680.14", "NQVolume": "1857580000", "NQAdjClose": "4680.14\r", "uid": 6 }, { "Date": "11/12/2017", "SPOpen": "2037.75", "SPHigh": "2040.33", "SPLow": "2031.95", "SPClose": "2038.25", "SPVolume": "3246650000", "SPAdjClose": "2038.25", "NQOpen": "4644.63", "NQHigh": "4678.58", "NQLow": "4643.78", "NQClose": "4675.14", "NQVolume": "1773060000", "NQAdjClose": "4675.14\r", "uid": 7 }, { "Date": "11/11/2017", "SPOpen": "2038.2", "SPHigh": "2041.28", "SPLow": "2035.28", "SPClose": "2039.68", "SPVolume": "2958320000", "SPAdjClose": "2039.68", "NQOpen": "4649.19", "NQHigh": "4661.23", "NQLow": "4640.24", "NQClose": "4660.56", "NQVolume": "1663690000", "NQAdjClose": "4660.56\r", "uid": 8 }, { "Date": "11/10/2017", "SPOpen": "2032.01", "SPHigh": "2038.7", "SPLow": "2030.17", "SPClose": "2038.26", "SPVolume": "3284940000", "SPAdjClose": "2038.26", "NQOpen": "4635.1", "NQHigh": "4653.38", "NQLow": "4626.49", "NQClose": "4651.62", "NQVolume": "1830010000", "NQAdjClose": "4651.62\r", "uid": 9 }, { "Date": "11/7/2017", "SPOpen": "2032.36", "SPHigh": "2034.26", "SPLow": "2025.07", "SPClose": "2031.92", "SPVolume": "3704280000", "SPAdjClose": "2031.92", "NQOpen": "4636.89", "NQHigh": "4638.8", "NQLow": "4606.81", "NQClose": "4632.53", "NQVolume": "1978830000", "NQAdjClose": "4632.53\r", "uid": 10 }, { "Date": "11/6/2017", "SPOpen": "2023.33", "SPHigh": "2031.61", "SPLow": "2015.86", "SPClose": "2031.21", "SPVolume": "3669770000", "SPAdjClose": "2031.21", "NQOpen": "4616.78", "NQHigh": "4639.17", "NQLow": "4604.76", "NQClose": "4638.47", "NQVolume": "1986820000", "NQAdjClose": "4638.47\r", "uid": 11 }, { "Date": "11/5/2017", "SPOpen": "2015.29", "SPHigh": "2023.77", "SPLow": "2017.42", "SPClose": "2023.57", "SPVolume": "3766590000", "SPAdjClose": "2023.57", "NQOpen": "4649.47", "NQHigh": "4650.39", "NQLow": "4607.73", "NQClose": "4620.72", "NQVolume": "2001870000", "NQAdjClose": "4620.72\r", "uid": 12 }, { "Date": "11/4/2017", "SPOpen": "2015.81", "SPHigh": "2015.98", "SPLow": "2001.01", "SPClose": "2015.1", "SPVolume": "3956260000", "SPAdjClose": "2015.1", "NQOpen": "4623.77", "NQHigh": "4635.95", "NQLow": "4594.92", "NQClose": "4623.64", "NQVolume": "1939320000", "NQAdjClose": "4623.64\r", "uid": 13 }, { "Date": "11/3/2017", "SPOpen": "2018.21", "SPHigh": "2024.46", "SPLow": "2016.68", "SPClose": "2017.81", "SPVolume": "3555440000", "SPAdjClose": "2017.81", "NQOpen": "4633.71", "NQHigh": "4654.19", "NQLow": "4627.42", "NQClose": "4638.91", "NQVolume": "2033000000", "NQAdjClose": "4638.91\r", "uid": 14 }, { "Date": "10/31/2017", "SPOpen": "2001.2", "SPHigh": "2018.19", "SPLow": "2001.2", "SPClose": "2018.05", "SPVolume": "4292290000", "SPAdjClose": "2018.05", "NQOpen": "4639.45", "NQHigh": "4641.51", "NQLow": "4616.58", "NQClose": "4630.74", "NQVolume": "2424360000", "NQAdjClose": "4630.74\r", "uid": 15 }, { "Date": "10/30/2017", "SPOpen": "1979.49", "SPHigh": "1999.4", "SPLow": "1974.75", "SPClose": "1994.65", "SPVolume": "3586150000", "SPAdjClose": "1994.65", "NQOpen": "4532.1", "NQHigh": "4575.5", "NQLow": "4521.79", "NQClose": "4566.14", "NQVolume": "2034960000", "NQAdjClose": "4566.14\r", "uid": 16 }, { "Date": "10/29/2017", "SPOpen": "1983.29", "SPHigh": "1991.4", "SPLow": "1969.04", "SPClose": "1982.3", "SPVolume": "3740350000", "SPAdjClose": "1982.3", "NQOpen": "4551.37", "NQHigh": "4564.44", "NQLow": "4517.02", "NQClose": "4549.23", "NQVolume": "2184050000", "NQAdjClose": "4549.23\r", "uid": 17 }, { "Date": "10/28/2017", "SPOpen": "1964.14", "SPHigh": "1985.05", "SPLow": "1964.14", "SPClose": "1985.05", "SPVolume": "3653260000", "SPAdjClose": "1985.05", "NQOpen": "4505.73", "NQHigh": "4564.29", "NQLow": "4505.11", "NQClose": "4564.29", "NQVolume": "1966920000", "NQAdjClose": "4564.29\r", "uid": 18 }, { "Date": "10/27/2017", "SPOpen": "1962.97", "SPHigh": "1964.64", "SPLow": "1951.37", "SPClose": "1961.63", "SPVolume": "3538860000", "SPAdjClose": "1961.63", "NQOpen": "4469.02", "NQHigh": "4489.6", "NQLow": "4450.29", "NQClose": "4485.93", "NQVolume": "1585580000", "NQAdjClose": "4485.93\r", "uid": 19 }, { "Date": "10/24/2017", "SPOpen": "1951.59", "SPHigh": "1965.27", "SPLow": "1946.27", "SPClose": "1964.58", "SPVolume": "3078380000", "SPAdjClose": "1964.58", "NQOpen": "4459.46", "NQHigh": "4486.26", "NQLow": "4445.85", "NQClose": "4483.72", "NQVolume": "1754300000", "NQAdjClose": "4483.72\r", "uid": 20 }, { "Date": "10/23/2017", "SPOpen": "1931.02", "SPHigh": "1961.95", "SPLow": "1931.02", "SPClose": "1950.82", "SPVolume": "3789250000", "SPAdjClose": "1950.82", "NQOpen": "4427.44", "NQHigh": "4475.55", "NQLow": "4421.56", "NQClose": "4452.79", "NQVolume": "1952380000", "NQAdjClose": "4452.79\r", "uid": 21 }, { "Date": "10/22/2017", "SPOpen": "1941.29", "SPHigh": "1949.31", "SPLow": "1926.83", "SPClose": "1927.11", "SPVolume": "3761930000", "SPAdjClose": "1927.11", "NQOpen": "4429.16", "NQHigh": "4435.86", "NQLow": "4381.28", "NQClose": "4382.85", "NQVolume": "1967020000", "NQAdjClose": "4382.85\r", "uid": 22 }, { "Date": "10/21/2017", "SPOpen": "1909.38", "SPHigh": "1942.45", "SPLow": "1909.38", "SPClose": "1941.28", "SPVolume": "3987090000", "SPAdjClose": "1941.28", "NQOpen": "4359.17", "NQHigh": "4419.48", "NQLow": "4356.1", "NQClose": "4419.48", "NQVolume": "1997580000", "NQAdjClose": "4419.48\r", "uid": 23 }, { "Date": "10/20/2017", "SPOpen": "1885.62", "SPHigh": "1905.03", "SPLow": "1882.3", "SPClose": "1904.01", "SPVolume": "3331210000", "SPAdjClose": "1904.01", "NQOpen": "4254.16", "NQHigh": "4316.87", "NQLow": "4248.22", "NQClose": "4316.07", "NQVolume": "1717370000", "NQAdjClose": "4316.07\r", "uid": 24 }, { "Date": "10/17/2017", "SPOpen": "1864.91", "SPHigh": "1898.16", "SPLow": "1864.91", "SPClose": "1886.76", "SPVolume": "4482120000", "SPAdjClose": "1886.76", "NQOpen": "4275.09", "NQHigh": "4296.11", "NQLow": "4241.67", "NQClose": "4258.44", "NQVolume": "2260070000", "NQAdjClose": "4258.44\r", "uid": 25 }, { "Date": "10/16/2017", "SPOpen": "1855.95", "SPHigh": "1876.01", "SPLow": "1835.02", "SPClose": "1862.76", "SPVolume": "5073150000", "SPAdjClose": "1862.76", "NQOpen": "4133.25", "NQHigh": "4246.01", "NQLow": "4131.65", "NQClose": "4217.39", "NQVolume": "2591940000", "NQAdjClose": "4217.39\r", "uid": 26 }, { "Date": "10/15/2017", "SPOpen": "1874.18", "SPHigh": "1874.18", "SPLow": "1820.66", "SPClose": "1862.49", "SPVolume": "6090800000", "SPAdjClose": "1862.49", "NQOpen": "4154.1", "NQHigh": "4231.54", "NQLow": "4116.6", "NQClose": "4215.32", "NQVolume": "3058740000", "NQAdjClose": "4215.32\r", "uid": 27 }, { "Date": "10/14/2017", "SPOpen": "1877.11", "SPHigh": "1898.71", "SPLow": "1871.79", "SPClose": "1877.7", "SPVolume": "4812013000", "SPAdjClose": "1877.7", "NQOpen": "4246.23", "NQHigh": "4281.34", "NQLow": "4212.82", "NQClose": "4227.17", "NQVolume": "2496120000", "NQAdjClose": "4227.17\r", "uid": 28 }, { "Date": "10/13/2017", "SPOpen": "1905.65", "SPHigh": "1912.09", "SPLow": "1874.14", "SPClose": "1874.74", "SPVolume": "4352580000", "SPAdjClose": "1874.74", "NQOpen": "4274.91", "NQHigh": "4303.82", "NQLow": "4212.87", "NQClose": "4213.66", "NQVolume": "63104908800", "NQAdjClose": "4213.66\r", "uid": 29 }, { "Date": "10/10/2017", "SPOpen": "1925.63", "SPHigh": "1936.98", "SPLow": "1906.05", "SPClose": "1906.13", "SPVolume": "4550540000", "SPAdjClose": "1906.13", "NQOpen": "4354.63", "NQHigh": "4380.51", "NQLow": "4276.24", "NQClose": "4276.24", "NQVolume": "3097360000", "NQAdjClose": "4276.24\r", "uid": 30 }, { "Date": "10/9/2017", "SPOpen": "1967.68", "SPHigh": "1967.68", "SPLow": "1927.56", "SPClose": "1928.21", "SPVolume": "4324020000", "SPAdjClose": "1928.21", "NQOpen": "4458.29", "NQHigh": "4464.13", "NQLow": "4377.28", "NQClose": "4378.34", "NQVolume": "2264220000", "NQAdjClose": "4378.34\r", "uid": 31 }, { "Date": "10/8/2017", "SPOpen": "1935.55", "SPHigh": "1970.36", "SPLow": "1925.25", "SPClose": "1968.89", "SPVolume": "4441890000", "SPAdjClose": "1968.89", "NQOpen": "4385.7", "NQHigh": "4473.73", "NQLow": "4355.34", "NQClose": "4468.59", "NQVolume": "2451630000", "NQAdjClose": "4468.59\r", "uid": 32 }, { "Date": "10/7/2017", "SPOpen": "1962.36", "SPHigh": "1962.36", "SPLow": "1934.87", "SPClose": "1935.1", "SPVolume": "3687870000", "SPAdjClose": "1935.1", "NQOpen": "4433.91", "NQHigh": "4441.76", "NQLow": "4385.15", "NQClose": "4385.2", "NQVolume": "2111360000", "NQAdjClose": "4385.2\r", "uid": 33 }, { "Date": "10/6/2017", "SPOpen": "1970.01", "SPHigh": "1977.84", "SPLow": "1958.43", "SPClose": "1964.82", "SPVolume": "3358690000", "SPAdjClose": "1964.82", "NQOpen": "4492.4", "NQHigh": "4496.26", "NQLow": "4444.1", "NQClose": "4454.8", "NQVolume": "1828240000", "NQAdjClose": "4454.8\r", "uid": 34 }, { "Date": "10/3/2017", "SPOpen": "1948.12", "SPHigh": "1971.19", "SPLow": "1948.12", "SPClose": "1967.9", "SPVolume": "3561320000", "SPAdjClose": "1967.9", "NQOpen": "4456.81", "NQHigh": "4488.07", "NQLow": "4445.72", "NQClose": "4475.62", "NQVolume": "1777640000", "NQAdjClose": "4475.62\r", "uid": 35 }, { "Date": "10/2/2017", "SPOpen": "1945.83", "SPHigh": "1952.32", "SPLow": "1926.03", "SPClose": "1946.17", "SPVolume": "4012510000", "SPAdjClose": "1946.17", "NQOpen": "4421.25", "NQHigh": "4441.95", "NQLow": "4367.74", "NQClose": "4430.2", "NQVolume": "2165500000", "NQAdjClose": "4430.2\r", "uid": 36 }, { "Date": "10/1/2017", "SPOpen": "1971.44", "SPHigh": "1971.44", "SPLow": "1941.72", "SPClose": "1946.16", "SPVolume": "4188590000", "SPAdjClose": "1946.16", "NQOpen": "4486.65", "NQHigh": "4486.79", "NQLow": "4409.3", "NQClose": "4422.09", "NQVolume": "2312630000", "NQAdjClose": "4422.09\r", "uid": 37 }, { "Date": "9/30/2017", "SPOpen": "1978.21", "SPHigh": "1985.17", "SPLow": "1968.96", "SPClose": "1972.29", "SPVolume": "3951100000", "SPAdjClose": "1972.29", "NQOpen": "4512.64", "NQHigh": "4522.06", "NQLow": "4483.91", "NQClose": "4493.39", "NQVolume": "2200380000", "NQAdjClose": "4493.39\r", "uid": 38 }, { "Date": "9/29/2017", "SPOpen": "1978.96", "SPHigh": "1981.28", "SPLow": "1964.04", "SPClose": "1977.8", "SPVolume": "3094440000", "SPAdjClose": "1977.8", "NQOpen": "4465.84", "NQHigh": "4515.24", "NQLow": "4464.44", "NQClose": "4505.85", "NQVolume": "1737750000", "NQAdjClose": "4505.85\r", "uid": 39 }, { "Date": "9/26/2017", "SPOpen": "1966.22", "SPHigh": "1986.37", "SPLow": "1966.22", "SPClose": "1982.85", "SPVolume": "2929440000", "SPAdjClose": "1982.85", "NQOpen": "4476.48", "NQHigh": "4515.75", "NQLow": "4475.48", "NQClose": "4512.19", "NQVolume": "1637480000", "NQAdjClose": "4512.19\r", "uid": 40 }, { "Date": "9/25/2017", "SPOpen": "1997.32", "SPHigh": "1997.32", "SPLow": "1965.99", "SPClose": "1965.99", "SPVolume": "3273050000", "SPAdjClose": "1965.99", "NQOpen": "4540.82", "NQHigh": "4546.93", "NQLow": "4466.64", "NQClose": "4466.75", "NQVolume": "1939610000", "NQAdjClose": "4466.75\r", "uid": 41 }, { "Date": "9/24/2017", "SPOpen": "1983.34", "SPHigh": "1999.79", "SPLow": "1978.63", "SPClose": "1998.3", "SPVolume": "3313850000", "SPAdjClose": "1998.3", "NQOpen": "4514.92", "NQHigh": "4557.27", "NQLow": "4500.13", "NQClose": "4555.22", "NQVolume": "1765260000", "NQAdjClose": "4555.22\r", "uid": 42 }, { "Date": "9/23/2017", "SPOpen": "1992.78", "SPHigh": "1995.41", "SPLow": "1982.77", "SPClose": "1982.77", "SPVolume": "3279350000", "SPAdjClose": "1982.77", "NQOpen": "4511.32", "NQHigh": "4536.03", "NQLow": "4508.42", "NQClose": "4508.69", "NQVolume": "1847730000", "NQAdjClose": "4508.69\r", "uid": 43 }, { "Date": "9/22/2017", "SPOpen": "2009.08", "SPHigh": "2009.08", "SPLow": "1991.01", "SPClose": "1994.29", "SPVolume": "3349670000", "SPAdjClose": "1994.29", "NQOpen": "4568.45", "NQHigh": "4568.87", "NQLow": "4513.12", "NQClose": "4527.69", "NQVolume": "1881520000", "NQAdjClose": "4527.69\r", "uid": 44 }, { "Date": "9/19/2017", "SPOpen": "2015.74", "SPHigh": "2019.26", "SPLow": "2006.59", "SPClose": "2013.4", "SPVolume": "4880220000", "SPAdjClose": "2013.4", "NQOpen": "4606.13", "NQHigh": "4610.57", "NQLow": "4563.44", "NQClose": "4579.79", "NQVolume": "3178490000", "NQAdjClose": "4579.79\r", "uid": 45 }, { "Date": "9/18/2017", "SPOpen": "2003.07", "SPHigh": "2015.34", "SPLow": "2003.07", "SPClose": "2014.36", "SPVolume": "3235340000", "SPAdjClose": "2014.36", "NQOpen": "4575.74", "NQHigh": "4593.98", "NQLow": "4572.62", "NQClose": "4593.43", "NQVolume": "1774840000", "NQAdjClose": "4593.43\r", "uid": 46 }, { "Date": "9/17/2017", "SPOpen": "1999.3", "SPHigh": "2013.74", "SPLow": "1993.29", "SPClose": "2001.57", "SPVolume": "3209420000", "SPAdjClose": "2001.57", "NQOpen": "4553.96", "NQHigh": "4582.4", "NQLow": "4539.36", "NQClose": "4562.19", "NQVolume": "1796710000", "NQAdjClose": "4562.19\r", "uid": 47 }, { "Date": "9/16/2017", "SPOpen": "1981.93", "SPHigh": "2002.28", "SPLow": "1979.06", "SPClose": "1998.98", "SPVolume": "3160310000", "SPAdjClose": "1998.98", "NQOpen": "4502.11", "NQHigh": "4558.24", "NQLow": "4499.87", "NQClose": "4552.76", "NQVolume": "1879350000", "NQAdjClose": "4552.76\r", "uid": 48 }, { "Date": "9/15/2017", "SPOpen": "1986.04", "SPHigh": "1987.18", "SPLow": "1978.48", "SPClose": "1984.13", "SPVolume": "2776530000", "SPAdjClose": "1984.13", "NQOpen": "4567.45", "NQHigh": "4567.47", "NQLow": "4506.73", "NQClose": "4518.9", "NQVolume": "1940520000", "NQAdjClose": "4518.9\r", "uid": 49 }, { "Date": "9/12/2017", "SPOpen": "1996.74", "SPHigh": "1996.74", "SPLow": "1980.26", "SPClose": "1985.54", "SPVolume": "3206570000", "SPAdjClose": "1985.54", "NQOpen": "4588.77", "NQHigh": "4590.08", "NQLow": "4555.68", "NQClose": "4567.6", "NQVolume": "1784170000", "NQAdjClose": "4567.6\r", "uid": 50 }, { "Date": "9/11/2017", "SPOpen": "1992.85", "SPHigh": "1997.65", "SPLow": "1985.93", "SPClose": "1997.45", "SPVolume": "2941690000", "SPAdjClose": "1997.45", "NQOpen": "4567.64", "NQHigh": "4591.81", "NQLow": "4559.75", "NQClose": "4591.81", "NQVolume": "1704850000", "NQAdjClose": "4591.81\r", "uid": 51 }, { "Date": "9/10/2017", "SPOpen": "1988.41", "SPHigh": "1996.66", "SPLow": "1982.99", "SPClose": "1995.69", "SPVolume": "2912430000", "SPAdjClose": "1995.69", "NQOpen": "4554.15", "NQHigh": "4587.1", "NQLow": "4544.84", "NQClose": "4586.52", "NQVolume": "1808650000", "NQAdjClose": "4586.52\r", "uid": 52 }, { "Date": "9/9/2017", "SPOpen": "2000.73", "SPHigh": "2001.01", "SPLow": "1984.61", "SPClose": "1988.44", "SPVolume": "2882830000", "SPAdjClose": "1988.44", "NQOpen": "4588.83", "NQHigh": "4599.03", "NQLow": "4544.44", "NQClose": "4552.29", "NQVolume": "1956550000", "NQAdjClose": "4552.29\r", "uid": 53 }, { "Date": "9/8/2017", "SPOpen": "2007.17", "SPHigh": "2007.17", "SPLow": "1995.6", "SPClose": "2001.54", "SPVolume": "2789090000", "SPAdjClose": "2001.54", "NQOpen": "4579.06", "NQHigh": "4600.4", "NQLow": "4570.23", "NQClose": "4592.29", "NQVolume": "1670210000", "NQAdjClose": "4592.29\r", "uid": 54 }, { "Date": "9/5/2017", "SPOpen": "1998", "SPHigh": "2007.71", "SPLow": "1990.1", "SPClose": "2007.71", "SPVolume": "2818300000", "SPAdjClose": "2007.71", "NQOpen": "4560.63", "NQHigh": "4583", "NQLow": "4542.74", "NQClose": "4582.9", "NQVolume": "1641830000", "NQAdjClose": "4582.9\r", "uid": 55 }, { "Date": "9/4/2017", "SPOpen": "2001.67", "SPHigh": "2014.17", "SPLow": "1992.54", "SPClose": "1997.65", "SPVolume": "3072410000", "SPAdjClose": "1997.65", "NQOpen": "4581.52", "NQHigh": "4603.15", "NQLow": "4553.31", "NQClose": "4562.29", "NQVolume": "1728700000", "NQAdjClose": "4562.29\r", "uid": 56 }, { "Date": "9/3/2017", "SPOpen": "2003.57", "SPHigh": "2009.28", "SPLow": "1998.14", "SPClose": "2000.72", "SPVolume": "2809980000", "SPAdjClose": "2000.72", "NQOpen": "4610.14", "NQHigh": "4610.14", "NQLow": "4565.38", "NQClose": "4572.56", "NQVolume": "1897450000", "NQAdjClose": "4572.56\r", "uid": 57 }, { "Date": "9/2/2017", "SPOpen": "2004.07", "SPHigh": "2006.12", "SPLow": "1994.85", "SPClose": "2002.28", "SPVolume": "2819980000", "SPAdjClose": "2002.28", "NQOpen": "4592.42", "NQHigh": "4598.64", "NQLow": "4576.81", "NQClose": "4598.19", "NQVolume": "1859080000", "NQAdjClose": "4598.19\r", "uid": 58 }]; Smart('#chart', class { get properties() { return { caption: 'NASDAQ and S&P 500 - OHLC Example', description: '(September 2017 - November 2017)', animationDuration: 1500, enableCrosshairs: true, padding: { left: 5, top: 5, right: 5, bottom: 5 }, dataSource: sampleData, colorScheme: 'scheme21', xAxis: { dataField: 'Date', type: 'date', valuesOnTicks: true, }, seriesGroups: [ { type: 'ohlc', valueAxis: { description: 'S&P 500<br>', minValue: 1800 }, series: [ { dataFieldClose: 'SPClose', displayTextClose: 'S&P Close price', dataFieldOpen: 'SPOpen', displayTextOpen: 'S&P Open price', dataFieldHigh: 'SPHigh', displayTextHigh: 'S&P High price', dataFieldLow: 'SPLow', displayTextLow: 'S&P Low price', displayText: 'S&P 500', lineWidth: 1 } ] } ] }; } }); </script> </head> <body> <smart-chart id="chart"></smart-chart> </body> </html>
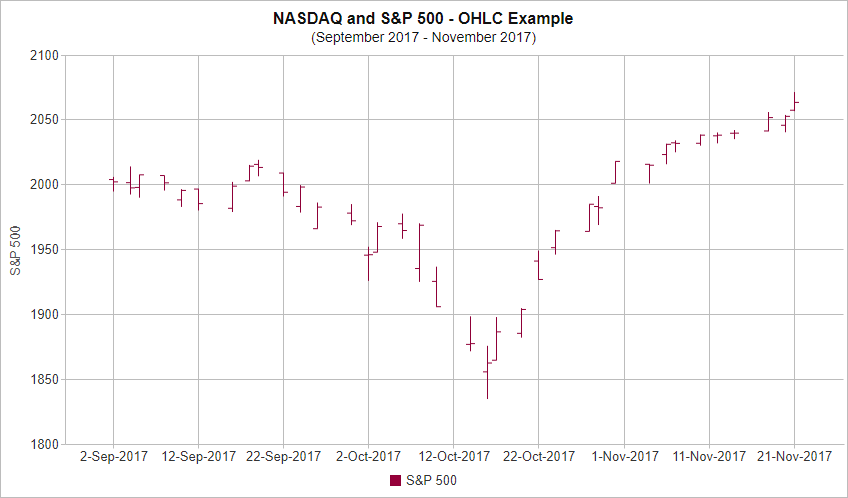
By changing the series group type to 'candlestick', we get the following visualization of the data:
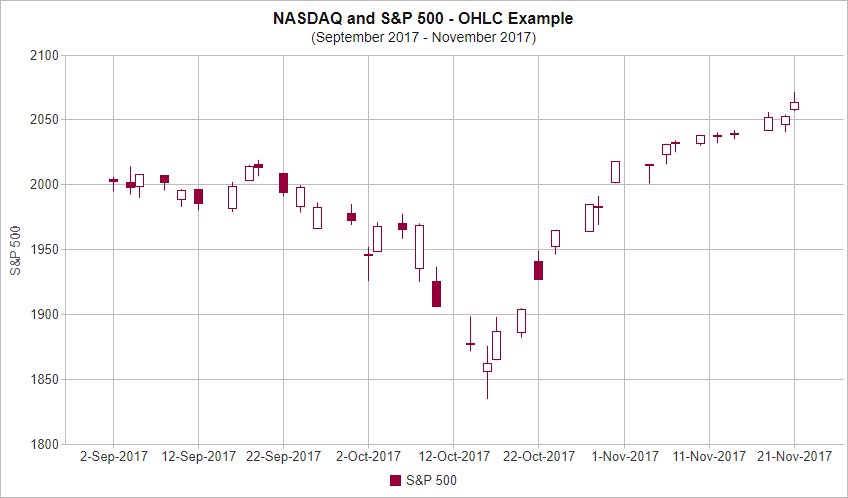