Blazor - Get Started with Smart.QueryBuilder
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic QueryBuilder
Smart.QueryBuilder is a custom component that allows you to easily build queries with complex structures. The output of the component is a JSON object with the query.
Add the QueryBuilder component to the Pages/Index.razor file
<QueryBuilder></QueryBuilder>
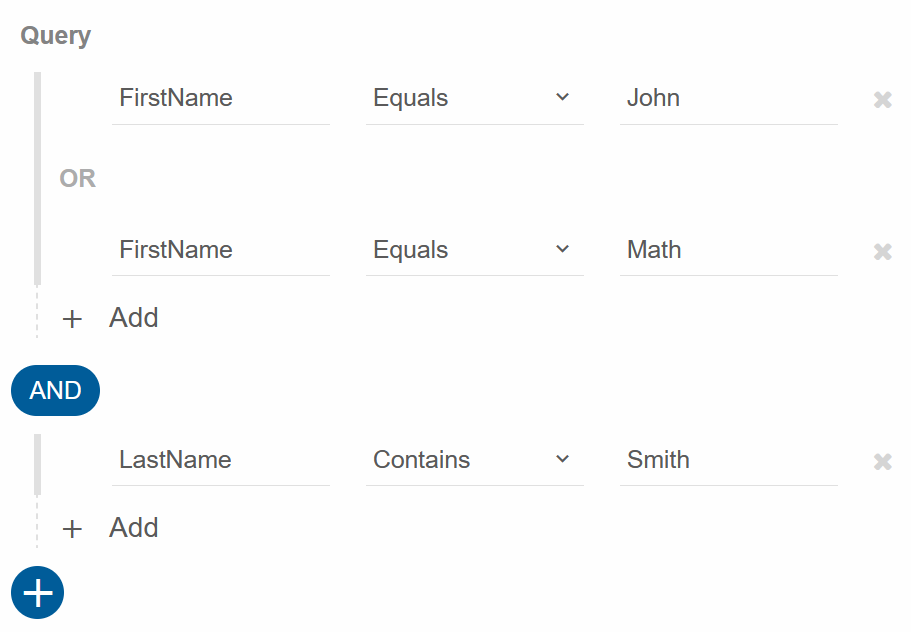
QueryBuilder Fields
Fields are set as an Array of QueryBuilderField
objects.
Each field must have Label, DataField, DataType.
The optional FilterOperations allows you to select the which operations are available to the user
<QueryBuilder Fields="fields"></QueryBuilder> @code{ private List<QueryBuilderField> fields = new List<QueryBuilderField>() { new QueryBuilderField() { Label = "Id", DataField = "id", DataType = "number" }, new QueryBuilderField() { Label = "Product", DataField = "productName", DataType = "string" }, new QueryBuilderField() { Label = "Unit Price", DataField = "price", DataType = "number", FilterOperations = new string[]{"=", "<", ">"} }, new QueryBuilderField() { Label = "Purchased", DataField = "purchased", DataType = "date" }, new QueryBuilderField() { Label = "Available", DataField = "available", DataType = "boolean" } }; }
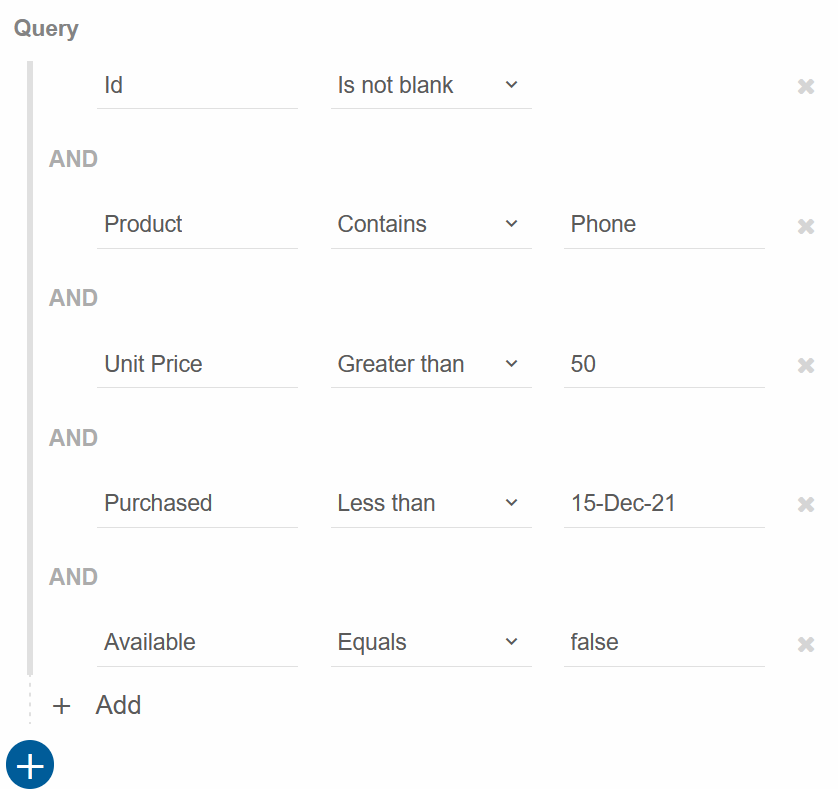
Note that the Value input fields automatically changes depending on the fields's DataType:
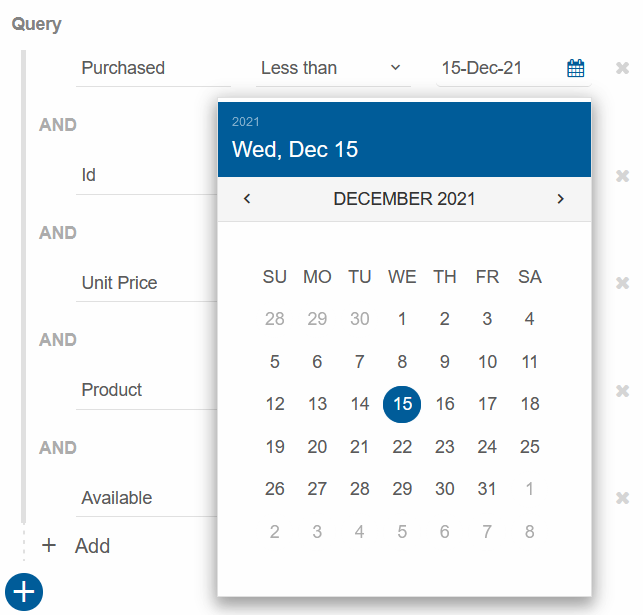
Custom Operations
Smart.QueryBuilder allows you to create custom operations that can be added to the query bilder's conditions structure.
After creating a new custom operation, add it to a field's options list using the FilterOperations
:
<QueryBuilder Fields="fields" CustomOperations="customOperations"></QueryBuilder> @code{ private List<QueryBuilderField> fields = new List<QueryBuilderField>() { ..... new QueryBuilderField() { Label = "Product", DataField = "productName", DataType = "string", FilterOperations = new string[]{"=", "MatchRegex"} }, new QueryBuilderField() { Label = "Unit Price", DataField = "price", DataType = "number", FilterOperations = new string[]{"=", "<", ">"} }, .... }; private List<QueryBuilderCustomOperation> customOperations = new List<QueryBuilderCustomOperation>{ new QueryBuilderCustomOperation(){ Label="Match Regex", Name="MatchRegex" }, new QueryBuilderCustomOperation(){ Label="Match Regex", Name="MatchRegex" } }; }
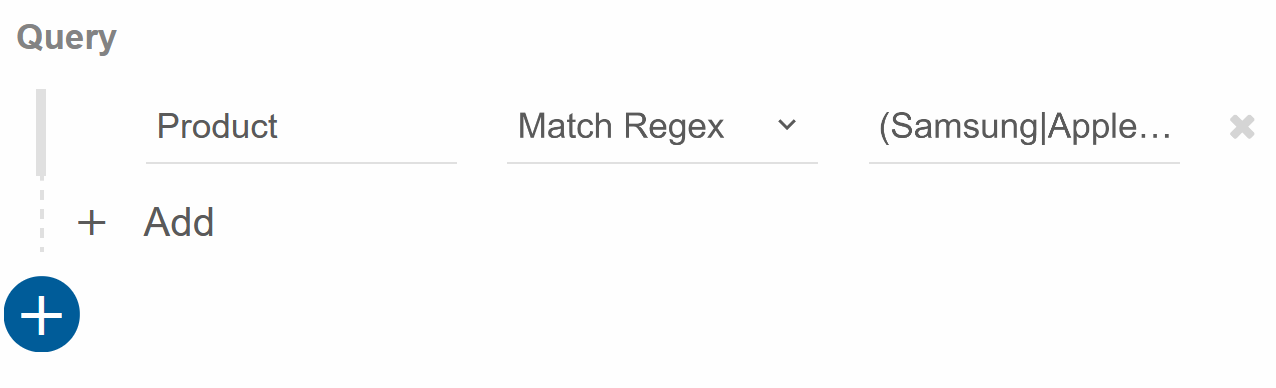
Allow Drag
The built-in Dragging functionality can be enabled by the AllowDrag
:
<QueryBuilder Fields="fields" CustomOperations="customOperations" AllowDrag="true"></QueryBuilder>
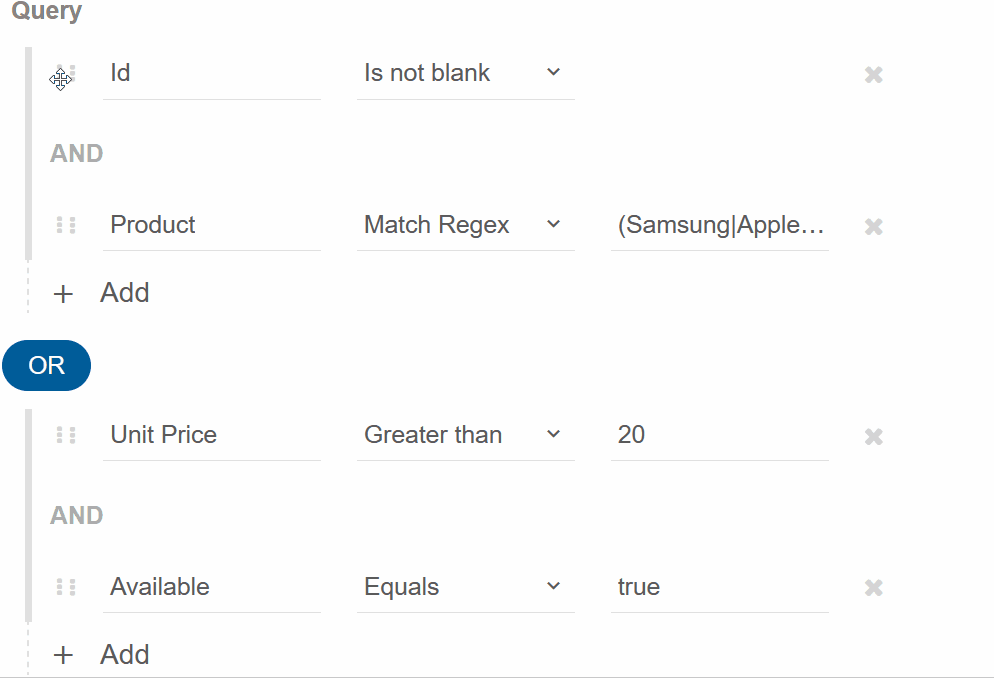
Events
Smart.QueryBulder provides built-in Events that can help you expand the component's
functionality.
The event object can have unique event.detail parameters.
OnChange
- triggered when the query builder's value is changed.
Event Details
: dynamic item, dynamic data, dynamic originalEventOnDragEnd
- triggered when a dragged condition is dropped.
Event Details
: dynamic item, dynamic data, dynamic target, dynamic targetData, string targetSideOnDragging
- triggered when a condition is being dragged.
Event Details
: dynamic item, dynamic data, dynamic originalEventOnDragStart
- triggered when a dragging operation is started.
Event Details
: dynamic item, dynamic data, dynamic originalEventOnItemClick
- triggered when one of the query builder's building blocks ( operator, fieldName, value, close button, etc) is clicked.
Event Details
: string id, dynamic type, dynamic dataOnPropertySelected
- triggered when a field has been selected.
Event Details
: string label, dynamic value
<h2 style="min-height:40px;">@property <QueryBuilder Fields="fields" CustomOperations="customOperations" AllowDrag="true" OnPropertySelected="OnPropertySelected"></QueryBuilder> @code{ private List<QueryBuilderField> fields = new List<QueryBuilderField>() { new QueryBuilderField() { Label = "Id", DataField = "id", DataType = "number" }, new QueryBuilderField() { Label = "Product", DataField = "productName", DataType = "string", FilterOperations = new string[]{"=", "MatchRegex"} }, new QueryBuilderField() { Label = "Unit Price", DataField = "price", DataType = "number", FilterOperations = new string[]{"=", "<", ">"} }, new QueryBuilderField() { Label = "Purchased", DataField = "purchased", DataType = "date" }, new QueryBuilderField() { Label = "Available", DataField = "available", DataType = "boolean" } }; private List<QueryBuilderCustomOperation> customOperations = new List<QueryBuilderCustomOperation>{ new QueryBuilderCustomOperation(){ Label="Match Regex", Name="MatchRegex" }, new QueryBuilderCustomOperation(){ Label="Match Regex", Name="MatchRegex" } }; string property; private void OnPropertySelected(Event ev){ if(ev.ContainsKey("Detail")){ QueryBuilderPropertySelectedEventDetail detail = ev["Detail"]; property = detail.Label; } } }
The demo below display the last selected property:
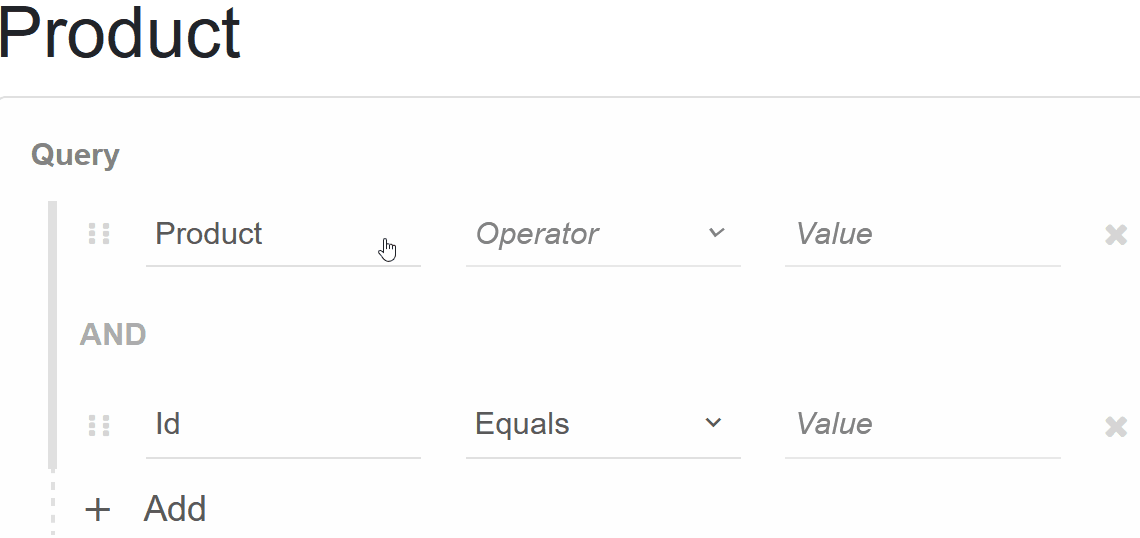