Refresh Angular Grid Data Source
Setup The Angular Application
Follow the Getting Started guide to set up your Angular application with Smart UI.
Setup the Angular Smart.Grid Component
Follow the Angular Grid guide to read more about how you can use the Angular Grid component.
Refresh Data Source
You can add and delete records or update the data source of the Grid by calling the related methods of the dataSource property.
For example you can maniputate the bound data by using external buttons.
Adding Records
You can add records to the Grid using the insert method of the dataSource
, like this:
that.grid.dataSource.insert(0, data);
Deleting Records
You can remove records using the removeAt method of the dataSource
, like this:
that.grid.dataSource.removeAt(0);
Updating Data Source
You can update all records by assigning new source to the dataSource
, like this:
that.grid.dataSource = new window.Smart.DataAdapter({ dataSource: data, dataFields: [ 'id: number', 'firstName: string', 'lastName: string', 'productName: string', 'quantity: number', 'price: number', 'total: number' ] });
Example
Here is the code of the full example:app.component.html:
<smart-grid [dataSource]="dataSource" [columns]="columns" #grid id="grid"></smart-grid> <div class="options"> <div class="option"> <smart-button #updateBtn id="updateBtn">Update New DataSource</smart-button> </div> <div class="option"> <smart-button #addRowBtn id="addRowBtn">Add Row</smart-button> </div> <div class="option"> <smart-button #deleteRow id="deleteRow">Delete Row</smart-button> </div> </div>app.component.ts:
import { Component, ViewChild, OnInit, AfterViewInit } from '@angular/core'; import { ButtonComponent } from 'smart-webcomponents-angular/button'; import { GridComponent, GridColumn, Smart } from 'smart-webcomponents-angular/grid'; import { GetData } from '../assets/data'; @Component({ selector: 'app-root', templateUrl: './app.component.html' }) export class AppComponent implements AfterViewInit, OnInit { @ViewChild('updateBtn', { read: ButtonComponent, static: false }) updateBtn!: ButtonComponent; @ViewChild('addRowBtn', { read: ButtonComponent, static: false }) addRowBtn!: ButtonComponent; @ViewChild('deleteRow', { read: ButtonComponent, static: false }) deleteRow!: ButtonComponent; @ViewChild('grid', { read: GridComponent, static: false }) grid!: GridComponent; dataSource = new Smart.DataAdapter({ dataSource: GetData(500), dataFields: [ 'id: number', 'firstName: string', 'lastName: string', 'productName: string', 'quantity: number', 'price: number', 'total: number' ] }) columns: GridColumn[] = [ { label: 'First Name', dataField: 'firstName', columnGroup: 'name' }, { label: 'Last Name', dataField: 'lastName', columnGroup: 'name' }, { label: 'Product', dataField: 'productName', columnGroup: 'order' }, { label: 'Quantity', dataField: 'quantity', columnGroup: 'order' }, { label: 'Unit Price', dataField: 'price', cellsFormat: 'c2', columnGroup: 'order'}, { label: 'Total', dataField: 'total', cellsFormat: 'c2', columnGroup: 'order' } ] ngOnInit(): void { // onInit code. } ngAfterViewInit(): void { const that = this; // afterViewInit code. this.updateBtn.addEventListener('click', () => { that.grid.dataSource = new window.Smart.DataAdapter({ dataSource: GetData(500), dataFields: [ 'id: number', 'firstName: string', 'lastName: string', 'productName: string', 'quantity: number', 'price: number', 'total: number' ] }); }); this.addRowBtn.addEventListener('click', () => { const data = GetData(1)[0]; that.grid.dataSource.insert(0, data); }); this.deleteRow.addEventListener('click', () => { that.grid.dataSource.removeAt(0); }); } }assets/data.ts:
interface IRowGenerateData { id: number; reportsTo: number | null; available: boolean | null; firstName: string; lastName: string; name: string; productName: string; quantity: string | number; total: string | number; price: string | number; date: Date; leaf: boolean; } export function GetData(rowscount?: number, last?: number, hasNullValues?: boolean): IRowGenerateData[] { const data: IRowGenerateData[] = new Array(); if (rowscount === undefined) { rowscount = 100; } let startIndex = 0; if (last) { startIndex = rowscount; rowscount = last - rowscount; } const firstNames = [ 'Andrew', 'Nancy', 'Shelley', 'Regina', 'Yoshi', 'Antoni', 'Mayumi', 'Ian', 'Peter', 'Lars', 'Petra', 'Martin', 'Sven', 'Elio', 'Beate', 'Cheryl', 'Michael', 'Guylene' ]; const lastNames = [ 'Fuller', 'Davolio', 'Burke', 'Murphy', 'Nagase', 'Saavedra', 'Ohno', 'Devling', 'Wilson', 'Peterson', 'Winkler', 'Bein', 'Petersen', 'Rossi', 'Vileid', 'Saylor', 'Bjorn', 'Nodier' ]; const productNames = [ 'Black Tea', 'Green Tea', 'Caffe Espresso', 'Doubleshot Espresso', 'Caffe Latte', 'White Chocolate Mocha', 'Caramel Latte', 'Caffe Americano', 'Cappuccino', 'Espresso Truffle', 'Espresso con Panna', 'Peppermint Mocha Twist' ]; const priceValues = [ '2.25', '1.5', '3.0', '3.3', '4.5', '3.6', '3.8', '2.5', '5.0', '1.75', '3.25', '4.0' ]; for (let i = 0; i < rowscount; i++) { const row = {} as IRowGenerateData; const productindex = Math.floor(Math.random() * productNames.length); const price = parseFloat(priceValues[productindex]); const quantity = 1 + Math.round(Math.random() * 10); row.id = startIndex + i; row.reportsTo = Math.floor(Math.random() * firstNames.length); if (i % Math.floor(Math.random() * firstNames.length) === 0) { row.reportsTo = null; } row.available = productindex % 2 === 0; if (hasNullValues === true) { if (productindex % 2 !== 0) { const random = Math.floor(Math.random() * rowscount); row.available = i % random === 0 ? null : false; } } row.firstName = firstNames[Math.floor(Math.random() * firstNames.length)]; row.lastName = lastNames[Math.floor(Math.random() * lastNames.length)]; row.name = row.firstName + ' ' + row.lastName; row.productName = productNames[productindex]; row.price = price; row.quantity = quantity; row.total = price * quantity; const date = new Date(); date.setFullYear(2016, Math.floor(Math.random() * 11), Math.floor(Math.random() * 27)); date.setHours(0, 0, 0, 0); row.date = date; data[i] = row; } return data; }Result:
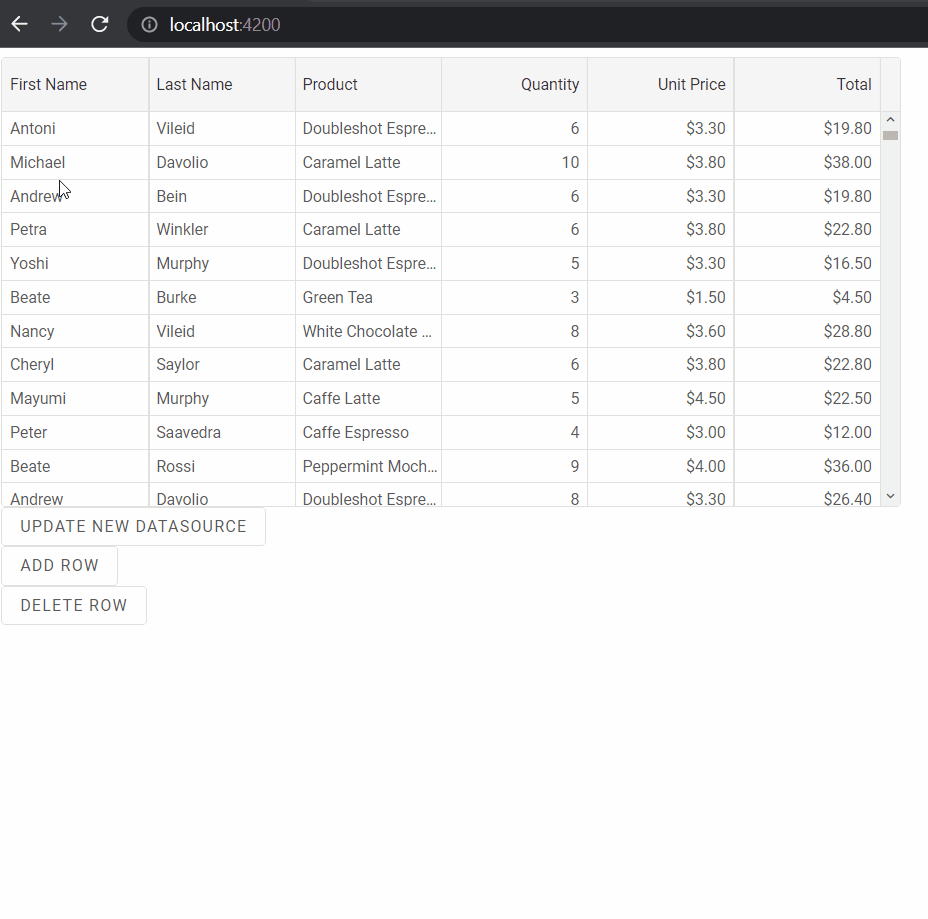