Build your web apps using Smart UI
Smart.TimePicker - configuration and usage
Overview
Smart.TimePicker represents an element used for selection of time (hours and minutes).
Getting Started with TimePicker Web Component
Smart UI for Web Components is distributed as smart-webcomponents NPM package. You can also get the full download from our website with all demos from the Download page.Setup the TimePicker
Smart UI for Web Components is distributed as smart-webcomponents NPM package
- Download and install the package.
npm install smart-webcomponents
- Once installed, import the TimePicker module in your application.
<script type="module" src="node_modules/smart-webcomponents/source/modules/smart.timepicker.js"></script>
-
Adding CSS reference
The smart.default.css CSS file should be referenced using following code.
<link rel="stylesheet" type="text/css" href="node_modules/smart-webcomponents/source/styles/smart.default.css" />
- Add the TimePicker tag to your Web Page
<smart-time-picker id="timepicker"></smart-time-picker>
- Create the TimePicker Component
<script type="module"> Smart('#timepicker', class { get properties() { return { format: "24-hour" } } }); </script>
Another option is to create the TimePicker is by using the traditional Javascript way:
const timepicker = document.createElement('smart-time-picker'); timepicker.disabled = true; document.body.appendChild(timepicker);
Smart framework provides a way to dynamically create a web component on demand from a DIV tag which is used as a host. The following imports the web component's module and creates it on demand, when the document is ready. The #timepicker is the ID of a DIV tag.
import "../../source/modules/smart.timepicker.js"; document.readyState === 'complete' ? init() : window.onload = init; function init() { const timepicker = new Smart.TimePicker('#timepicker', { format: "24-hour" }); }
- Open the page in your web server.
The following code adds the custom element to the page.
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> <script type="text/javascript" src="../../source/smart.element.js"></script> <script type="text/javascript" src="../../source/smart.numeric.js"></script> <script type="text/javascript" src="../../source/smart.math.js"></script> <script type="text/javascript" src="../../source/smart.draw.js"></script> <script type="text/javascript" src="../../source/smart.timepicker.js"></script> </head> <body> <smart-time-picker></smart-time-picker> </body> </html>
Note how smart.element.js is declared before everything else. This is mandatory for all custom elements.
Demo
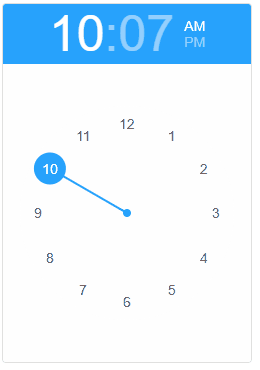
Smart.TimePicker accepts javascript Date objects as time values. The value property is responsible for the time value of the element. Like every custom element property it can be set as an attribute inside the HTML tag of the element:
<smart-time-picker value="new Date(2017,12,1,12,25)"></smart-time-picker>
Demo
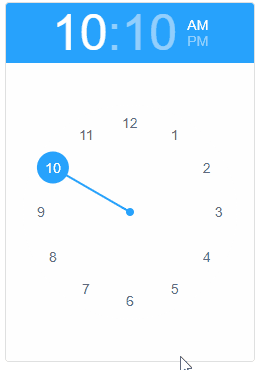
or using javascript:
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> <script type="text/javascript" src="../../source/smart.element.js"></script> <script type="text/javascript" src="../../source/smart.numeric.js"></script> <script type="text/javascript" src="../../source/smart.math.js"></script> <script type="text/javascript" src="../../source/smart.draw.js"></script> <script type="text/javascript" src="../../source/smart.timepicker.js"></script> <script> window.onload = function () { document.querySelector('smart-time-picker').value = new Date(2017, 12, 1, 15, 24, 0); } </script> </head> <body> <smart-time-picker></smart-time-picker> </body> </html>
Demo
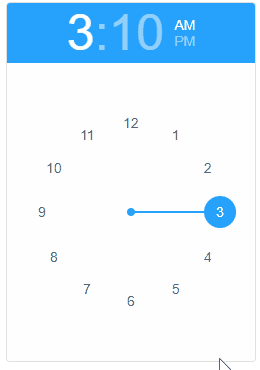
Appearance
Smart.TimePicker supoprts 12 and 24 hour time format. By default the element uses 12 hour format but the user can change that via the format property like so:
<smart-time-picker format="24-hour"></smart-time-picker>
Demo
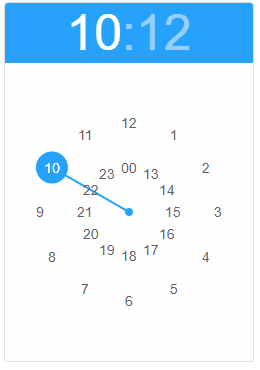
The time picker has two orientation modes that can be changed using the view property. The possible values are:
- landscape - horizontally positioned
- portrait - vertically positioned. Default value.
The property can be set as HTML attribute:
<smart-time-picker view="landscape"></smart-time-picker>
Demo
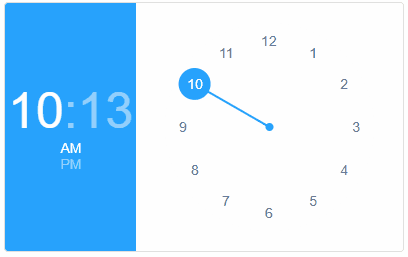
or javascript:
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> <script type="text/javascript" src="../../source/smart.element.js"></script> <script type="text/javascript" src="../../source/smart.numeric.js"></script> <script type="text/javascript" src="../../source/smart.math.js"></script> <script type="text/javascript" src="../../source/smart.draw.js"></script> <script type="text/javascript" src="../../source/smart.timepicker.js"></script> <script> window.onload = function () { document.querySelector('smart-time-picker').view = 'landscape'; } </script> </head> <body> <smart-time-picker></smart-time-picker> </body> </html>
Demo
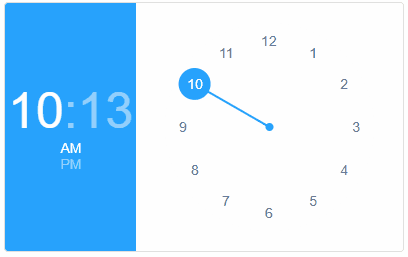
The element has a footer section that is hidden by default. The visibility of the section is controlled by the footer property. The contneto of the footer can be customized using an HTML Template. Here's how to enable the footer and place HTML content inside:
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> <script type="text/javascript" src="../../source/smart.element.js"></script> <script type="text/javascript" src="../../source/smart.button.js"></script> <script type="text/javascript" src="../../source/smart.numeric.js"></script> <script type="text/javascript" src="../../source/smart.math.js"></script> <script type="text/javascript" src="../../source/smart.draw.js"></script> <script type="text/javascript" src="../../source/smart.timepicker.js"></script> </head> <body> <template id="footerTemplate"> <smart-button>Close</smart-button> <smart-button>Ok</smart-button> </template> <smart-time-picker footer footer-template="footerTemplate"></smart-time-picker> </body> </html>
Demo
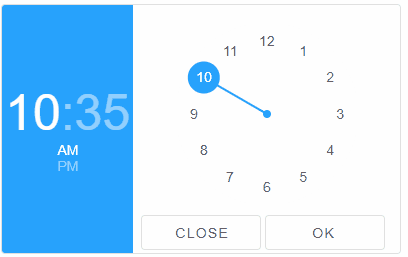
The same can be accomplished via javascript:
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> <script type="text/javascript" src="../../source/smart.element.js"></script> <script type="text/javascript" src="../../source/smart.button.js"></script> <script type="text/javascript" src="../../source/smart.numeric.js"></script> <script type="text/javascript" src="../../source/smart.math.js"></script> <script type="text/javascript" src="../../source/smart.draw.js"></script> <script type="text/javascript" src="../../source/smart.timepicker.js"></script> <script> window.onload = function () { var timePicker = document.querySelector('smart-time-picker'); timePicker.footerTemplate = 'footerTemplate'; timePicker.footer = true; } </script> </head> <body> <template id="footerTemplate"> <smart-button>Close</smart-button> <smart-button>Ok</smart-button> </template> <smart-time-picker></smart-time-picker> </body> </html>
Demo
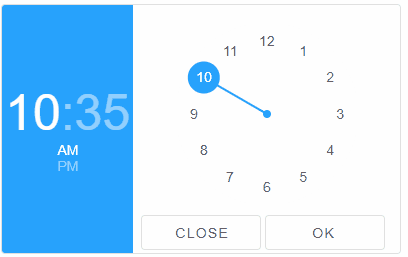
Behavior
Time selection is devided into hour selection and minute selection and the user can switch between the views by clicking on the appropriate part of the time string. However the user can enable the autoSwitchToMinutes property which redirects the user to the minute selection view after selecting an hour.
<smart-time-picker auto-switch-to-minutes></smart-time-picker>
Demo
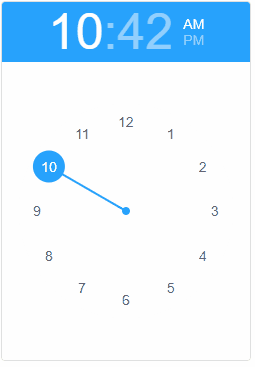
The selection view can also be controlled via the selection property. The available options are:
- hour - hour selection.
- minute - minute selection.
The view can be changed at any time using javascript or applied on HTML initialization:
<smart-time-picker selection="minute"></smart-time-picker>
Demo
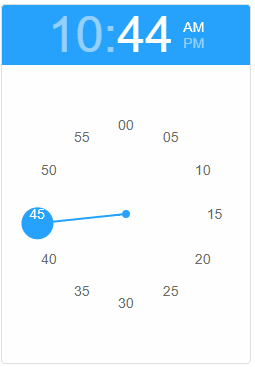
The user can also change the minute interval. This can be accomplished by applying the new minute interval in numeric format to the minuteInterval property.
<smart-time-picker selection="minute" minute-interval="15"></smart-time-picker>
Demo
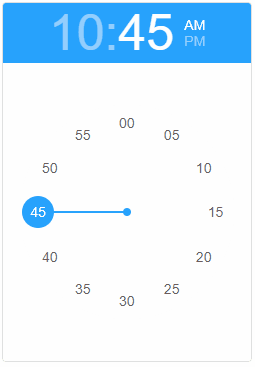
Styling
Styling Smart.TimePicker is done exclusively trough CSS class selectors.
<!DOCTYPE html> <html> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> <script type="text/javascript" src="../../source/smart.element.js"></script> <script type="text/javascript" src="../../source/smart.numeric.js"></script> <script type="text/javascript" src="../../source/smart.math.js"></script> <script type="text/javascript" src="../../source/smart.draw.js"></script> <script type="text/javascript" src="../../source/smart.timepicker.js"></script> <style> smart-time-picker { width: 150px; height: 300px; } smart-time-picker .smart-main-container { background-color: #EEEEEE; } </style> </head> <body> <smart-time-picker></smart-time-picker> </body> </html>
Demo
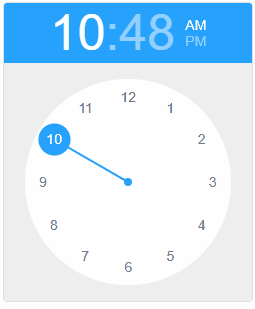
Create, Append, Remove, Get/Set Property, Invoke Method, Bind to Event
Create a new element:
const timepicker = document.createElement('smart-time-picker');
Append it to the DOM:
document.body.appendChild(timepicker);
Remove it from the DOM:
timepicker.parentNode.removeChild(timepicker);
Set a property:
timepicker.propertyName = propertyValue;
Get a property value:
const propertyValue = timepicker.propertyName;
Invoke a method:
timepicker.methodName(argument1, argument2);
Add Event Listener:
const eventHandler = (event) => { // your code here. }; timepicker.addEventListener(eventName, eventHandler);
Remove Event Listener:
timepicker.removeEventListener(eventName, eventHandler, true);
Using with Typescript
Smart Web Components package includes TypeScript definitions which enables strongly-typed access to the Smart UI Components and their configuration.
Inside the download package, the typescript directory contains .d.ts file for each web component and a smart.elements.d.ts typescript definitions file for all web components. Copy the typescript definitions file to your project and in your TypeScript file add a reference to smart.elements.d.ts
Read more about using Smart UI with Typescript.Getting Started with Angular TimePicker Component
Setup Angular Environment
Angular provides the easiest way to set angular CLI projects using Angular CLI tool.
Install the CLI application globally to your machine.
npm install -g @angular/cli
Create a new Application
ng new smart-angular-timepicker
Navigate to the created project folder
cd smart-angular-timepicker
Setup the TimePicker
Smart UI for Angular is distributed as smart-webcomponents-angular NPM package
- Download and install the package.
npm install smart-webcomponents-angular
- Adding CSS reference
The following CSS file is available in ../node_modules/smart-webcomponents-angular/ package folder. This can be referenced in [src/styles.css] using following code.@import 'smart-webcomponents-angular/source/styles/smart.default.css';
Another way to achieve the same is to edit the angular.json file and in the styles add the style."styles": [ "node_modules/smart-webcomponents-angular/source/styles/smart.default.css" ]
If you want to use Bootstrap, Fluent or other themes available in the package, you need to add them after 'smart.default.css'. -
Example with Angular Standalone Components
app.component.html
<smart-time-picker #timepicker></smart-time-picker>
app.component.ts
import { Component, ViewChild, OnInit, AfterViewInit } from '@angular/core'; import { TimePickerComponent } from 'smart-webcomponents-angular/timepicker'; import { CommonModule } from '@angular/common'; import { RouterOutlet } from '@angular/router'; import { TimePickerModule } from 'smart-webcomponents-angular/timepicker'; @Component({ selector: 'app-root', standalone: true, imports: [CommonModule, TimePickerModule, RouterOutlet], templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements AfterViewInit, OnInit { @ViewChild('timepicker', { read: TimePickerComponent, static: false }) timepicker!: TimePickerComponent; ngOnInit(): void { // onInit code. } ngAfterViewInit(): void { // afterViewInit code. this.init(); } init(): void { // init code. } }
-
Example with Angular NGModule
app.component.html
<smart-time-picker #timepicker></smart-time-picker>
app.component.ts
import { Component, ViewChild, OnInit, AfterViewInit } from '@angular/core'; import { TimePickerComponent } from 'smart-webcomponents-angular/timepicker'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements AfterViewInit, OnInit { @ViewChild('timepicker', { read: TimePickerComponent, static: false }) timepicker!: TimePickerComponent; ngOnInit(): void { // onInit code. } ngAfterViewInit(): void { // afterViewInit code. this.init(); } init(): void { // init code. } }
app.module.ts
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { TimePickerModule } from 'smart-webcomponents-angular/timepicker'; import { AppComponent } from './app.component'; @NgModule({ declarations: [AppComponent], imports: [BrowserModule, TimePickerModule], bootstrap: [AppComponent] }) export class AppModule { }
Running the Angular application
After completing the steps required to render a TimePicker, run the following command to display the output in your web browser
ng serveand open localhost:4200 in your favorite web browser.
Read more about using Smart UI for Angular: https://www.htmlelements.com/docs/angular-cli/.
Getting Started with React TimePicker Component
Setup React Environment
The easiest way to start with React is to use NextJS Next.js is a full-stack React framework. It’s versatile and lets you create React apps of any size—from a mostly static blog to a complex dynamic application.
npx create-next-app my-app cd my-app npm run devor
yarn create next-app my-app cd my-app yarn run dev
Preparation
Setup the TimePicker
Smart UI for React is distributed as smart-webcomponents-react package
- Download and install the package.
In your React Next.js project, run one of the following commands to install Smart UI TimePicker for ReactWith NPM:
npm install smart-webcomponents-react
With Yarn:yarn add smart-webcomponents-react
- Once installed, import the React TimePicker Component and CSS files in your application and render it.
app.js
import 'smart-webcomponents-react/source/styles/smart.default.css'; import React from "react"; import ReactDOM from 'react-dom/client'; import { TimePicker } from 'smart-webcomponents-react/timepicker'; class App extends React.Component { init() { } componentDidMount() { } render() { return ( <div> <TimePicker></TimePicker> </div> ); } } export default App;
Running the React application
Start the app withnpm run devor
yarn run devand open localhost:3000 in your favorite web browser to see the output.
Setup with Vite
Vite (French word for "quick", pronounced /vit/, like "veet") is a build tool that aims to provide a faster and leaner development experience for modern web projectsWith NPM:
npm create vite@latestWith Yarn:
yarn create viteThen follow the prompts and choose React as a project.
Navigate to your project's directory. By default it is 'vite-project' and install Smart UI for React
In your Vite project, run one of the following commands to install Smart UI TimePicker for ReactWith NPM:
npm install smart-webcomponents-reactWith Yarn:
yarn add smart-webcomponents-reactOpen src/App.tsx App.tsx
import 'smart-webcomponents-react/source/styles/smart.default.css'; import React from "react"; import ReactDOM from 'react-dom/client'; import { TimePicker } from 'smart-webcomponents-react/timepicker'; class App extends React.Component { init() { } componentDidMount() { } render() { return ( <div> <TimePicker></TimePicker> </div> ); } } export default App;
Read more about using Smart UI for React: https://www.htmlelements.com/docs/react/.
Getting Started with Vue TimePicker Component
Setup Vue with Vite
In this section we will introduce how to scaffold a Vue Single Page Application on your local machine. The created project will be using a build setup based on Vite and allow us to use Vue Single-File Components (SFCs). Run the following command in your command linenpm create vue@latestThis command will install and execute create-vue, the official Vue project scaffolding tool. You will be presented with prompts for several optional features such as TypeScript and testing support:
✔ Project name: …If you are unsure about an option, simply choose No by hitting enter for now. Once the project is created, follow the instructions to install dependencies and start the dev server:✔ Add TypeScript? … No / Yes ✔ Add JSX Support? … No / Yes ✔ Add Vue Router for Single Page Application development? … No / Yes ✔ Add Pinia for state management? … No / Yes ✔ Add Vitest for Unit testing? … No / Yes ✔ Add an End-to-End Testing Solution? … No / Cypress / Playwright ✔ Add ESLint for code quality? … No / Yes ✔ Add Prettier for code formatting? … No / Yes Scaffolding project in ./ ... Done.
cdnpm install npm install smart-webcomponents npm run dev
-
Make Vue ignore custom elements defined outside of Vue (e.g., using the Web Components APIs). Otherwise, it will throw a warning about an Unknown custom element, assuming that you forgot to register a global component or misspelled a component name.
Open vite.config.js in your favorite text editor and change its contents to the following:
vite.config.js
import { fileURLToPath, URL } from 'node:url' import { defineConfig } from 'vite' import vue from '@vitejs/plugin-vue' // https://vitejs.dev/config/ export default defineConfig({ plugins: [ vue({ template: { compilerOptions: { isCustomElement: tag => tag.startsWith('smart-') } } }) ], resolve: { alias: { '@': fileURLToPath(new URL('./src', import.meta.url)) } } })
-
Open src/App.vue in your favorite text editor and change its contents to the following:
App.vue
<template> <div class="vue-root"> <smart-time-picker></smart-time-picker> </div> </template> <script> import { onMounted } from "vue"; import "smart-webcomponents/source/styles/smart.default.css"; import "smart-webcomponents/source/modules/smart.timepicker.js"; export default { name: "app", setup() { onMounted(() => {}); } }; </script> <style> </style>
We can now use the smart-time-picker with Vue 3. Data binding and event handlers will just work right out of the box.
Running the Vue application
Start the app withnpm run devand open http://localhost:5173/ in your favorite web browser to see the output below:
When you are ready to ship your app to production, run the following:
npm run buildThis will create a production-ready build of your app in the project's ./dist directory.
Read more about using Smart UI for Vue: https://www.htmlelements.com/docs/vue/.