Gantt Resources
Gantt Resources allow you to provide additional information about the tasks.
By default, they are displayed inside the Resource View Panel.
Resources are set as an array of key-value pairs inside the Resources
property of a Task.
When Resources are set, Smart.Gantt will automatically create a Resource View Panel.
@using System.Text.Json.Serialization; <GanttChart DataSource = "Tasks" TaskColumns = "taskColumns" View = "GanttChartView.Week" ResourceFiltering="true"> </GanttChart> @code{ public partial class GanttDataRecord { [JsonPropertyName("id")] public string Id { get; set; } [JsonPropertyName("label")] public string Label { get; set; } [JsonPropertyName("dateStart")] public string DateStart { get; set; } [JsonPropertyName("dateEnd")] public string DateEnd { get; set; } [JsonPropertyName("class")] public string Class { get; set; } [JsonPropertyName("type")] public string Type { get; set; } [JsonPropertyName("progress")] public int Progress { get; set; } [JsonPropertyName("duration")] public int Duration { get; set; } [JsonPropertyName("synchronized")] public bool Synchronized { get; set; } [JsonPropertyName("expanded")] public bool Expanded { get; set; } [JsonPropertyName("tasks")] public List < GanttDataRecord > Tasks { get; set; } [JsonPropertyName("disableResources")] public bool DisableResources { get; set; } [JsonPropertyName("resources")] public object Resources { get; set; } = new object[] {}; [JsonPropertyName("connections")] public List < Dictionary < string, int >> Connections { get; set; } = new List < Dictionary < string, int >> () {}; [JsonPropertyName("disableDrag")] public bool DisableDrag { get; set; } [JsonPropertyName("disableResize")] public bool DisableResize { get; set; } [JsonPropertyName("minDateStart")] public string MinDateStart { get; set; } [JsonPropertyName("maxDateStart")] public string MaxDateStart { get; set; } [JsonPropertyName("minDateEnd")] public string MinDateEnd { get; set; } [JsonPropertyName("maxDateEnd")] public string MaxDateEnd { get; set; } [JsonPropertyName("minDuration")] public int MinDuration { get; set; } [JsonPropertyName("maxDuration")] public int MaxDuration { get; set; } [JsonPropertyName("value")] public int Value { get; set; } [JsonPropertyName("dragProject")] public bool DragProject { get; set; } } List < GanttChartTaskColumn > taskColumns = new List < GanttChartTaskColumn > () { new GanttChartTaskColumn() { Label = "Name", Value = "label", Size = "40%" }, new GanttChartTaskColumn() { Label = "From", Value = "dateStart", Size = "20%" }, new GanttChartTaskColumn() { Label = "To", Value = "dateEnd", Size = "20%" }, new GanttChartTaskColumn() { Label = "Owner", Value = "resources" } }; public List < GanttDataRecord > Tasks = new List < GanttDataRecord > () { new GanttDataRecord() { Label = "Development", Synchronized = true, Expanded = true, DisableResources = true, Type = "project", Tasks = new List < GanttDataRecord > () { new GanttDataRecord() { Label = "User Interface Design", DateStart = "2020-02-01", DateEnd = "2020-02-10", Progress = 50, Resources = new Dictionary < string, object > () { { "id", "megan" }, { "label", "Megan" } } }, new GanttDataRecord() { Label = "Controllers & Event", DateStart = "2020-02-05", DateEnd = "2020-02-20", Progress = 25, Type = "task", Resources = "megan" }, new GanttDataRecord() { Label = "Database Connectivity", DateStart = "2020-02-05", DateEnd = "2020-03-01", Progress = 50, Type = "task", Resources = new Dictionary < string, object > () { { "id", "taylor" }, { "label", "Taylor" } } } } }, new GanttDataRecord() { Label = "Quality Assurance", Synchronized = true, Expanded = true, DisableResources = true, Type = "project", Tasks = new List < GanttDataRecord > () { new GanttDataRecord() { Label = "User Interaction Testing", DateStart = "2020-03-11", DateEnd = "2020-03-20", Progress = 90, Type = "task", Resources = new Dictionary < string, object > () { { "id", "chris" }, { "label", "Chris" } } }, new GanttDataRecord() { Label = "Load and Stress Testing", DateStart = "2020-03-21", DateEnd = "2020-03-31", Progress = 45, Type = "task", Resources = "taylor" } } }, new GanttDataRecord() { Label = "Development", Synchronized = true, Expanded = true, DisableResources = true, Type = "project", Tasks = new List < GanttDataRecord > () { new GanttDataRecord() { Label = "URL Acquiring", DateStart = "2020-02-10", DateEnd = "2020-02-20", Type = "task", Resources = "chris" }, new GanttDataRecord() { Label = "Server Cost & Maintanance", DateStart = "2020-02-15", DateEnd = "2020-02-20", Progress = 75, Type = "task", Resources = new List < object > () { new Dictionary < string, object > () { { "id", "david" }, { "label", "David" } } } }, new GanttDataRecord() { Label = "Backup & Security", DateStart = "2020-02-21", DateEnd = "2020-03-01" }, new GanttDataRecord() { Label = "Updates", DateStart = "2020-02-08", DateEnd = "2020-03-10", Resources = new List < object > () { new Dictionary < string, object > () { { "id", "maria" }, { "label", "Maria" } } } } } } }; }
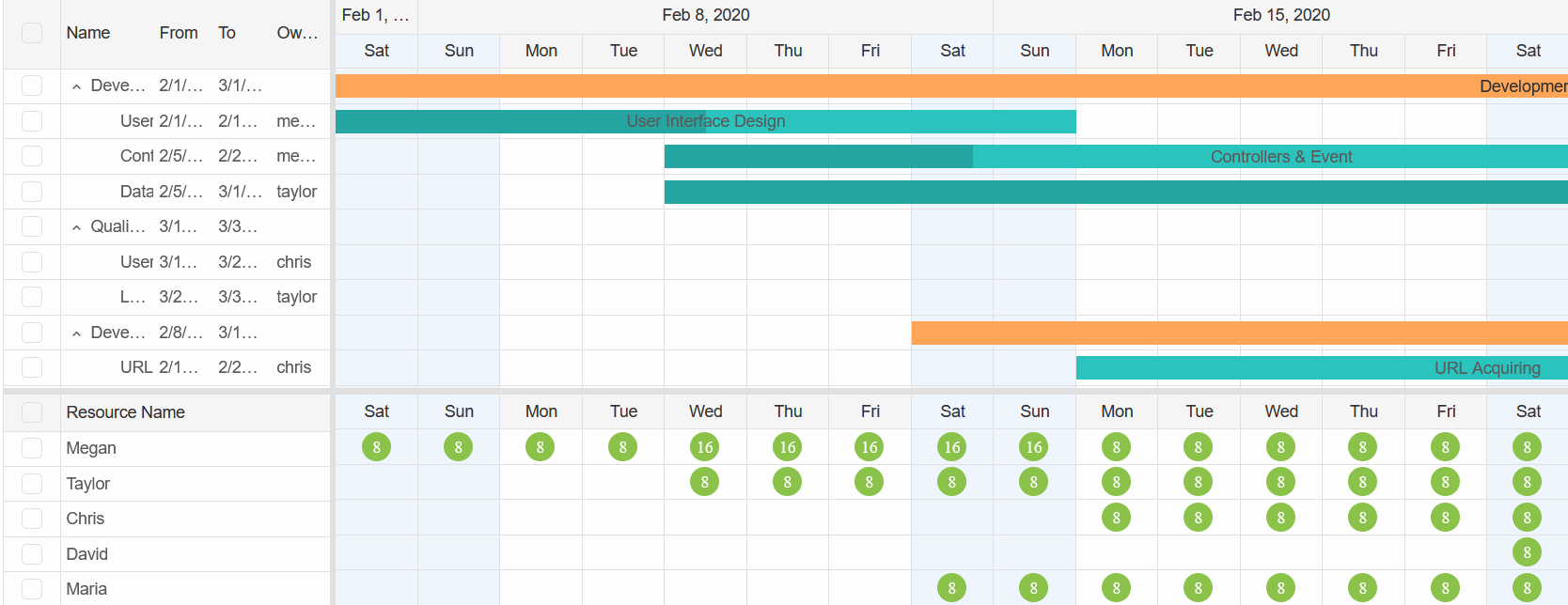
In addition, tasks can be selected using the Resource Panel - clicking on a Resource will highlight all tasks that share the Resource.
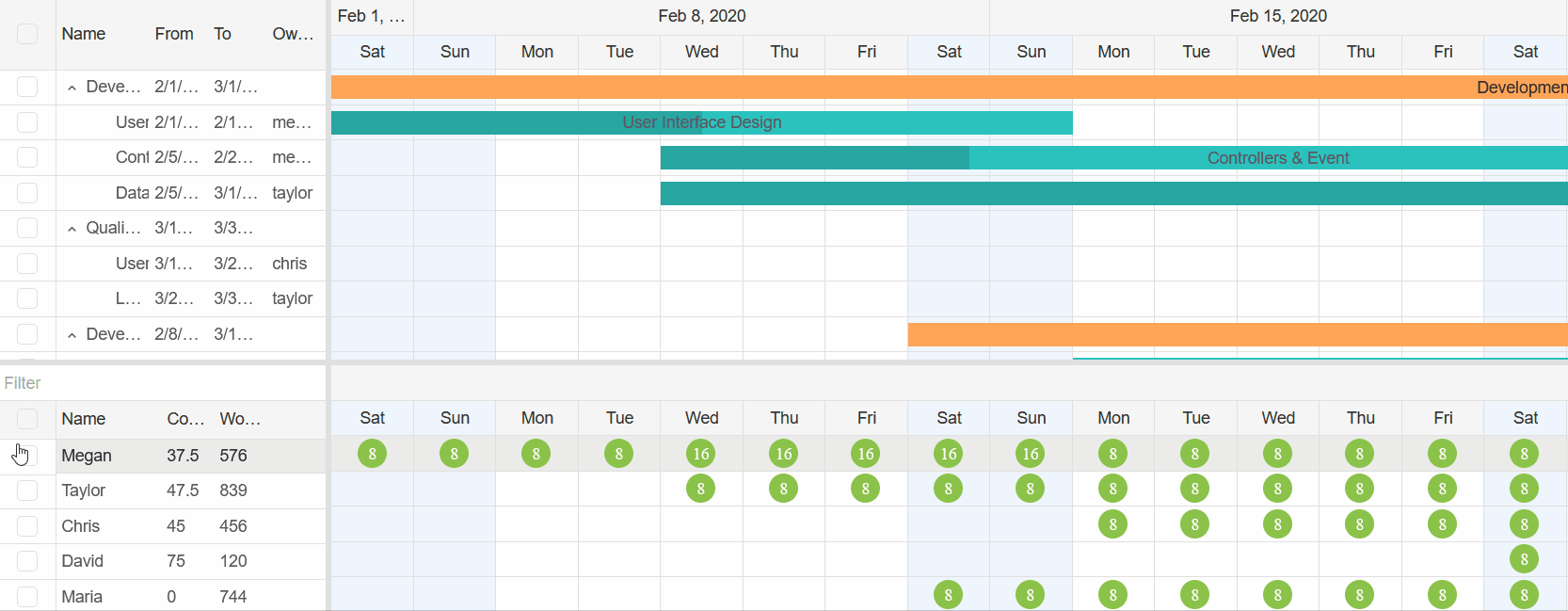
Resource Columns
Using the ResourceColumns
property, you can customize the columns displayed in the Resource View Panel.
Addiional properties, such as filtering and refresh rate, can also be set:
<GanttChart DataSource = "Tasks" TaskColumns = "taskColumns" View = "GanttChartView.Week" ResourceColumns = "resourceColumns" ResourceFiltering="true"> </GanttChart> @code{ List < GanttChartResourceColumn > resourceColumns = new List < GanttChartResourceColumn > () { new GanttChartResourceColumn() { Label = "Name", Value = "label", Size = "40%" }, new GanttChartResourceColumn() { Label = "Completion", Value = "progress", Size = "20%" }, new GanttChartResourceColumn() { Label = "Workload", Value = "workload", Size = "20%" } }; .... }
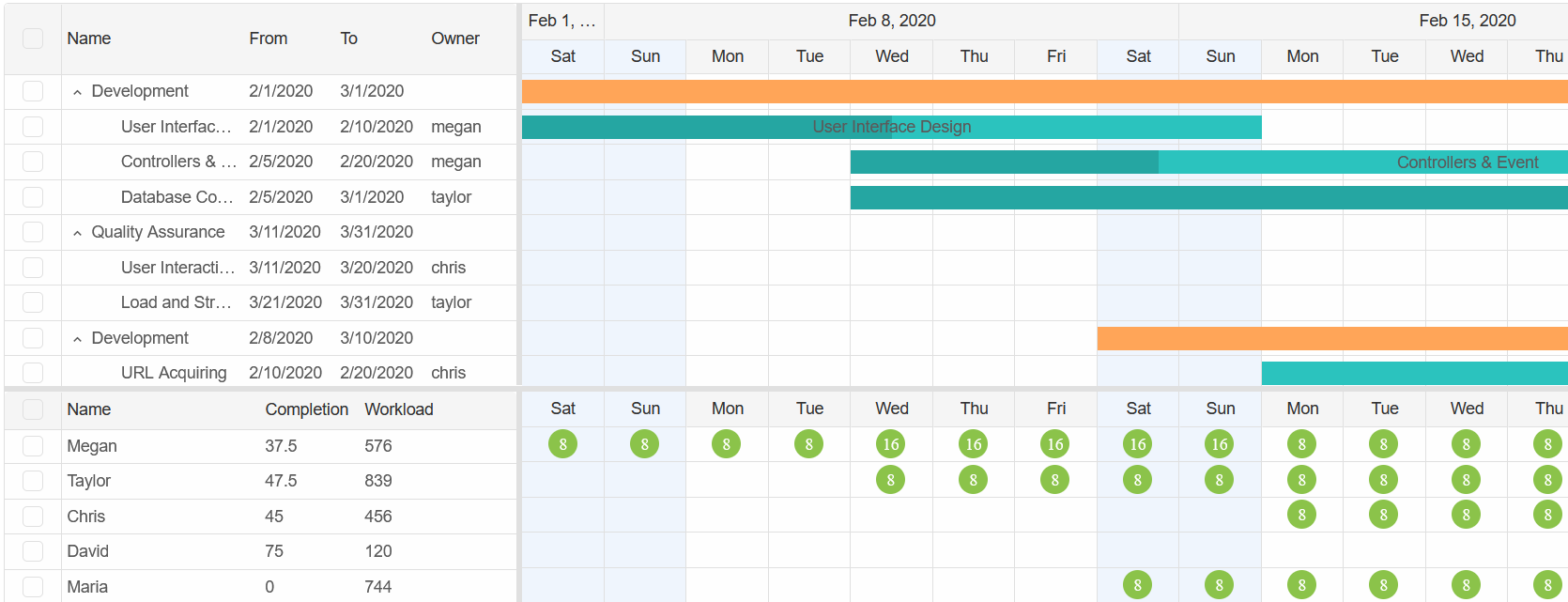
Resource TimeLineMode
The TimeLineMode determines how the capacity of the tasks will be visualized.
In Historgram
mode, the amount of tasks will be compared to the maximum capacity.
TimeLineMode measures the amout of tasks according to ResourceTimelineView
.
By default ResourceTimelineView
is set to Hours, but it can also be set to Tasks or Custom
<GanttChart DataSource = "Tasks" TaskColumns = "taskColumns" ResourceColumns = "resourceColumns" ResourcePanelSize = "150" View = "GanttChartView.Week" ResourceFiltering="true" ResourceTimelineMode="GanttChartResourceTimelineMode.Histogram"> </GanttChart>
