Selection
Smart.Grid provides multiple ways for the user to select data from the Grid - Row Selection, Checkbox Selection and Cell Selection.
In addition to many other customization options, it is also possible to set the Grid Selection Mode
- whether the user can select just one or multiple items
Row Selection
The default Grid Selection type is Row Selection. To enable row selection, create a GridSelection
object and set it as a property of the Grid
@inject WeatherForecastService ForecastService <Grid DataSource="@forecast" Selection="@selection"> <Columns> <Column DataField="Date" Label="Date" AllowReorder="true"> </Column> <Column DataField="TemperatureC" Label="Temp. (C)" AllowReorder="true"> </Column> <Column DataField="TemperatureF" Label="Temp. (F)" AllowReorder="true"> </Column> <Column DataField="Summary" Label="Summary" AllowReorder="true"> </Column> </Columns> </Grid> @code{ GridSelection selection = new GridSelection() { Enabled = true, Mode = GridSelectionMode.Many }; private WeatherForecast[] forecast; protected override async Task OnInitializedAsync() { forecast = await ForecastService.GetForecastAsync(DateTime.Now); } }
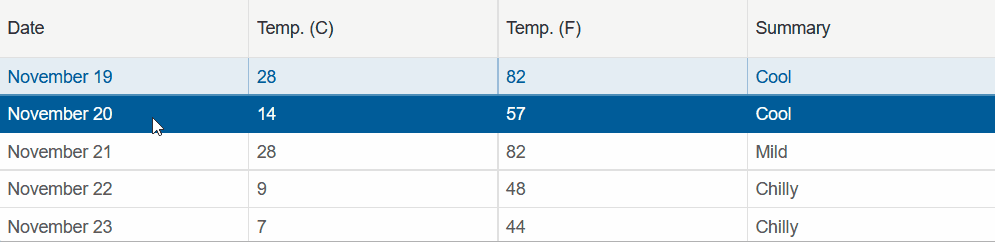
Note that multiple rows can be selected here since GridSelectionMode
is set to Many
CheckBox Selection
When CheckBox Selection is enabled, the Smart.Grid will automatically generate a new checkbox column, which can be used to select rows
GridSelection selection = new GridSelection() { Enabled = true, Mode = GridSelectionMode.Many, CheckBoxes = new GridSelectionCheckBoxes() { Enabled = true, Position = Position.Near } };
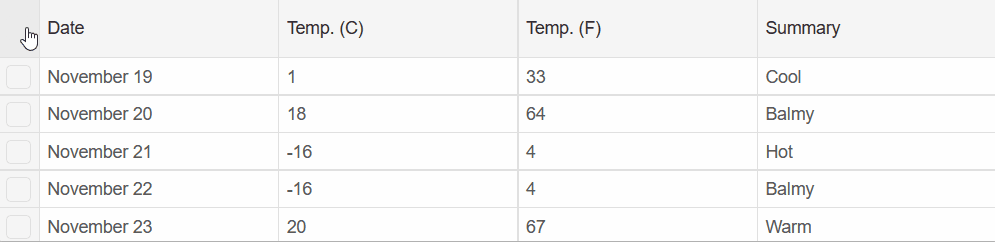
Note that the properties of the checkboxes are set inside the GridSelectionCheckBoxes
object
Cell Selection
It is possible to allow selection of individual cells, instead of the entire rows, using the AllowCellSelection
property
GridSelection selection = new GridSelection() { Enabled = true, AllowRowSelection = false, AllowCellSelection = true, AllowColumnHeaderSelection = true, Mode = GridSelectionMode.Many, CheckBoxes = new GridSelectionCheckBoxes() { Enabled = true, Position = Position.Near } };
Note that since AllowColumnHeaderSelection
is set to true
,
all cells of a column can be selected by clicking the column's header
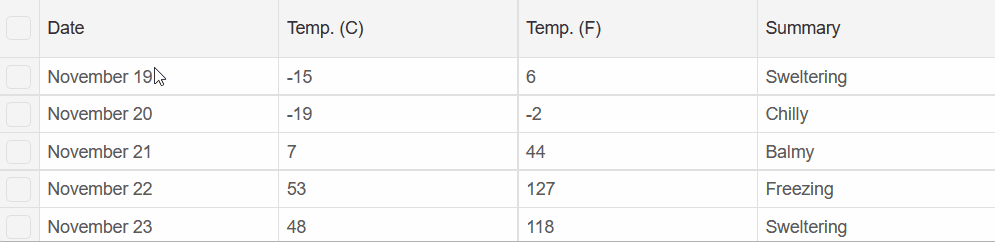
Selection Methods
Smart.Grid allows you to programmaticaly set or get the selected
state of a cell or row. Some of the main methods are:
GetSelectedCells
- Gets the selected cells. Returns an array of cells. Each cell is an array with row id, column data field and cell valueGetSelectedRows
- Gets the selected rows. Returns an arrat of the selected row idsGetSelectedRows
- Gets the selected rows. Returns an arrat of the selected row idsClearSelection
- Clears the selection that user has made. All row, cell and column selection highlights will be removedSelect
- Selects a row, cell or column. Expects:- rowId -
string | number
- dataField - not required -
string
- rowId -
SelectRows
- Select multiple rows by id. Expects array of rowId.Unselect
- Unselects a row, cell or column. Expects:- rowId -
string | number
- dataField - not required -
string
- rowId -