Build your web apps using Smart UI
Drawing API
Overview
The Draw object represents an object that can be used to plot SVG elements inside a container element.
To instantiate a Draw object, the user has to include the following files to the head of the page:
- smart.default.css - the CSS file containing the styles for SVG container;
- smart.element.js - the base class;
- smart.draw.js - the JS file containing the class Draw.
Initialization
A Draw object can be instantiated by calling the Draw class constructor with a single argument - the HTMLElement which will act as a parent for plotted SVG elements:
<!DOCTYPE html> <html> <head> <link type="text/css" rel="stylesheet" href="../../source/styles/smart.default.css" /> <style type="text/css"> #container { width: 500px; height: 500px; } </style> <script type="text/javascript" src="../../source/smart.element.js"></script> <script type="text/javascript" src="../../source/smart.draw.js"></script> <script type="text/javascript"> window.onload = function () { const draw = new Smart.Utilities.Draw(document.getElementById('container')); } </script> </head> <body> <div id="container"> </div> </body> </html>
Methods for Drawing Shapes
The class Draw provides methods for plotting various SVG shapes. These are listed below:
-
circle - draws a circle element. Returns a reference to the element. Parameters:
- x-coordinate of circle center
- y-coordinate of circle center
- radius of circle
- an object with applicable attributes (optional)
const draw = new Smart.Utilities.Draw(document.getElementById('container')); const circle = draw.circle(250, 250, 30, { 'class': 'myCircle' });
-
line - draws a line element. Returns a reference to the element. Parameters:
- x-coordinate of beginning
- y-coordinate of beginning
- x-coordinate of end
- y-coordinate of end
- an object with applicable attributes (optional)
const draw = new Smart.Utilities.Draw(document.getElementById('container')); const line = draw.line(0, 250, 500, 250, { 'id': 'myLine', 'class': 'SVGLine', 'stroke': 'red', 'stroke-width': 5 });
-
path - draws a path element. Returns a reference to the element. Parameters:
- line commands string
- an object with applicable attributes (optional)
const draw = new Smart.Utilities.Draw(document.getElementById('container')); const path = draw.path('M 0 150 L 250 0 L 500 150 Z', { 'id': 'myLine', 'class': 'SVGLine', 'stroke': 'red', 'stroke-width': 2, 'fill': 'orange' });
-
pieslice - draws a pie slice path element. Returns a reference to the element. Parameters:
- x-coordinate of center
- y-coordinate of center
- inner radius
- outer radius
- angle from
- angle to
- center offset
- an object with applicable attributes (optional)
const draw = new Smart.Utilities.Draw(document.getElementById('container')); const pieslice = draw.pieslice(250, 350, 10, 50, 30, 150, 0, { 'id': 'myPieslice', 'class': 'SVGPieslice', 'stroke': 'red', 'stroke-width': 2, 'fill': 'orange' });
-
rect - draws a rect element. Returns a reference to the element. Parameters:
- x-coordinate of top-left corner
- y-coordinate of top-left corner
- width
- height
- an object with applicable attributes (optional)
const draw = new Smart.Utilities.Draw(document.getElementById('container')); const rect = draw.rect(0, 150, 500, 50, { 'id': 'myPieslice', 'class': 'SVGPieslice', 'stroke': 'red', 'stroke-width': 2, 'fill': 'gray' });
-
text - draws a text element. Returns a reference to the element. Parameters:
- text label
- x-coordinate of beginning
- y-coordinate of beginning
- width
- height
- angle
- an object with applicable attributes (optional)
- clip
- halign
- valign
- rotateAround
const draw = new Smart.Utilities.Draw(document.getElementById('container')); const text = draw.text('Demo drawing', 100, 400, 0, 0, 20, { 'id': 'myТext', 'class': 'SVGТext', 'fill': 'gray', 'style': 'font-size: 50px;' });
Each of these methods returns an instance of an SVGElement that can be used to manipulate the element (e.g. add event handlers to it).
The example code provided for above methods results in the following SVG drawing:
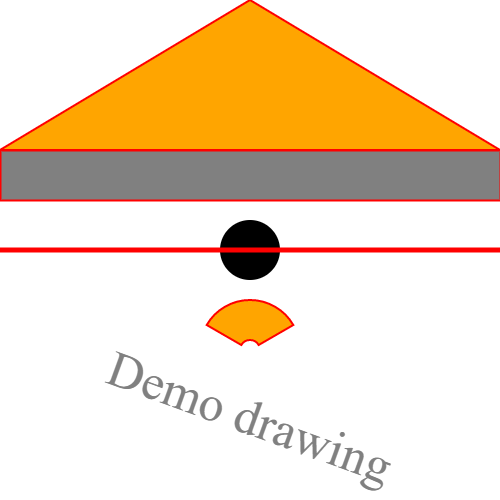
Additional Methods
Here is a list of additional methods that are also part of the Draw API:
-
attr - sets attributes of an element. The method has two parameters - an SVGElement and an object with applicable attributes.
const draw = new Smart.Utilities.Draw(document.getElementById('container')); const circle = draw.circle(250, 250, 30); draw.attr(circle, { 'fill': 'blue', 'stroke': 'green' });
-
clear - clears the elements drawn by this instance of Draw.
const draw = new Smart.Utilities.Draw(document.getElementById('container')); const circle = draw.circle(250, 250, 30); draw.clear();
-
getAttr - gets attribute of an element. The method has two parameters - an SVGElement and an applicable attribute.
const draw = new Smart.Utilities.Draw(document.getElementById('container')); const circle = draw.circle(250, 250, 30, { 'fill': 'blue', 'stroke': 'green' }); const circleFill = draw.getAttr(circle, 'fill'); // circleFill is "blue"
-
measureText - estimates the size of a hypothetical text element. The method has three parameters - text label, angle, and an object with applicable attributes (optional). The method returns an object with two fields - width and height.
const draw = new Smart.Utilities.Draw(document.getElementById('container')); const textSize = draw.measureText('My text', 0, { 'style': 'font-size: 50px;' }); // textSize is {"width":159,"height":34}
-
refresh - refreshes/re-draws the elements drawn by this instance of Draw.
const draw = new Smart.Utilities.Draw(document.getElementById('container')); const circle = draw.circle(250, 250, 30); draw.refresh();