Bind data to Blazor Smart.Scheduler
Setup The Blazor Application
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Scheduler appointments
Smart.Scheduler is designed to work with appointments. This tutorial will explain their properties and how to use them.
Appointment Properties
The scheduler appointments use the SchedulerDataSource
class that provide the following properties that you can use to control the data binding:
-
Id
- (string
) - Event unique id. -
Label
- (string
) - Event Label. -
Description
- (string
) - Event Description. -
Repeat
- (SchedulerDataSourceRepeat
) - Event Repeat Object. -
Class
- (string
) - Event CSS class. -
DateStart
- (object
) - Event start date. -
DateEnd
- (object
) - Event end date. -
DisableDrag
- (bool
) - Determines whether dragging is disabled for the event. -
DisableResize
- (bool
) - Determines whether resizing is disabled for the event. -
AllDay
- (bool
) - Determines whether an event is an all day event ot nor. All day events ignore time. -
BackgroundColor
- (string
) - Sets a background color for the event. The background color should be in HEX format. -
Color
- (string
) - Sets a color for the event. The color should be in HEX format. -
Status
- (string
) - Sets the appointment status. -
ResourceId
- (string
) - Event resource unique id. -
Notifications
- (IEnumerable<ISchedulerDataSourceNotification>
) - Event notifications.
SchedulerDataSource
and has additional properties.
You can find more information and details about the appointments on the Scheduler events docs page.
Here is an example of a list with 3 appointments:
List<SchedulerDataSource> data = new List<SchedulerDataSource>() { new SchedulerDataSource() { Label = "Brochure Design Review", DateStart = new DateTime(today.Year, today.Month, 10, 13, 15, 0), DateEnd = new DateTime(today.Year, today.Month, 12, 16, 15, 0), Class = "event" }, new SchedulerDataSource() { Label = "Website Re-Design Plan", DateStart = new DateTime(today.Year, today.Month, 16, 16, 45, 0), DateEnd = new DateTime(today.Year, today.Month, 18, 11, 15, 0), Class = "event" }, new SchedulerDataSource() { Label = "Non-Compete Agreements", DateStart = new DateTime(today.Year, today.Month, today.Day, 8, 15, 0).AddDays(-1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 9, 0, 0).AddDays(-1), Class = "event" } };
Scheduler Data Binding example
<Scheduler DataSource="dataRecords" View="SchedulerViewType.Week" /> @code { private List<SchedulerDataSource> dataRecords; protected override void OnInitialized() { base.OnInitialized(); dataRecords = GetData(); } private List<SchedulerDataSource> GetData() { DateTime today = DateTime.Today; List<SchedulerDataSource> data = new List<SchedulerDataSource>() { new SchedulerDataSource() { Label = "Brochure Design Review", DateStart = new DateTime(today.Year, today.Month, today.Day, 13, 15, 0).AddDays(2), DateEnd = new DateTime(today.Year, today.Month, today.Day, 16, 15, 0).AddDays(4), BackgroundColor = "#00C853" }, new SchedulerDataSource() { Label = "Website Re-Design Plan", DateStart = new DateTime(today.Year, today.Month, 16, 16, 45, 0), DateEnd = new DateTime(today.Year, today.Month, 20, 11, 15, 0), BackgroundColor = "#00ACC1" }, new SchedulerDataSource() { Label = "Rollout of New Website and Marketing Brochures", DateStart = new DateTime(today.Year, today.Month, 2, 8, 15, 0), DateEnd = new DateTime(today.Year, today.Month, 2, 10, 45, 0), BackgroundColor = "#FFA000" }, new SchedulerDataSource() { Label = "Update Sales Strategy Documents", DateStart = new DateTime(today.Year, today.Month, 2, 12, 0, 0), DateEnd = new DateTime(today.Year, today.Month, 2, 13, 45, 0), BackgroundColor = "#7986CB" }, new SchedulerDataSource() { Label = "Non-Compete Agreements", DateStart = new DateTime(today.Year, today.Month, today.Day, 8, 15, 0), DateEnd = new DateTime(today.Year, today.Month, today.Day, 9, 0, 0), BackgroundColor = "#9575CD" }, new SchedulerDataSource() { Label = "Approve Hiring of John Jeffers", DateStart = new DateTime(today.Year, today.Month, today.Day, 10, 0, 0), DateEnd = new DateTime(today.Year, today.Month, today.Day, 11, 15, 0), BackgroundColor = "#EF5350" }, new SchedulerDataSource() { Label = "Update NDA Agreement", DateStart = new DateTime(today.Year, today.Month, today.Day, 11, 45, 0), DateEnd = new DateTime(today.Year, today.Month, today.Day, 13, 45, 0), BackgroundColor = "#5D4037" }, new SchedulerDataSource() { Label = "Update Employee Files with New NDA", DateStart = new DateTime(today.Year, today.Month, today.Day, 14, 0, 0), DateEnd = new DateTime(today.Year, today.Month, today.Day, 16, 45, 0), BackgroundColor = "#E040FB" }, new SchedulerDataSource() { Label = "Compete Agreements", DateStart = new DateTime(today.Year, today.Month, today.Day, 8, 15, 0), DateEnd = new DateTime(today.Year, today.Month, today.Day, 9, 0, 0), BackgroundColor = "#FB8C00" }, new SchedulerDataSource() { Label = "Approve Hiring of Mark Waterberg", DateStart = new DateTime(today.Year, today.Month, today.Day, 10, 0, 0), DateEnd = new DateTime(today.Year, today.Month, today.Day, 11, 15, 0), BackgroundColor = "#BF360C" }, new SchedulerDataSource() { Label = "Update NDA Contracts", DateStart = new DateTime(today.Year, today.Month, today.Day, 11, 45, 0), DateEnd = new DateTime(today.Year, today.Month, today.Day, 13, 45, 0), BackgroundColor = "#00897B" }, new SchedulerDataSource() { Label = "Update Employees Information", DateStart = new DateTime(today.Year, today.Month, today.Day, 14, 0, 0), DateEnd = new DateTime(today.Year, today.Month, today.Day, 16, 45, 0), BackgroundColor = "#9575CD" }, new SchedulerDataSource() { Label = "Customer Feedback Report Analysis", DateStart = new DateTime(today.Year, today.Month, today.Day, 9, 30, 0).AddDays(1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 10, 30, 0).AddDays(1), BackgroundColor = "#9C27B0" }, new SchedulerDataSource() { Label = "Prepare Shipping Cost Analysis Report", DateStart = new DateTime(today.Year, today.Month, today.Day, 12, 30, 0).AddDays(1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 13, 30, 0).AddDays(1), BackgroundColor = "#00C853" }, new SchedulerDataSource() { Label = "Provide Feedback on Shippers", DateStart = new DateTime(today.Year, today.Month, today.Day, 14, 15, 0).AddDays(1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 16, 0, 0).AddDays(1), BackgroundColor = "#FFA726" }, new SchedulerDataSource() { Label = "Select Preferred Shipper", DateStart = new DateTime(today.Year, today.Month, today.Day, 17, 30, 0).AddDays(1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 20, 0, 0).AddDays(1), BackgroundColor = "#9575CD" }, new SchedulerDataSource() { Label = "Complete Shipper Selection Form", DateStart = new DateTime(today.Year, today.Month, today.Day, 8, 30, 0).AddDays(1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 10, 0, 0).AddDays(1), BackgroundColor = "#00B8D4" }, new SchedulerDataSource() { Label = "Upgrade Server Hardware", DateStart = new DateTime(today.Year, today.Month, today.Day, 12, 0, 0).AddDays(1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 14, 15, 0).AddDays(1), BackgroundColor = "#0097A7" }, new SchedulerDataSource() { Label = "Upgrade Personal Computers", DateStart = new DateTime(today.Year, today.Month, today.Day, 14, 45, 0).AddDays(1), DateEnd = new DateTime(today.Year, today.Month, today.Day, 16, 30, 0).AddDays(1), BackgroundColor = "#9575CD" }, new SchedulerDataSource() { Label = "Upgrade Apps to Windows RT or stay with WinForms", DateStart = new DateTime(today.Year, today.Month, today.Day, 10, 30, 0).AddDays(2), DateEnd = new DateTime(today.Year, today.Month, today.Day, 13, 0, 0).AddDays(2), BackgroundColor = "#00ACC1" }, new SchedulerDataSource() { Label = "Estimate Time Required to Touch-Enable Apps", DateStart = new DateTime(today.Year, today.Month, today.Day, 14, 45, 0).AddDays(2), DateEnd = new DateTime(today.Year, today.Month, today.Day, 16, 30, 0).AddDays(2), BackgroundColor = "#EF6C00" }, new SchedulerDataSource() { Label = "Report on Tranistion to Touch-Based Apps", DateStart = new DateTime(today.Year, today.Month, today.Day, 18, 30, 0).AddDays(2), DateEnd = new DateTime(today.Year, today.Month, today.Day, 19, 0, 0).AddDays(2), BackgroundColor = "#3F51B5" }, new SchedulerDataSource() { Label = "Submit New Website Design", DateStart = new DateTime(today.Year, today.Month, today.Day, 8, 0, 0).AddDays(5), DateEnd = new DateTime(today.Year, today.Month, today.Day, 10, 0, 0).AddDays(5), BackgroundColor = "#E57373" }, new SchedulerDataSource() { Label = "Create Icons for Website", DateStart = new DateTime(today.Year, today.Month, today.Day, 11, 30, 0).AddDays(5), DateEnd = new DateTime(today.Year, today.Month, today.Day, 13, 15, 0).AddDays(5), BackgroundColor = "#C51162" } }; return data; } }Result:
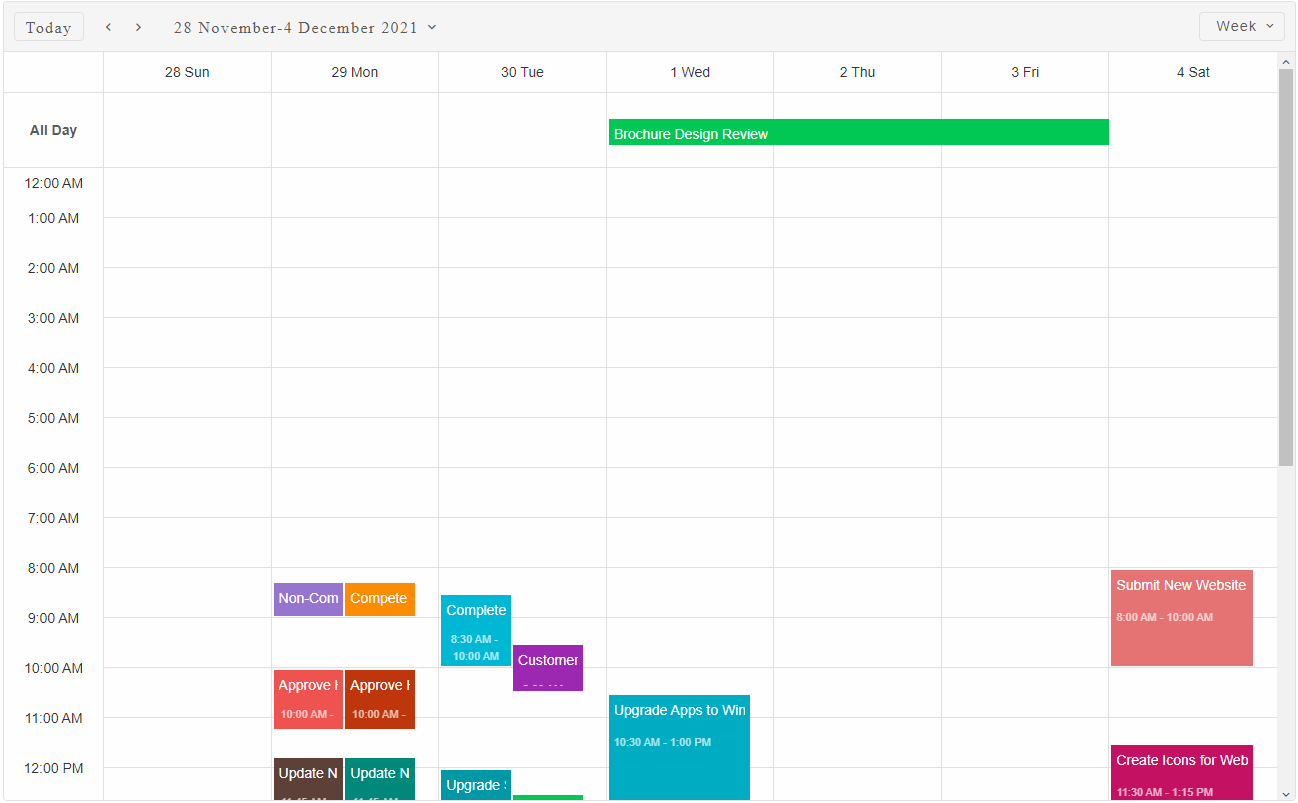