Smart.Kanban Nested Columns
Setup The Blazor Application
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup the Blazor Smart.Kanban
Follow the Get Started with Smart.Kanban guide to set up the component.
Nested Columns
Smart.Kanban supports nested columns so that complex layouts that best suit user projects can be created.
By adding the Columns to any column definition, nested columns can be visualized on the kanban board. They represent more specific statuses for tasks.
Here is an example:
@using Smart.Blazor.Demos.Data @inject RandomDataService dataService <Kanban DataSource="dataRecords" Columns="@columns" Collapsible /> @code { List<KanbanColumn> columns = new List<KanbanColumn>() { new KanbanColumn() { DataField = "ToDo", Label = "To do" }, new KanbanColumn() { DataField = "InProgress", Label = "In progress" }, new KanbanColumn() { DataField = "Testing", Label = "Testing", Orientation = KanbanColumnOrientation.Horizontal, Columns = new List<KanbanColumn>() { new KanbanColumn() { Label = "Manual testing", DataField = "ManualTesting", Columns = new List<KanbanColumn>() { new KanbanColumn() { Label = "Desktop devices", DataField = "Desktop" }, new KanbanColumn() { Label = "Mobile devices", DataField = "Mobile" } } }, new KanbanColumn() { Label = "Unit testing", DataField = "UnitTesting" } } }, new KanbanColumn() { DataField = "Done", Label = "Done" } }; private List<KanbanDataRecord> dataRecords; protected override void OnInitialized() { base.OnInitialized(); dataRecords = dataService.GenerateKanbanHierarchicalData(); } }Result:
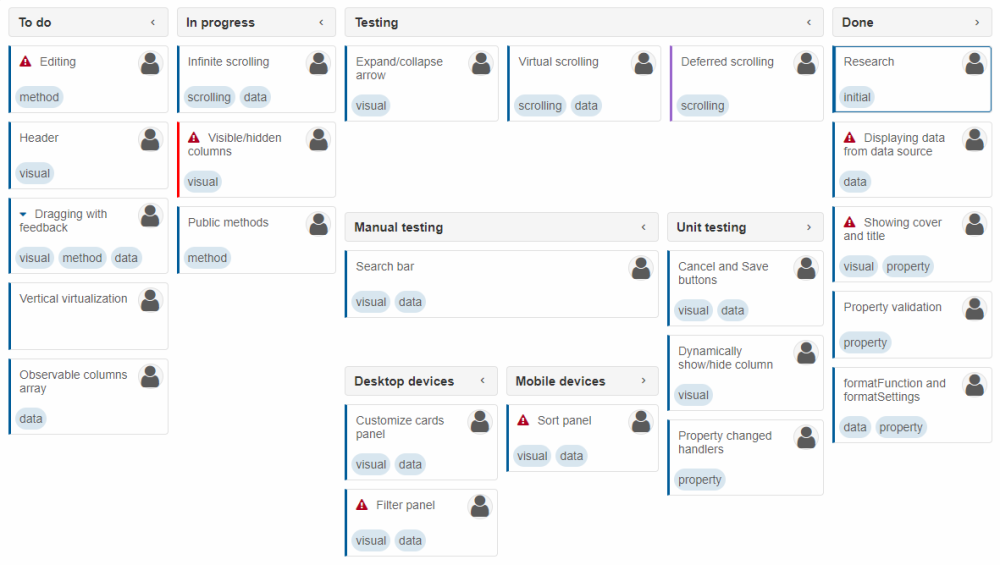
This code creates two sub-columns for "Testing" - "Manual testing" and "Unit testing". Moreover, "Manual testing" has two sub-columns of its own - "Desktop devices" and "Mobile devices".
In some cases, it may be necessary for "parent" columns to only act as headers and not have tasks of their own.
In that case, the property <>TaskPosition strong>, which is "All" by default, can be set to "Leaf" to allow tasks only in columns that do not have sub-columns of their own (leaves):
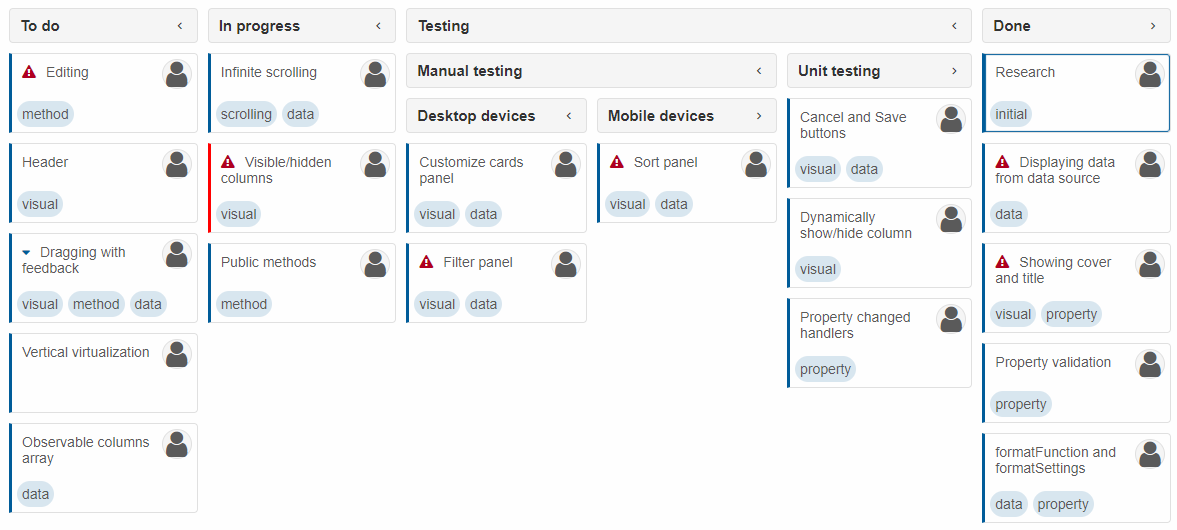
Nested Tabs
By default, status hierarchy is represented by nested columns. This behavior is controlled by the property Hierarchy (by default its value is "KanbanHierarchy.Columns
").
If Hierarchy is set to "KanbanHierarchy.Tabs
", the nested columns are replaced with tab views instead:
<Kanban DataSource="dataRecords" Columns="@columns" Collapsible Hierarchy="KanbanHierarchy.Tabs"/>
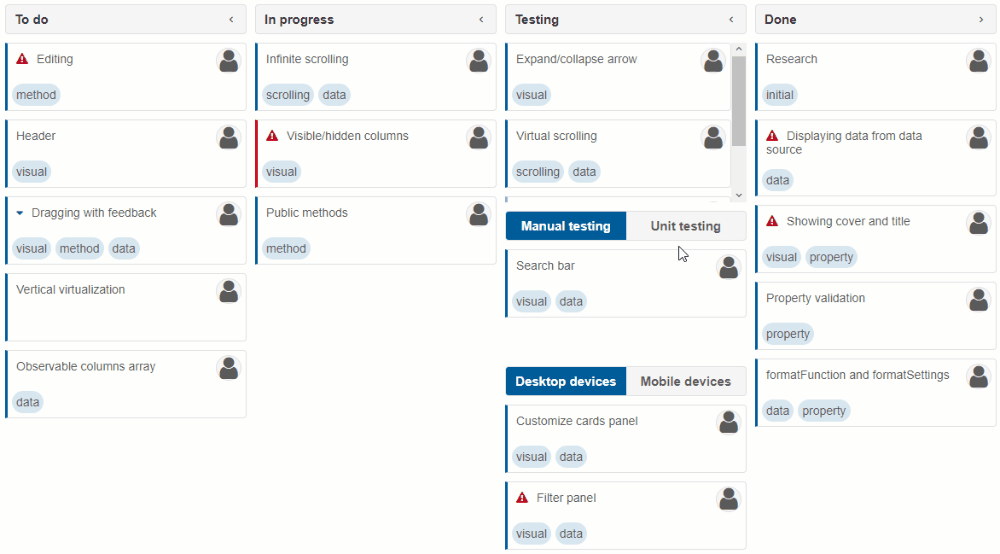