Command Column
The Smart.Grid built-in Command Column
can be used to Edit, Delete or perform any other custom function on a row.
To create a command column, create a new instance of GridEditing()
object
and set the Visible property of the GridCommandColumn
Finally, attach the editing object to the Grid:
@inject WeatherForecastService ForecastService <Grid Editing="@editing"> <Columns> <Column DataField="Date" Label="Date"> </Column> <Column DataField="TemperatureC" Label="Temp. (C)"> </Column> <Column DataField="TemperatureF" Label="Temp. (F)"> </Column> <Column DataField="Summary" Label="Summary"> </Column> </Columns> </Grid> @code{ GridEditing editing = new GridEditing() { Enabled = true, Action = GridEditingAction.None, Mode = GridEditingMode.Row, CommandColumn = new GridEditingCommandColumn() { Visible = true } }; private WeatherForecast[] forecast; protected override async Task OnInitializedAsync() { forecast = await ForecastService.GetForecastAsync(DateTime.Now); } }
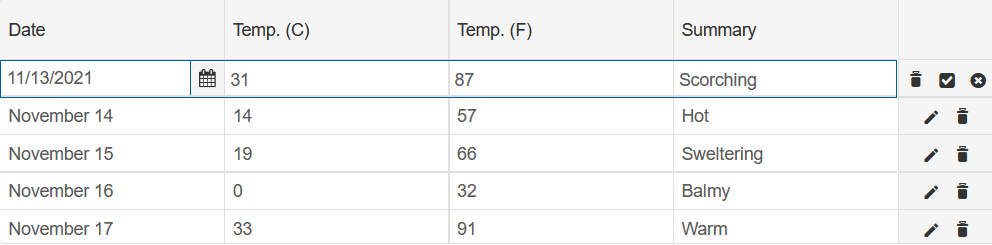
Command Column Inline
The Command Column can be displayed inline, meaning that it only appears when the row is hovered.
GridEditing editing = new GridEditing() { Enabled = true, Action = GridEditingAction.None, Mode = GridEditingMode.Row, CommandColumn = new GridEditingCommandColumn() { Visible = true, Inline = true} };
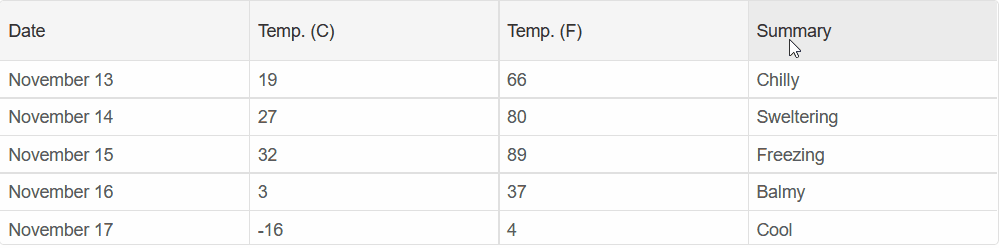
Command Column Position
It is possible to change the position of the command column by using the Position
property.
GridEditing editing = new GridEditing() { Enabled = true, Action = GridEditingAction.None, Mode = GridEditingMode.Row, CommandColumn = new GridEditingCommandColumn() { Visible = true, Position = Position.Near} };
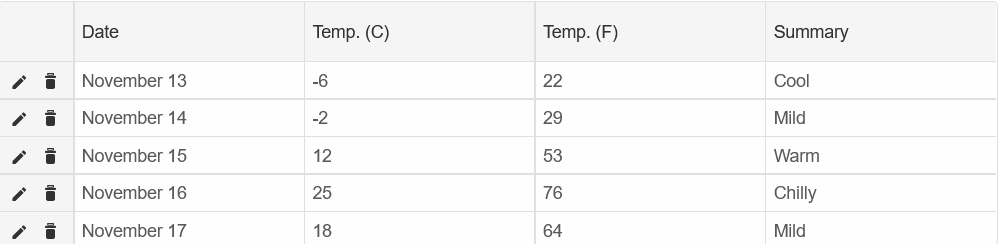
Custom Command Column
You can also create a custom command column by using the DataSource
property.
In this example, we hide the Delete Column and create a new custom one:
GridEditing editing = new GridEditing() { Enabled = true, Action = GridEditingAction.None, Mode = GridEditingMode.Row, CommandColumn = new GridEditingCommandColumn() { Visible = true, Inline = false, DataSource = new GridEditingCommandColumnDataSource() { CommandColumnDelete = new GridCommand() { Visible = false }, CommandColumnCustom = new GridCommand() { Icon = "smart-icon-star", Command = "notify", Visible = true, Label = "Notify Me" } } } };
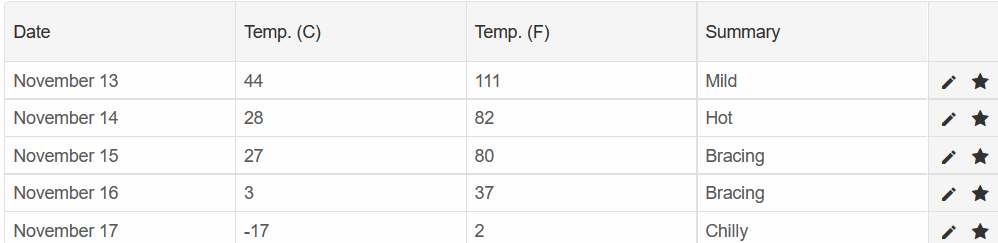