Blazor - Get Started with Smart.GanttChart
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic GanttChart
Smart.GanttChart represents a bar chart that illustrates a project schedule with dependency links between each individual task and their current status.
- Add the GanttChart component to the Pages/Index.razor file
<GanttChart></GanttChart>
- Inside the
@code
block, declare theGanttChartTaskColumns
and set them as properties of the GanttChart component.List<GanttChartTaskColumn> taskColumns = new List<GanttChartTaskColumn>{ new GanttChartTaskColumn() { Label = "Tasks", Value = "label" }, new GanttChartTaskColumn() { Label = "Duration (hours)", Value = "duration" } };
- Declare the properties of the
GanttDataRecord
object.
Then create an example Task data and bind it to the GanttChart using theDataSource
property of the Component.<PivotTable DataSource="Tasks" TaskColumns="taskColumns"></PivotTable> @code{ ... public partial class GanttDataRecord { [JsonPropertyName("id")] public string Id {get; set;} [JsonPropertyName("label")] public string Label {get; set;} [JsonPropertyName("dateStart")] public string DateStart {get; set;} [JsonPropertyName("dateEnd")] public string DateEnd {get; set;} [JsonPropertyName("class")] public string Class {get; set;} [JsonPropertyName("type")] public string Type {get; set;} [JsonPropertyName("progress")] public int Progress {get; set;} [JsonPropertyName("duration")] public int Duration {get; set;} [JsonPropertyName("synchronized")] public bool Synchronized {get; set;} [JsonPropertyName("expanded")] public bool Expanded {get; set;} [JsonPropertyName("tasks")] public List<GanttDataRecord> Tasks {get; set;} [JsonPropertyName("disableResources")] public bool DisableResources {get; set;} [JsonPropertyName("resources")] public object Resources {get; set;} = new object[] { }; [JsonPropertyName("connections")] public List<Dictionary<string, int>> Connections {get; set;} = new List<Dictionary<string, int>>() {}; [JsonPropertyName("disableDrag")] public bool DisableDrag {get; set;} [JsonPropertyName("disableResize")] public bool DisableResize {get; set;} [JsonPropertyName("minDateStart")] public string MinDateStart {get; set;} [JsonPropertyName("maxDateStart")] public string MaxDateStart {get; set;} [JsonPropertyName("minDateEnd")] public string MinDateEnd {get; set;} [JsonPropertyName("maxDateEnd")] public string MaxDateEnd {get; set;} [JsonPropertyName("minDuration")] public int MinDuration {get; set;} [JsonPropertyName("maxDuration")] public int MaxDuration {get; set;} [JsonPropertyName("value")] public int Value {get; set;} [JsonPropertyName("dragProject")] public bool DragProject {get; set;} } List<GanttDataRecord> Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Develop Website", DateStart = "2020-03-10", DateEnd = "2020-08-22", Expanded = true, Type = "project" }, new GanttDataRecord() { Label = "Hire Employees", DateStart = "2020-4-05", DateEnd = "2021-04-10", Type = "task" }, new GanttDataRecord() { Label = "Market Website", DateStart = "2020-05-10", DateEnd = "2021-03-06", Type = "project" }, new GanttDataRecord() { Label = "Manafucture Products", DateStart = "2020-03-10", DateEnd = "2021-08-12" } }; }
Note: Smart.Gantt natively supports Dragging and Resizing functionalities without the need to write any code.
Nested Projects & Tasks
Smart.Gantt allows you to form project hierarchy by nesting tasks into each other.
Modify the "Develop Website" Project by adding additional Tasks, Milestones and Projects in the Tasks
property.
List<GanttDataRecord> Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Develop Website", DateStart = "2020-03-10", DateEnd = "2020-08-22", Expanded = true, Type = "project", Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Create HTML Structure", DateStart = "2020-03-10", DateEnd = "2020-05-20", Type = "task" }, new GanttDataRecord() { Label = "Style Using CSS", DateStart = "2020-03-20", DateEnd = "2020-06-10", Type = "task" }, new GanttDataRecord() { Label = "Website Structure is complete", DateStart = "2020-06-11", Type = "milestone" }, new GanttDataRecord() { Label = "Add Products to Website", DateStart = "2020-06-11", Duration=200, Expanded = true, Type = "project", Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Take Photos of Products", DateStart = "2020-06-12", DateEnd = "2020-08-20", Type = "task" }, new GanttDataRecord() { Label = "Write Description of Each Products", DateStart = "2020-05-02", DateEnd = "2020-12-01", Type = "task" }, new GanttDataRecord() { Label = "Add Product List to Main Page", DateStart = "2020-06-30", DateEnd = "2020-11-15", Type = "task" } } } } }, .... } ....
Task Connections
It is possible indicate the relation between tasks by using the Connection property.
Modify the "Develop Website" Project by connecting related tasks in the Connections
property.
.... new GanttDataRecord() { Label = "Develop Website", DateStart = "2020-03-10", DateEnd = "2020-08-22", Expanded = true, Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 1 }, { "type", 0 } }, new Dictionary<string, int>() { { "target", 4 }, { "type", 0 } }, new Dictionary<string, int>() { { "target", 9}, { "type", 0 } } }, Type = "project", Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Create HTML Structure", DateStart = "2020-03-10", DateEnd = "2020-05-20", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 2 }, { "type", 2 } } } }, new GanttDataRecord() { Label = "Style Using CSS", DateStart = "2020-03-20", DateEnd = "2020-06-10", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 3 }, { "type", 0 } } } }, new GanttDataRecord() { Label = "Website Structure is complete", DateStart = "2020-06-11", Type = "milestone" }, new GanttDataRecord() { Label = "Add Products to Website", DateStart = "2020-06-11", Duration=200, Expanded = true, Type = "project", Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Take Photos of Products", DateStart = "2020-06-12", DateEnd = "2020-08-20", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 6 }, { "type", 2 } } }, }, new GanttDataRecord() { Label = "Write Description of Each Products", DateStart = "2020-05-02", DateEnd = "2020-12-01", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 7 }, { "type", 2 } } }, }, new GanttDataRecord() { Label = "Add Product List to Main Page", DateStart = "2020-06-30", DateEnd = "2020-11-15", Type = "task" } } } } }, ....
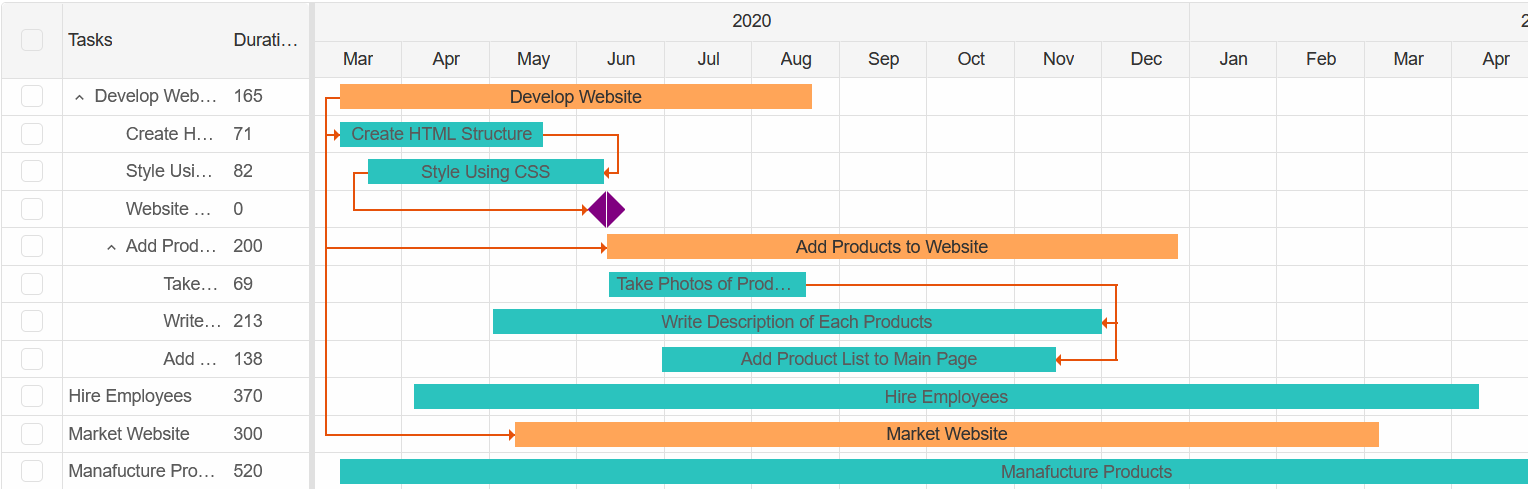
Task Progress
The Progress property allows you to set a progress value between 0 and 100.
Modify the "Hire Employees" Task by setting the progress value in the Progress
porperty.
.... new GanttDataRecord() { Label = "Hire Employees", DateStart = "2020-4-05", DateEnd = "2021-04-10", Type = "task", Progress=60 }, ....
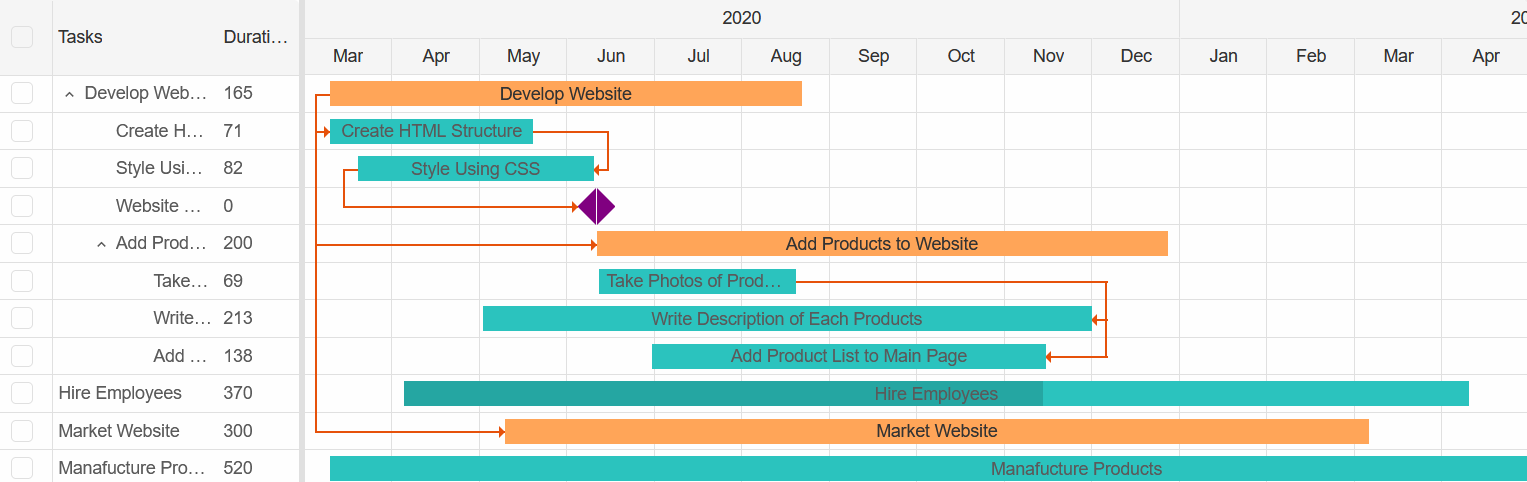
Non-Working Hours & Days
Additionaly, it is also possible to set non-working days or hours.
If the AdjustToNonworkingTime
property is set to true,
Smart.Gantt will automatically adjust the duration of the tasks according to the new rules.
<GanttChart DataSource="Tasks" TaskColumns="taskColumns" DurationUnit="Duration.Day" NonworkingHours="nonworkingHours" AdjustToNonworkingTime="true"> </GanttChart> @code{ private List<object> nonworkingHours = new List<object>() { 19,20,21,22,23,0,1,2,3,4,5,6}; .... }
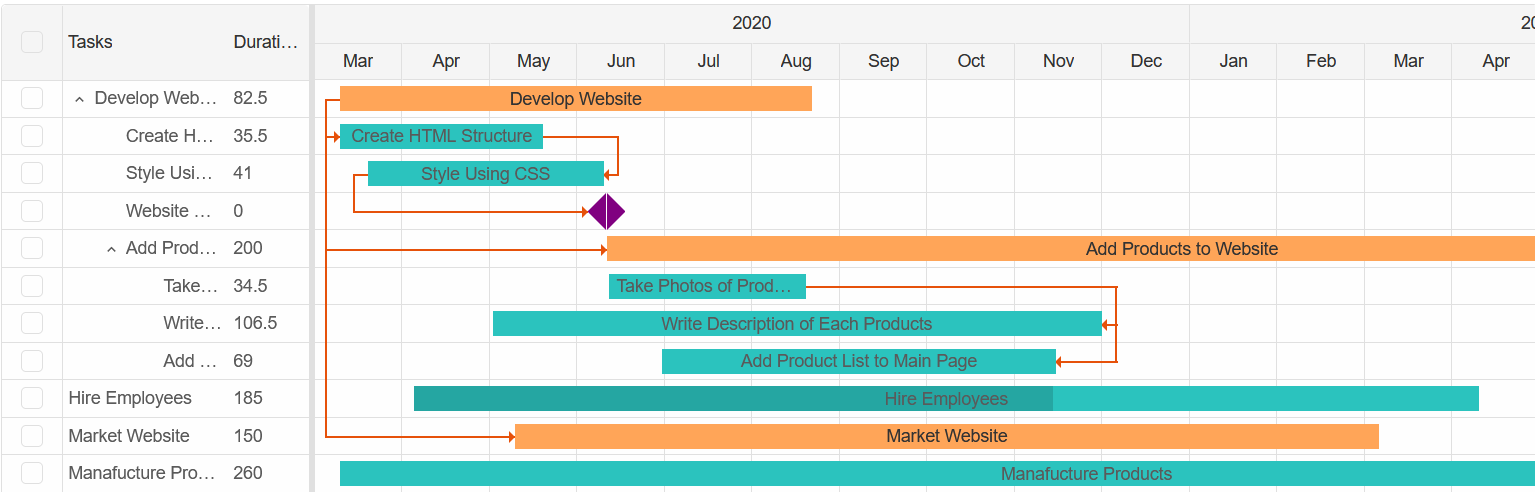
Task Duration
If you are unsure of the EndDate of a Task, it is also possible to set the Duration value.
The value will use the units as set in the DurationUnit property of the Component.
Modify the Market Website Task by replacing the EndDate
property with Duration
value.
.... new GanttDataRecord() { Label = "Market Website", DateStart = "2020-05-10", Duration = 200, Type = "project" }, ....
Task Resources
It is possible to pass any other additional values to the Gantt Chart. They can be used to provied more information to the user,
as well as for Sorting & Filtering the Gantt Chart.
Create a new Employee TaskColumn. Then modify the Tasks by including Resources with employee names.
The Resource View Panel is enabled by default.
List<GanttChartTaskColumn> taskColumns = new List<GanttChartTaskColumn>{ new GanttChartTaskColumn() { Label = "Tasks", Value = "label", Size = "50%" }, new GanttChartTaskColumn() { Label = "Employee", Value = "resources" }, new GanttChartTaskColumn() { Label = "Duration (days)", Value = "duration" } }; .... List<GanttDataRecord> Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Develop Website", DateStart = "2020-03-10", DateEnd = "2020-08-22", DisableResources = true, Expanded = true, Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 1 }, { "type", 0 } }, new Dictionary<string, int>() { { "target", 4 }, { "type", 0 } }, new Dictionary<string, int>() { { "target", 9}, { "type", 0 } } }, Type = "project", Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Create HTML Structure", DateStart = "2020-03-10", DateEnd = "2020-05-20", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 2 }, { "type", 2 } } }, Resources = new List<Dictionary<string, object>>() { new Dictionary<string, object>() { { "id", "taylor" }, { "label", "Taylor" } } } }, new GanttDataRecord() { Label = "Style Using CSS", DateStart = "2020-03-20", DateEnd = "2020-06-10", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 3 }, { "type", 0 } } }, Resources = new List<Dictionary<string, object>>() { new Dictionary<string, object>() { { "id", "taylor" }, { "label", "Taylor" } } } }, new GanttDataRecord() { Label = "Website Structure is complete", DateStart = "2020-06-11", Type = "milestone" }, new GanttDataRecord() { Label = "Add Products to Website", DateStart = "2020-06-11", Duration=200, Expanded = true, Type = "project", Tasks = new List<GanttDataRecord>() { new GanttDataRecord() { Label = "Take Photos of Products", DateStart = "2020-06-12", DateEnd = "2020-08-20", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 6 }, { "type", 2 } } }, Resources = new List<Dictionary<string, object>>() { new Dictionary<string, object>() { { "id", "john" }, { "label", "John" } } } }, new GanttDataRecord() { Label = "Write Description of Each Products", DateStart = "2020-05-02", DateEnd = "2020-12-01", Type = "task", Connections = new List<Dictionary<string, int>>() { new Dictionary<string, int>() { { "target", 7 }, { "type", 2 } } }, }, new GanttDataRecord() { Label = "Add Product List to Main Page", DateStart = "2020-06-30", DateEnd = "2020-11-15", Type = "task" } } } } }, new GanttDataRecord() { Label = "Hire Employees", DateStart = "2020-4-05", DateEnd = "2021-04-10", Type = "task", Progress=60, Resources = new List<Dictionary<string, object>>() { new Dictionary<string, object>() { { "id", "kevin" }, { "label", "Kevin" } } } }, new GanttDataRecord() { Label = "Market Website", DateStart = "2020-05-10", DateEnd = "2021-03-06", Type = "project", Resources = new List<object>() { new Dictionary<string, object>() { { "id", "jenna" }, { "label", "Jenna" } } } }, new GanttDataRecord() { Label = "Manafucture Products", DateStart = "2020-03-10", DateEnd = "2021-08-12" } }; ....
Column names inside the Resource View Panel can be customized by setting the ResourceColumns property
<GanttChart DataSource="Tasks" TaskColumns="taskColumns" DurationUnit="Duration.Day" NonworkingHours="nonworkingHours" AdjustToNonworkingTime="true" ResourceColumns="resourceColumns"> </GanttChart> @code{ List<GanttChartResourceColumn> resourceColumns = new List<GanttChartResourceColumn>() { new GanttChartResourceColumn() { Label = "Employees", Value = "label" } }; .... }
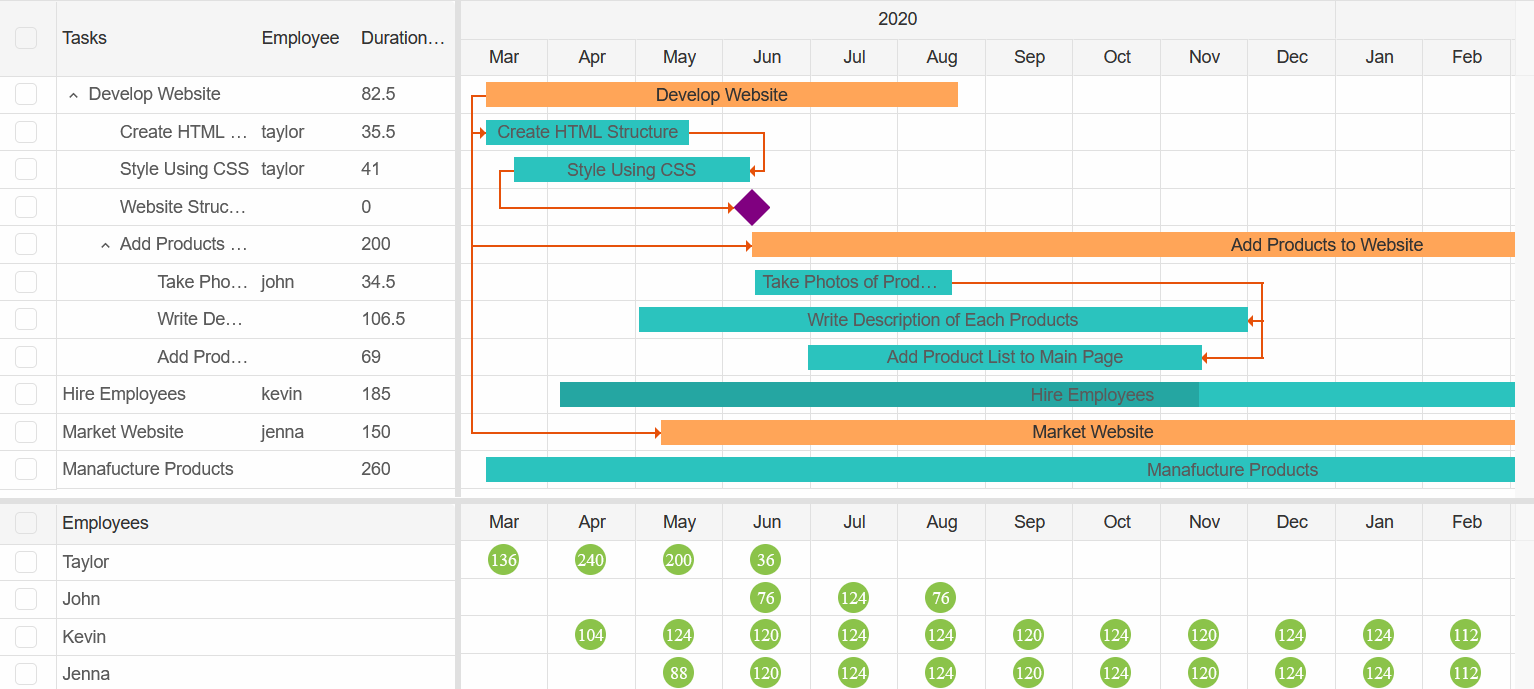
Sort Tasks
Tasks can be sorted using the SortMode
property.
<GanttChart DataSource="Tasks" TaskColumns="taskColumns" DurationUnit="Duration.Day" NonworkingHours="nonworkingHours" AdjustToNonworkingTime="true" ResourceColumns="resourceColumns" SortMode="GanttChartSortMode.Many"> </GanttChart>
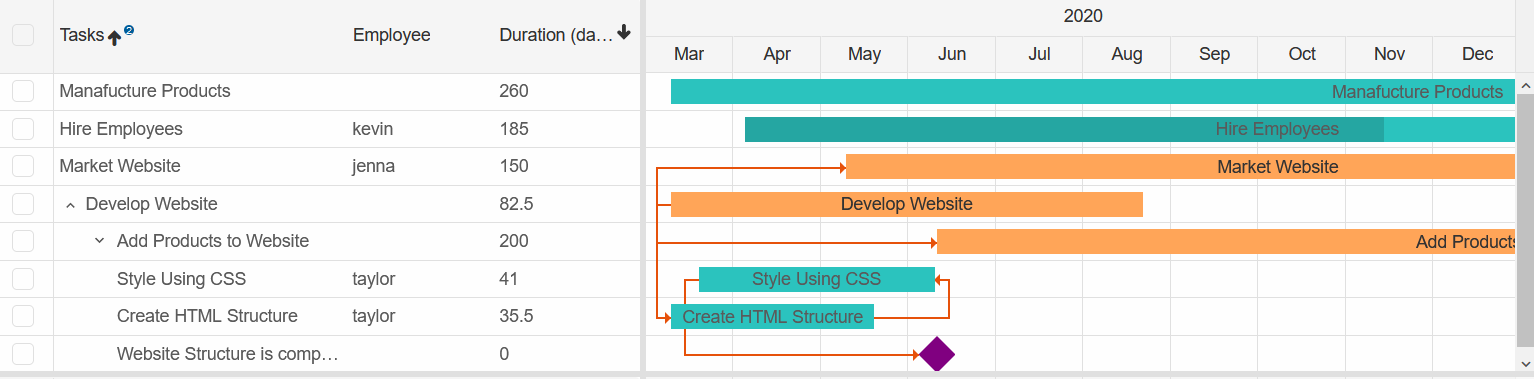
Filter Tasks
To enable Task Filtering, set the TaskFiltering
property to true.
<GanttChart DataSource="Tasks" TaskColumns="taskColumns" DurationUnit="Duration.Day" NonworkingHours="nonworkingHours" AdjustToNonworkingTime="true" ResourceColumns="resourceColumns" SortMode="GanttChartSortMode.Many" TaskFiltering="true"> </GanttChart>

By enabling the FilterRow
property, you can use the advanced built-in filtering options
<GanttChart DataSource="Tasks" TaskColumns="taskColumns" DurationUnit="Duration.Day" NonworkingHours="nonworkingHours" AdjustToNonworkingTime="true" ResourceColumns="resourceColumns" SortMode="GanttChartSortMode.Many" TaskFiltering="true" FilterRow="true"> </GanttChart>
