Blazor - Get Started with Smart.MultiInput
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic Multi Input
Smart.MultiInput is an input that allows selecting from a predefined drop down list.
The list of avaible options is set using the DataSource property.
- Add the MultiInput component to the Pages/Index.razor file
<MultiInput></MultiInput>
- Inside the
@code
block, create an array of elements and set it as DataSource of the component<MultiInput DataSource="drinks"></MultiInput> @code{ private string[] drinks = new string[]{"Water", "Juice", "Soda", "Tea", "Beer", "Wine", }; }
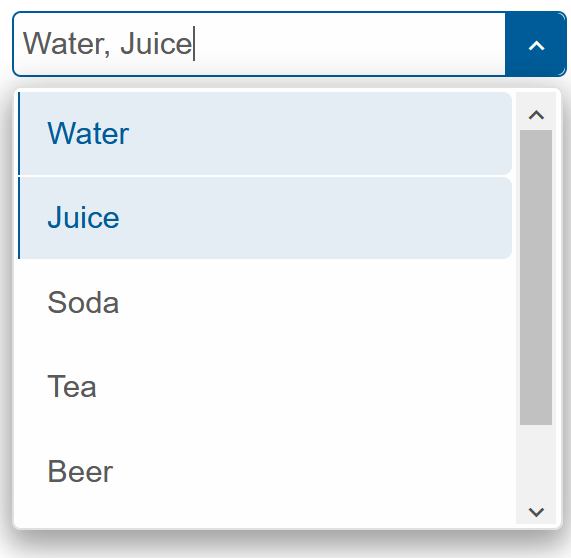
Readonly
When the Readonly property is set to true, the input field will be disabled and changes will be allowed only by using the dropdown list.
<MultiInput DataSource="drinks" Readonly="true"></MultiInput>

MultiInput Methods
Smart.MultiInput can be easily controlled programmatically using some of the built-in Methods.
Create buttons that can control the Open/Close state of the dropdown list:
<Button OnClick="Open">Open</Button> <Button OnClick="Close">Close</Button> <MultiInput @ref="multiInput" DataSource="drinks"></MultiInput> @code{ MultiInput multiInput; private string[] drinks = new string[]{"Water", "Juice", "Soda", "Tea", "Beer", "Wine", }; private void Open(){ multiInput.Open(); } private void Close(){ multiInput.Close(); } }
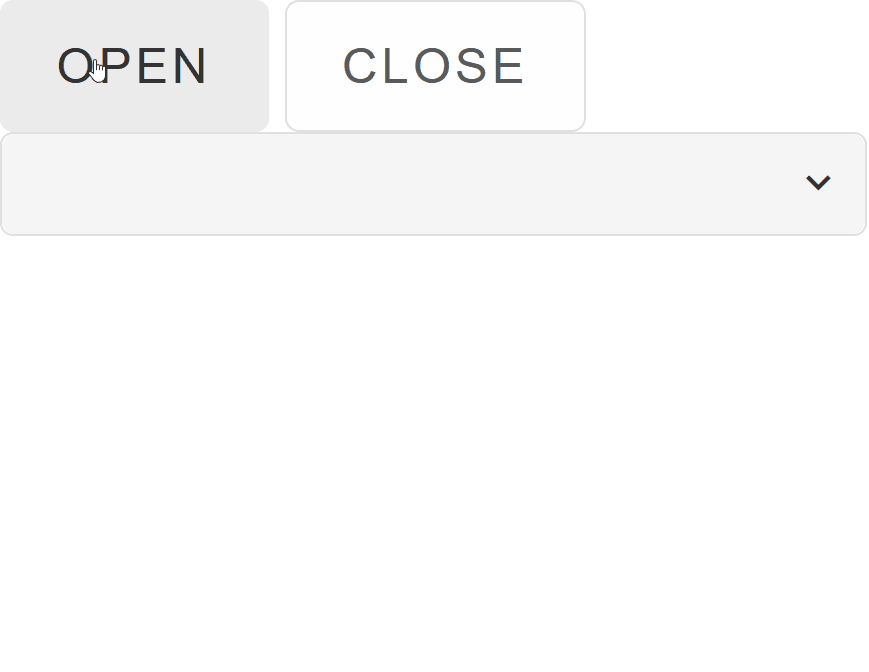
MultiInput Customization
Smart.MultiInput can be styled in different ways by setting the Class property.
The MultiInput can be default
, outlined
or underlined
<MultiInput DataSource="drinks" Class="underlined"></MultiInput>
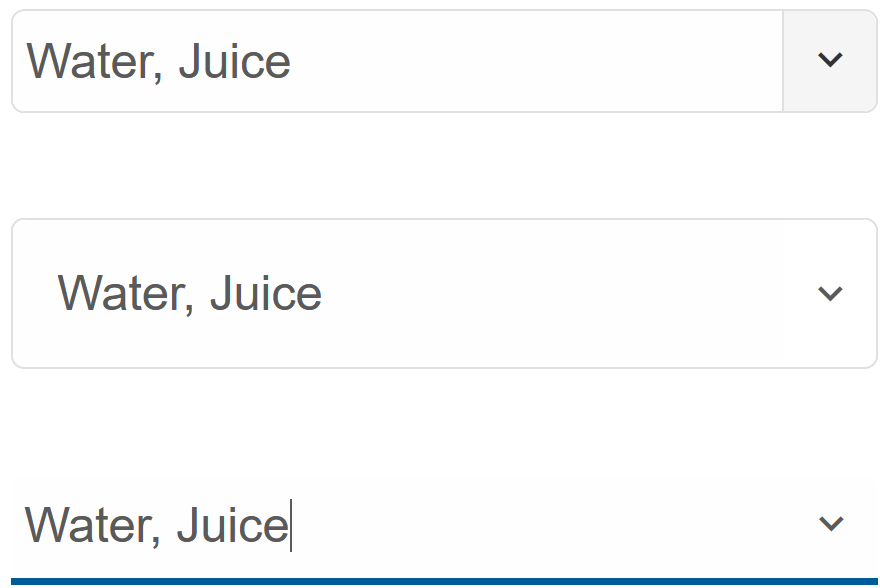
MultiInput Events
Smart.MultiInput provides an OnChange Event that can help you expand the component's functionality.
The event object has unique event.detail parameters.
OnChange
- triggered when the selection is changed.
Event Details
: string label, dynamic oldLabel, dynamic oldValue, dynamic value
The demo below uses the OnChange Event to display the selected options:
<h2>Selected values: @values</h2> <MultiInput DataSource="drinks" OnChange="OnChange"></MultiInput> @code{ private string[] drinks = new string[]{"Water", "Juice", "Soda", "Tea", "Beer", "Wine", }; string values; private void OnChange(Event ev){ if(ev.ContainsKey("Detail")){ MultiInputChangeEventDetail detail = ev["Detail"]; values = detail.Value; } } }
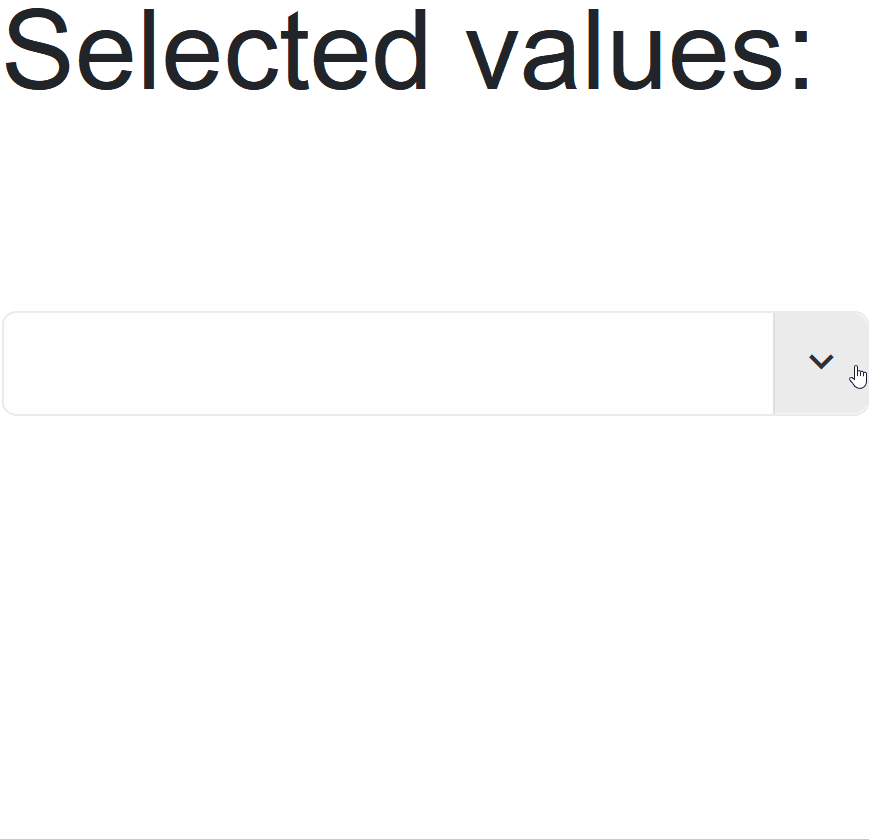
Two-way Value Binding
The MultiInput component also supports two-way value binding:
<h3>@textValue</h3> <MultiInput DataSource="drinks" @bind-Value = "@textValue"></MultiInput> @code{ private string[] drinks = new string[]{"Water", "Juice", "Soda", "Tea", "Beer", "Wine" }; string textValue = ""; }
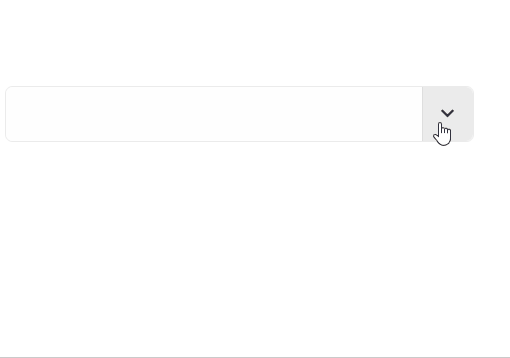