Blazor - Get Started with Smart.Window
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic Window
Smart.Window is a custom component that can be used to provide additional information, ask for confirmation or show the progress of a task.
-
Add the Window component to the Pages/Index.razor file:
<Window></Window>
-
Set the
IsOpened
property totrue
:<Window IsOpened="true" Label="Basic Window"></Window>
-
Optionally, create a button that can control the state of the Window:
<Button id="openButton" OnClick="OnClick">Open/Close </Button> <Window IsOpened="@opened" label="Window 1"></Window> @code { private bool opened = true; private void OnClick() { opened = !opened; } }
-
Add the content of the Window. The component accepts any valid HTML:
<Window IsOpened="@opened" label="Window 1"> <div id="article"> <section> <h3>What is Lorem Ipsum?</h3> <p> Lorem Ipsum is simply dummy text of the printing and typesetting industry. Lorem Ipsum has been the industry's standard dummy text ever since the 1500s, when an unknown printer took a galley of type and scrambled it to make a type specimen book. It has survived not only five centuries, but also the leap into electronic typesetting, remaining essentially unchanged. It was popularised in the 1960s with the release of Letraset sheets containing Lorem Ipsum passages, and more recently with desktop publishing software like Aldus PageMaker including versions of Lorem Ipsum. </p> <img src="https://wallpapercave.com/wp/wp2754864.jpg"> </section> </div> </Window>
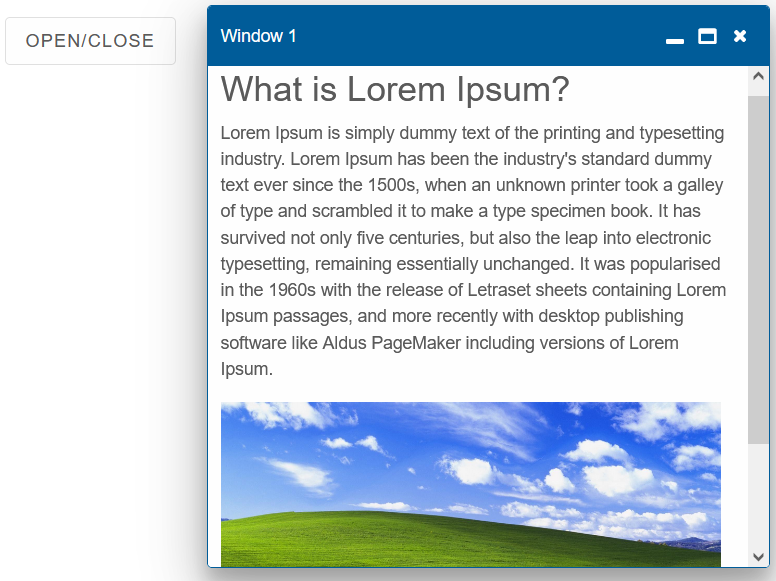
By default, the Window can be dragged with the mouse. When the Window reaches the end of the container, it will snap in the same direction:
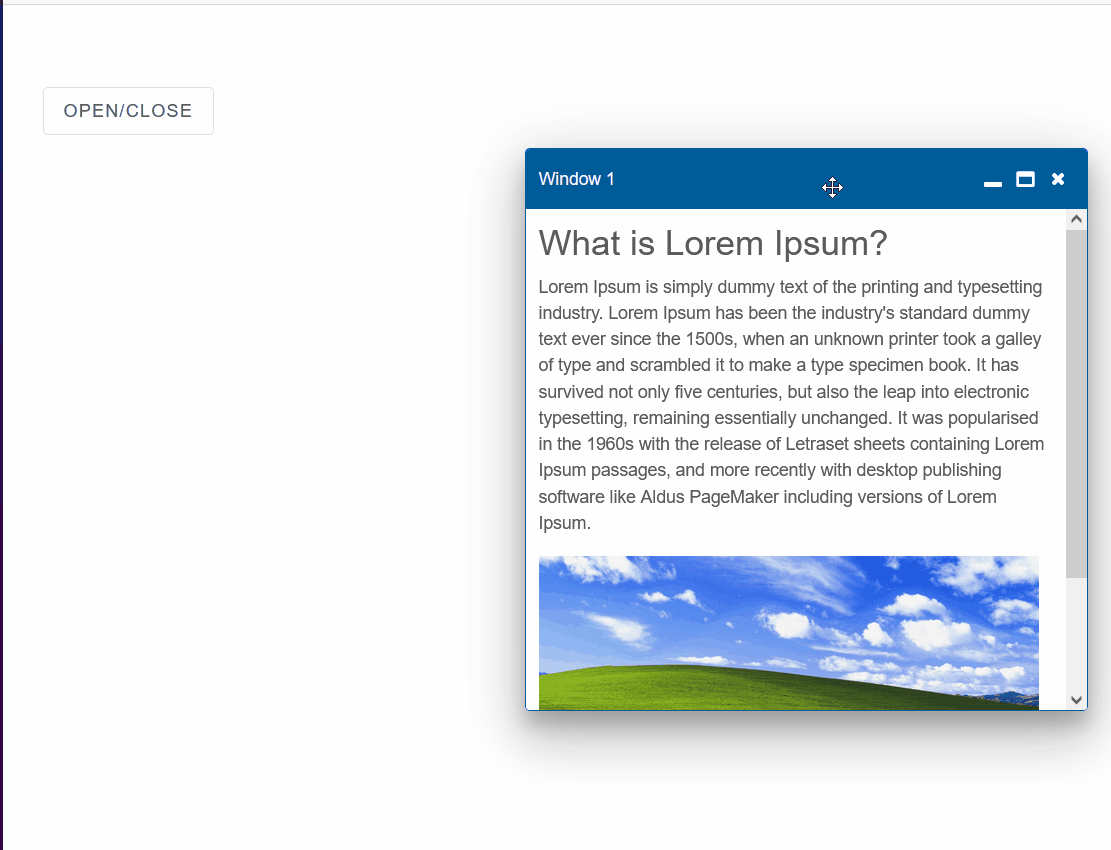
Resizing
The Window Resize can be set to multiple different modes. Allow both Vertical & Horizontal by setting the ResizeMode to WindowResizeMode.Both
<Window ResizeMode="WindowResizeMode.Both" LiveResize="true">....</Window>
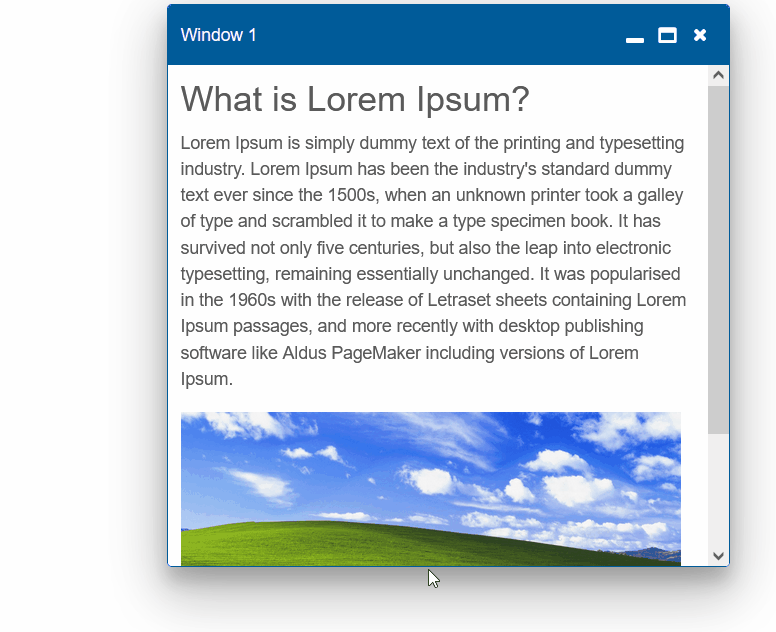
Window Header
Smart.Window allows you to modify the buttons set in the header using the HeaderButtons
property.
It is aslo possible to change the Header's position in the Window:
<template id="someTemplate"><div style="background-color:red">{{value}}</div></template> <Window HeaderButtons="@headerButtons" HeaderPosition="TabPosition.Bottom">...</Window> @code{ private bool opened = true; private string[] headerButtons = new string[]{"close", "pin", "maximize", "minimize"}; private void OnClick() { opened = !opened; } }
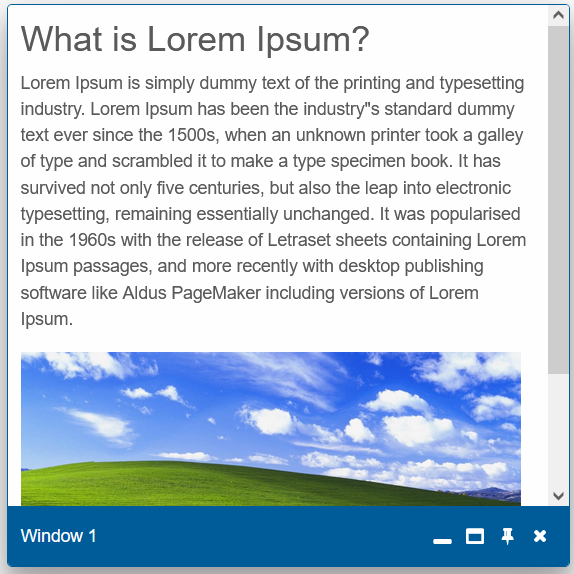
Window Templates
Smart.Window allows you to create custom template for the Header & Footer content.
Create <template>
elements with any valid HTML inside.
Then set their ids as values of the HeaderTemplate
and FooterTemplate
properties.
<template id="headerTemplate"> <div>Are you sure you want to delete your account?</div> </template> <template id="footerTemplate"> <smart-button style="margin: 0 10px" class="primary">Confirm</smart-button><smart-button class="error">Cancel</smart-button> </template> <Button id="openButton" OnClick="OnClick">Open/Close</Button> <Window IsOpened="@opened" ResizeMode="WindowResizeMode.Both" LiveResize="true" HeaderTemplate="@headerTemplate" FooterTemplate="@footerTemplate" label="Window 1"> <div id="article"> <section> <h3>Warning: Deleted Accounts cannot be restored.</h3> <h3>Read more about account deletion in our <a>Terms of Use</a></h3> </section> </div> </Window> @code { private bool opened = true; private string Status = ""; private string headerTemplate = "headerTemplate"; private string footerTemplate = "footerTemplate"; private void OnClick() { opened = !opened; } }
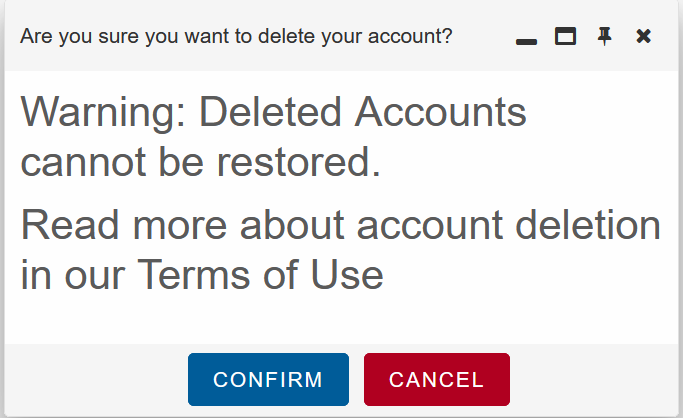
Modal
The Window element can be turned into a Modal - When opened, the user can only interact with the Window and nothing else on the page:
<Window HeaderButtons="@headerButtons" Modal="true">...</Window>
In the example below, the Modal is the only interactable element in the page
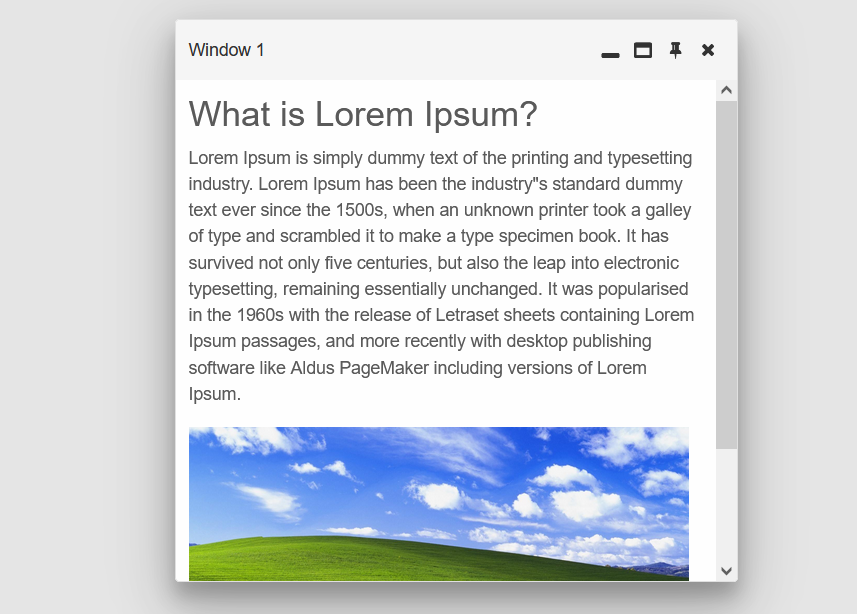
Events
Smart.Window provides built-in Events that can help you expand the component's default functionality.
OnOpening
- triggered just before the window starts opening.
Event Details
: N/AOnOpen
- triggered when the window is opened / visible.
Event Details
: N/AOnClosing
- triggered just before the window starts closing.
Event Details
: N/AOnClose
- triggered when the window is closed / hidden.
Event Details
: N/AOnCollapse
- triggered when the window is collapsed.
Event Details
: N/AOnDragEnd
- triggered when window's dragging is ended.
Event Details
: N/AOnDragStart
- triggered when window's dragging is started.
Event Details
: N/AOnExpand
- triggered when the window is expanded.
Event Details
: N/AOnMaximize
- triggered when the window is maximized.
Event Details
: N/AOnMinimize
- triggered when the window is minimized.
Event Details
: N/AOnResizeEnd
- triggered when window's resizing is ended.
Event Details
: N/AOnResizeStart
- triggered when window's resizing is started.
Event Details
: N/AOnRestore
- triggered when the window is restored to it's previous state before maximization.
Event Details
: N/A
<Window IsOpened="@opened" ResizeMode="WindowResizeMode.Both" LiveResize="true" HeaderButtons="@headerButtons" OnDragStart="OnDragStart" OnDragEnd="OnDragEnd" OnResizeStart="OnResizeStart" OnResizeEnd="OnResizeEnd"></Window> @code { private bool opened = true; private string Status = ""; private void OnClick() { opened = !opened; } private string[] headerButtons = new string[]{"close", "pin", "maximize", "minimize"}; private void OnDragStart(){ Status = "Dragging started"; } private void OnDragEnd(){ Status = "Dragging ended"; } private void OnResizeStart(){ Status = "Resize started"; } private void OnResizeEnd(){ Status = "Resize ended"; } }
The demo below displays information about the status of the Window:
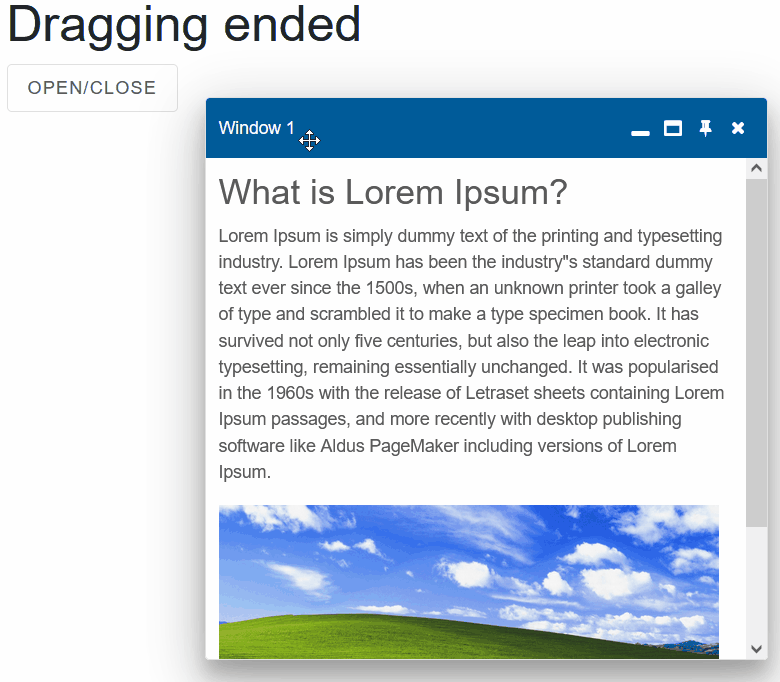