Using Smart.Grid with DynamoDB
Load data from DynamoDB in Smart.Grid
Overview
In this tutorial, you will create a simple web application with Smart.Grid and load its data from DynamoDB.Setting up DynamoDB Web Service
To use DynamoDB, you will need to sign up for AWS and get AWS access key. You can learn more hereCreate a DynamoDB Table
- Log into the Amazon DynamoDB Console.
- Make sure you note what region you are creating your function in. You can see this at the very top of the page, next to your account name.
- Click the blue "Create table" button.
- Next to "Table name" type in MyDynamoDBTable.
- In the "Primary Key" field type in id.
- Add additional field, called name.
- Click the blue "Create" button.
- Add data to the table, by using the Create item blue button. We added the following list of items:
{"id": 1, "name": "Hydrogen"}, {"id": 2, "name": "Helium"}, {"id": 3, "name": "Lithium"}, {"id": 4, "name": "Beryllium"}, {"id": 5, "name": "Boron"}, {"id": 6, "name": "Carbon"}, {"id": 7, "name": "Nitrogen"}, {"id": 8, "name": "Oxygen"}, {"id": 9, "name": "Fluorine"}, {"id": 10, "name": "Neon"}, {"id": 11, "name": "Sodium"}, {"id": 12, "name": "Magnesium"}, {"id": 13, "name": "Aluminum"}, {"id": 14, "name": "Silicon"}, {"id": 15, "name": "Phosphorus"}, {"id": 16, "name": "Sulfur"}, {"id": 17, "name": "Chlorine"}, {"id": 18, "name": "Argon"}, {"id": 19, "name": "Potassium"}, {"id": 20, "name": "Calcium"}
Create a new DataGrid instance and load the data from DynamoDB
- Set up the AWS SDK for JavaScript. To do this, add or modify the following script tag to your HTML pages: "https://sdk.amazonaws.com/js/aws-sdk-2.7.16.min.js"></script>"
- Set up an AWS access key to use AWS SDKs.
- Add a script reference to "smart.grid.js" in order to use Smart.Grid.
- Add the CSS styles required for the Grid by adding a reference to "smart.default.css".
- Query the data by using DynamoDB "scan" method. The scan method reads every item in the entire table, and returns all the data in the table. You can provide an optional filter_expression, so that only the items matching your criteria are returned. However, the filter is applied only after the entire table has been scanned. In our tutorial, we display all items from the DynamoDB table, we created in the previous step.
- Within the "scan" command, when we get the data, we create a new Smart.Grid instance and add sorting, selection and column resize capabilities to it.
<!DOCTYPE html> <html> <head> <title></title> <meta charset="utf-8" /> <script src="https://sdk.amazonaws.com/js/aws-sdk-2.7.16.min.js"></script> <script type="module" src="../../source/modules/smart.grid.js"></script> <link rel="stylesheet" type="text/css" href="../../source/styles/smart.default.css" /> <script type="module"> AWS.config.update({ region: "us-east-1", // accessKeyId default can be used while using the downloadable version of DynamoDB. // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead. accessKeyId: "YOUR-ACCESS-KEY-ID", // secretAccessKey default can be used while using the downloadable version of DynamoDB. // For security reasons, do not store AWS Credentials in your files. Use Amazon Cognito instead. secretAccessKey: "YOUR-SERCRET-ACCESS-KEY" }); const dynamoClient = new AWS.DynamoDB.DocumentClient(); const params = { TableName: 'MyDynamoDBTable', // give it your table name Select: "ALL_ATTRIBUTES" }; dynamoClient.scan(params, function(err, data) { if (err) { console.error("Unable to read item. Error JSON:", JSON.stringify(err, null, 2)); } else { const grid = new smartGrid("#grid", { behavior: { columnResizeMode: 'growAndShrink' }, appearance: { alternationCount: 2, showRowHeader: true, showRowHeaderSelectIcon: true, showRowHeaderFocusIcon: true }, sorting: { enabled: true }, selection: { enabled: true, allowCellSelection: true, allowRowHeaderSelection: true, allowColumnHeaderSelection: true, mode: 'extended' }, behavior: { columnResizeMode: 'growAndShrink' }, dataSource: data.Items } ); } }); </script> </head> <body> <div id="grid"></div> </body> </html>
Result:
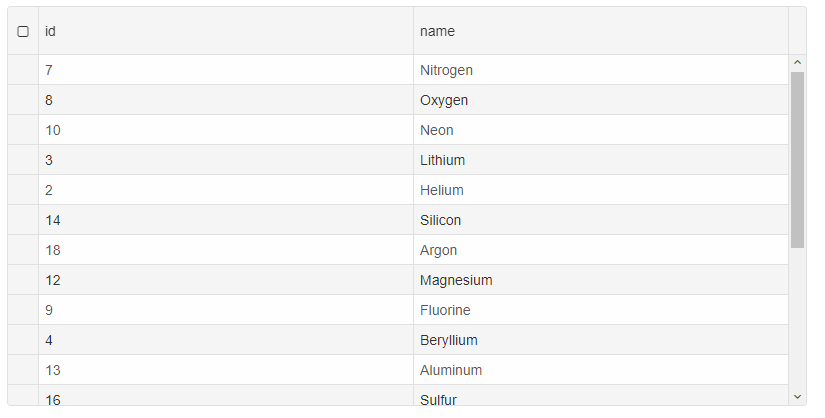