Using with Lit Element
In this page, we will show you how to use LitElement with Smart UI.
The LitElement is a simple base class for creating fast, lightweight web components that work in any web page with any framework.
LitElement is similar to our Smart.BaseElement. Both provide API for creating Web Components with support for typed properties,
two-way and one-way property bindings, HTML templates and Shadow DOM.
In Smart.BaseElement, we added additional things like: Built-in localization support, nullable properties, Int64 support, WAI-ARIA accessibility support,
Device agnostic event handling, Style changed notifications, Web Component Resize notifications and View Model data binding,
1. Installation
The quickest way to try out a lit element project locally is to download one of the starter projects as a zip file.- Download the LitElement starter project from GitHub as a zip file: Javascript starter project.
- Uncompress the zip file.
- Install dependencies:
cd project-folder npm i npm install smart-webcomponents
- Run the dev server
npm run serve
-
Open the project demo page in a browser tab. For example:
http://localhost:8000/dev/
Your server may use a different port number. Check the URL in the terminal output for the correct port number.
2. Edit your component
Edit your component definition. The file you edit depends on which language you’re using:- JavaScript. Edit the
my-element.js
file in the project root. - TypeScript. Edit the
my-element.ts
file in thesrc
directory.
3. Add a Smart Web Component in a LitElement as a child
Open dev/index.html and add a reference to the Smart.Grid's single-file component smart.ui.grid.js.
This component includes the Smart.Grid's CSS and Javascript dependencies.
<script type="module" src="../node_modules/smart-webcomponents/source/components/smart.ui.grid.js"></script>
4. Setup Smart Web Component
The code below sets the Grid data source.
<script type="module">
window.grid.dataSource = [
{ "EmployeeID": 1, "FirstName": "Nancy", "LastName": "Davolio", "ReportsTo": 2, "Country": "USA", "Title": "Sales Representative", "HireDate": "1992-05-01 00:00:00", "BirthDate": "1948-12-08 00:00:00", "City": "Seattle", "Address": "507 - 20th Ave. E.Apt. 2A" },
{ "EmployeeID": 2, "FirstName": "Andrew", "LastName": "Fuller", "ReportsTo": null, "Country": "USA", "Title": "Vice President, Sales", "HireDate": "1992-08-14 00:00:00", "BirthDate": "1952-02-19 00:00:00", "City": "Tacoma", "Address": "908 W. Capital Way" },
{ "EmployeeID": 3, "FirstName": "Janet", "LastName": "Leverling", "ReportsTo": 2, "Country": "USA", "Title": "Sales Representative", "HireDate": "1992-04-01 00:00:00", "BirthDate": "1963-08-30 00:00:00", "City": "Kirkland", "Address": "722 Moss Bay Blvd." },
{ "EmployeeID": 4, "FirstName": "Margaret", "LastName": "Peacock", "ReportsTo": 2, "Country": "USA", "Title": "Sales Representative", "HireDate": "1993-05-03 00:00:00", "BirthDate": "1937-09-19 00:00:00", "City": "Redmond", "Address": "4110 Old Redmond Rd." },
{ "EmployeeID": 5, "FirstName": "Steven", "LastName": "Buchanan", "ReportsTo": 2, "Country": "UK", "Title": "Sales Manager", "HireDate": "1993-10-17 00:00:00", "BirthDate": "1955-03-04 00:00:00", "City": "London", "Address": "14 Garrett Hill" },
{ "EmployeeID": 6, "FirstName": "Michael", "LastName": "Suyama", "ReportsTo": 5, "Country": "UK", "Title": "Sales Representative", "HireDate": "1993-10-17 00:00:00", "BirthDate": "1963-07-02 00:00:00", "City": "London", "Address": "Coventry House Miner Rd." },
{ "EmployeeID": 7, "FirstName": "Robert", "LastName": "King", "ReportsTo": 5, "Country": "UK", "Title": "Sales Representative", "HireDate": "1994-01-02 00:00:00", "BirthDate": "1960-05-29 00:00:00", "City": "London", "Address": "Edgeham Hollow Winchester Way" },
{ "EmployeeID": 8, "FirstName": "Laura", "LastName": "Callahan", "ReportsTo": 2, "Country": "USA", "Title": "Inside Sales Coordinator", "HireDate": "1994-03-05 00:00:00", "BirthDate": "1958-01-09 00:00:00", "City": "Seattle", "Address": "4726 - 11th Ave. N.E." },
{ "EmployeeID": 9, "FirstName": "Anne", "LastName": "Dodsworth", "ReportsTo": 5, "Country": "UK", "Title": "Sales Representative", "HireDate": "1994-11-15 00:00:00", "BirthDate": "1966-01-27 00:00:00", "City": "London", "Address": "7 Houndstooth Rd." }
]
</script>
Add the Grid to the LitElement's my-element tag.
<my-element>
<p>This is child content</p>
<smart-ui-grid id="grid"></smart-ui-grid>
</my-element>
The full example code is:
<!doctype html>
<html>
<head>
<meta charset="utf-8">
<title><my-element> Demo</title>
<script type="module" src="../my-element.js"></script>
<script type="module" src="../node_modules/smart-webcomponents/source/components/smart.ui.grid.js"></script>
<script type="module">
window.grid.dataSource = [
{ "EmployeeID": 1, "FirstName": "Nancy", "LastName": "Davolio", "ReportsTo": 2, "Country": "USA", "Title": "Sales Representative", "HireDate": "1992-05-01 00:00:00", "BirthDate": "1948-12-08 00:00:00", "City": "Seattle", "Address": "507 - 20th Ave. E.Apt. 2A" },
{ "EmployeeID": 2, "FirstName": "Andrew", "LastName": "Fuller", "ReportsTo": null, "Country": "USA", "Title": "Vice President, Sales", "HireDate": "1992-08-14 00:00:00", "BirthDate": "1952-02-19 00:00:00", "City": "Tacoma", "Address": "908 W. Capital Way" },
{ "EmployeeID": 3, "FirstName": "Janet", "LastName": "Leverling", "ReportsTo": 2, "Country": "USA", "Title": "Sales Representative", "HireDate": "1992-04-01 00:00:00", "BirthDate": "1963-08-30 00:00:00", "City": "Kirkland", "Address": "722 Moss Bay Blvd." },
{ "EmployeeID": 4, "FirstName": "Margaret", "LastName": "Peacock", "ReportsTo": 2, "Country": "USA", "Title": "Sales Representative", "HireDate": "1993-05-03 00:00:00", "BirthDate": "1937-09-19 00:00:00", "City": "Redmond", "Address": "4110 Old Redmond Rd." },
{ "EmployeeID": 5, "FirstName": "Steven", "LastName": "Buchanan", "ReportsTo": 2, "Country": "UK", "Title": "Sales Manager", "HireDate": "1993-10-17 00:00:00", "BirthDate": "1955-03-04 00:00:00", "City": "London", "Address": "14 Garrett Hill" },
{ "EmployeeID": 6, "FirstName": "Michael", "LastName": "Suyama", "ReportsTo": 5, "Country": "UK", "Title": "Sales Representative", "HireDate": "1993-10-17 00:00:00", "BirthDate": "1963-07-02 00:00:00", "City": "London", "Address": "Coventry House Miner Rd." },
{ "EmployeeID": 7, "FirstName": "Robert", "LastName": "King", "ReportsTo": 5, "Country": "UK", "Title": "Sales Representative", "HireDate": "1994-01-02 00:00:00", "BirthDate": "1960-05-29 00:00:00", "City": "London", "Address": "Edgeham Hollow Winchester Way" },
{ "EmployeeID": 8, "FirstName": "Laura", "LastName": "Callahan", "ReportsTo": 2, "Country": "USA", "Title": "Inside Sales Coordinator", "HireDate": "1994-03-05 00:00:00", "BirthDate": "1958-01-09 00:00:00", "City": "Seattle", "Address": "4726 - 11th Ave. N.E." },
{ "EmployeeID": 9, "FirstName": "Anne", "LastName": "Dodsworth", "ReportsTo": 5, "Country": "UK", "Title": "Sales Representative", "HireDate": "1994-11-15 00:00:00", "BirthDate": "1966-01-27 00:00:00", "City": "London", "Address": "7 Houndstooth Rd." }
]
</script>
<style>
p {
border: solid 1px blue;
padding: 8px;
}
</style>
</head>
<body>
<my-element>
<p>This is child content</p>
<smart-ui-grid id="grid"></smart-ui-grid>
</my-element>
</body>
</html>
Adding a LitElement as a child of a Smart Web Component
Now, we will add our LitElement's my-element tag as a child of Smart.Tabs Web Component.
In order to achieve that, we need to add a reference to smart.ui.tabs.js and add the necessary HTML tags.
<!doctype html> <html> <head> <meta charset="utf-8"> <title>LitElement Demo</title> <script type="module" src="../my-element.js"></script> <script type="module" src="../node_modules/smart-webcomponents/source/components/smart.ui.tabs.js"></script> <style> p { border: solid 1px blue; padding: 8px; } </style> </head> <body> <smart-ui-tabs> <smart-tab-item label="Tab 1"> <my-element> <p>This is child content</p> </my-element> </smart-tab-item> <smart-tab-item label="Tab 2"> <my-element> <p>This is child content 2</p> </my-element> </smart-tab-item> </smart-ui-tabs> </body> </html>
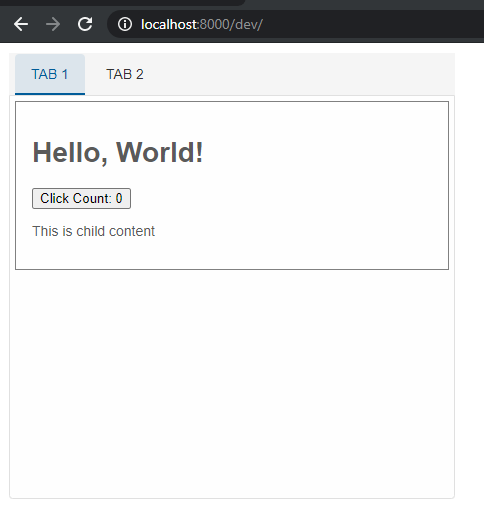