Smart.ListBox - Load data on demand when scrolling
Overview
Load on demand when scrolling means that the ListBox loads its Items when you type any text in the input and when the vertical scrollbar reaches the bottom of the ListBox. To setup the feature, you need to set the following properties:dataSource
property. The dataSource
property should be set to a function with two arguments - query and callback function.
The query argument is used to fetch data. On success, you need to call the callback function and pass the returned data.
Example
// prepare the items. let items = []; for (let i = 0; i < 100000; i++) { items.push('item' + i); } // setup the listbox properties. list is an instance of the Listbox and its "virtualized" property is set to true in the HTML markup as a tag attribute. list.itemHeight = 30; // load the first 150 items. list.dataSource = items.slice(0, 150); let scrollBottomReached = 0; // subscribe to the scroll bottom reached. list.addEventListener('scrollBottomReached', () => { // load the next 150 items. const start = 150 * scrollBottomReached; scrollBottomReached++; const end = 150 * scrollBottomReached; const newItems = items.slice(start, end) list.add(newItems); })Example with Remote Data
<smart-list-box filterable selection-mode="zeroOrOne"></smart-list-box>For a demo source, we use a file, called customers.json and use alasql to query it.
list.dataSource = (filterQuery, callback) => { list.scrollBottomIndex = 0; if (!window.DemoServer) { fetch('https://raw.githubusercontent.com/HTMLElements/smart-webcomponents/master/sampledata/customers.json').then(response => response.json()) .then(data => { window.demoServer = DemoServer(data); const result = window.demoServer.getData({ query: filterQuery, offset: list.scrollBottomIndex }); callback(result.data.map(item => item.CompanyName)); }); } else { setTimeout(() => { const result = window.demoServer.getData({ query: filterQuery, offset: list.scrollBottomIndex }); callback(result.data.map(item => item.CompanyName)); }, 100); } } // add items when the scroll bottom is reached. list.onScrollBottomReached = () => { // add offset when the bottom is reached so we can query the items with an offset from the start. list.scrollBottomIndex++; const result = window.demoServer.getData({ query: '', offset: list.scrollBottomIndex }); const items = result.data.map(item => item.CompanyName); // add items here. list.add(items); }Here is how we setup the DemoServer
// In this sample, we use http://alasql.org/ to show how to use SQL queries with Smart.Grid export function DemoServer(allData) { window.alasql.options.cache = false; return { getData: function (request) { const queryResult = executeSql(request); return { data: queryResult.data, length: queryResult.length }; }, }; function executeSql(detail) { // use the filter query and scroll offset to get the list of items. const sql = 'SELECT * FROM ? WHERE CompanyName LIKE "%' + detail.query + '%" LIMIT 10 OFFSET ' + (10 * detail.offset); const result = window.alasql(sql, [allData]); return { data: result, length: length }; } }
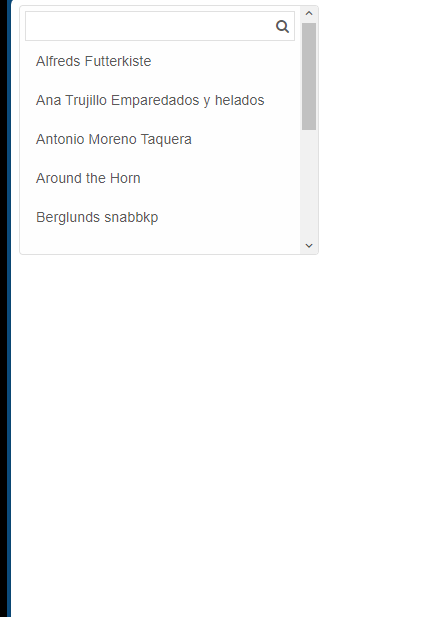