Blazor Smart.PivotTable Designer
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup the Blazor Smart.PivotTable
Follow the Get Started with Smart.PivotTable guide to set up the component.
Designer
A Pivot Table designer can be displayed by setting the property Designer to true
. By default, the designer appears on the right of the Pivot Table. Its position is controlled by the property DesignerPosition that is an enum (PivotTableDesignerPosition
) with two possible values:
Near
- designer appears on the side nearest to the group column.Far
(default) - designer appears on the side farthest from the group column.
The Pivot Table's designer is essentially tabs with two views - Columns and Filters.
Designer "Columns" View
In the "Columns" view, there are four lists of columns, dragging between which is possible, as follows:
- Columns - all columns, as set up in the Columns property. Dragging to this list from another list removes the role associated with the source list.
- Row Groups - all columns with RowGroup enabled. Only columns with AllowRowGroup can be dragged here.
- Summaries - all columns with a Summary function. Any column can be dragged here. If a column is dragged here, the summary function applied is either the one returned by the callback function GetDefaultSummaryFunction (if implemented) or 'count'. There must always be at least one column in this list.
- Pivots - all columns with Pivot enabled. Only columns with AllowPivot can be dragged here.
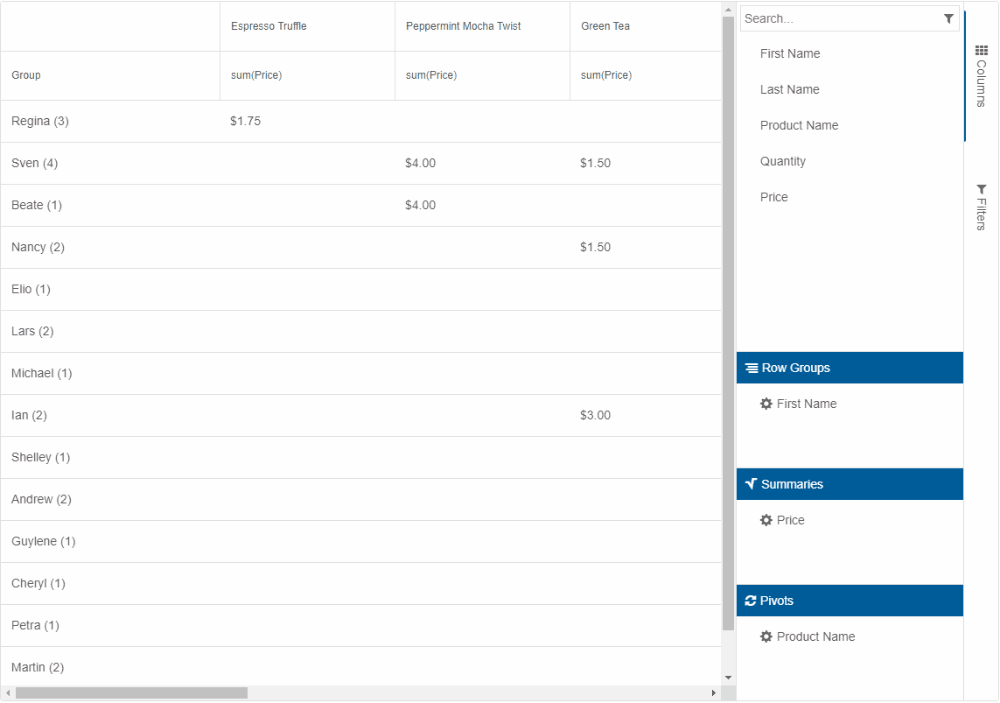
Further configuration of columns is available by clicking the cog icon next to a column label. This opens a Column settings dialog with the following options:
-
Available to all columns
- Move to - changes the role of a column;
- Text alignement - changes the text alignement of the headers of the dynamic columns corresponding to this column. Corresponds and is in sync with the column property Align.
-
Available to Summaries columns only
- Calculation - changes the summary function. Corresponds and is in sync with the column property Summary;
- Number alignment - changes the text alignment in summary column cells. Corresponds and is in sync with the column property SummarySettings.align;
- Number prefix - changes the text prefix in summary column cells. Useful for adding currency symbols. Corresponds and is in sync with the column property SummarySettings.prefix;
- Decimal places - changes the decimal places in summary column cells. Corresponds and is in sync with the column property SummarySettings.decimalPlaces;
- Thousands separator - changes the thousands separator character in summary column cells. Corresponds and is in sync with the column property SummarySettings.thousandsSeparator;
- Decimal separator - changes the decimal separator character in summary column cells. Corresponds and is in sync with the column property SummarySettings.decimalSeparator;
- Negatives in brackets - if enabled, negative values appear in brackets instead of with the minus sign. Corresponds and is in sync with the column property SummarySettings.negativesInBrackets.
Designer "Filters" View
In the designer's "Filters" view, Excel-like filters with all unique values from the original data source (and not aggregated ones) are available for each column. These filter panels are displayed in an accordion for ease of access.
Filters applied via the Pivot Table Designer discard any filters applied programmatically with the method AddFilter and vice versa.
Designer and Toolbar Interaction
Both the Pivot Table's toolbar and a standalone designer can be shown at the same time, allowing columns to be dragged from the designer to the toolbar's breadcrumbs and vice versa and change the column roles dynamically.
Example
Here is a full example of Pivot Designer with Toolbar:
@using Smart.Blazor.Demos.Data; @inject RandomDataService RandomDataService <PivotTable DataSource="Data" Designer Toolbar FreezeHeader KeyboardNavigation Columns="@columns" /> @code { List<PivotTableColumn> columns = new List<PivotTableColumn>() { new PivotTableColumn() { Label = "First Name", DataField = "FirstName", DataType = PivotTableColumnDataType.String, AllowRowGroup = true, RowGroup = true }, new PivotTableColumn() { Label = "Last Name", DataField = "LastName", DataType = PivotTableColumnDataType.String, AllowPivot = true, AllowRowGroup = true, RowGroup = true }, new PivotTableColumn() { Label = "Product Name", DataField = "ProductName", DataType = PivotTableColumnDataType.String, AllowPivot = true, Pivot = true }, new PivotTableColumn() { Label = "Quantity", DataField = "Quantity", DataType = PivotTableColumnDataType.Number }, new PivotTableColumn() { Label = "Price", DataField = "Price", DataType = PivotTableColumnDataType.Number, Summary = PivotTableColumnSummary.Sum, SummarySettings = new { prefix = "$", decimalPlaces = 2 } } }; public DataRecord[] Data; protected override async Task OnInitializedAsync() { Data = await RandomDataService.GetDataAsync(25); } }Result:
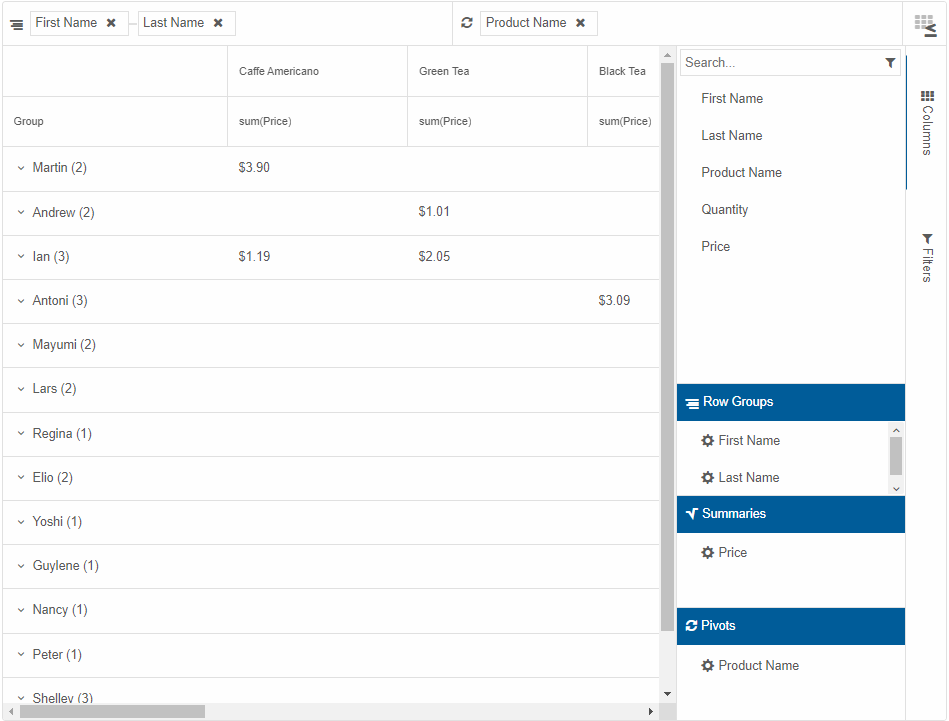