DropDownList - Label & Value Pairs
Smart.DropDownList allows you to differentiate between the displayed label and the actual value of each list item.
Using ListItem
Add the DropDownList component. Set the list of options as a series of <ListItem>.
When the user selects an item, the DropDownList will return the value of the selected item:
<h3>@(string.Join(", ", selectedItems))</h3> <DropDownList SelectionMode="ListSelectionMode.CheckBox" @bind-SelectedValues="@selectedItems"> <ListItem Value="1">Affogato</ListItem> <ListItem Value="2">Americano</ListItem> <ListItem Value="3">Bicerin</ListItem> <ListItem Value="4">Breve</ListItem> <ListItem Value="5">Cappuccino</ListItem> <ListItem Value="6">Cafe Crema</ListItem> <ListItem Value="7">Cafe Corretto</ListItem> </DropDownList> @code{ string[] selectedItems = new string[] { }; }
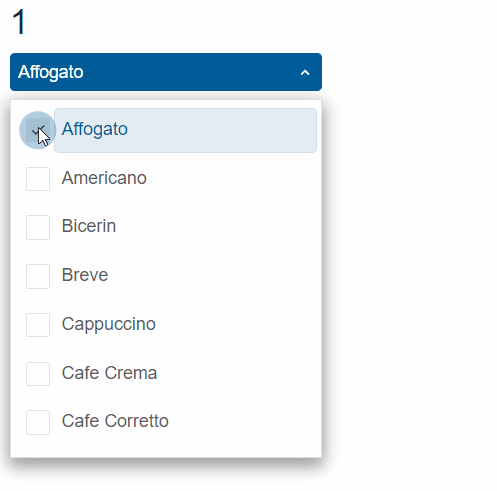
Using DataSource
Alternatively, you can set the list of options as a DataSource.
If using an Array of custom class, use the [JsonPropertyName] attribute to set which field should be treated as the label, and which as the value.
Example:
@using System.Text.Json.Serialization <h3>@(string.Join(", ", selectedItems))</h3> <DropDownList DataSource="countries" SelectionMode="ListSelectionMode.CheckBox" @bind-SelectedValues="@selectedItems"> </DropDownList> @code{ class Country { [JsonPropertyName("label")] public string Name { get; set; } [JsonPropertyName("value")] public string Code { get; set; } public Country(string name, string code) { Name = name; Code = code; } } string[] selectedItems = new string[] { }; List<Country> countries = new List<Country>() { new Country("France", "FR"), new Country("Germany", "DE"), new Country("Italy", "IT"), new Country("Spain", "ES"), new Country("Portugal", "PT"), new Country("United Kingdom", "GB") }; }
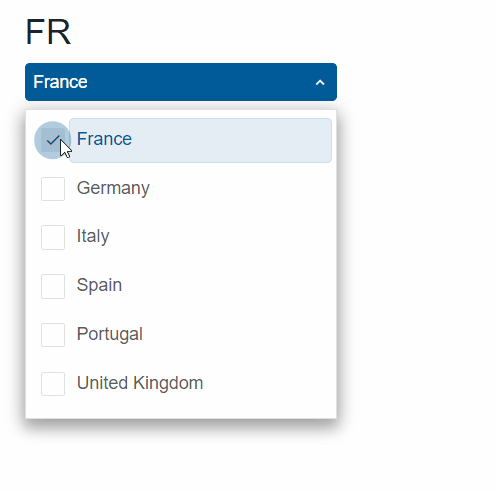