Reorder Columns
Smart.Grid allows you to select which columns can be reordered.
To enable reordering set the column's AllowReorder
property to true:
@inject WeatherForecastService ForecastService <Grid DataSource="@forecast" > <Columns> <Column DataField="Date" Label="Date" AllowReorder="true"> </Column> <Column DataField="TemperatureC" Label="Temp. (C)" AllowReorder="true"> </Column> <Column DataField="TemperatureF" Label="Temp. (F)" AllowReorder="true"> </Column> <Column DataField="Summary" Label="Summary" AllowReorder="true"> </Column> </Columns> </Grid> @code{ private WeatherForecast[] forecast; protected override async Task OnInitializedAsync() { forecast = await ForecastService.GetForecastAsync(DateTime.Now); } }
Then you need to enable AllowColumnReorder of the Grid using the GridBehavior object.
<Grid DataSource="@forecast" Behavior="gridBehavior"> .... </Grid> @code{ GridBehavior gridBehavior = new GridBehavior(){ AllowColumnReorder = true }; .... }
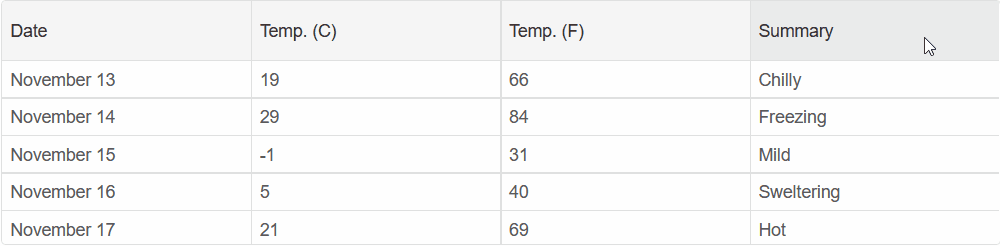
ReorderColumns() Method
Smart.Grid allows you to programmatically change the order of a column.
The ReorderColumns()
function admits 3 arguments -
dataField
- The data field or column index of the column you wish to move
referenceDataField
- The data field or column index of the second grid column
referenceDataField
(optional) - Determines whether to insert the first column after the reference
column
<Button OnClick="ChangeOrder">Change Order</Button> <Grid @ref="grid" DataSource="@forecast" Behavior="gridBehavior"> .... </Grid> @code{ Grid grid; private void ChangeOrder(){ grid.ReorderColumns("Summary","TemperatureC"); } .... }
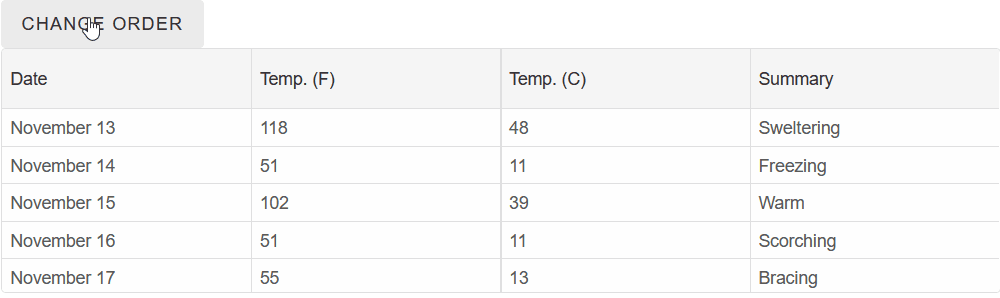