Build your web apps using Smart Custom Elements
Smart HTML Elements Router
Smart HTML Elements provides a JavaScript Router plug-in that can be used to power routing in a modern single-page application. The Smart.Router plug-in is built entirely with standard JavaScript and does not depend on third-party software.
The main features of Smart.Router are:
- Routing within a single-page (no refresh required);
- Implementation based on JavaScript History API; this allows navigating to previous views with the browser's Back and Forward buttons;
- Automatic routing when clicking anchor tags on the page;
- View templates can be a local template or an external HTML file;
- Loading specific external styles and scripts for each view;
- Smooth transition when changing views;
- Allowing for different page titles for each view.
Smart.Router API
Using Smart.Router requires the scripts smart.element.js and smart.router.js to be included on a page. The Router plug-in can be initialized as an instance of the Smart.Router class:
const router = new Smart.Router('mainView', '/demos/router/basic');
The constructor receives two parameters:
- view: string | HTMLElement - the HTMLElement reference or the id of the element on the page that will serve as container for the router's views. Essentially, this is the dynamic part of the web page (excluding menus, toolbars, etc.)
- base: string - the base URL to consider relative paths from.
- You can pass an absolute path from the server root, such as
'/demos/router/basic'
- You can also pass a relative path, such as
'./'
- You can pass an absolute path from the server root, such as
Additionally, there are two more settings that can be applied by setting them to an initialized Smart.Router instance:
- cache: boolean - if enabled, external scripts are loaded only the first time a view is loaded.
- templateApplied: (route: any) => void - a callback function called each time a routing occurs and the target view's template is applied; useful for initializing widgets after all necessary styles, scripts, and HTML have been loaded.
Methods
setRoutes(routes: { path: string, template: string, styleUrls: string[], style: string, scriptUrls: (string | { src: string, type: string })[], script: string, title: string }[])
To set up the routing on the page, call the setRoutes method and pass the routes configuration as a parameter. This parameter is an Array, each member (route) of which is an object with fields described below.
- path: string - the view's path, relative to the main page. Default paths are
ususally just
'/'
. Subsequent route paths are forward slash followed by the view's name, e.g.'/about'
. The path appears on the browsers location bar when routing. - template: string - either the id of a local HTMLTemplateElement or a path to an external HTML file. The HTML contents serves as a template for the view of the route.
- styleUrls: string[] - a list of the paths to external style sheets to be loaded when routing. The order of the list is preserved when loading.
- style: string - inline CSS to be parsed as a local style.
- scriptUrls: (string | { src: string, type: string })[] - a list of external
scripts to be loaded when routing. The order of the list is preserved when loading. Members of
the list can be:
- string only - considered a path to the script; the script itself is considered of type module;
- an object with the fields src (path to the script) and
type (e.g.
'text/javascript'
).
- script: string - inline JavaScript to be parsed as a local script.
- title: string - the page's title when the view is loaded. Seen in the page's browser tab and when observing the browser's history.
route(path: string)
To route to a view programmatically, call the route method and pass the path to the view, as set up in the respective route passed to setRoutes.
Example
Presented here is a simple single-page web application that uses Smart.Router to route to three different views.
HTML and Templates
In a sample HTML page we have the following structure:
<div id="menu"> <a href="./">Home</a> <a href="./about">About</a> <a href="./contacts">Contacts</a> </div> <div id="mainView"></div> <template id="homeTemplate"> <h1>Home</h1> <p>Welcome to our website!</p> </template> <template id="aboutTemplate"> <h1>About</h1> <p>This is a sample page.</p> </template> <template id="contactsTemplate"> <h1>Contacts</h1> <p>Write us an email!</p> </template>
We have three options in a menu and a div with id mainView - which will act as the container for all views. The views' templates are defined locally in HTMLTemplateElements.
JavaScript Routing
The following code initializes a Smart.Router instance and sets it up with three routes with paths corresponding to the anchor tags in the page's menu and templates corresponding to the ones in the HTML structure. Finally, the router routes directly to the Home view after initialization.
window.onload = function () { const router = new Smart.Router('mainView', './'); router.setRoutes([ { path: '/', template: 'homeTemplate', title: 'Home' }, { path: '/about', template: 'aboutTemplate', title: 'About' }, { path: '/contacts', template: 'contactsTemplate', title: 'Contacts' } ]); router.route('/'); };
Smart.Router automatically handles clicking links on the page (in this case, the ones in the menu) and if they correspond to a defined route, uses them for navigation directly, without the need for users to set up anything further.
Here is the application in action:
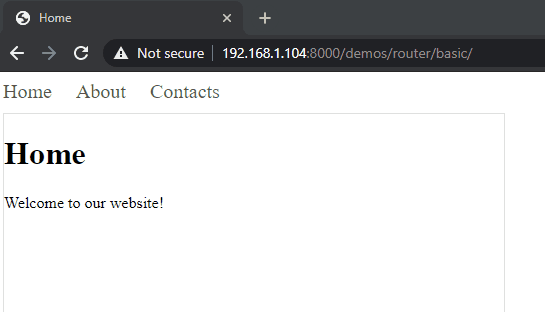
A large-scale dashboard application with a more complex structure and with loading external templates, styles, and scripts for each view can be seen here: Smart HTML Elements | Admin & Dashboard Template With Bootstrap.