Blazor - Get Started with Smart.RepeatButton
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic RepeatButton
Smart.RepeatButton is a button element that repeats a single action until release.
- Add the RepeatButton component to the Pages/Index.razor file
<RepeatButton></RepeatButton>
Delay
indicates the time in miliseconds between the execution of each action, whileInitialDelay
applies delay only on the very first Click event:<RepeatButton Delay="100">Repeat Button</RepeatButton>
- Optionally, set the name and value of the RepeatButton:
<RepeatButton Delay="100" Name="repeat" Value="enabled">Repeat Button</RepeatButton>
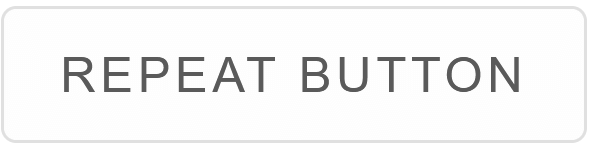
RepeatButton Event
In order to atach a change listener to the RepeatButton, it is necessary to use the IJSRuntime interface
@inject IJSRuntime JS
Create an Increase function that will increment a number by one every time it is called:
public static int number = 1; [JSInvokable] public void Increase() { number++; StateHasChanged(); }
Using JSInterop, inoke a custom JS function called "setListener":
protected override void OnInitialized() { base.OnInitialized(); JS.InvokeVoidAsync("setListener", DotNetObjectReference.Create(this), nameof(Increase)); }
Inside the \_Host.cshtml file (server-side Blazor) or wwwroot/index.html (client-side WebAssembly Blazor), create a function that will attach an event listener to the DOM element and will then call our C# function:
<script> (function (global) { global.setListener = (dotNetHelper) => { setTimeout(function(){ document.querySelector("smart-repeat-button").addEventListener('click', function(){ dotNetHelper.invokeMethodAsync('Increase') }) }, 0) } })(window); </script>
The demo below will increase the counter, while the user is pressing the RepeatButton:
<RepeatButton Delay="100" id="increase-btn">+</RepeatButton> <h3>@number</h3> @code{ public static int number = 1; [JSInvokable] public void Increase() { number++; StateHasChanged(); } protected override void OnInitialized() { base.OnInitialized(); JS.InvokeVoidAsync("setListener", DotNetObjectReference.Create(this), nameof(Increase)); } }
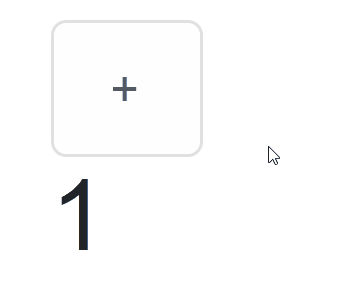