Grid Row Filtering
Enable Filtering
Grid filtering is enabled by setting thefiltering.enabled
property to true. You can then filter a column by using a column filter menu, filter row editor, filter panel displayed in the Grid toolbar or by using the Grid API.
const gridOptions = { dataSourceSettings: { dataFields: [ { name: 'firstName', dataType: 'string' }, { name: 'lastName', dataType: 'string' }, { name: 'productName', map: 'product.name', dataType: 'string' }, { name: 'quantity', map: 'product.quantity', dataType: 'number' }, { name: 'price', map: 'product.price', dataType: 'number' }, { name: 'total', map: 'product.total', dataType: 'number' } ] }, behavior: { columnResizeMode: 'growAndShrink' }, filtering: { enabled: true }, dataSource: [ { firstName: 'Andrew', lastName: 'Burke', product: { name: 'Ice Coffee', price: 10, quantity: 3, total: 30 } }, { firstName: 'Petra', lastName: 'Williams', product: { name: 'Espresso', price: 7, quantity: 5, total: 35 } }, { firstName: 'Kevin', lastName: 'Baker', product: { name: 'Frappucino', price: 6, quantity: 4, total: 24 } } ], columns: [ { label: 'First Name', dataField: 'firstName', allowSort: false }, { label: 'Last Name', dataField: 'lastName' }, { label: 'Product', dataField: 'productName' }, { label: 'Quantity', dataField: 'quantity', cellsAlign: 'right' }, { label: 'Unit Price', dataField: 'price', cellsAlign: 'right', cellsFormat: 'c2' } ] }
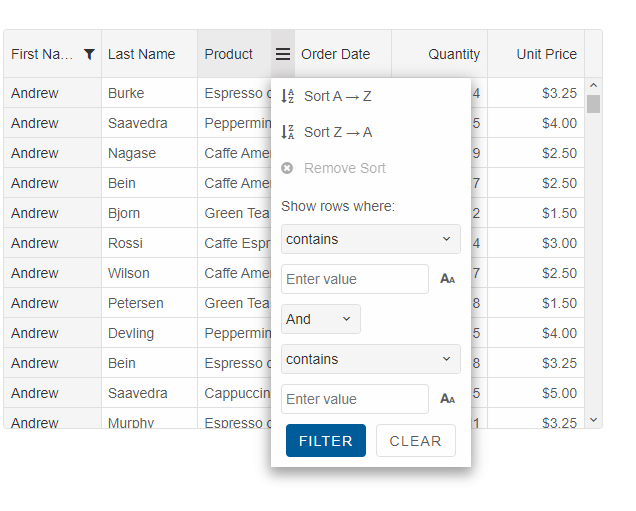
Disable Column Filtering
Disable filtering for columns by setting the allowFilter
column definition to false
.
Filter Column when you create the Grid
You can use thefiltering.filter
property. The property expects an Array with filter definitions. Each sub filter represents a 'dataField' and filter expression.
filtering: { enabled: true, filter: [ ['firstName', 'contains Andrew or contains Nancy'], ['quantity', '>= 3 and <= 8'] ] }
Excel Filter Menu
ThefilterMenuMode
property determines whether a column menu is displayed in default or excel-like mode.
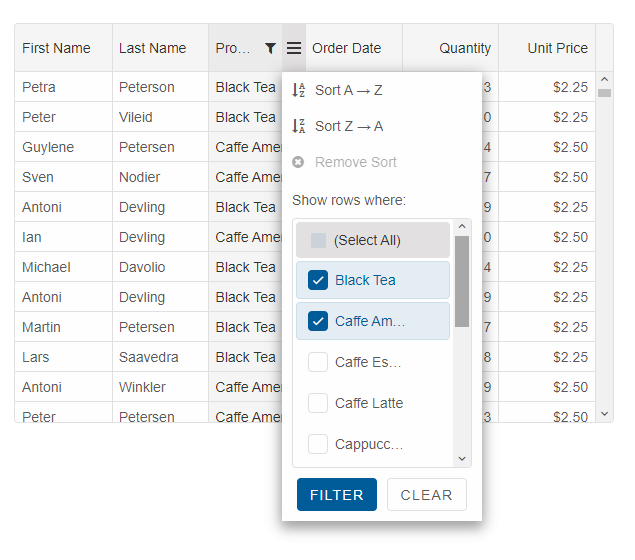
{ label: 'Product', dataField: 'productName', columnGroup: 'order', filterMenuMode: 'excel' }
Filter Groups
The filter group is an object used in the filtering process.
const filterGroup = new Smart.FilterGroup(); filterGroup.addFilter('or', filterGroup.createFilter('string', 'Andrew', 'EQUAL')); filterGroup.addFilter('or', filterGroup.createFilter('string', 'Nancy', 'EQUAL')); grid.addFilter('firstName', filterGroup);Let’s see how to use the filter group.
- The first step is to create a filter group. The filter group is a group of one or more filtering criterias. const filterGroup = new Smart.FilterGroup();
-
The second step is to create the filters. Each filter must have a filter value – this is the value we compare each cell value with. The filter condition specifies how the filter will compare each cell value with the filter value. The filter condition value depends on the filter’s type(the Grid supports string, numeric and date filters). If you want to get the list of the supported grid filtering conditions, you can use the ‘getConditions(filterType)’ method of filter group.The createFilter method is used to create the filter.
// possible conditions for string filter: 'EMPTY', 'NOT_EMPTY', 'CONTAINS', 'CONTAINS_CASE_SENSITIVE', // 'DOES_NOT_CONTAIN', 'DOES_NOT_CONTAIN_CASE_SENSITIVE', 'STARTS_WITH', 'STARTS_WITH_CASE_SENSITIVE', // 'ENDS_WITH', 'ENDS_WITH_CASE_SENSITIVE', 'EQUAL', 'EQUAL_CASE_SENSITIVE', 'NULL', 'NOT_NULL' // possible conditions for numeric filter: 'EQUAL', 'NOT_EQUAL', 'LESS_THAN', 'LESS_THAN_OR_EQUAL', 'GREATER_THAN', 'GREATER_THAN_OR_EQUAL', 'NULL', 'NOT_NULL' // possible conditions for date filter: 'EQUAL', 'NOT_EQUAL', 'LESS_THAN', 'LESS_THAN_OR_EQUAL', 'GREATER_THAN', 'GREATER_THAN_OR_EQUAL', 'NULL', 'NOT_NULL' const filter1 = filterGroup.createfilter('stringfilter', 'Beate', 'contains'); const filter2 = filterGroup.createfilter('stringfilter', 'Andrew', 'starts_with'); - The third step is to add the filters to the filter group. In the code example below, we add two filters in the filter group with operator ‘or’. This means that each cell value will be evaluated by filter1 and filter2 and the evaluation result will be true, if the filter1′s returned value is true or filter2′s returned value is true. filterGroup.addFilter('or', filter1); filterGroup.addFilter('or', filter2);
- In the final step, we add the filter group to the first column. // add the filters. grid.addFilter('firstName', filterGroup); If you want to remove the filter, call the ‘removefilter’ method and then the ‘applyfilters’ method. grid.removeFilter('firstName', filterGroup); If you want to clear all filters, use the ‘clearfilters’ method. grid.clearFilter();
Clear Filtering API
To clear the filtering, you can use theclearFilter()
method.
grid.clearFilter();
Filter by Column API
To filter by a column, using the column definition, you can use the column'sfilter
property. Example:
columns: [ { label: 'First Name', dataField: 'firstName', columnGroup: 'name', filter: 'starts_with An' }, { label: 'Last Name', dataField: 'lastName', columnGroup: 'name' }, { label: 'Product', dataField: 'productName', columnGroup: 'order' }, { label: 'Order Date', cellsFormat: 'MM/dd/yyyy', dataField: 'date', columnGroup: 'order' }, { label: 'Quantity', dataField: 'quantity', columnGroup: 'order' }, { label: 'Unit Price', dataField: 'price', cellsFormat: 'c2', columnGroup: 'order' } ]
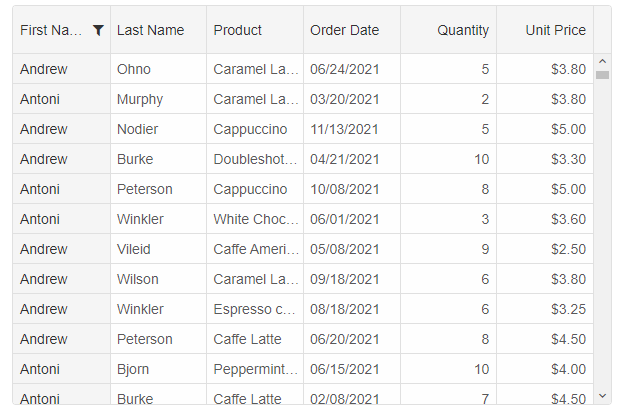
After the filter is set to the column, it is internally transformed to a FilterGroup object. You can then use the Column API to get the applied column filters:
const columnFilters = column.filter.filters;The column filters is an Array. Each item in the array has 'value', 'condition'. By using the column.filter, you can also get an array of the available filtering conditions.
const booleanConditions = column.filter.booleanConditions; const dateConditions = column.filter.dateConditions; const numericConditions = column.filter.numericConditions; const stringConditions = column.filter.stringConditions;
Custom Filtering
Custom Filter menu can be created by implementing two functions - 'updateFilterPanel' and 'getFilterPanel'. 'getFilterPanel' is called once when the filter menu is opened. The function should return HTMLElement which represents custom UI for filtering. We can then use the Grid API for applying filters e.g. column.filter = .... The other function - 'updateFilterPanel' is called when the filter menu is opened after the filter panel is created. It is used for updating the custom filter panel.The following source code shows how to implement the 'updateFilterPanel' and 'getFilterPanel' functions.
columns: [ { label: 'First Name', dataField: 'firstName', columnGroup: 'name', updateFilterPanel: (filterPanel, column) => { const input = filterPanel.querySelector('jqx-input'); if (column.filter) { input.value = column.filter.filters[0].value; } else { input.value = ''; } }, getFilterPanel: (column) => { // create filter panel. const panel = document.createElement('div'); panel.style.height = '150px'; panel.style.display = 'flex'; panel.style.flexDirection = 'column'; // create the label for the filter panel. const label = document.createElement('label'); label.innerHTML = 'Filter by:'; label.style.marginTop = '10px'; label.style.marginLeft = '10px'; // create filter input. const filterInput = document.createElement('jqx-input'); filterInput.style.width = '150px'; filterInput.style.marginTop = '10px'; filterInput.style.marginLeft = '10px'; // create button for applying a filter. const applyFilter = document.createElement('jqx-button'); applyFilter.style.marginTop = '20px'; applyFilter.style.marginLeft = '20px'; applyFilter.innerHTML = 'Apply'; applyFilter.style.width = '100px'; applyFilter.classList.add('primary'); // add the panel inputs. panel.appendChild(label); panel.appendChild(filterInput); panel.appendChild(applyFilter); // apply filter filterInput.onkeyup = (event) => { if (event.key === 'Enter') { column.filter = 'contains ' + filterInput.value; } } applyFilter.onclick = () => { column.filter = 'contains ' + filterInput.value; } return panel; } }, { label: 'Last Name', dataField: 'lastName', columnGroup: 'name' }, { label: 'Product', dataField: 'productName', columnGroup: 'order' }, { label: 'Order Date', cellsFormat: 'MM/dd/yyyy', dataField: 'date', columnGroup: 'order' }, { label: 'Quantity', dataField: 'quantity', columnGroup: 'order' }, { label: 'Unit Price', dataField: 'price', cellsFormat: 'c2', columnGroup: 'order' } ]
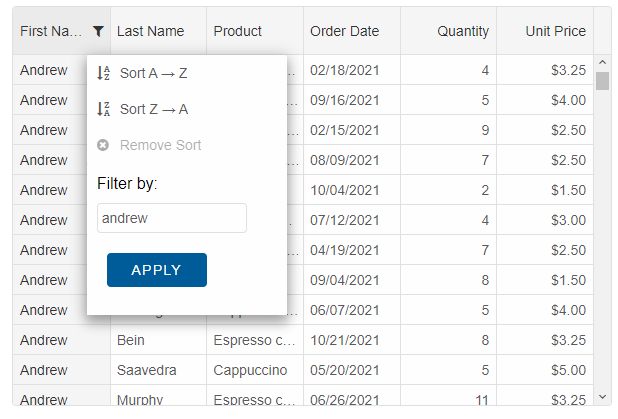
Continue reading about filtering Grid Filtering & Sorting