Smart.Button - configuration and usage
Overview
Smart.Button represents a simple button that can be clicked.
Getting Started with Button Web Component
Smart UI for Web Components is distributed as smart-webcomponents NPM package. You can also get the full download from our website with all demos from the Download page.Setup the Button
Smart UI for Web Components is distributed as smart-webcomponents NPM package
- Download and install the package.
npm install smart-webcomponents
- Once installed, import the Button module in your application.
<script type="module" src="node_modules/smart-webcomponents/source/modules/smart.button.js"></script>
-
Adding CSS reference
The smart.default.css CSS file should be referenced using following code.
<link rel="stylesheet" type="text/css" href="node_modules/smart-webcomponents/source/styles/smart.default.css" />
- Add the Button tag to your Web Page
<smart-button id="button"></smart-button>
- Create the Button Component
<script type="module"> Smart('#button', class { get properties() { return {"type":"submit"} } }); </script>
Another option is to create the Button is by using the traditional Javascript way:
const button = document.createElement('smart-button'); button.disabled = true; document.body.appendChild(button);
Smart framework provides a way to dynamically create a web component on demand from a DIV tag which is used as a host. The following imports the web component's module and creates it on demand, when the document is ready. The #button is the ID of a DIV tag.
import "../../source/modules/smart.button.js"; document.readyState === 'complete' ? init() : window.onload = init; function init() { const button = new Smart.Button('#button', {"type":"submit"}); }
- Open the page in your web server.
The following code adds a simple button to the page.
<!DOCTYPE html> <html lang="en"> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> </head> <body> <smart-button>Click me</smart-button> <script type="module" src="../../source/modules/smart.button.js"></script> </body> </html>
Note how smart.element.js and webcomponents.min.js are declared before everything else. This is mandatory for all custom elements.
Demo
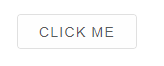
Appearance
If the user wants to change the content of the button, this can be accomplished by setting the innerHTML property of the element, like so:
<!DOCTYPE html> <html lang="en"> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> <script> window.onload = function () { document.querySelector('smart-button').innerHTML = 'Button 1'; } </script> </head> <body> <smart-button>Click Me</smart-button> <script type="module" src="../../source/modules/smart.button.js"></script> </body> </html>
Using the innerHTML property the user can change the content of the element dynamically as well.
Demo
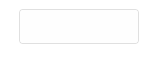
Behavior
By default the innerHTML of the button is an empty string.
The element offers multiple click modes:
- hover
- press
- release
- pressAndRelease
clickMode is a property of the button that can be changed either from the HTML tag by setting the attribute click-mode and assigning a new value to it or by following the earlier approach and change it dynamically via javascript during the onload stage of the window object or later.
Here's how to set a new click mode on element initialization:
<!DOCTYPE html> <html lang="en"> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> </head> <body> <smart-button click-mode="release">Click Me</smart-button> <script type="module" src="../../source/modules/smart.button.js"></script> </body> </html>
Demo
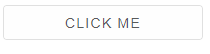
And here's how to change it via javascript after the element has been initialized:
<!DOCTYPE html> <html lang="en"> <head> <link rel="stylesheet" href="../../source/styles/smart.default.css" type="text/css" /> <script> window.onload = function () { document.querySelector('smart-button').clickMode = 'pressAndRelease'; } </script> </head> <body> <smart-button>Click Me</smart-button> <script type="module" src="../../source/modules/smart.button.js"></script> </body> </html>
Demo
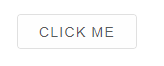
Create, Append, Remove, Get/Set Property, Invoke Method, Bind to Event
Create a new element:
const button = document.createElement('smart-button');
Append it to the DOM:
document.body.appendChild(button);
Remove it from the DOM:
button.parentNode.removeChild(button);
Set a property:
button.propertyName = propertyValue;
Get a property value:
const propertyValue = button.propertyName;
Invoke a method:
button.methodName(argument1, argument2);
Add Event Listener:
const eventHandler = (event) => { // your code here. }; button.addEventListener(eventName, eventHandler);
Remove Event Listener:
button.removeEventListener(eventName, eventHandler, true);
Using with Typescript
Smart Web Components package includes TypeScript definitions which enables strongly-typed access to the Smart UI Components and their configuration.
Inside the download package, the typescript directory contains .d.ts file for each web component and a smart.elements.d.ts typescript definitions file for all web components. Copy the typescript definitions file to your project and in your TypeScript file add a reference to smart.elements.d.ts
Read more about using Smart UI with Typescript.Getting Started with Angular Button Component
Setup Angular Environment
Angular provides the easiest way to set angular CLI projects using Angular CLI tool.
Install the CLI application globally to your machine.
npm install -g @angular/cli
Create a new Application
ng new smart-angular-button
Navigate to the created project folder
cd smart-angular-button
Setup the Button
Smart UI for Angular is distributed as smart-webcomponents-angular NPM package
- Download and install the package.
npm install smart-webcomponents-angular
- Once installed, import the ButtonModule in your application root or feature module.
app.module.ts
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { ButtonModule } from 'smart-webcomponents-angular/button'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, ButtonModule ], bootstrap: [ AppComponent ] }) export class AppModule { }
- Adding CSS reference
The following CSS file is available in ../node_modules/smart-webcomponents-angular/ package folder. This can be referenced in [src/styles.css] using following code.@import 'smart-webcomponents-angular/source/styles/smart.default.css';
Another way to achieve the same is to edit the angular.json file and in the styles add the style."styles": [ "node_modules/smart-webcomponents-angular/source/styles/smart.default.css" ]
-
Example
app.component.html
<div class="demo-horizontal-layout"> <div> <label>Default Buttons</label> <div class="demo-buttons-group"> <smart-button>Normal</smart-button> <smart-button #button class="raised">Raised</smart-button> <smart-button #button2 class="outlined">Outlined</smart-button> <smart-button #button3 class="flat">Flat</smart-button> <smart-button #button4 class="floating"><span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon"><i class="material-icons"></i></span> </smart-button> </div> </div> <div> <label>Primary Buttons</label> <div class="demo-buttons-group"> <smart-button #button5 class="primary">Normal</smart-button> <smart-button #button6 class="raised primary">Raised</smart-button> <smart-button #button7 class="outlined primary">Outlined</smart-button> <smart-button #button8 class="flat primary">Flat</smart-button> <smart-button #button9 class="floating primary"><span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon"><i class="material-icons"></i></span> </smart-button> </div> </div> <div> <label>Secondary Buttons</label> <div class="demo-buttons-group"> <smart-button #button10 class="secondary">Normal</smart-button> <smart-button #button11 class="raised secondary">Raised</smart-button> <smart-button #button12 class="outlined secondary">Outlined</smart-button> <smart-button #button13 class="flat secondary">Flat</smart-button> <smart-button #button14 class="floating secondary"><span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon"><i class="material-icons"></i></span> </smart-button> </div> </div> <div> <label>Success Buttons</label> <div class="demo-buttons-group"> <smart-button #button15 class="success">Normal</smart-button> <smart-button #button16 class="raised success">Raised</smart-button> <smart-button #button17 class="outlined success">Outlined</smart-button> <smart-button #button18 class="flat success">Flat</smart-button> <smart-button #button19 class="floating success"><span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon"><i class="material-icons"></i></span> </smart-button> </div> </div> <div> <label>Error Buttons</label> <div class="demo-buttons-group"> <smart-button #button20 class="error">Normal</smart-button> <smart-button #button21 class="raised error">Raised</smart-button> <smart-button #button22 class="outlined error">Outlined</smart-button> <smart-button #button23 class="flat error">Flat</smart-button> <smart-button #button24 class="floating error"><span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon"><i class="material-icons"></i></span> </smart-button> </div> </div> </div>
app.component.ts
import { Component, ViewChild, OnInit, AfterViewInit } from '@angular/core'; import { ButtonComponent } from 'smart-webcomponents-angular/button'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements AfterViewInit, OnInit { ngOnInit(): void { // onInit code. } ngAfterViewInit(): void { // afterViewInit code. this.init(); } init(): void { // init code. } }
app.module.ts
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { ButtonModule } from 'smart-webcomponents-angular/button'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, ButtonModule ], bootstrap: [ AppComponent ] }) export class AppModule { }
Running the Angular application
After completing the steps required to render a Button, run the following command to display the output in your web browser
ng serveand open localhost:4200 in your favorite web browser.
Read more about using Smart UI for Angular: https://www.htmlelements.com/docs/angular-cli/.
Getting Started with React Button Component
Setup React Environment
The easiest way to start with React is to use create-react-app. To scaffold your project structure, follow the installation instructions.
npx create-react-app my-app cd my-app npm start
Preparation
Open src/App.js andsrc/App.css
- Remove everything inside the App div tag in src/App.js:
<div className="App"> </div>
- Remove the logo.svg import
- Remove the contents of src/App.css
- Remove src/logo.svg
Setup the Button
Smart UI for React is distributed as smart-webcomponents-react NPM package
- Download and install the package.
npm install smart-webcomponents-react
Once installed, import the React Button Component and CSS files in your application and render it
app.js
import 'smart-webcomponents-react/source/styles/smart.default.css'; import React from "react"; import ReactDOM from 'react-dom/client'; import { Button, RepeatButton, ToggleButton, PowerButton } from 'smart-webcomponents-react/button'; class App extends React.Component { componentDidMount() { } render() { return ( <div> <div className="demo-horizontal-layout"> <div> <label>Default Buttons</label> <div className="demo-buttons-group"> <Button >Normal</Button> <Button className="raised">Raised</Button> <Button className="outlined">Outlined</Button> <Button className="flat">Flat</Button> <Button className="floating"><span className="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon"><i className="material-icons"></i></span> </Button> </div> </div> <div> <label>Primary Buttons</label> <div className="demo-buttons-group"> <Button className="primary">Normal</Button> <Button className="raised primary">Raised</Button> <Button className="outlined primary">Outlined</Button> <Button className="flat primary">Flat</Button> <Button className="floating primary"><span className="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon"><i className="material-icons"></i></span> </Button> </div> </div> <div> <label>Secondary Buttons</label> <div className="demo-buttons-group"> <Button className="secondary">Normal</Button> <Button className="raised secondary">Raised</Button> <Button className="outlined secondary">Outlined</Button> <Button className="flat secondary">Flat</Button> <Button className="floating secondary"><span className="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon"><i className="material-icons"></i></span> </Button> </div> </div> <div> <label>Success Buttons</label> <div className="demo-buttons-group"> <Button className="success">Normal</Button> <Button className="raised success">Raised</Button> <Button className="outlined success">Outlined</Button> <Button className="flat success">Flat</Button> <Button className="floating success"><span className="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon"><i className="material-icons"></i></span> </Button> </div> </div> <div> <label>Error Buttons</label> <div className="demo-buttons-group"> <Button className="error">Normal</Button> <Button className="raised error">Raised</Button> <Button className="outlined error">Outlined</Button> <Button className="flat error">Flat</Button> <Button className="floating error"><span className="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon"><i className="material-icons"></i></span> </Button> </div> </div> </div> </div> ); } } export default App;
Running the React application
Start the app withnpm startand open localhost:3000 in your favorite web browser to see the output.
Read more about using Smart UI for React: https://www.htmlelements.com/docs/react/.
Getting Started with Vue Button Component
Setup Vue Environment
We will use vue-cli to get started. Let's install vue-cli
npm install -g @vue/cli
Then we can start creating our Vue.js projects with:
vue create my-project
Setup the Button
Open the "my-project" folder and run:
npm install smart-webcomponents
Setup with Vue 3.x
-
Make Vue ignore custom elements defined outside of Vue (e.g., using the Web Components APIs). Otherwise, it will throw a warning about an Unknown custom element, assuming that you forgot to register a global component or misspelled a component name.
Open vite.config.js in your favorite text editor and change its contents to the following:
vite.config.js
import { fileURLToPath, URL } from 'node:url' import { defineConfig } from 'vite' import vue from '@vitejs/plugin-vue' // https://vitejs.dev/config/ export default defineConfig({ plugins: [ vue({ template: { compilerOptions: { isCustomElement: tag => tag.startsWith('smart-') } } }) ], resolve: { alias: { '@': fileURLToPath(new URL('./src', import.meta.url)) } } })
-
Open src/App.vue in your favorite text editor and change its contents to the following:
App.vue
<template> <div class="vue-root"> <div class="demo-horizontal-layout"> <div> <label>Default Buttons</label> <div class="demo-buttons-group"> <smart-button>Normal</smart-button> <smart-button class="raised">Raised</smart-button> <smart-button class="outlined">Outlined</smart-button> <smart-button class="flat">Flat</smart-button> <smart-button class="floating"> <span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon" > <i class="material-icons"></i> </span> </smart-button> </div> </div> <div> <label>Primary Buttons</label> <div class="demo-buttons-group"> <smart-button class="primary">Normal</smart-button> <smart-button class="raised primary">Raised</smart-button> <smart-button class="outlined primary">Outlined</smart-button> <smart-button class="flat primary">Flat</smart-button> <smart-button class="floating primary"> <span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon" > <i class="material-icons"></i> </span> </smart-button> </div> </div> <div> <label>Secondary Buttons</label> <div class="demo-buttons-group"> <smart-button class="secondary">Normal</smart-button> <smart-button class="raised secondary">Raised</smart-button> <smart-button class="outlined secondary">Outlined</smart-button> <smart-button class="flat secondary">Flat</smart-button> <smart-button class="floating secondary"> <span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon" > <i class="material-icons"></i> </span> </smart-button> </div> </div> <div> <label>Success Buttons</label> <div class="demo-buttons-group"> <smart-button class="success">Normal</smart-button> <smart-button class="raised success">Raised</smart-button> <smart-button class="outlined success">Outlined</smart-button> <smart-button class="flat success">Flat</smart-button> <smart-button class="floating success"> <span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon" > <i class="material-icons"></i> </span> </smart-button> </div> </div> <div> <label>Error Buttons</label> <div class="demo-buttons-group"> <smart-button class="error">Normal</smart-button> <smart-button class="raised error">Raised</smart-button> <smart-button class="outlined error">Outlined</smart-button> <smart-button class="flat error">Flat</smart-button> <smart-button class="floating error"> <span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon" > <i class="material-icons"></i> </span> </smart-button> </div> </div> </div> </div> </template> <script> import { onMounted } from "vue"; import "smart-webcomponents/source/styles/smart.default.css"; import "smart-webcomponents/source/modules/smart.button.js"; export default { name: "app", setup() { onMounted(() => {}); } }; </script> <style> .demo-horizontal-layout { display: flex; flex-direction: column; } .dark-mode .demo-icon { background-image: none; } label { font-size: 14px; } .demo-buttons-group { margin: 8px; } .demo-buttons-group smart-ui-button { margin: 8px; } .button-demo-icon { position: relative; left: -5px; } </style>
We can now use the smart-button with Vue 3. Data binding and event handlers will just work right out of the box.
Setup with Vue 2.x
-
Make Vue ignore custom elements defined outside of Vue (e.g., using the Web Components APIs). Otherwise, it will throw a warning about an Unknown custom element, assuming that you forgot to register a global component or misspelled a component name.
Open src/main.js in your favorite text editor and change its contents to the following:
main.js
import Vue from 'vue' import App from './App.vue' Vue.config.productionTip = false Vue.config.ignoredElements = [ 'smart-button' ] new Vue({ render: h => h(App), }).$mount('#app')
-
Open src/App.vue in your favorite text editor and change its contents to the following:
App.vue
<template> <div class="demo-horizontal-layout"> <div> <label>Default Buttons</label> <div class="demo-buttons-group"> <smart-button>Normal</smart-button> <smart-button class="raised">Raised</smart-button> <smart-button class="outlined">Outlined</smart-button> <smart-button class="flat">Flat</smart-button> <smart-button class="floating"> <span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon" ></span> </smart-button> </div> </div> <div> <label>Primary Buttons</label> <div class="demo-buttons-group"> <smart-button class="primary">Normal</smart-button> <smart-button class="raised primary">Raised</smart-button> <smart-button class="outlined primary">Outlined</smart-button> <smart-button class="flat primary">Flat</smart-button> <smart-button class="floating primary"> <span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon" ></span> </smart-button> </div> </div> <div> <label>Secondary Buttons</label> <div class="demo-buttons-group"> <smart-button class="secondary">Normal</smart-button> <smart-button class="raised secondary">Raised</smart-button> <smart-button class="outlined secondary">Outlined</smart-button> <smart-button class="flat secondary">Flat</smart-button> <smart-button class="floating secondary"> <span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon" ></span> </smart-button> </div> </div> <div> <label>Success Buttons</label> <div class="demo-buttons-group"> <smart-button class="success">Normal</smart-button> <smart-button class="raised success">Raised</smart-button> <smart-button class="outlined success">Outlined</smart-button> <smart-button class="flat success">Flat</smart-button> <smart-button class="floating success"> <span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon" ></span> </smart-button> </div> </div> <div> <label>Error Buttons</label> <div class="demo-buttons-group"> <smart-button class="error">Normal</smart-button> <smart-button class="raised error">Raised</smart-button> <smart-button class="outlined error">Outlined</smart-button> <smart-button class="flat error">Flat</smart-button> <smart-button class="floating error"> <span class="button-demo-icon demo-icon demo-device-icon-phone-portrait demo-button-demo-icon" ></span> </smart-button> </div> </div> </div> </template> <script> import 'smart-webcomponents/source/modules/smart.button.js'; import "smart-webcomponents/source/styles/smart.default.css"; export default { name: "app" }; </script> <style> .demo-horizontal-layout { display: flex; flex-direction: column; } .dark-mode .demo-icon { background-image: none; } label { font-size: 14px; } .demo-buttons-group { margin: 8px; } .demo-buttons-group smart-button { margin: 8px; } .button-demo-icon { position: relative; left: -5px; } </style>
We can now use the smart-button with Vue. Data binding and event handlers will just work right out of the box.
We have bound the properties of the smart-button to values in our Vue component.
Running the Vue application
Start the app withnpm run devand open localhost:8080 in your favorite web browser to see the output below:
Read more about using Smart UI for Vue: https://www.htmlelements.com/docs/vue/.
Keyboard Support
Smart.Button follows HTML button's keyboard navigation behavior. This means that the button can be selected using Space. The element is focusable and can be focused using the Tab button.