Build your web apps using Smart Custom Elements
Smart.Scheduler - Events Import/Export
Events
Smart.Scheduler Events are defined as objects. Each object has specific attributes that surve a purpose and most of them are validated strictly by the element. However the Smart.Scheduler allows setting any other custom attributes to the Events and will always return them on export.
The dataSource property of the Smart.Scheduler accepts an Array of event objects or a Smart.DataAdapter instance. When set to a DataAdapter instance it is possible to load Scheduler events from external data sources.
Exporting Events
Exporting Smart.Scheduler events is possible via the exportData method. However there's an additional dataExport property which allows to customize the exported file. It's a hierarchical property with the following sub-properties:
Property | Type | Description |
---|---|---|
header | boolean | Determines whether the Header row should be included in the exported file or not. By default a header row is included at the top of the exported table for xlsx, pdf and html export. This is not applicable to 'ical' export. |
columns | array | Determines which properties of the events will be exported as table columns. By default the exported columns include: 'id', 'label', 'description', 'dateStart', 'dateEnd'. |
style | object | Defines custom styles for the exported table. Check the official API documentation for Smart.DataExporter for more information. |
fileName | string | Determines the name of the exported file. By default the node name of the element is used to name the file. |
pageOrientation | string | Determines the page orientation of the exported table. Possible values are 'portrait' or 'landscape'. |
Here's an example on how to change the name of the exported file:
const scheduler = document.querySelector('smart-scheduler'); scheduler.dataExport.fileName = 'myEvents';
The dataExport method takes two arguments: exportData(dataFormat: string, callback?: Funtion)
- dataFormat - a string that determines the format of the exported file.
- callback - Optional parameter. Defines a callback that will be called with the output before it is returned by the Smart.DataExporter. Useful when the user wants to modify the file before download.
There's a Smart.Scheduler export demo on the website that we are going to use as a reference.
Here's the code of the demo:
const today = new Date(), currentDate = today.getDate(), currentYear = today.getFullYear(), currentMonth = today.getMonth(), data = [ { label: 'Website Re-Design Plan', dateStart: new Date(currentYear, currentMonth, currentDate, 9, 30), dateEnd: new Date(currentYear, currentMonth, currentDate, 11, 30) }, { label: 'Book Flights to San Fran for Sales Trip', priorityId: 1, dateStart: new Date(currentYear, currentMonth, currentDate + 3, today.getHours() + 1, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 3, today.getHours() + 3, 0), notifications: [ { interval: 0, type: 'days', time: [today.getHours(), today.getMinutes() + 4], message: 'Book Flights to San Fran for Sales Trip Early' }, { interval: 0, type: 'weeks', time: [today.getHours(), today.getMinutes() + 5], message: 'Book Flights to San Fran for Sales Trip Late' } ] }, { label: 'Install New Router in Dev Room', priorityId: 1, dateStart: new Date(currentYear, currentMonth, currentDate, 13), dateEnd: new Date(currentYear, currentMonth, currentDate, 15, 30), repeat: { repeatFreq: 'monthly', repeatInterval: 1, repeatOn: today.getDate() } }, { label: 'Google AdWords Strategy', dateStart: new Date(currentYear, currentMonth, 10), dateEnd: new Date(currentYear, currentMonth, 11), allDay: true, backgroundColor: '#F57F17', repeat: { repeatFreq: 'weekly', repeatInterval: 5, repeatOn: [0, 2, 5], repeatEnd: new Date(currentYear, currentMonth + 2, 24), exceptions: [ { date: new Date(currentYear, currentMonth, 13), dateStart: new Date(currentYear, currentMonth, 14), label: 'Google AdWords Strategy', backgroundColor: '#F57F17' } ] } }, { label: 'Approve Personal Computer Upgrade Plan', priorityId: 2, dateStart: new Date(currentYear, currentMonth, currentDate + 1, 10, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 1, 11, 0) }, { label: 'Final Budget Review', priorityId: 2, dateStart: new Date(currentYear, currentMonth, currentDate + 1, 12, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 1, 13, 35) }, { label: 'New Brochures', priorityId: 2, dateStart: new Date(currentYear, currentMonth, currentDate, 13, 0), dateEnd: new Date(currentYear, currentMonth, currentDate, 15, 15) }, { label: 'Install New Database', priorityId: 1, dateStart: new Date(currentYear, currentMonth, currentDate + 1, 9), dateEnd: new Date(currentYear, currentMonth, currentDate + 1, 12, 15) }, { label: 'Approve New Online Marketing Strategy', priorityId: 2, dateStart: new Date(currentYear, currentMonth, currentDate + 2, 12, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 2, 14, 0) }, { label: 'Upgrade Personal Computers', priorityId: 1, dateStart: new Date(currentYear, currentMonth, currentDate, 9), dateEnd: new Date(currentYear, currentMonth, currentDate, 11, 30) } ]; const priorityData = [ { label: 'Low Priority', id: 1, backgroundColor: '#1e90ff' }, { label: 'Medium Priority', id: 2, backgroundColor: '#ff9747' } ]; window.Smart('#scheduler', class { get properties() { return { //Properties view: 'week', dataSource: data, views: ['day', 'week', 'month', 'timelineDay', 'timelineWeek', 'timelineMonth', 'agenda'], hourStart: 7, hourEnd: 19, firstDayOfWeek: 1, resources: [ { label: 'Priority', value: 'priorityId', dataSource: priorityData } ] }; } }); window.onload = function () { const scheduler = document.querySelector('smart-scheduler'); document.getElementById('exportToXLSX').addEventListener('click', () => scheduler.exportData('xlsx')); document.getElementById('exportToPDF').addEventListener('click', () => scheduler.exportData('pdf')); document.getElementById('exportToHTML').addEventListener('click', () => scheduler.exportData('html')); document.getElementById('exportToICal').addEventListener('click', () => scheduler.exportData('iCal')); document.getElementById('print').addEventListener('click', () => scheduler.print()); };
Demo
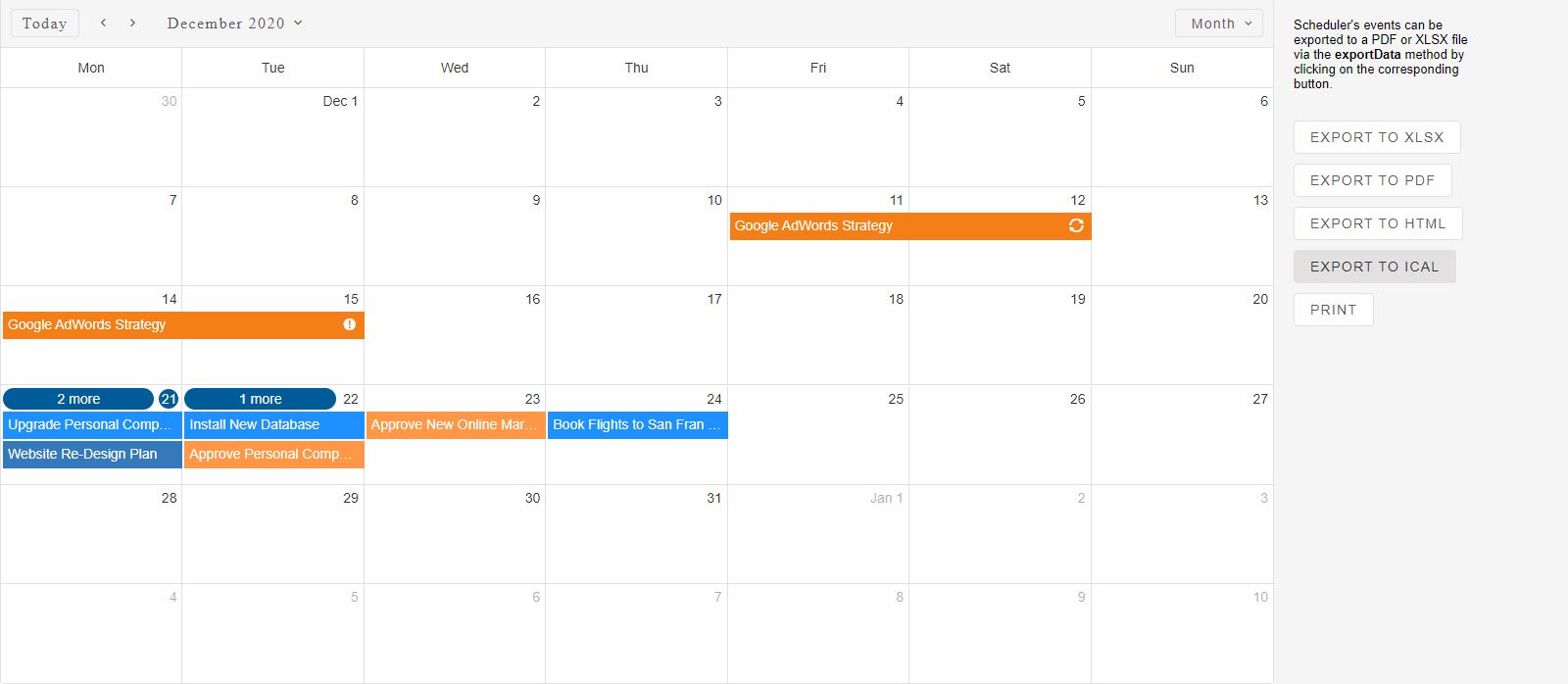
When the user clicks on one of the Export buttons on the right side of the demo the corresponding action will be executed and the expected file will be automatically downloaded.
An additional print method is also available wihch creates a Print Preview of the Scheduler.
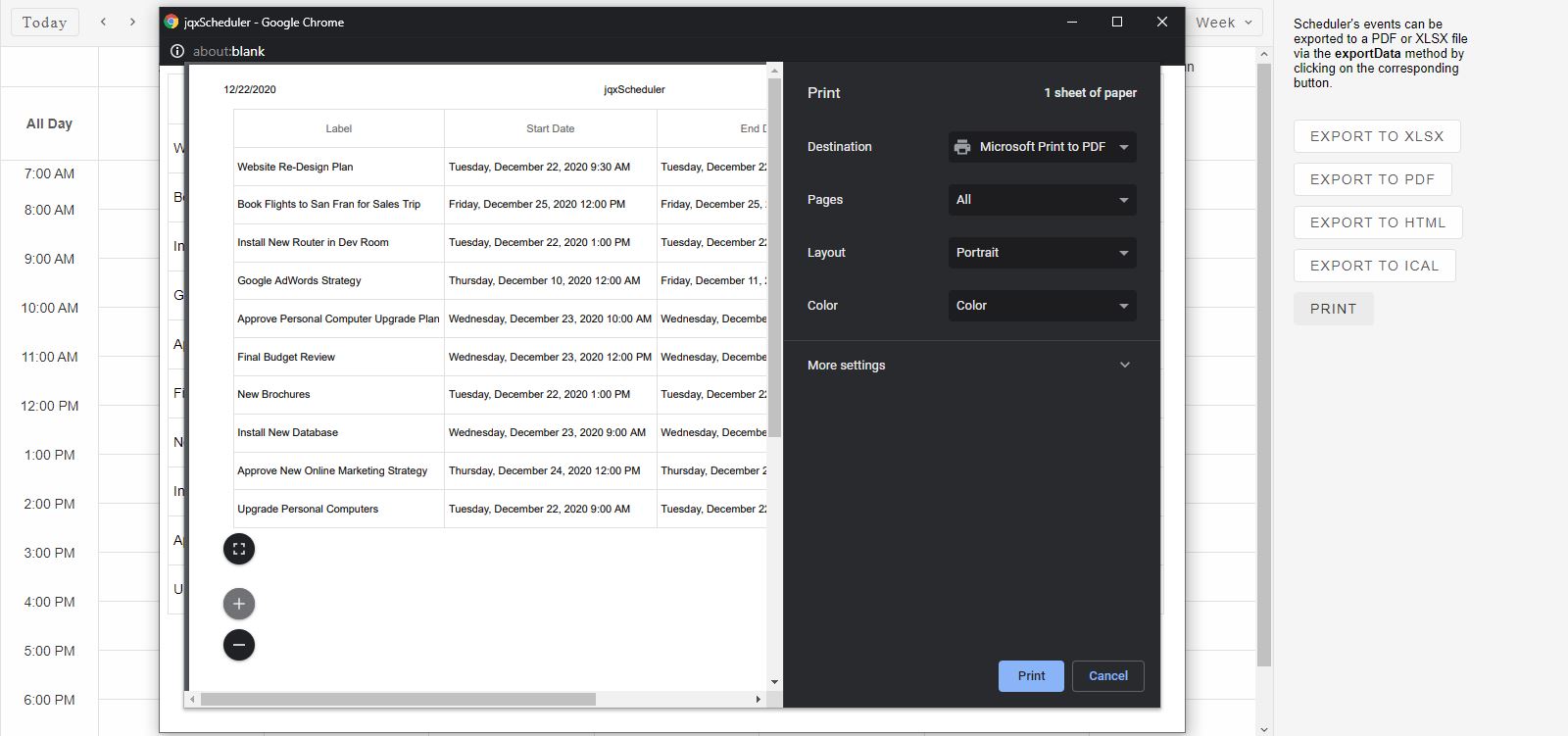
Importing Events
Importing Events to Smart.Scheduler can be achieved thanks to the Smart.DataAdapter component. The dataSource property accepts a Smart.DataAdapter instance which allows to define the dataSource and dataFields that are expected.
Here's an example on how to load Scheduler Events from a JSON file:
First we create a JSON file in the same diretory as the demo with the following content:
[ { "label": "Website Re-Design Plan", "dateStart": "2020-12-22T07:30:00.000Z", "dateEnd": "2020-12-22T10:30:00.000Z", "allDay": false }, { "label": "Book Flights to San Fran for Sales Trip", "priorityId": 1, "dateStart": "2020-12-25T07:00:00.000Z", "dateEnd": "2020-12-25T10:00:00.000Z", "notifications": [ { "interval": 0, "type": "days", "time": [ 8, 47 ], "message": "Book Flights to San Fran for Sales Trip Early" }, { "interval": 0, "type": "weeks", "time": [ 8, 48 ], "message": "Book Flights to San Fran for Sales Trip Late" } ], "allDay": false }, { "label": "Install New Router in Dev Room", "priorityId": 1, "dateStart": "2020-12-22T11:00:00.000Z", "dateEnd": "2020-12-22T14:30:00.000Z", "repeat": { "repeatFreq": "monthly", "repeatInterval": 1, "repeatOn": 22 }, "allDay": false }, { "label": "Google AdWords Strategy", "dateStart": "2020-12-09T22:00:00.000Z", "dateEnd": "2020-12-11T21:59:59.999Z", "allDay": true, "backgroundColor": "#F57F17", "repeat": { "repeatFreq": "weekly", "repeatInterval": 5, "repeatOn": [ 0, 2, 5 ], "repeatEnd": "2021-02-23T22:00:00.000Z", "exceptions": [ { "date": "2020-12-12T22:00:00.000Z", "dateStart": "2020-12-13T22:00:00.000Z", "label": "Google AdWords Strategy", "backgroundColor": "#F57F17", "dateEnd": "2020-12-15T21:59:59.999Z" } ] } }, { "label": "Approve Personal Computer Upgrade Plan", "priorityId": 2, "dateStart": "2020-12-23T08:00:00.000Z", "dateEnd": "2020-12-23T10:00:00.000Z", "allDay": false }, { "label": "Final Budget Review", "priorityId": 2, "dateStart": "2020-12-23T10:00:00.000Z", "dateEnd": "2020-12-23T12:35:00.000Z", "allDay": false }, { "label": "New Brochures", "priorityId": 2, "dateStart": "2020-12-22T11:00:00.000Z", "dateEnd": "2020-12-22T14:15:00.000Z", "allDay": false }, { "label": "Install New Database", "priorityId": 1, "dateStart": "2020-12-23T07:00:00.000Z", "dateEnd": "2020-12-23T11:15:00.000Z", "allDay": false }, { "label": "Approve New Online Marketing Strategy", "priorityId": 2, "dateStart": "2020-12-24T10:00:00.000Z", "dateEnd": "2020-12-24T13:00:00.000Z", "allDay": false }, { "label": "Upgrade Personal Computers", "priorityId": 1, "dateStart": "2020-12-22T07:00:00.000Z", "dateEnd": "2020-12-22T10:30:00.000Z", "allDay": false } ]
Next we setup the Smart.Scheduler to import that file via the following configuration:
const dataSource = new window.Smart.DataAdapter({ dataSource: './events.json', dataSourceType: 'json', dataFields: [ { name: 'dateStart', dataType: 'date' }, { name: 'dateEnd', dataType: 'date' }, { name: 'label', dataType: 'string' }, { name: 'allDay', dataType: 'boolean' }, { name: 'priorityId', dataType: 'any' }, { name: 'repeat', dataType: 'any' }, { name: 'notifications', dataType: 'any' }, { name: 'backgroundColor', dataType: 'string' } ] });
We have to define the dataFields for the events that are going to be used by the Smart.Scheduler and their approriate types.
More information on field mapping and etc for the Smart.DataAdapter can be found on the website.
Here's the expected result:
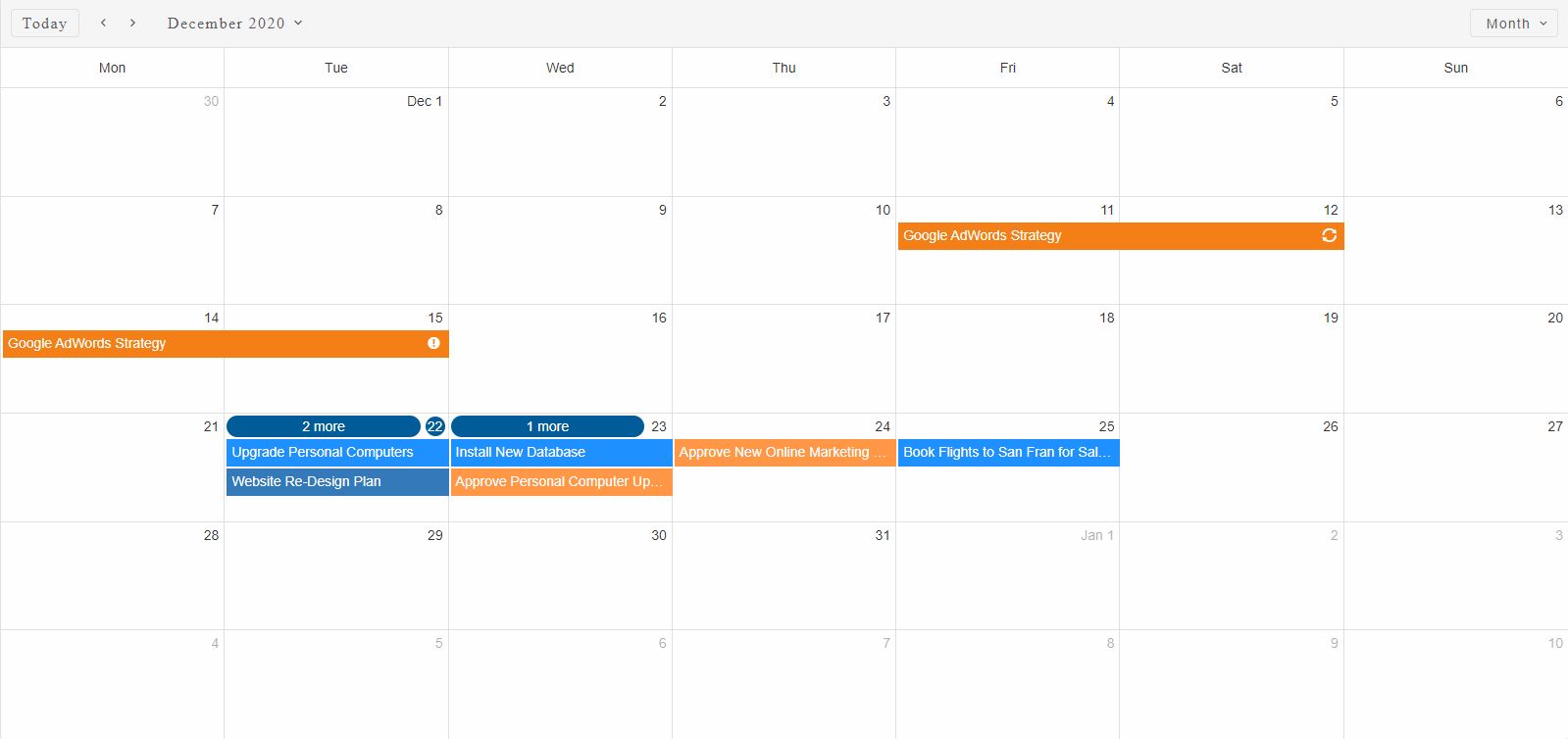
There's a dedicated topic on the website about Importing Events from iCal files.