Columns Cells Format
Smart.Grid allows you to apply formatting to the columns with number, date & time values using the CellsFormat
property.
Number Formatting
Number values can be formatted as decimal d
, floating-point f
,
integer n
, currency c
and percentage p
numbers
Additionaly, you can set the decimal places of the numbers by adding a number at the end of the formatting string
currency with 2 decimal places -> 'c2'
Date & Time Formatting
Date and Time values can be formatted by either using the built-in Date formats:
d
- short date pattern - 'M/d/yyyy'D
- long date pattern - 'dddd, MMMM dd, yyyy't
- short time pattern - 'h:mm tt'T
- long time pattern - 'h:mm:ss tt'f
- long date, short time pattern - 'dddd, MMMM dd, yyyy h:mm tt'F
- long date, long time pattern - 'dddd, MMMM dd, yyyy h:mm:ss tt'M
- month/day pattern - 'MMMM dd'Y
- month/year pattern - 'yyyy MMMM'S
- sortable format that does not vary by culture - 'yyyy'-'MM'-'dd'T'HH':'mm':'ss'
d
- the day of the month ->1
,15
dd
- the day of the month using 2-digit format ->01
,15
ddd
- the abbreviated name of the day of the week ->Mon
,Tue
dddd
- the full name of the day of the week ->Monday
,Tuesday
h
- the hour, using a 12-hour clock from 1 to 12 ->04
,10
hh
- the hour, using a 12-hour clock from 01 to 12 ->4
,10
H
- the hour, using a 24-hour clock from 0 to 23 ->5
,23
HH
- the hour, using a 24-hour clock from 00 to 23 ->05
,23
m
- the minutes, from 0 through 59 ->6
,45
mm
- the minutes, from 00 through 59 ->06
,45
M
- the month, from 1 through 12 ->3
,12
MM
- the month, from 01 through 12 ->03
,12
MMM
- the abbreviated name of the month ->Jun
,Nov
MMMM
- the full name of the month ->June
,November
s
- the seconds ->5
,30
ss
- the seconds ->05
,30
t
-the first character of the AM/PM designator ->A
,P
tt
- the AM/PM designator ->AM
,PM
y
- the year, from 0 to 99 ->5
,21
yy
- the year, from 00 to 99 ->05
,21
yyy
- the year, with a minimum of three digits ->040
,800
,2021
yyyy
- the year as a four-digit number ->0040
,0800
,1450
,2021
yyyy
- the year as a five-digit number ->00040
,0800
,02021
The following Grid example showcases some of the cell formatting options:
<Grid DataSource="dataRecords"> <Columns> <Column DataField="Num1" Label="Percent" DataType="number" CellsFormat="p0"></Column> <Column DataField="Num2" Label="Currency" DataType="number" CellsFormat="c2"></Column> <Column DataField="Num3" Label="Exponential" DataType="number" CellsFormat="e"></Column> <Column DataField="Num4" Label="Scientific" DataType="number" CellsFormat="s"></Column> <Column DataField="Date1" Label="Date" DataType="date" CellsFormat="dd/MM/yyyy"></Column> <Column DataField="Date2" Label="Date/Time" DataType="date" CellsFormat="F"></Column> </Columns> </Grid> @code{ private List<IDictionary<string, object>> dataRecords = new List<IDictionary<string, object>>() { new Dictionary<string, object>() { { "Num1", 38 }, { "Num2", 272 }, { "Num3", 411403131 }, { "Num4", 585940046 }, { "Date1", "9/17/2020" }, { "Date2", "2019-04-14T09:44:10Z" } }, new Dictionary<string, object>() { { "Num1", 58 }, { "Num2", 5940 }, { "Num3", 9369865 }, { "Num4", 966976265 }, { "Date1", "4/29/2020" }, { "Date2", "2019-07-22T23:33:34Z" } }, new Dictionary<string, object>() { { "Num1", 92 }, { "Num2", 4053 }, { "Num3", 113479426 }, { "Num4", 744238454 }, { "Date1", "3/5/2020" }, { "Date2", "2019-08-29T21:54:22Z" } }, new Dictionary<string, object>() { { "Num1", 92 }, { "Num2", 150 }, { "Num3", 717604578 }, { "Num4", 944555499 }, { "Date1", "9/24/2019" }, { "Date2", "2019-03-23T14:57:24Z" } } }; }
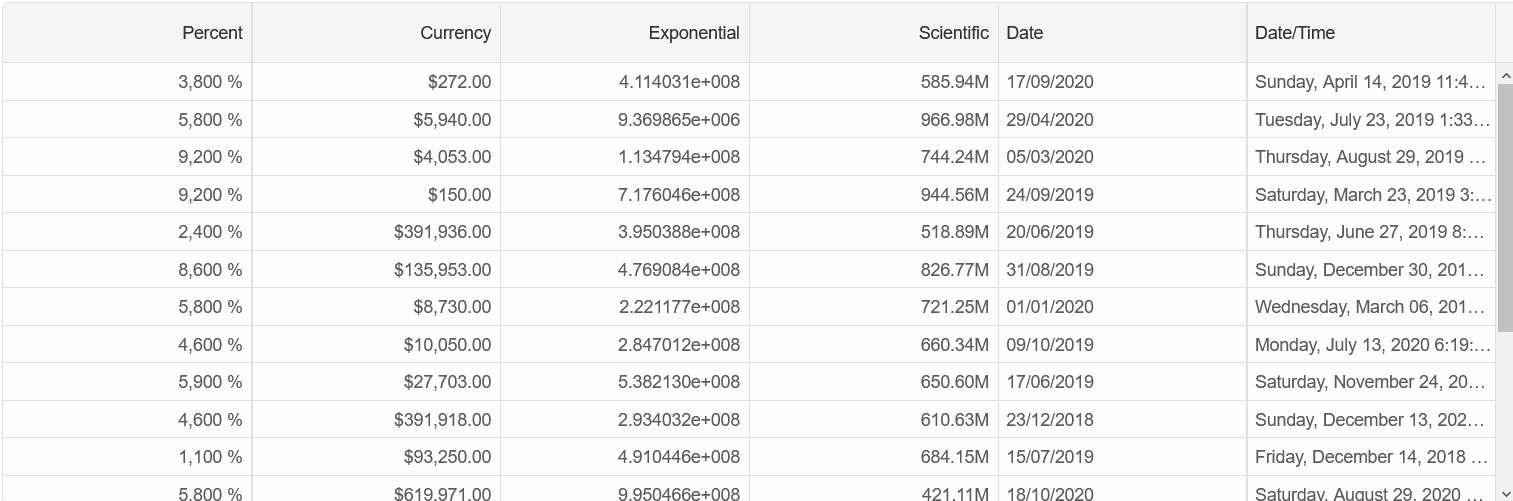
Conditional Formatting
Conditional Formatting allows you to apply custom formatting rules based on the value inside each cell.
To enable Conditional Formatting, establish the formatting rules inside an array of GridConditionalFormatting
.
Then set it as a property of the Grid.
<Grid DataSource="dataRecords" ConditionalFormatting="@conditionalFormatting"> .... </Grid> @code{ IList<GridConditionalFormatting> conditionalFormatting = new List<GridConditionalFormatting>() { new GridConditionalFormatting() { Column = "Num2", Condition = GridConditionalFormattingCondition.GreaterThan, FirstValue = 5000, Text = "#FFFFFF", Highlight = "#FB6304" }, new GridConditionalFormatting() { Column = "Num2", Condition = GridConditionalFormattingCondition.Between, FirstValue = 1000, SecondValue = 5000, Text = "#FFFFFF", Highlight = "#37C145" }, new GridConditionalFormatting() { Column = "Num2", Condition = GridConditionalFormattingCondition.LessThan, FirstValue = 300, Text = "#FFFFFF", Highlight = "#0C88DA" } }; }
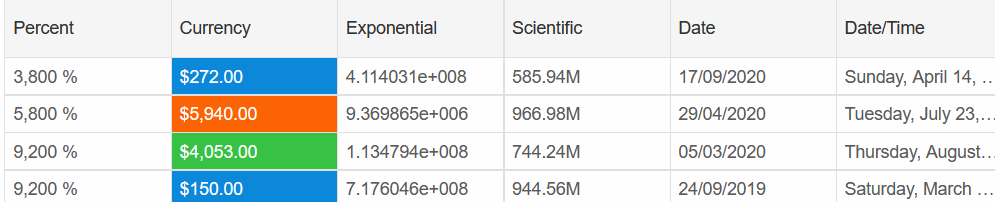