Grid Row Height
By default the Grid displays all rows with a height of 30px. It is defined by the --smart-grid-row-height CSS variable i.e you can change the row height by setting a new value to this variable. It is possible to change the row height by setting thelayout.rowHeight
property, too.
Example:
const gridOptions = { dataSourceSettings: { dataFields: [ { name: 'firstName', dataType: 'string' }, { name: 'lastName', dataType: 'string' }, { name: 'productName', map: 'product.name', dataType: 'string' }, { name: 'quantity', map: 'product.quantity', dataType: 'number' }, { name: 'price', map: 'product.price', dataType: 'number' }, { name: 'total', map: 'product.total', dataType: 'number' } ] }, behavior: { columnResizeMode: 'growAndShrink' }, sorting: { enabled: true }, dataSource: [ { firstName: 'Andrew', lastName: 'Burke', product: { name: 'Ice Coffee', price: 10, quantity: 3, total: 30 } }, { firstName: 'Petra', lastName: 'Williams', product: { name: 'Espresso', price: 7, quantity: 5, total: 35 } }, { firstName: 'Anthony', lastName: 'Baker', product: { name: 'Frappucino', price: 6, quantity: 4, total: 24 } } ], layout: { rowHeight: 50 }, columns: [ { label: 'First Name', dataField: 'firstName', cellsVerticalAlign: 'center' }, { label: 'Last Name', dataField: 'lastName' }, { label: 'Product', dataField: 'productName' }, { label: 'Quantity', dataField: 'quantity', cellsAlign: 'right' }, { label: 'Unit Price', dataField: 'price', cellsAlign: 'right', cellsFormat: 'c2' } ] }
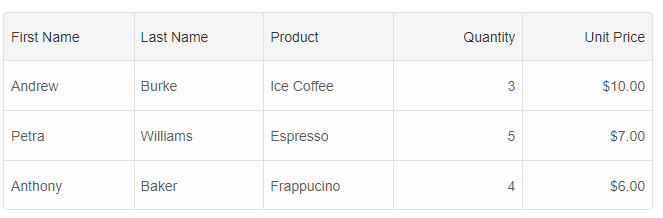
Custom Row Height
Each row may have a different row height. You can use therow.height
property to set a new height to the row. In the following example, we will show
how to set a row height, during the row initialization process.
const gridOptions = { dataSourceSettings: { dataFields: [ { name: 'firstName', dataType: 'string' }, { name: 'lastName', dataType: 'string' }, { name: 'productName', map: 'product.name', dataType: 'string' }, { name: 'quantity', map: 'product.quantity', dataType: 'number' }, { name: 'price', map: 'product.price', dataType: 'number' }, { name: 'total', map: 'product.total', dataType: 'number' } ] }, behavior: { columnResizeMode: 'growAndShrink' }, sorting: { enabled: true }, dataSource: [ { firstName: 'Andrew', lastName: 'Burke', product: { name: 'Ice Coffee', price: 10, quantity: 3, total: 30 } }, { firstName: 'Petra', lastName: 'Williams', product: { name: 'Espresso', price: 7, quantity: 5, total: 35 } }, { firstName: 'Anthony', lastName: 'Baker', product: { name: 'Frappucino', price: 6, quantity: 4, total: 24 } } ], onRowInit: (index, row) => { if (index === 0) { row.height = 35; } else if (index === 1) { row.height = 50; } else if (index === 2) { row.height = 80; } else row.height = 50; }, columns: [ { label: 'First Name', dataField: 'firstName', cellsVerticalAlign: 'center' }, { label: 'Last Name', dataField: 'lastName' }, { label: 'Product', dataField: 'productName' }, { label: 'Quantity', dataField: 'quantity', cellsAlign: 'right' }, { label: 'Unit Price', dataField: 'price', cellsAlign: 'right', cellsFormat: 'c2' } ] }
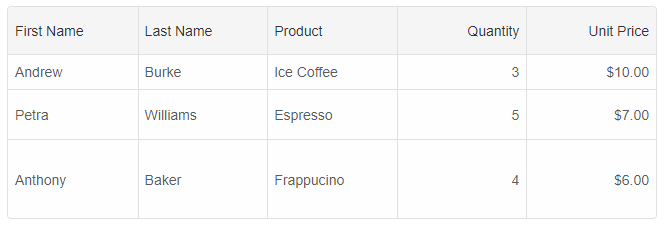
Cells Text Wrapping and auto row height
By setting the layout.rowHeight property to 'auto' and layout.allowCellsWrap property to true, the text inside rows will wrap and the rows will auto-resize.layout: { allowCellsWrap: true, rowHeight: 'auto' }
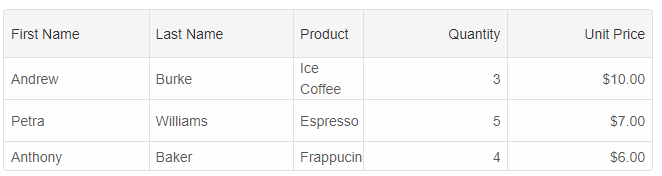