Build your web apps using Smart Custom Elements
Smart.Scheduler - Resources & Grouping
Resources & Groups
Resources and Groups are two separate properties of the Smart.Scheduler.
Resources are assigned to Events and can be used for styling.
Smart.Scheduler grouping is based on resources. When the groups property is set to a resource the view is created in order to show only events that have the resource assigned to them.
The official Smart.Scheduler demos from the HTMLElement website will be used for demonstration purposes in this topic.
Resources
Smart.Scheduler Resources are defined via the resources property. It accepts an array of objects. Each object defines a single resource.
Resources have the following properties:
Property | Type | Description | Optional |
---|---|---|---|
label | string | The name of the resource that will appear when necessary. | no |
value | string | The unique value of the resource. This value will be used to assign the resource to an event. | no |
dataSource | array | Defines the items for the resource. | no |
A resource item represents an object with the following attributes:
Property | Type | Description | Optional |
---|---|---|---|
id | string | number | The unique id of the resource item. The id is used to assign a resource item to the resource of a Scheduler Event. | no |
label | string | The name of the resource item that will appear in the Scheduler timeline. | no |
backgroundColor | string | The HEX representation of a color that will be used as the default background color for Scheduler Events that have the resource item assigned. | yes |
color | string | A HEX representation of a color that will be used as the default text color for the Scheduler events that have the resource item assigned. | yes |
A resource can have more then one items.
Resources are assigned to Scheduler Events as key-value pairs. Where the key represents the value property of the resources. The value in the pair represents the id of the resource item.
Here's how to define a resource:
const today = new Date(), currentDate = today.getDate(), currentYear = today.getFullYear(), currentMonth = today.getMonth(); const dataWithGroups = [ { label: 'Website Re-Design Plan', priorityId: 1, employeeId: 1, dateStart: new Date(currentYear, currentMonth, currentDate - 2, 9, 30), dateEnd: new Date(currentYear, currentMonth, currentDate - 2, 11, 30) }, { label: 'Book Flights to San Fran for Sales Trip', priorityId: 2, employeeId: 2, dateStart: new Date(currentYear, currentMonth, currentDate - 1, 10, 0), dateEnd: new Date(currentYear, currentMonth, currentDate - 1, 12, 0), }, { label: 'Install New Router in Conference Room', priorityId: 2, employeeId: 1, dateStart: new Date(currentYear, currentMonth, currentDate - 2, 12), dateEnd: new Date(currentYear, currentMonth, currentDate - 2, 14, 30) }, { label: 'Add a new desk to the Dev Room', priorityId: 1, employeeId: 2, dateStart: new Date(currentYear, currentMonth, currentDate - 1, 12, 30), dateEnd: new Date(currentYear, currentMonth, currentDate - 1, 14, 45) }, { label: 'Install New Router in Dev Room', priorityId: 2, employeeId: 1, dateStart: new Date(currentYear, currentMonth, currentDate, 13), dateEnd: new Date(currentYear, currentMonth, currentDate, 15, 30) }, { label: 'Approve Personal Computer Upgrade Plan', priorityId: 2, employeeId: 2, dateStart: new Date(currentYear, currentMonth, currentDate + 1, 10, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 1, 11, 0) }, { label: 'Final Budget Review', priorityId: 2, employeeId: 1, dateStart: new Date(currentYear, currentMonth, currentDate + 1, 12, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 1, 13, 35) }, { label: 'Old Brochures', priorityId: 2, employeeId: 1, dateStart: new Date(currentYear, currentMonth, currentDate, 13, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 1, 15, 15) }, { label: 'New Brochures', priorityId: 2, employeeId: 2, dateStart: new Date(currentYear, currentMonth, currentDate + 1, 13, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 2, 15, 15) }, { label: 'Install New Database', priorityId: 1, employeeId: 2, dateStart: new Date(currentYear, currentMonth, currentDate + 1, 9), dateEnd: new Date(currentYear, currentMonth, currentDate + 1, 12, 15) }, { label: 'Approve New Online Marketing Strategy', priorityId: 2, employeeId: 1, dateStart: new Date(currentYear, currentMonth, currentDate + 2, 12, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 2, 14, 0) }, { label: 'Upgrade Personal Computers', priorityId: 1, employeeId: 1, dateStart: new Date(currentYear, currentMonth, currentDate, 9), dateEnd: new Date(currentYear, currentMonth, currentDate, 11, 30) }, { label: 'Prepare current Year Marketing Plan', priorityId: 2, employeeId: 1, dateStart: new Date(currentYear, currentMonth, currentDate + 3, 11, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 3, 13, 30) }, { label: 'Prepare current Year Marketing Plan', priorityId: 2, employeeId: 1, dateStart: new Date(currentYear, currentMonth, currentDate + 4, 11, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 4, 13, 30) } ]; const priorityData = [ { label: 'Low Priority', id: 1, backgroundColor: '#1e90ff' }, { label: 'Medium Priority', id: 2, backgroundColor: '#ff9747' } ]; const employees = [ { label: 'Andrew Heart', id: 1, age: 28, discipline: 'ABS, Fitball, StepFit', backgroundColor: '#28a745' }, { label: 'Nancy Johnson', id: 2, age: 31, discipline: 'Tennis, Yoga, Swimming', backgroundColor: '#8f73af' } ]; window.Smart('#scheduler', class { get properties() { return { //Properties view: 'workWeek', dataSource: dataWithGroups, views: [ { label: 'Work Week', value: 'workWeek', type: 'week', hideWeekend: true }, 'month' ], timelineDayScale: 'quarterHour', hourStart: 7, hourEnd: 17, firstDayOfWeek: 1, resources: [ { label: 'Priority', value: 'priorityId', dataSource: priorityData, }, { label: 'Employee', value: 'employeeId', dataSource: employees } ], }; } });
Here we define a Smart.Scheduler with two resources each of which has two additional items.
Notice that the events can have more than one resource assigned to them. Here's an example on how to assign resources to an event:
{ label: 'Prepare current Year Marketing Plan', priorityId: 2, employeeId: 1, dateStart: new Date(currentYear, currentMonth, currentDate + 4, 11, 0), dateEnd: new Date(currentYear, currentMonth, currentDate + 4, 13, 30) }
The order of the resources in the resources property determines their priority.
Since the events have more then one resource assigned the backgroundColor of the first resource in the resources property takes precedens over the rest and the events will be colored using it's settings.
But when the order of the resources in the resources property is changed:
Demo
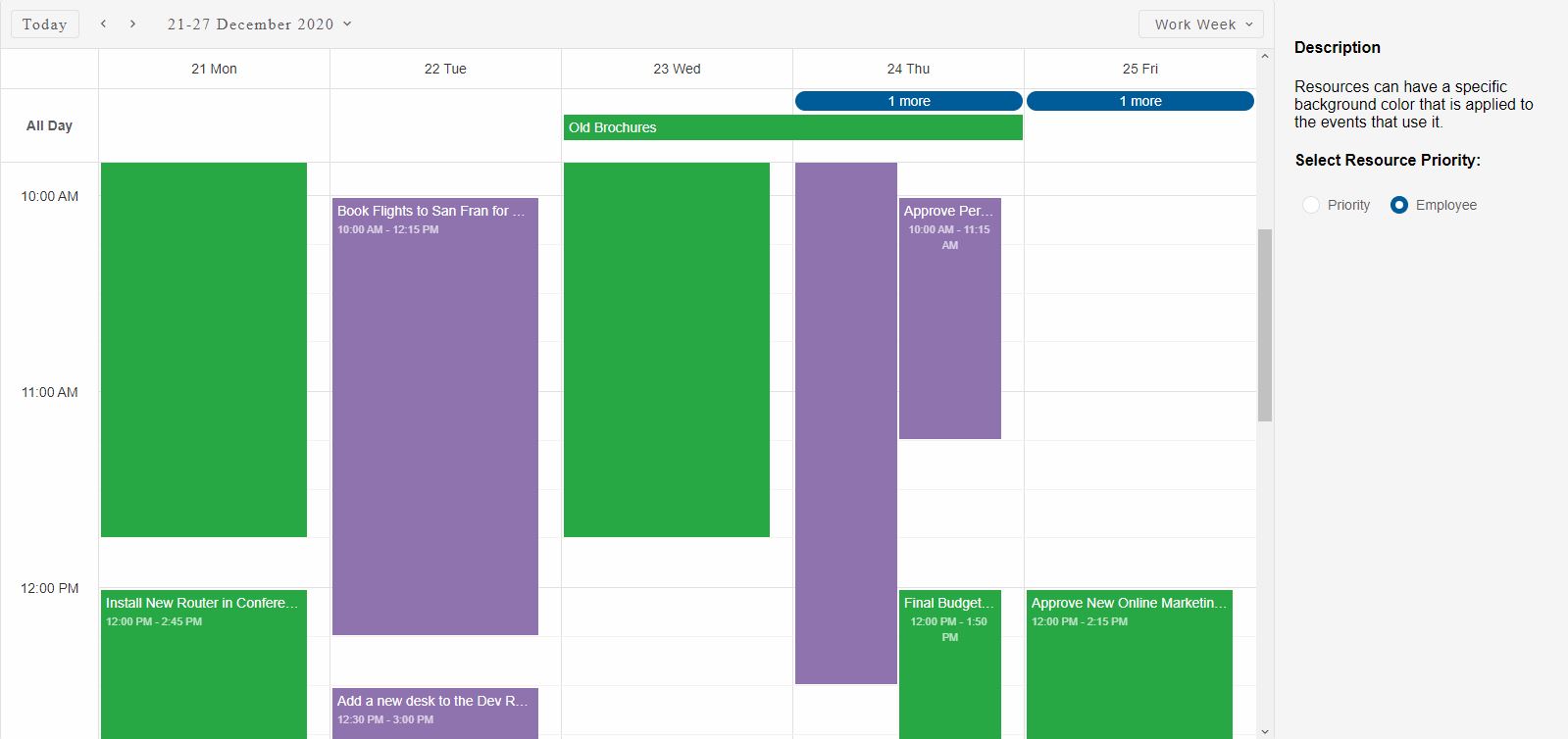
The resource 'employeeId' is prioritirized by setting it as the first item in the groups array and the Events use it's color settings instead.
Scheduler Events can have an unlimited number or resources assigned.
Event Resources can be dynamically changed via the Scheduler Window:
Demo
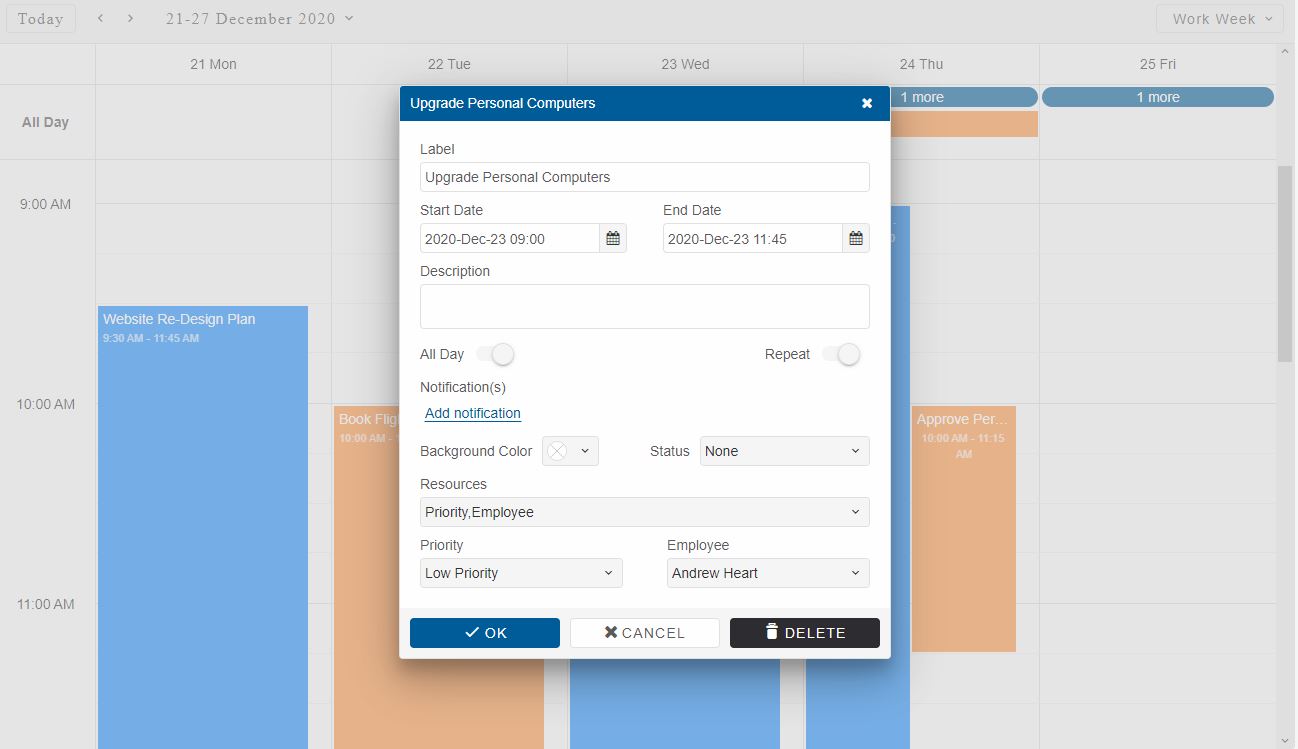
Grouping
Smart.Scheduler grouping is done via the groups property wihch accepts strings that correspond to resource's value property.
The order of the resources in the groups property determine the way the events are grouped which also reflects on the timeline appearance.
The groupOrientation property determines how the Scheduler timeline is oriented. Two orientation options are available: 'horizontal' and 'vertical'.
Horizontal orientation in Agenda view
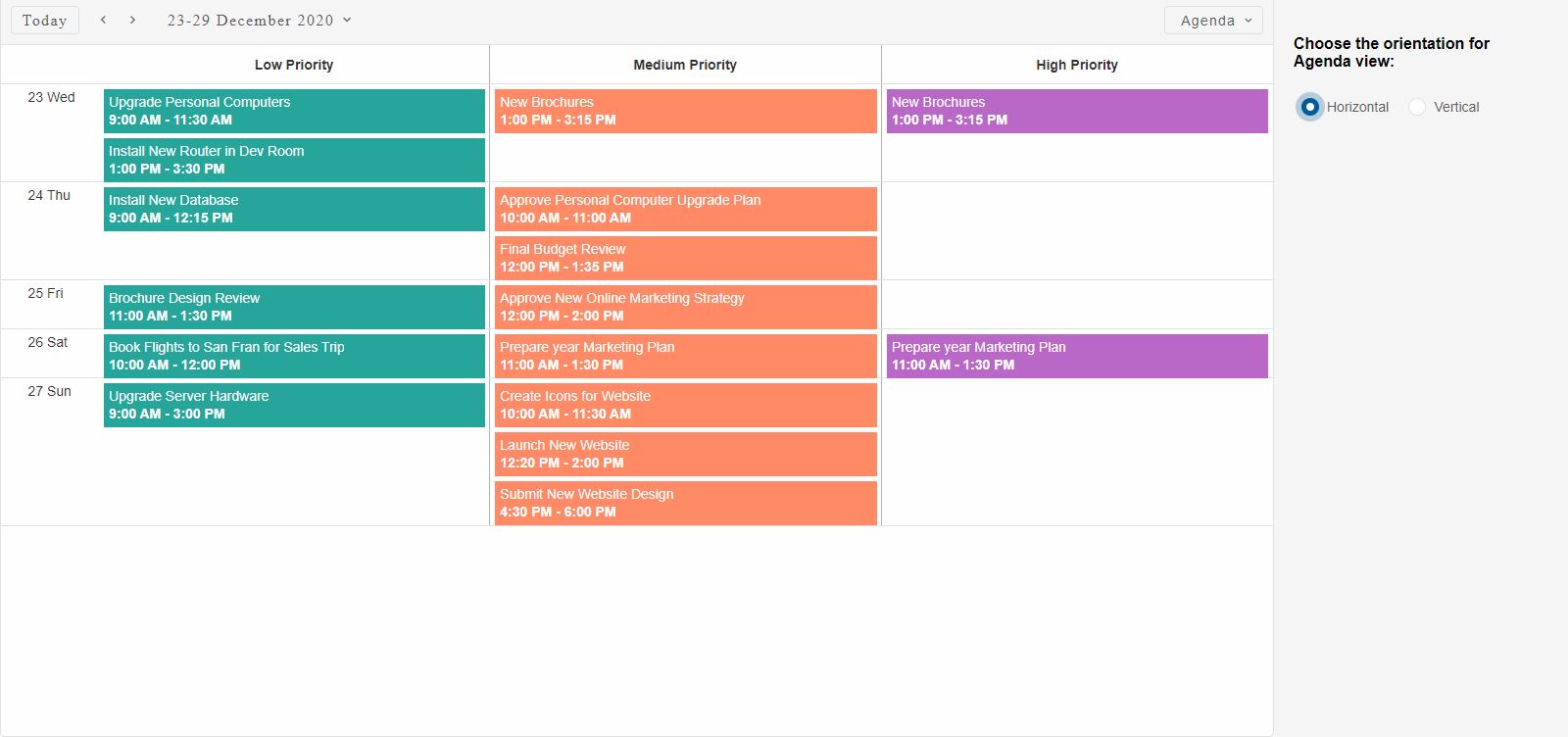
Vertical Orientation in Agenda view
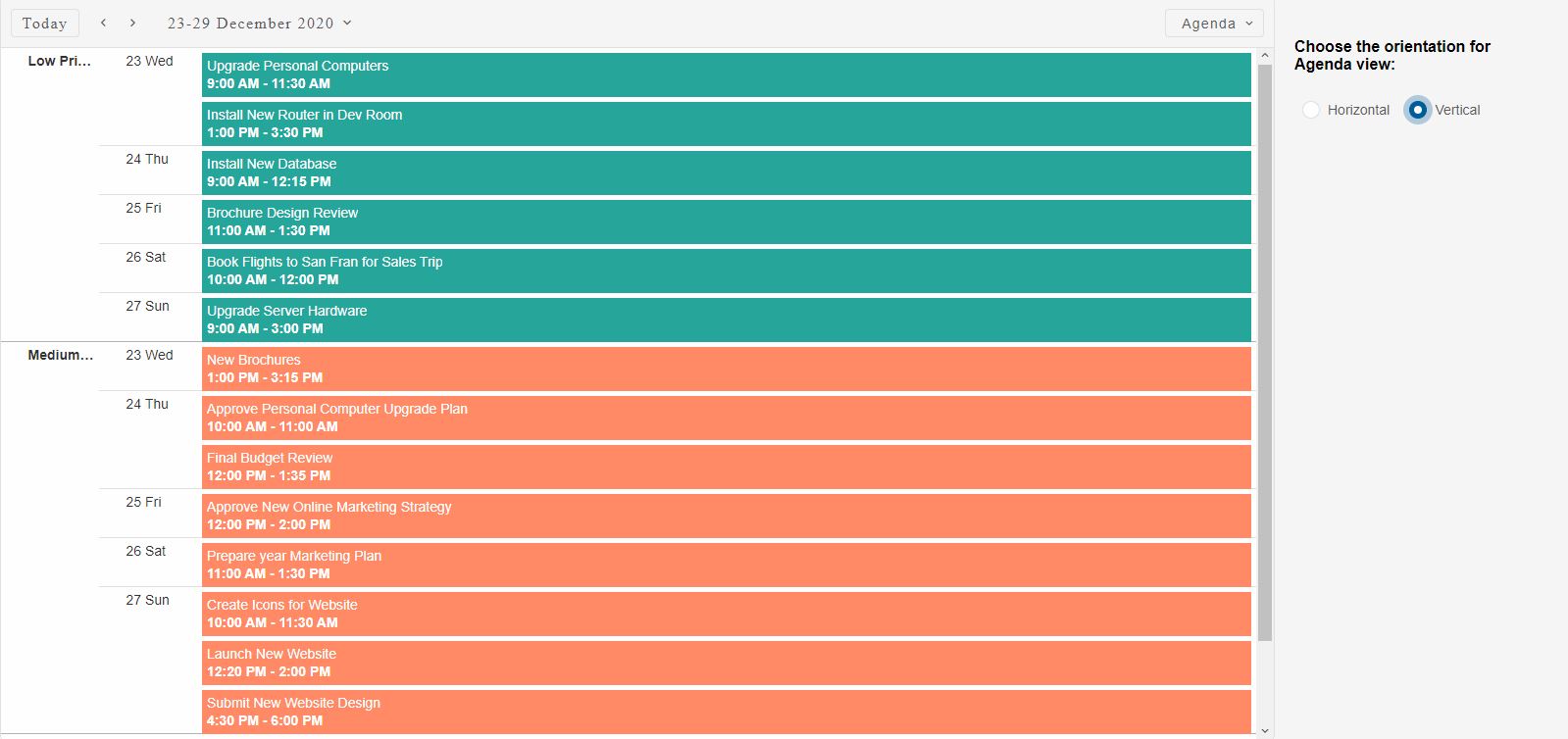
All views of the Smart.Scheduler support grouping with both orientation types.
An additional groupByDate property is available. It allows to group the Events based on the timeline date first and then by their resources:
Group By Date in TimelineDay view:
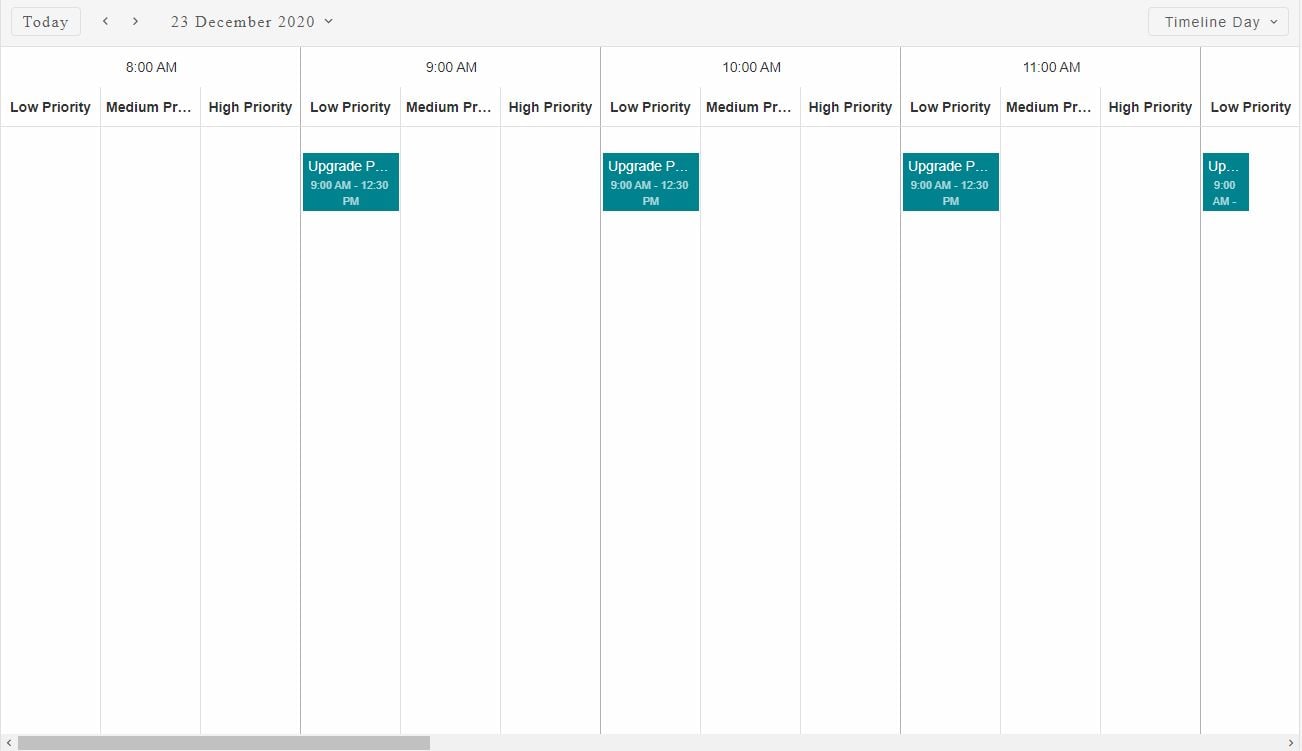
groupByDate only works when groups property is applied.
Smart.Scheduler grouping offers a lot of customizibility.
Here's an example of a Scheduler Day View with horizontal grouping by three resources:
const priorityData = [ { label: 'Low Priority', id: 1, backgroundColor: '#F9A825' }, { label: 'Medium Priority', id: 2, backgroundColor: '#AB47BC' }, { label: 'High Priority', id: 3, backgroundColor: '#4CAF50' } ]; window.Smart('#scheduler', class { get properties() { return { //Properties view: 'dayHorizontal', views: [ { type: 'day', label: 'Day Horizontal', value: 'dayHorizontal', groupOrientation: 'horizontal' }, { type: 'week', label: 'Week Horizontal', value: 'weekHorizontal', groupOrientation: 'horizontal' }, { type: 'month', label: 'Month Horizontal', value: 'monthHorizontal', groupOrientation: 'horizontal' }, { type: 'agenda', label: 'Agenda Horizontal', value: 'agendaHorizontal', groupOrientation: 'horizontal' }, { type: 'day', label: 'Day Vertical' }, { type: 'week', label: 'Week Vertical' }, { type: 'month', label: 'Month Vertical' }], dataSource: dataWithGroups, hourStart: 6, hourEnd: 21, firstDayOfWeek: 1, groupOrientation: 'vertical', groups: ['priorityId'], resources: [ { label: 'Priority', value: 'priorityId', dataSource: priorityData, } ] }; } });
Demo
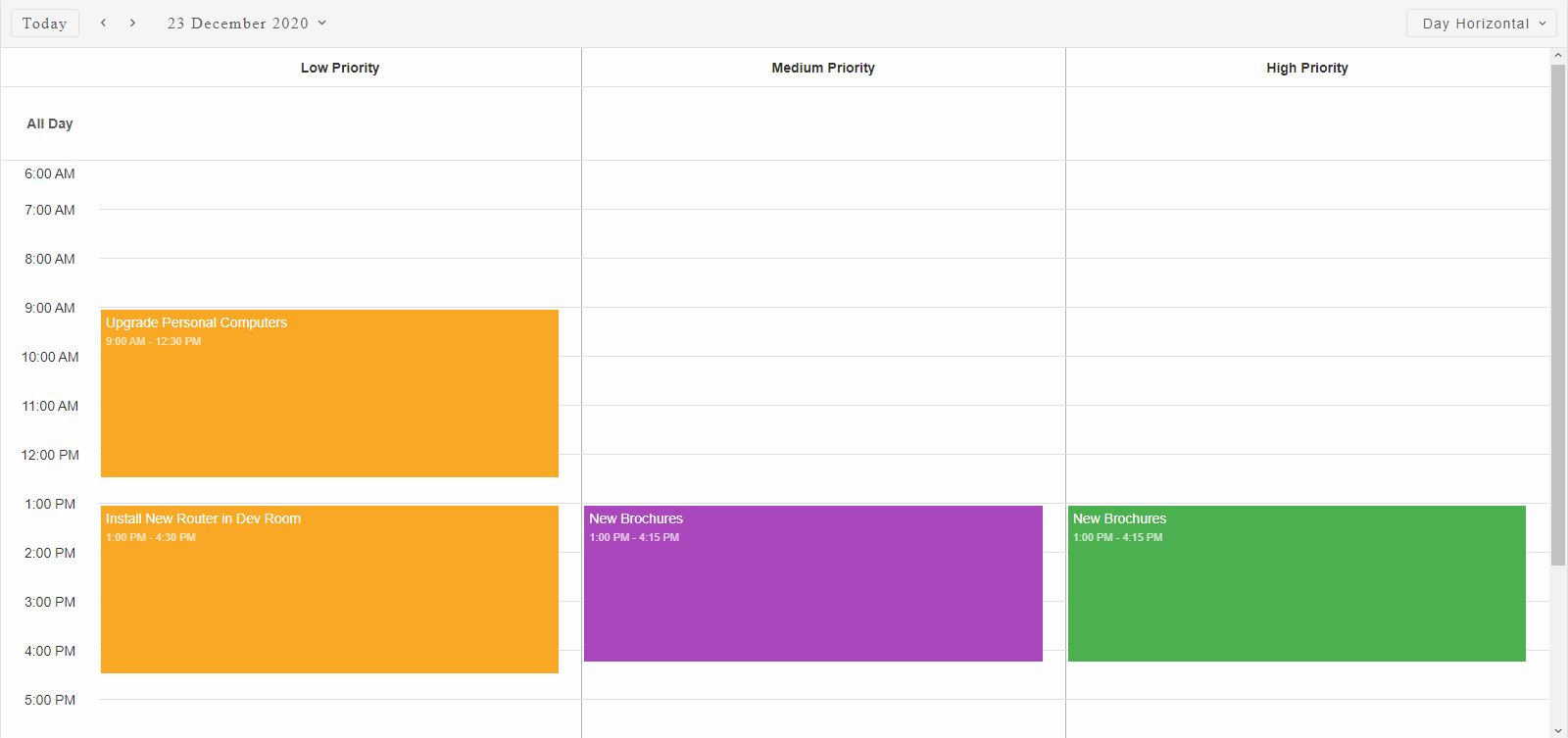
The same example with Scheduler set to Month View with horizontal grouping by three resources:
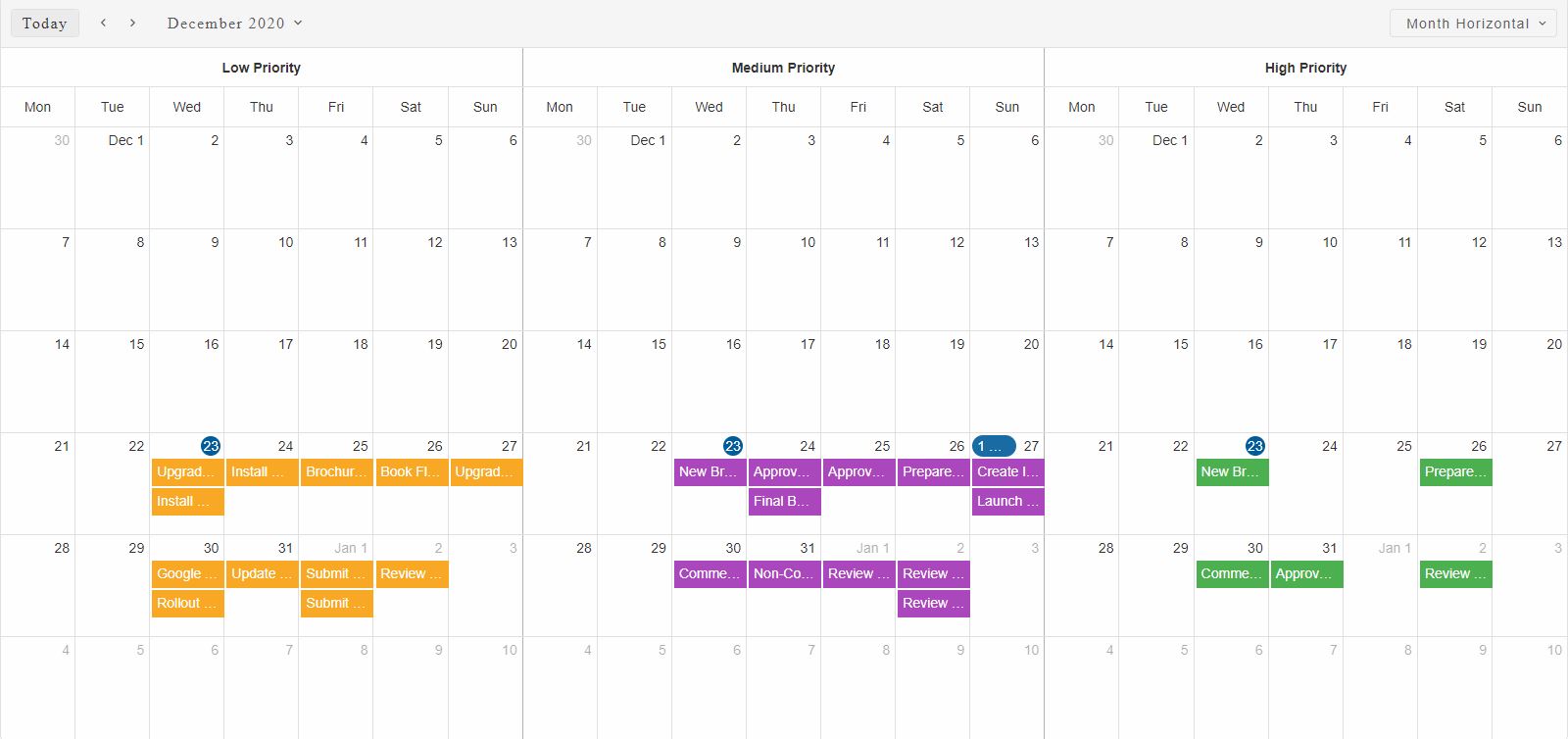
The same example with Scheduler set to Week View with vertical grouping by three resources:
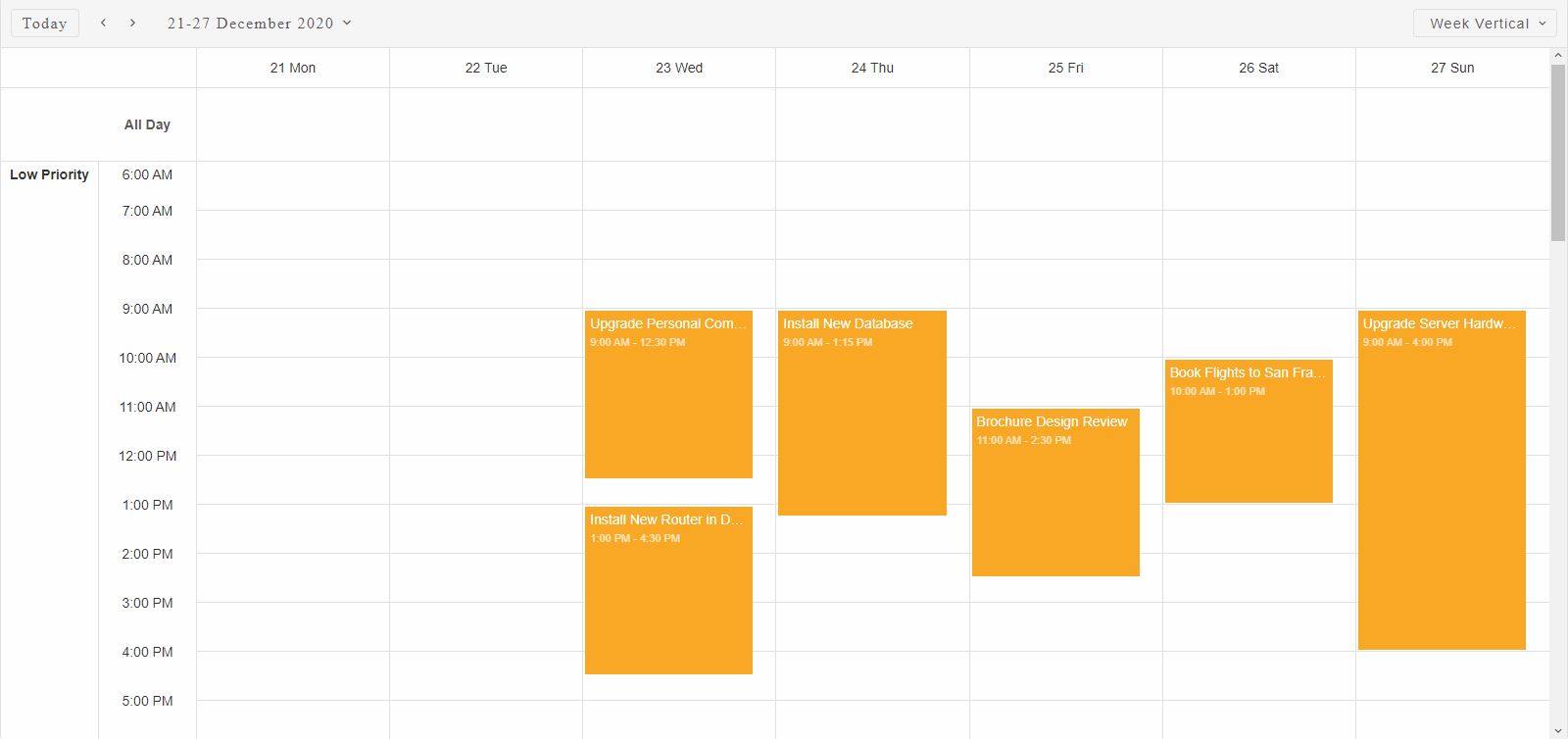
Multiple resource grouping is possible by simply setting the target resources in the groups property.
Here is an example of grouping by three resources simultaneously where each resources has additional three items:
const priorityData = [ { label: 'Low', id: 1, backgroundColor: '#0288D1' }, { label: 'Normal', id: 2, backgroundColor: '#FFB74D' }, { label: 'Critical', id: 3, backgroundColor: '#26A69A' } ]; const heroes = [ { label: 'Tony Stark', id: 1, backgroundColor: '#D32F2F' }, { label: 'Thor', id: 2, backgroundColor: '#7986CB' }, { label: 'Steven Rogers', id: 3, backgroundColor: '#F9A825' }, { label: 'Thanos', id: 4, backgroundColor: '#5D4037' } ]; const movies = [ { label: 'Infinity War', id: 1, backgroundColor: '#FF4081' }, { label: 'Endgame', id: 2, backgroundColor: '#00B0FF' } ]; window.Smart('#scheduler', class { get properties() { return { //Properties view: 'timelineMonth', dateCurrent: '2020-11-1', dataSource: dataWithGroups, views: ['timelineDay', 'timelineWeek', 'timelineMonth'], firstDayOfWeek: 1, hourStart: 6, hourEnd: 20, groups: ['movieId', 'heroId', 'priorityId'], groupOrientation: 'vertical', resources: [ { label: 'Priority', value: 'priorityId', dataSource: priorityData, }, { value: 'heroId', dataSource: heroes, label: 'Employee' }, { value: 'movieId', dataSource: movies, label: 'Movies' } ] }; } });
The events are grouped first by 'movieId', then by 'heroId' and lastly by 'priorityId'.
Demo
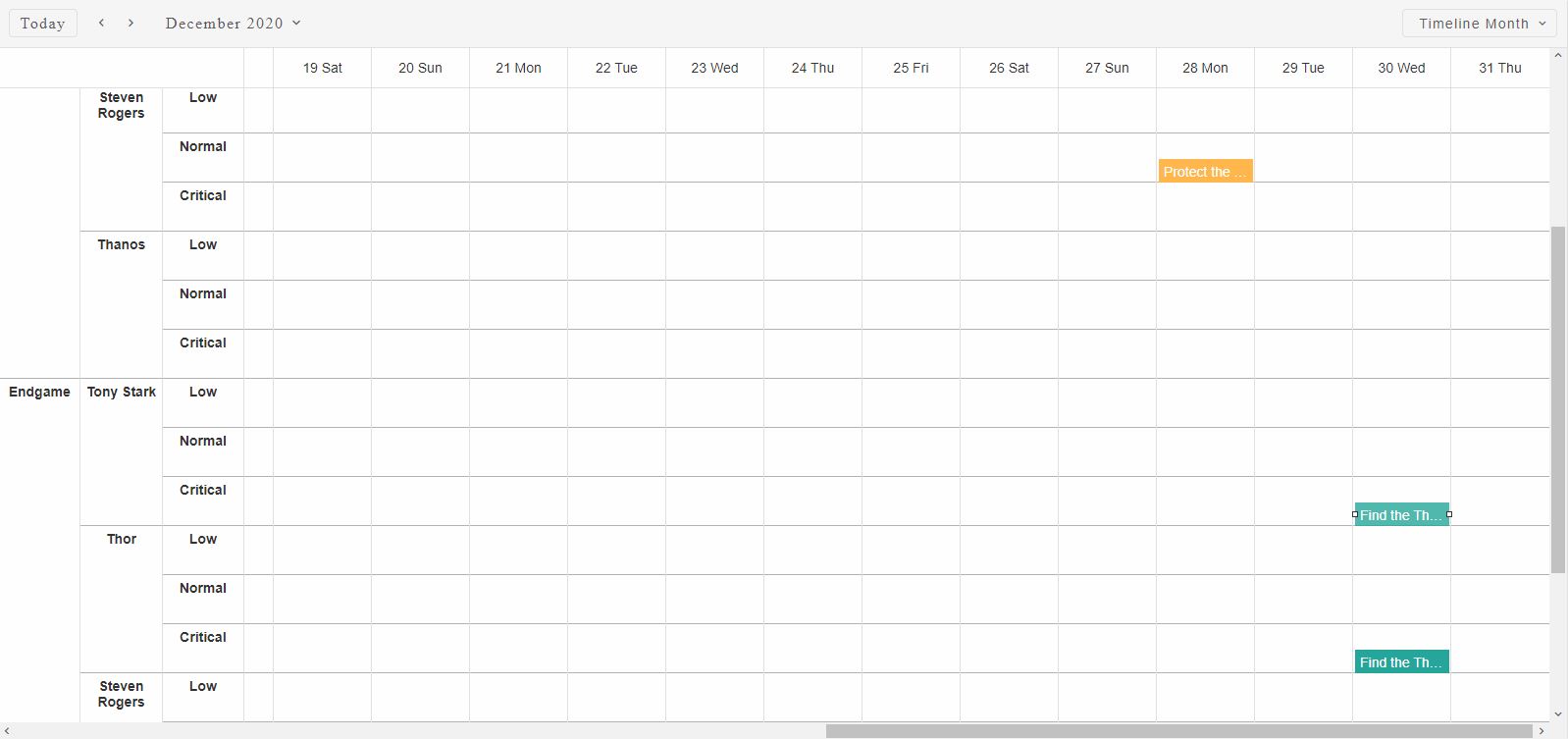
Smart.Scheduler is virtualized and has no limitation on the number of grouping resources.
When grouping is applied, Scheduler Events that do not have resources listed in the groups property will not be displayed.