Blazor - Get Started with Smart.Kanban
Setup The Project
Follow the Getting Started guide to set up your Blazor Application with Smart UI.
Setup Basic Kanban
- Add the Kanban component to the Pages/Index.razor file. Then set its
Columns
andDataSource
property.<Kanban Columns="@columns" DataSource="@cards"></Kanban>
- In the
@code
block declare the columns you want to use in the Kanban. - Then create an example list of tasks.
Use thestatus
property to set the default column or sub-column of the card.
It is also possible to set thepriority
property to either "low", "normal" or "high".@code{ .... IEnumerable<dynamic> cards = new[]{ new{id=1, text="Editing", status="ToDo", priority="high"}, new{id=1, text="Header", status="ToDo", priority="normal"}, new{id=1, text="Dragging with feedback", status="ToDo", priority="low"}, new{id=1, text="Vertical virtualization", status="ToDo", priority="high"}, new{id=1, text="Observable columns array", status="ToDo", priority="normal"}, new{id=1, text="Infinite scrolling", status="InProgress", priority="high"}, new{id=1, text="Visible/hidden columns", status="InProgress", priority="low"}, new{id=1, text="Public methods", status="InProgress", priority="high"}, new{id=1, text="Expand/collapse arrow", status="Testing", priority="low"}, new{id=1, text="Virtual scrolling", status="Testing", priority="high"}, new{id=1, text="Deferred scrolling", status="Testing", priority="normal"}, new{id=1, text="Research", status="Done", priority="normal"}, new{id=1, text="Displaying data from data source", status="Done", priority="normal"}, new{id=1, text="Showing cover and title", status="Done", priority="normal"}, new{id=1, text="Property validation", status="Done", priority="normal"}, new{id=1, text="formatFunction and formatSettings", status="Done", priority="normal"}, }; }
By default, you can Drag & Drop Cards into the different columns.
@code{ List<KanbanColumn> columns = new List<KanbanColumn>() { new KanbanColumn() { DataField = "ToDo", Label = "To do", }, new KanbanColumn() { DataField = "InProgress", Label = "In progress" }, new KanbanColumn() { DataField = "Testing", Label = "Testing" }, new KanbanColumn() { DataField = "Done", Label = "Done" } }
Card Progress
Smart.Kanban allows you to set the progress value of individual tasks on a scale of 0 - 100.
In the component, set the TaskProgress
property. Then modify the DataSource array to include progress values.
<Kanban Columns="@columns" dataSource="@cards" TaskProgress="true"></Kanban> @code{ .... IEnumerable<dynamic> cards = new[]{ new{id=1, text="Editing", status="ToDo", priority="high", progress=5,}, new{id=1, text="Header", status="ToDo", priority="normal", progress=10}, new{id=1, text="Dragging with feedback", status="ToDo", priority="low", progress=30}, new{id=1, text="Vertical virtualization", status="ToDo", priority="high", progress=15}, new{id=1, text="Observable columns array", status="ToDo", priority="normal", progress=20}, new{id=1, text="Infinite scrolling", status="InProgress", priority="high", progress=45}, new{id=1, text="Visible/hidden columns", status="InProgress", priority="low", progress=60}, new{id=1, text="Public methods", status="InProgress", priority="high", progress=20}, new{id=1, text="Expand/collapse arrow", status="Testing", priority="low", progress=85}, new{id=1, text="Virtual scrolling", status="Testing", priority="high", progress=70}, new{id=1, text="Deferred scrolling", status="Testing", priority="normal", progress=90}, new{id=1, text="Research", status="Done", priority="normal", progress=100}, new{id=1, text="Displaying data from data source", status="Done", priority="normal", progress=100}, new{id=1, text="Showing cover and title", status="Done", priority="normal", progress=100}, new{id=1, text="Property validation", status="Done", priority="normal", progress=100}, new{id=1, text="formatFunction and formatSettings", status="Done", priority="normal", progress=100}, }; }
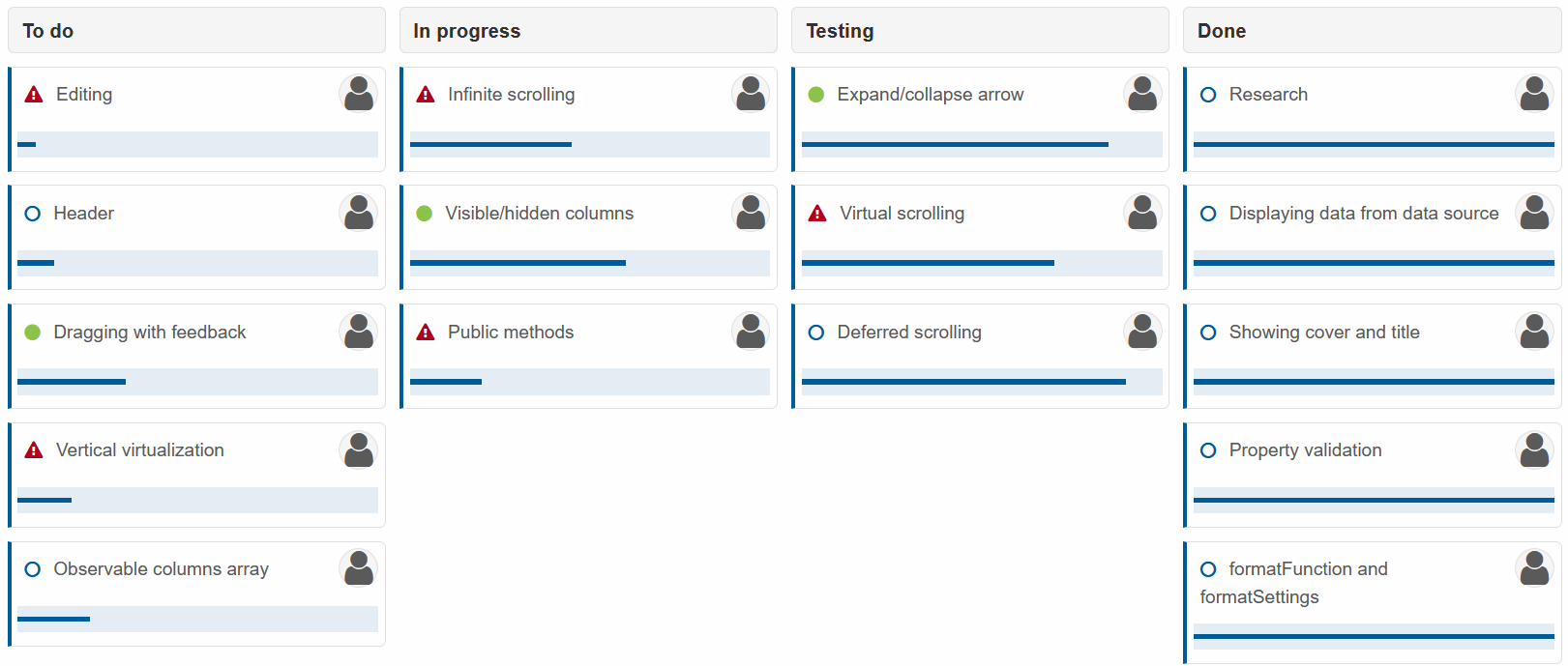
Adding and Editing Tasks
Smart.Kanban has built-in easy-to-use modal menus, which allow the users to create and edit tasks.
To allow Editing, set the Editable
property to true.
To create a button, which creates new tasks, set the AddNewButton
property to true.
<Kanban Columns="@columns" dataSource="@cards" TaskProgress="true" Editable="true" AddNewButton="true"></Kanban>
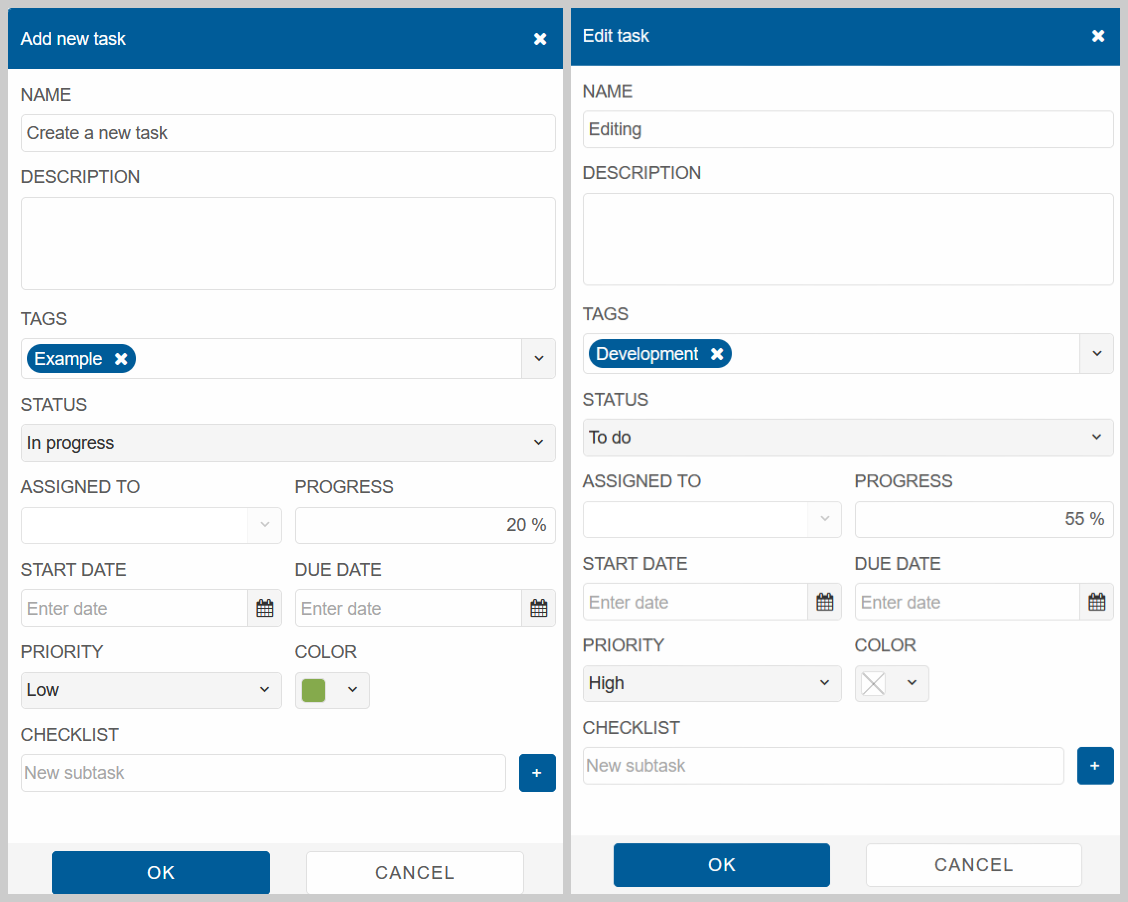
Kanban Filter & Sort
The Kanban Header allows you to easily Customize, Filter, Sort and Search the cards in a variety of ways.
In the @code
block set the position of the Header and then set the HeaderPosition
of the Kanban.
<Kanban Columns="@columns" dataSource="@cards" TaskProgress="true" Editable="true" AddNewButton="true" HeaderPosition="@headerPosition"></Kanban> @code{ KanbanHeaderPosition headerPosition = KanbanHeaderPosition.Top; .... }
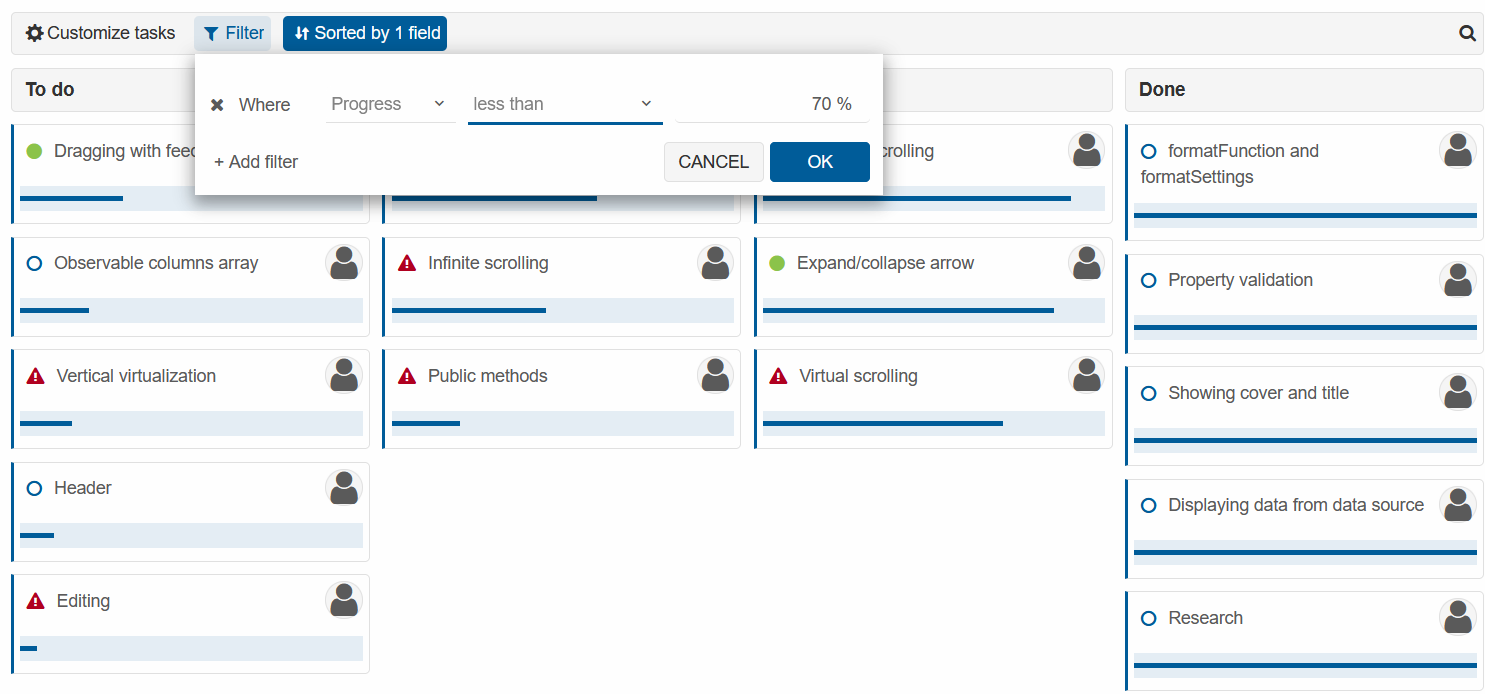
Kanban Swimlanes
The Kanban Swimlanes separate different type of work activities and classes of service.
You may have as many swimlanes as you want in order to organize your tasks. Declare the swimlanes you want to use.
<Kanban Columns="@columns" dataSource="@cards" TaskProgress="true" Editable="true" AddNewButton="true" HeaderPosition="@headerPosition" Swimlanes="@swimlanes"></Kanban> @code{ List <KanbanSwimlane> swimlanes = new List <KanbanSwimlane>(){ new KanbanSwimlane(){ Label = "New Features", DataField = "newFeatures" }, new KanbanSwimlane(){ Label = "Improvements", DataField = "improvements", Color = "#C90086" } }; .... }
Modify the dataSource by attaching the swimlanes to the appropirate tasks via the swimlane
property.
IEnumerable <dynamic> cards = new[]{ new{id=1, text="Editing", status="ToDo", priority="high", progress=5, swimlane="improvements"}, new{id=1, text="Header", status="ToDo", priority="normal", progress=10, swimlane="newFeatures"}, new{id=1, text="Dragging with feedback", status="ToDo", priority="low", progress=30, swimlane="newFeatures"}, new{id=1, text="Vertical virtualization", status="ToDo", priority="high", progress=15, swimlane="newFeatures"}, new{id=1, text="Observable columns array", status="ToDo", priority="normal", progress=20, swimlane="newFeatures"}, new{id=1, text="Infinite scrolling", status="InProgress", priority="high", progress=45, swimlane="improvements"}, new{id=1, text="Visible/hidden columns", status="InProgress", priority="low", progress=60, swimlane="newFeatures"}, new{id=1, text="Public methods", status="InProgress", priority="high", progress=20, swimlane="improvements"}, new{id=1, text="Expand/collapse arrow", status="Testing", priority="low", progress=85, swimlane="newFeatures"}, new{id=1, text="Virtual scrolling", status="Testing", priority="high", progress=70, swimlane="improvements"}, new{id=1, text="Deferred scrolling", status="Testing", priority="normal", progress=90, swimlane="improvements"}, new{id=1, text="Research", status="Done", priority="normal", progress=100, swimlane="improvements"}, new{id=1, text="Displaying data from data source", status="Done", priority="normal", progress=100, swimlane="newFeatures"}, new{id=1, text="Showing cover and title", status="Done", priority="normal", progress=100, swimlane="newFeatures"}, new{id=1, text="Property validation", status="Done", priority="normal", progress=100, swimlane="improvements"}, new{id=1, text="formatFunction and formatSettings", status="Done", priority="normal", progress=100, swimlane="newFeatures"}, };
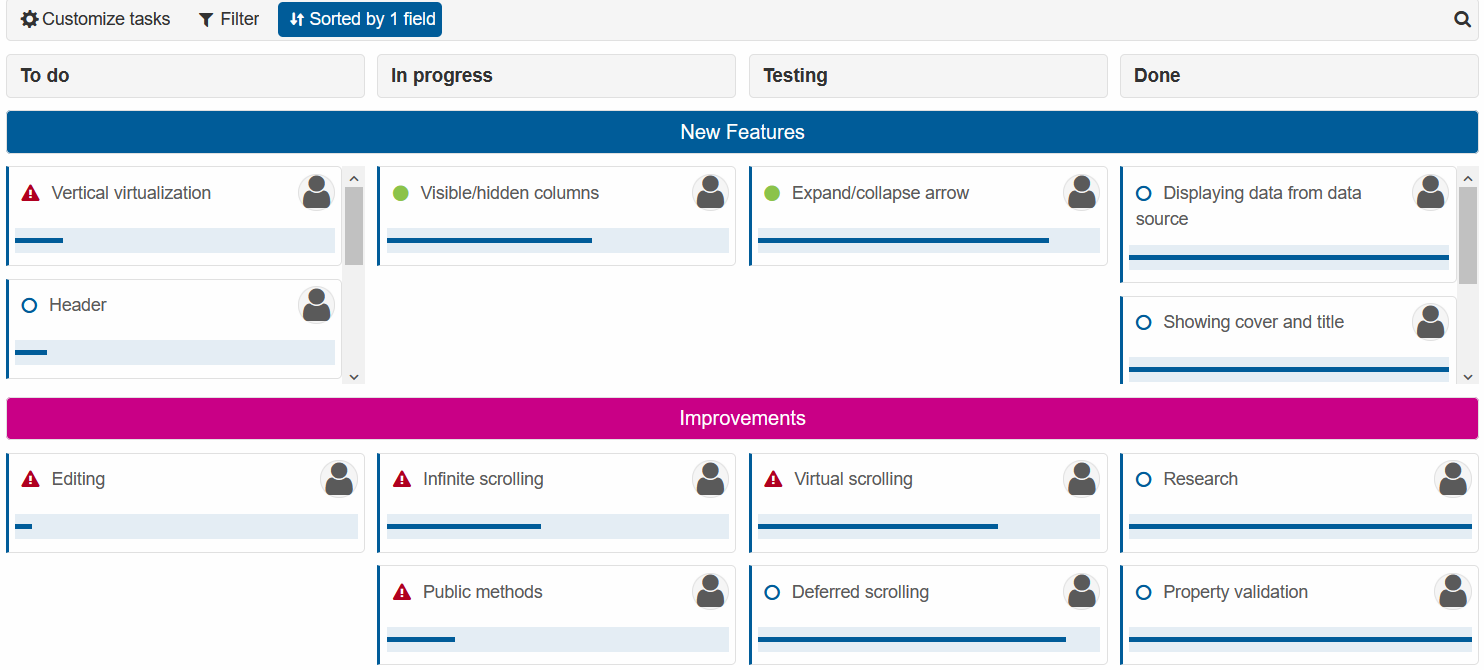